Android开发Tips(2)
2016-01-18 08:20
726 查看
欢迎Follow我的GitHub, 关注我的CSDN.
我会介绍关于Android的一些有趣的小知识点. 上一篇.
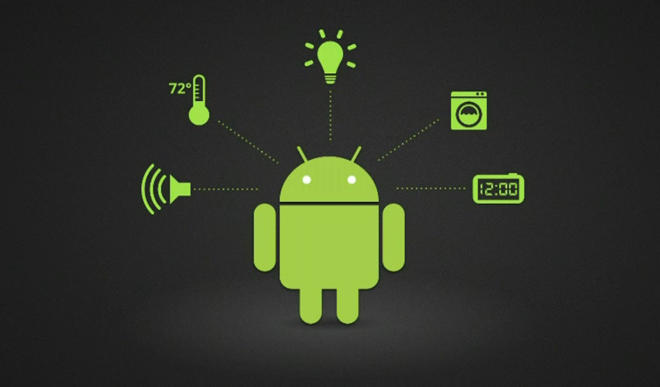
首先模块(Module)创建需要提供的类实例, 其次把模块添加到组件(Component)中并提供需要注入的类, 最后把组件添加到应用(Application)中并提供接口.
布局设置
逻辑设置
(1) 在MultiDex中dex的详细信息.
(2) 使用NativeLibrary的详细信息.
(3) 类的详细信息.
(4) 数量统计.

(1) standard, 标准模式, 启动重新创建示例, 默认.
(2) singleTop, 栈顶复用模式, 位于栈顶, 启动不会被创建, 调用onNewIntent.
(3) singleTask, 栈内复用模式, 存在不会被创建, 调用onNewIntent.
(4) singleInstance, 单实例模式, 单独位于一个任务栈内, 复用.
显示

安装Jinja2.
设置数据
下载代码库. 生成代码.
OK, That’s all! Enjoy It!
我会介绍关于Android的一些有趣的小知识点. 上一篇.
1. Dagger2的开发顺序
Module -> Component -> Application首先模块(Module)创建需要提供的类实例, 其次把模块添加到组件(Component)中并提供需要注入的类, 最后把组件添加到应用(Application)中并提供接口.
// 模块 @Module public class TestAppModule { private final Context mContext; public TestAppModule(Context context) { mContext = context.getApplicationContext(); } // 提供类实例 @AppScope @Provides public Context provideAppContext() { return mContext; } @Provides public WeatherApiClient provideWeatherApiClient() { return new MockWeatherApiClient(); } } // 组件 @AppScope @Component(modules = TestAppModule.class) // 注册模块 public interface TestAppComponent extends AppComponent { void inject(MainActivityTest test); } // 应用 public class TestWeatherApplication extends WeatherApplication { private TestAppComponent mTestAppComponent; @Override public void onCreate() { super.onCreate(); mTestAppComponent = DaggerTestAppComponent.builder() .testAppModule(new TestAppModule(this)) .build(); } // 提供组件 @Override public TestAppComponent getAppComponent() { return mTestAppComponent; } }
2. JRebel
Android调试工具, 不用编译, 就可以刷新一些项目修改. 不过功能已经被Android Studio 2.0 代替, 等待2.0正式发版.3. 数据绑定(DataBinding)
DataBinding实现数据与页面的分离, 更符合面向对象的编程模式.布局设置
<data> <variable name="weatherData" type="clwang.chunyu.me.wcl_espresso_dagger_demo.data.WeatherData"/> </data> <TextView android:id="@+id/temperature" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerInParent="true" android:layout_marginBottom="@dimen/margin_large" android:layout_marginTop="@dimen/margin_xlarge" android:text="@{weatherData.temperatureCelsius}" android:textAppearance="@style/TextAppearance.AppCompat.Display3" tools:text="10°"/>
逻辑设置
private ActivityMainBinding mBinding; // 页面绑定类 mBinding = DataBindingUtil.setContentView(this, R.layout.activity_main); // 绑定页面 mBinding.weatherLayout.setVisibility(View.VISIBLE); // 使用Id mBinding.setWeatherData(weatherData); // 绑定数据
4. ClassyShark
查看Apk信息的软件, 功能非常强大, 省去反编译的步骤, 主要功能:(1) 在MultiDex中dex的详细信息.
(2) 使用NativeLibrary的详细信息.
(3) 类的详细信息.
(4) 数量统计.

5. CocoaPod安装
升级Mac系统, 可能会导致Pod命令消失, 需要重新安装Pod.sudo gem install -n /usr/local/bin cocoapods
6. LaunchMode
LaunchMode包含四种模式,(1) standard, 标准模式, 启动重新创建示例, 默认.
(2) singleTop, 栈顶复用模式, 位于栈顶, 启动不会被创建, 调用onNewIntent.
(3) singleTask, 栈内复用模式, 存在不会被创建, 调用onNewIntent.
(4) singleInstance, 单实例模式, 单独位于一个任务栈内, 复用.
7. TextView的标准字体
样式style="@style/TextAppearance.AppCompat.Display4" style="@style/TextAppearance.AppCompat.Display3" style="@style/TextAppearance.AppCompat.Display2" style="@style/TextAppearance.AppCompat.Display1" style="@style/TextAppearance.AppCompat.Headline" style="@style/TextAppearance.AppCompat.Title" style="@style/TextAppearance.AppCompat.Subhead" style="@style/TextAppearance.AppCompat.Body2" style="@style/TextAppearance.AppCompat.Body1" style="@style/TextAppearance.AppCompat.Caption" style="@style/TextAppearance.AppCompat.Button"
显示

8. 自动生成DbHelper的脚本
下载地址安装Jinja2.
pip install Jinja2
设置数据
CLASS Repo String Id String Name String Description String Owner ENDCLASS
下载代码库. 生成代码.
python sql_lite_helper.py -f ~/Desktop/Repo -n SampleGenerate -p me.chunyu -a clwang
9. Gson的序列化参数
有些情况下, Json名称与变量不同, 需要指定.@SerializedName("avatar_url") private String avatarUrl;
10. Proguard保留库
最简洁的方式是全部保留. 去除警告dontwarn, 保留类keep class.# 在线更新 -dontwarn clwang.chunyu.me.** -keep class clwang.chunyu.me.**{*;}
OK, That’s all! Enjoy It!
相关文章推荐
- 使用C++实现JNI接口需要注意的事项
- Android IPC进程间通讯机制
- Android Manifest 用法
- [转载]Activity中ConfigChanges属性的用法
- Android之获取手机上的图片和视频缩略图thumbnails
- Android之使用Http协议实现文件上传功能
- Android学习笔记(二九):嵌入浏览器
- android string.xml文件中的整型和string型代替
- i-jetty环境搭配与编译
- android之定时器AlarmManager
- android wifi 无线调试
- Android Native 绘图方法
- Android java 与 javascript互访(相互调用)的方法例子
- android 代码实现控件之间的间距
- android FragmentPagerAdapter的“标准”配置
- Android"解决"onTouch和onClick的冲突问题
- android:installLocation简析
- android searchView的关闭事件
- SourceProvider.getJniDirectories