LayoutSubViews - 1
2016-01-26 11:05
489 查看
首先感谢写出这么好文章的大神:
http://bachiscoding.com/blog/2014/12/15/when-will-layoutsubviews-be-invoked/ http://www.cnblogs.com/pengyingh/articles/2417211.html
- (void)layoutSubviews;
来看看官方文档对这个方法的说明:
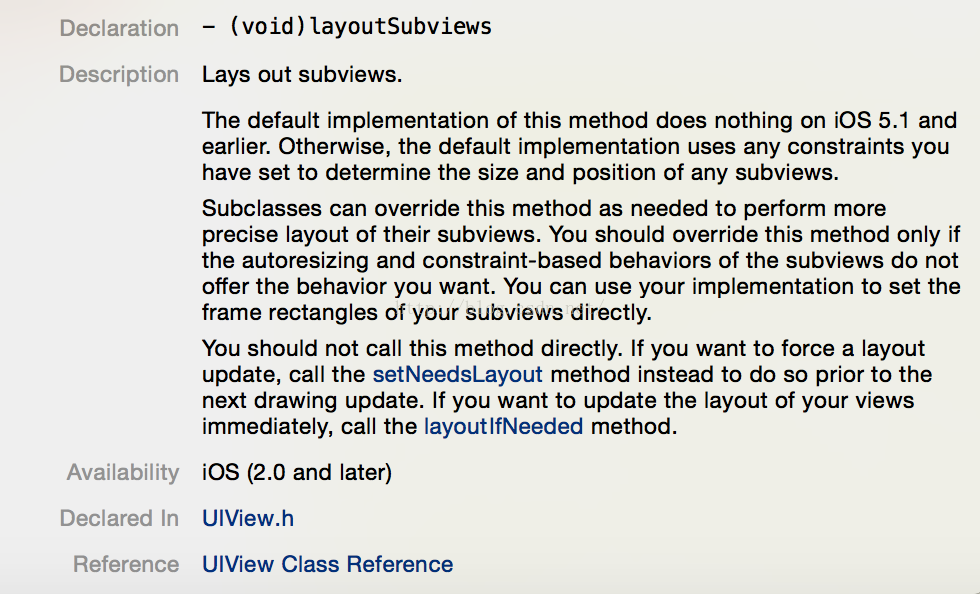
顾名思义,这个方式是对于subView的布局、大小等一些更新式,响应变化式的操作,上面提到另外两个方法:setNeedsLayout 和 layoutIfNeeded,我们先不讨论这额外的两个方法,我们单纯从方面的功能入手
开门见山,触发这个方法的几个最常用的(很多需要注意的地方,请移步大神博客,讲得非常好,本人的原则是在项目实际中遇到后才去甄别)
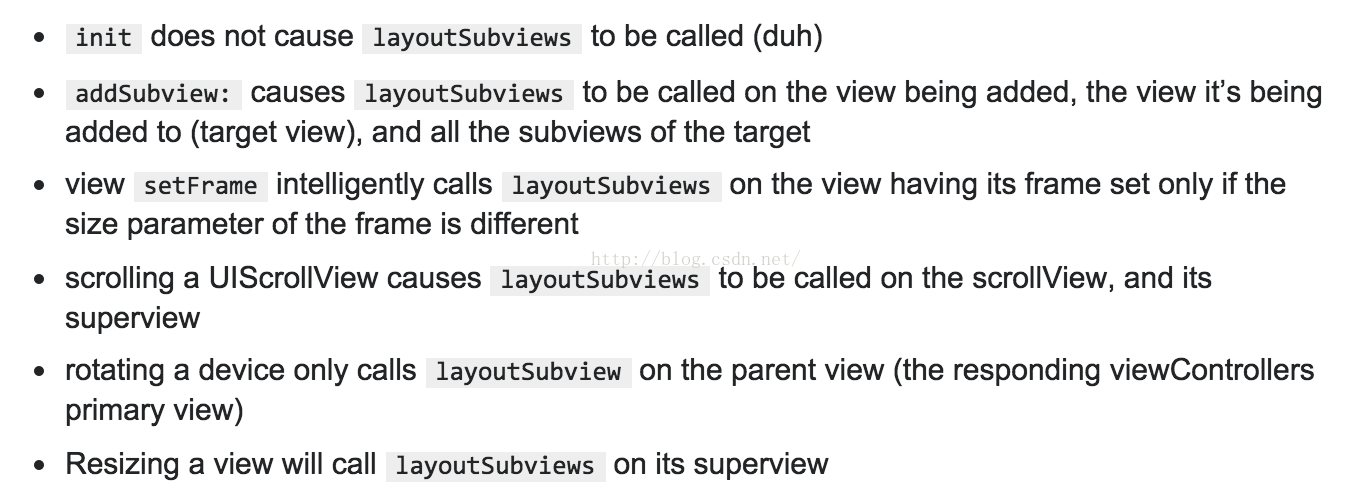
上面的几个情况我都亲自代码验证过了!参考第二篇文章,写了一个随屏幕布局变化的例子:
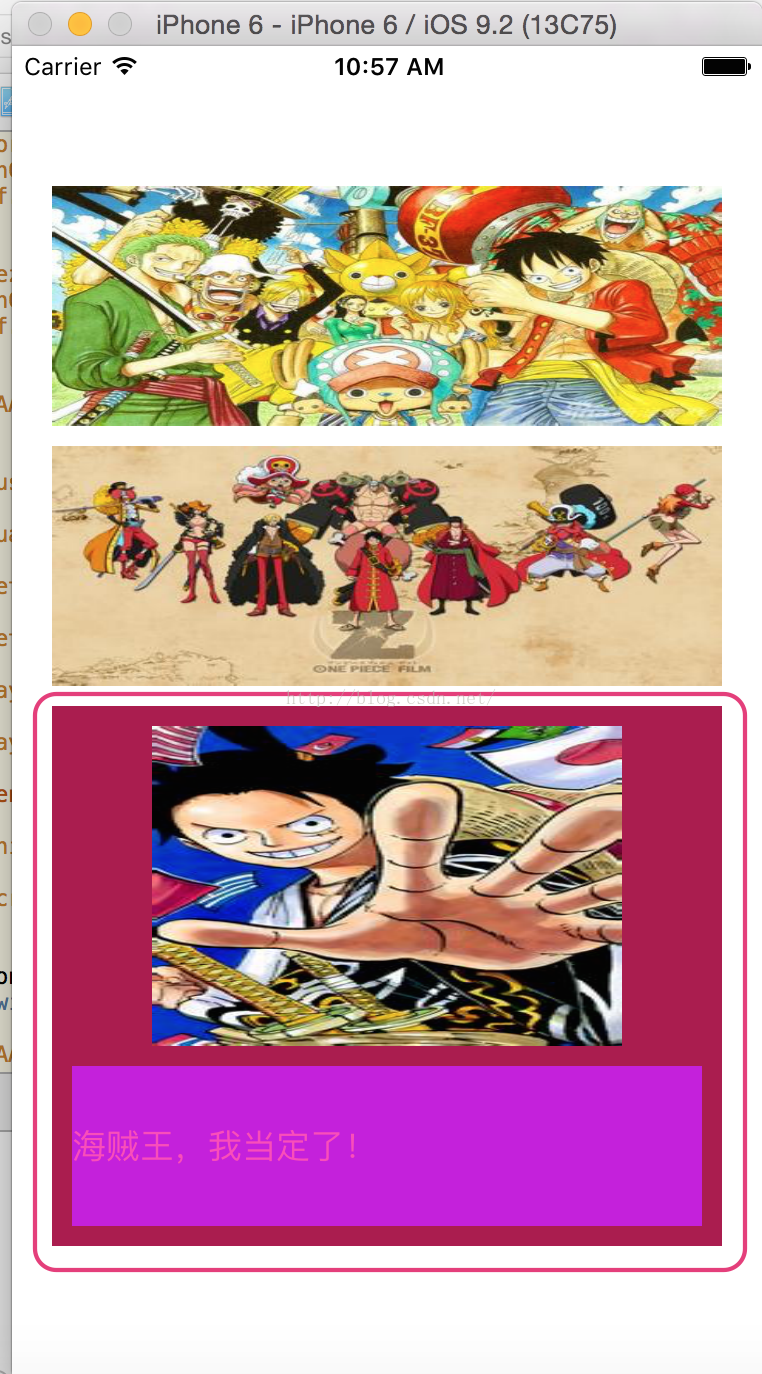
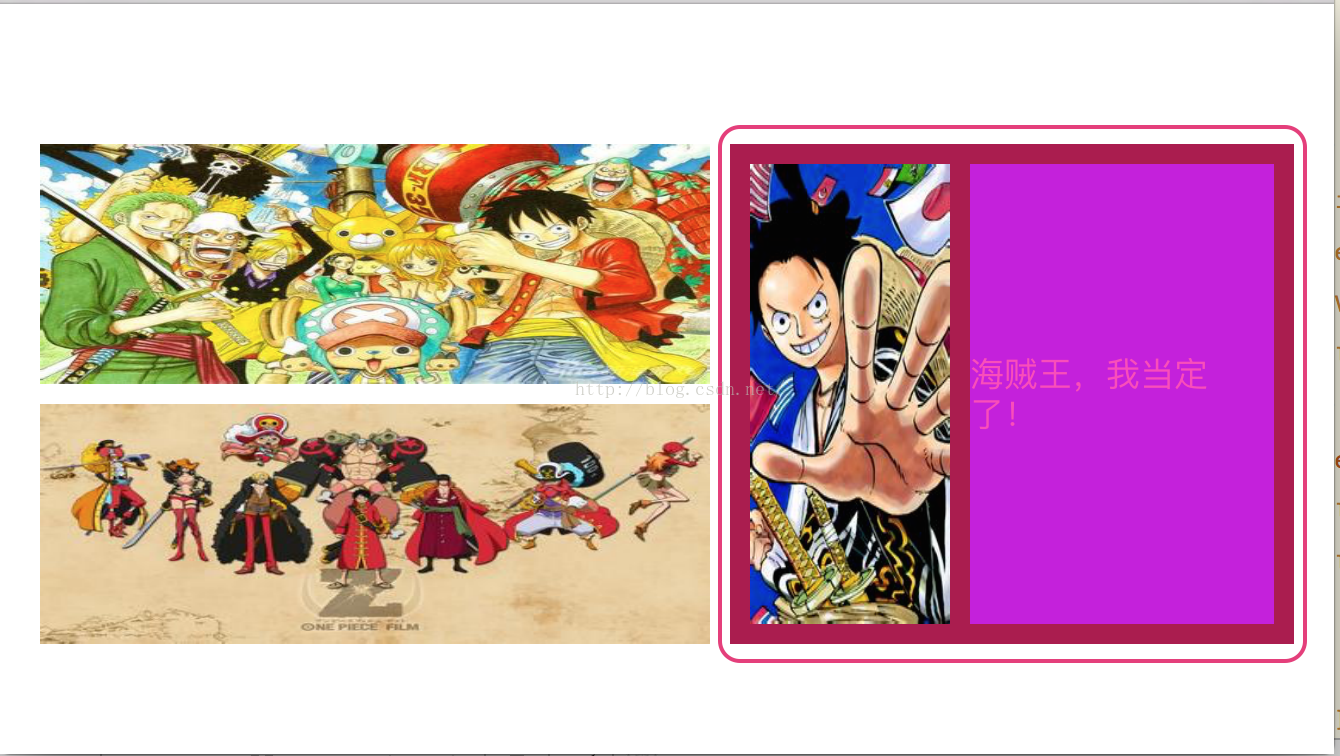
可以看到随屏幕方向不同,两张大图可以自己适应,但是红色框标出的自定义视图内部子视图发生了布局的变化,代码:
头文件:
@interface LBOrientationLayoutViewController : UIViewController
@end
#pragma mark - LBDynamicLayoutView
@interface LBDynamicLayoutView: UIView
@property (nonatomic, strong) UIImageView* iconImageView;
@property (nonatomic, strong) UILabel* profileLabel;
@end
实现文件:
#import "UIColor+RandomColor.h"
#import "LBOrientationLayoutViewController.h"
@interface LBOrientationLayoutViewController ()
@property (nonatomic, strong) LBDynamicLayoutView* dynamicLayoutView;
@property (nonatomic, strong) UIImageView* stuffImageView;
@property (nonatomic, strong) UIImageView* coolImageView;
@end
@implementation LBOrientationLayoutViewController
- (void)viewDidLoad
{
[super viewDidLoad];
[[UIDevice currentDevice] beginGeneratingDeviceOrientationNotifications];
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(handleOrientationDidChange:) name:UIDeviceOrientationDidChangeNotification object:nil];
self.stuffImageView = [[UIImageView alloc] init];
[self.stuffImageView setFrame:CGRectMake(20, 20 + 50, self.view.frame.size.width - 40, 120)];
[self.stuffImageView setImage:[UIImage imageNamed:@"stuff.jpg"]];
[self.view addSubview:self.stuffImageView];
self.coolImageView = [[UIImageView alloc] init];
[self.coolImageView setFrame:CGRectMake(20,
self.stuffImageView.frame.origin.y + self.stuffImageView.frame.size.height + 10,
self.view.frame.size.width - 40,
250 - 10 - 120)];
[self.coolImageView setImage:[UIImage imageNamed:@"cool.jpg"]];
[self.view addSubview:self.coolImageView];
CGRect rect = CGRectMake(20,
self.coolImageView.frame.origin.y + self.coolImageView.frame.size.height + 10,
self.view.frame.size.width - 40,
270);
self.dynamicLayoutView = [[LBDynamicLayoutView alloc] initWithFrame:rect];
[self.dynamicLayoutView.profileLabel setText:@"海贼王,我当定了!"];
[self.view addSubview:self.dynamicLayoutView];
}
- (void)handleOrientationDidChange:(id)sender
{
UIInterfaceOrientation interfaceOrientation = [[UIApplication sharedApplication] statusBarOrientation];
if (interfaceOrientation == UIInterfaceOrientationPortrait || interfaceOrientation == UIInterfaceOrientationPortraitUpsideDown)
{
CGRect rect = CGRectMake(20,
self.coolImageView.frame.origin.y + self.coolImageView.frame.size.height + 10,
self.view.frame.size.width - 40,
270);
[self.dynamicLayoutView setFrame:rect];
}
else if (interfaceOrientation == UIInterfaceOrientationLandscapeLeft || interfaceOrientation == UIInterfaceOrientationLandscapeRight)
{
CGRect rect = CGRectMake(self.stuffImageView.frame.origin.x + self.stuffImageView.frame.size.width + 10,
self.stuffImageView.frame.origin.y,
self.view.frame.size.width - self.stuffImageView.frame.origin.x - self.coolImageView.frame.size.width - 10 - 20,
self.stuffImageView.frame.size.height + 10 + self.coolImageView.frame.size.height);
[self.dynamicLayoutView setFrame:rect];
}
}
@end
#pragma mark - LBDynamicLayoutView
@implementation LBDynamicLayoutView
- (id)initWithFrame:(CGRect)frame
{
self = [super initWithFrame:frame];
if (self)
{
self.backgroundColor = [UIColor randomColor];
self.autoresizesSubviews = YES;
self.iconImageView = [[UIImageView alloc] init];
[self.iconImageView setFrame:CGRectMake(50, 10, self.frame.size.width - 100, 160)];
[self.iconImageView setBackgroundColor:[UIColor randomColor]];
[self.iconImageView setImage:[UIImage imageNamed:@"luffy.jpg"]];
[self addSubview:self.iconImageView];
self.profileLabel = [[UILabel alloc] init];
[self.profileLabel setFrame:CGRectMake(10, self.iconImageView.frame.origin.y + self.iconImageView.frame.size.height + 10, self.frame.size.width - 20, 80)];
[self.profileLabel setBackgroundColor:[UIColor randomColor]];
[self.profileLabel setTextColor:[UIColor randomColor]];
[self.profileLabel setNumberOfLines:0];
[self addSubview:self.profileLabel];
}
return self;
}
- (void)layoutSubviews
{
[super layoutSubviews];
UIInterfaceOrientation interfaceOrientation = [[UIApplication sharedApplication] statusBarOrientation];
if (interfaceOrientation == UIInterfaceOrientationPortrait || interfaceOrientation == UIInterfaceOrientationPortraitUpsideDown)
{
[self setVerticalFrame];
}
else if (interfaceOrientation == UIInterfaceOrientationLandscapeLeft || interfaceOrientation == UIInterfaceOrientationLandscapeRight)
{
[self setHorizontalFrame];
}
}
- (void)setVerticalFrame
{
NSLog(@"竖屏");
CGRect rect0 = CGRectMake(50, 10, self.frame.size.width - 100, 160);
[self.iconImageView setFrame:rect0];
CGRect rect1 = CGRectMake(10, self.iconImageView.frame.origin.y + self.iconImageView.frame.size.height + 10, self.frame.size.width - 20, 80);
[self.profileLabel setFrame:rect1];
}
- (void)setHorizontalFrame
{
NSLog(@"横屏");
CGRect rect0 = CGRectMake(10, 10, 100, self.frame.size.height - 20);
[self.iconImageView setFrame:rect0];
CGRect rect1 = CGRectMake(self.iconImageView.frame.origin.x + self.iconImageView.frame.size.width + 10,
10,
self.frame.size.width - self.iconImageView.frame.origin.x - self.iconImageView.frame.size.width - 10 - 10,
self.frame.size.height - 20);
[self.profileLabel setFrame:rect1];
}
@end
参考:
http://bachiscoding.com/blog/2014/12/15/when-will-layoutsubviews-be-invoked/ http://www.cnblogs.com/pengyingh/articles/2417211.html http://stackoverflow.com/questions/728372/when-is-layoutsubviews-called
http://bachiscoding.com/blog/2014/12/15/when-will-layoutsubviews-be-invoked/ http://www.cnblogs.com/pengyingh/articles/2417211.html
- (void)layoutSubviews;
来看看官方文档对这个方法的说明:
顾名思义,这个方式是对于subView的布局、大小等一些更新式,响应变化式的操作,上面提到另外两个方法:setNeedsLayout 和 layoutIfNeeded,我们先不讨论这额外的两个方法,我们单纯从方面的功能入手
开门见山,触发这个方法的几个最常用的(很多需要注意的地方,请移步大神博客,讲得非常好,本人的原则是在项目实际中遇到后才去甄别)
上面的几个情况我都亲自代码验证过了!参考第二篇文章,写了一个随屏幕布局变化的例子:
可以看到随屏幕方向不同,两张大图可以自己适应,但是红色框标出的自定义视图内部子视图发生了布局的变化,代码:
头文件:
@interface LBOrientationLayoutViewController : UIViewController
@end
#pragma mark - LBDynamicLayoutView
@interface LBDynamicLayoutView: UIView
@property (nonatomic, strong) UIImageView* iconImageView;
@property (nonatomic, strong) UILabel* profileLabel;
@end
实现文件:
#import "UIColor+RandomColor.h"
#import "LBOrientationLayoutViewController.h"
@interface LBOrientationLayoutViewController ()
@property (nonatomic, strong) LBDynamicLayoutView* dynamicLayoutView;
@property (nonatomic, strong) UIImageView* stuffImageView;
@property (nonatomic, strong) UIImageView* coolImageView;
@end
@implementation LBOrientationLayoutViewController
- (void)viewDidLoad
{
[super viewDidLoad];
[[UIDevice currentDevice] beginGeneratingDeviceOrientationNotifications];
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(handleOrientationDidChange:) name:UIDeviceOrientationDidChangeNotification object:nil];
self.stuffImageView = [[UIImageView alloc] init];
[self.stuffImageView setFrame:CGRectMake(20, 20 + 50, self.view.frame.size.width - 40, 120)];
[self.stuffImageView setImage:[UIImage imageNamed:@"stuff.jpg"]];
[self.view addSubview:self.stuffImageView];
self.coolImageView = [[UIImageView alloc] init];
[self.coolImageView setFrame:CGRectMake(20,
self.stuffImageView.frame.origin.y + self.stuffImageView.frame.size.height + 10,
self.view.frame.size.width - 40,
250 - 10 - 120)];
[self.coolImageView setImage:[UIImage imageNamed:@"cool.jpg"]];
[self.view addSubview:self.coolImageView];
CGRect rect = CGRectMake(20,
self.coolImageView.frame.origin.y + self.coolImageView.frame.size.height + 10,
self.view.frame.size.width - 40,
270);
self.dynamicLayoutView = [[LBDynamicLayoutView alloc] initWithFrame:rect];
[self.dynamicLayoutView.profileLabel setText:@"海贼王,我当定了!"];
[self.view addSubview:self.dynamicLayoutView];
}
- (void)handleOrientationDidChange:(id)sender
{
UIInterfaceOrientation interfaceOrientation = [[UIApplication sharedApplication] statusBarOrientation];
if (interfaceOrientation == UIInterfaceOrientationPortrait || interfaceOrientation == UIInterfaceOrientationPortraitUpsideDown)
{
CGRect rect = CGRectMake(20,
self.coolImageView.frame.origin.y + self.coolImageView.frame.size.height + 10,
self.view.frame.size.width - 40,
270);
[self.dynamicLayoutView setFrame:rect];
}
else if (interfaceOrientation == UIInterfaceOrientationLandscapeLeft || interfaceOrientation == UIInterfaceOrientationLandscapeRight)
{
CGRect rect = CGRectMake(self.stuffImageView.frame.origin.x + self.stuffImageView.frame.size.width + 10,
self.stuffImageView.frame.origin.y,
self.view.frame.size.width - self.stuffImageView.frame.origin.x - self.coolImageView.frame.size.width - 10 - 20,
self.stuffImageView.frame.size.height + 10 + self.coolImageView.frame.size.height);
[self.dynamicLayoutView setFrame:rect];
}
}
@end
#pragma mark - LBDynamicLayoutView
@implementation LBDynamicLayoutView
- (id)initWithFrame:(CGRect)frame
{
self = [super initWithFrame:frame];
if (self)
{
self.backgroundColor = [UIColor randomColor];
self.autoresizesSubviews = YES;
self.iconImageView = [[UIImageView alloc] init];
[self.iconImageView setFrame:CGRectMake(50, 10, self.frame.size.width - 100, 160)];
[self.iconImageView setBackgroundColor:[UIColor randomColor]];
[self.iconImageView setImage:[UIImage imageNamed:@"luffy.jpg"]];
[self addSubview:self.iconImageView];
self.profileLabel = [[UILabel alloc] init];
[self.profileLabel setFrame:CGRectMake(10, self.iconImageView.frame.origin.y + self.iconImageView.frame.size.height + 10, self.frame.size.width - 20, 80)];
[self.profileLabel setBackgroundColor:[UIColor randomColor]];
[self.profileLabel setTextColor:[UIColor randomColor]];
[self.profileLabel setNumberOfLines:0];
[self addSubview:self.profileLabel];
}
return self;
}
- (void)layoutSubviews
{
[super layoutSubviews];
UIInterfaceOrientation interfaceOrientation = [[UIApplication sharedApplication] statusBarOrientation];
if (interfaceOrientation == UIInterfaceOrientationPortrait || interfaceOrientation == UIInterfaceOrientationPortraitUpsideDown)
{
[self setVerticalFrame];
}
else if (interfaceOrientation == UIInterfaceOrientationLandscapeLeft || interfaceOrientation == UIInterfaceOrientationLandscapeRight)
{
[self setHorizontalFrame];
}
}
- (void)setVerticalFrame
{
NSLog(@"竖屏");
CGRect rect0 = CGRectMake(50, 10, self.frame.size.width - 100, 160);
[self.iconImageView setFrame:rect0];
CGRect rect1 = CGRectMake(10, self.iconImageView.frame.origin.y + self.iconImageView.frame.size.height + 10, self.frame.size.width - 20, 80);
[self.profileLabel setFrame:rect1];
}
- (void)setHorizontalFrame
{
NSLog(@"横屏");
CGRect rect0 = CGRectMake(10, 10, 100, self.frame.size.height - 20);
[self.iconImageView setFrame:rect0];
CGRect rect1 = CGRectMake(self.iconImageView.frame.origin.x + self.iconImageView.frame.size.width + 10,
10,
self.frame.size.width - self.iconImageView.frame.origin.x - self.iconImageView.frame.size.width - 10 - 10,
self.frame.size.height - 20);
[self.profileLabel setFrame:rect1];
}
@end
参考:
http://bachiscoding.com/blog/2014/12/15/when-will-layoutsubviews-be-invoked/ http://www.cnblogs.com/pengyingh/articles/2417211.html http://stackoverflow.com/questions/728372/when-is-layoutsubviews-called
相关文章推荐
- 峰回路转,Firefox 浏览器即将重返 iOS 平台
- 峰回路转,Firefox 浏览器即将重返 iOS 平台
- 不可修补的 iOS 漏洞可能导致 iPhone 4s 到 iPhone X 永久越狱
- iOS 12.4 系统遭黑客破解,漏洞危及数百万用户
- 每日安全资讯:NSO,一家专业入侵 iPhone 的神秘公司
- [转][源代码]Comex公布JailbreakMe 3.0源代码
- 讲解iOS开发中基本的定位功能实现
- js判断客户端是iOS还是Android等移动终端的方法
- IOS开发环境windows化攻略
- 浅析iOS应用开发中线程间的通信与线程安全问题
- 检测iOS设备是否越狱的方法
- .net平台推送ios消息的实现方法
- 探讨Android与iOS,我们将何去何从?
- Android、iOS和Windows Phone中的推送技术详解
- IOS 改变键盘颜色代码
- 举例详解iOS开发过程中的沙盒机制与文件
- Android和IOS的浏览器中检测是否安装某个客户端的方法
- 分享一个iOS下实现基本绘画板功能的简单方法
- javascript实现阻止iOS APP中的链接打开Safari浏览器