仿QQ空间用一个tableview显示多种自定义cell
2015-12-07 18:30
471 查看
http://my.oschina.net/iOSliuhui/blog/518317
第一部分 要实现效果
先来看看真实QQ空间的效果吧:

从这张截图中,可以看到两种自定义的cell,其实在QQ空间的我的空间中有三种自定义的cell,那么这就是我们要实现的效果。
第二部分 实现的思路
第一步(由于没有数据源,我们只好操作plist文件):
创建我们所需要的plist文件,如下图:
这是表格样式三所需的plist文件
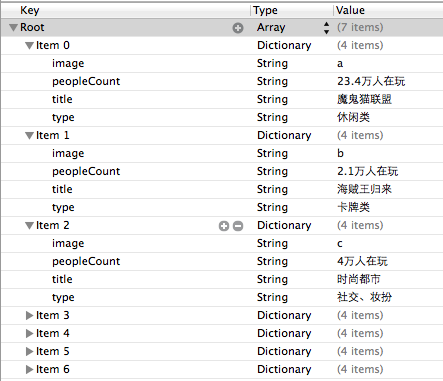
这是我们表格样式一所需的plist文件
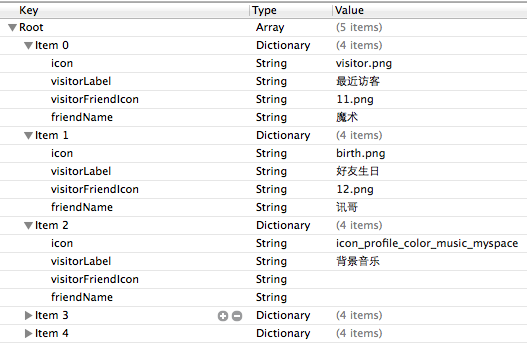
这是表格样式二所需要的文件
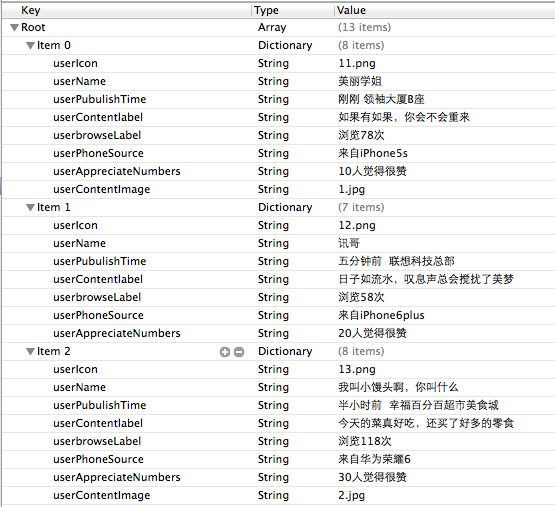
第二步 有了素材文件之后,就好办了,我们需要把它解析出来,由于这些plist文件都是以数组形式包装的,所以在解析出来的时候,我们要用数组给他们保存起来,所以我们在头文件中声明了这三个数组
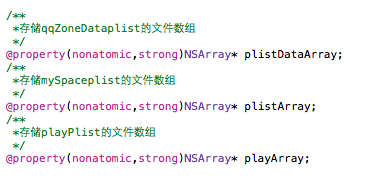
第三步 加载并保存plist文件
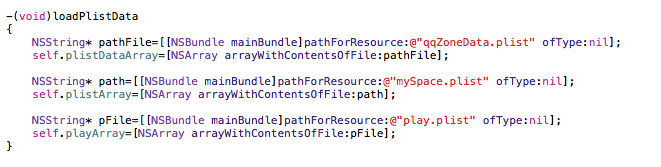
第四步 控制器成为表格的代理和数据源,并实现数据源相应的方法

第五步 实现表格的返回section的分区数的这个方法,这个很重要,也是实现我们今天的这个效果的关键部分之一
由于我们需要实现三个自定义的cell,所以在这里返回3就行了,如果你想返回很多个分区,你在这里写上你想要的分区数就行了
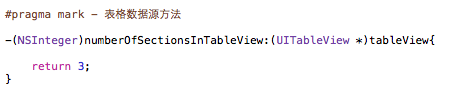
第六步 返回每个分区表格的行数,由于有三个分区,需要作相应的判断,方法如下
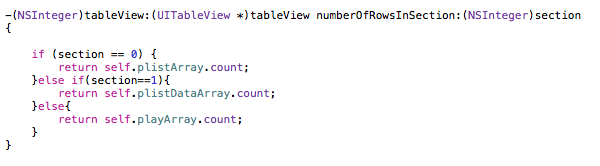
第七步 返回每个分区中表格中每行返回的要显示的cell
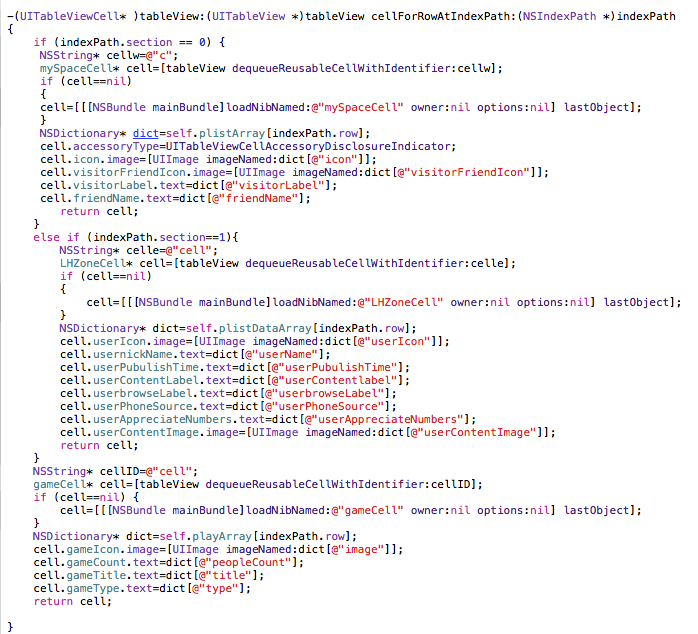
第八步 返回分区中每行表格需要显示的行高,不要忘了这个方法哦,不然表格不会正常的显示出来,而是显示默认的44的高度
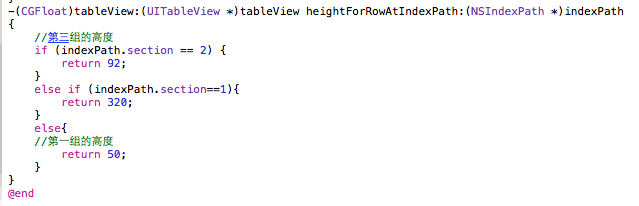
第三部分 程序实现的效果
好了,思路大概就是那样的了,现在来看看我们的结果吧

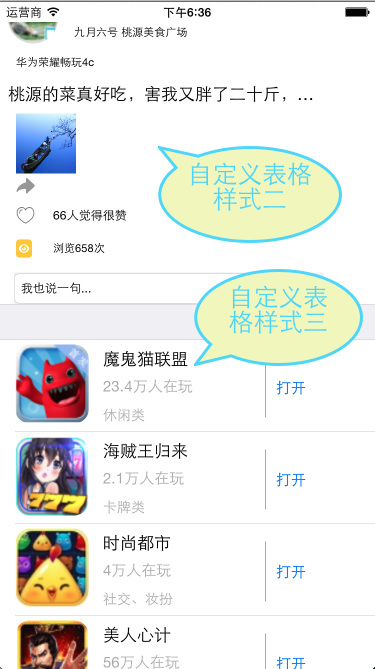
第四部分 源码
为了方便大家学习,我把源码复制上来
// 多样式的表格
//
// Created by 妖精的尾巴 on 15-10-16.
// Copyright (c) 2015年 妖精的尾巴. All rights reserved.
//
#import "ViewController.h"
#import "LHZoneCell.h"
#import "mySpaceCell.h"
#import "gameCell.h"
@interface ViewController ()<UITableViewDataSource,UITableViewDelegate>
/**
*存储qqZoneDataplist的文件数组
*/
@property(nonatomic,strong)NSArray*
plistDataArray;
/**
*存储mySpaceplist的文件数组
*/
@property(nonatomic,strong)NSArray*
plistArray;
/**
*存储playPlist的文件数组
*/
@property(nonatomic,strong)NSArray*
playArray;
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
[self createTable];
[self loadPlistData];
}
-(void)loadPlistData
{
NSString* pathFile=[[NSBundle mainBundle]pathForResource:@"qqZoneData.plist" ofType:nil];
self.plistDataArray=[NSArray arrayWithContentsOfFile:pathFile];
NSString* path=[[NSBundle mainBundle]pathForResource:@"mySpace.plist" ofType:nil];
self.plistArray=[NSArray arrayWithContentsOfFile:path];
NSString* pFile=[[NSBundle mainBundle]pathForResource:@"play.plist" ofType:nil];
self.playArray=[NSArray arrayWithContentsOfFile:pFile];
}
-(void)createTable
{
UITableView* tableView=[[UITableView alloc]initWithFrame:CGRectMake(0, 20, self.view.frame.size.width, self.view.frame.size.height) style:UITableViewStyleGrouped];
tableView.delegate=self;
tableView.dataSource=self;
[self.view addSubview:tableView];
}
#pragma mark - 表格数据源方法
-(NSInteger)numberOfSectionsInTableView:(UITableView *)tableView{
return 3;
}
-(NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
if (section == 0) {
return self.plistArray.count;
}else if(section==1){
return self.plistDataArray.count;
}else{
return self.playArray.count;
}
}
-(UITableViewCell* )tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
if (indexPath.section == 0) {
NSString* cellw=@"c";
mySpaceCell* cell=[tableView dequeueReusableCellWithIdentifier:cellw];
if (cell==nil)
{
cell=[[[NSBundle mainBundle]loadNibNamed:@"mySpaceCell" owner:nil options:nil] lastObject];
}
NSDictionary* dict=self.plistArray[indexPath.row];
cell.accessoryType=UITableViewCellAccessoryDisclosureIndicator;
cell.icon.image=[UIImage imageNamed:dict[@"icon"]];
cell.visitorFriendIcon.image=[UIImage imageNamed:dict[@"visitorFriendIcon"]];
cell.visitorLabel.text=dict[@"visitorLabel"];
cell.friendName.text=dict[@"friendName"];
return cell;
}
else if (indexPath.section==1){
NSString* celle=@"cell";
LHZoneCell* cell=[tableView dequeueReusableCellWithIdentifier:celle];
if (cell==nil)
{
cell=[[[NSBundle mainBundle]loadNibNamed:@"LHZoneCell" owner:nil options:nil] lastObject];
}
NSDictionary* dict=self.plistDataArray[indexPath.row];
cell.userIcon.image=[UIImage imageNamed:dict[@"userIcon"]];
cell.usernickName.text=dict[@"userName"];
cell.userPubulishTime.text=dict[@"userPubulishTime"];
cell.userContentLabel.text=dict[@"userContentlabel"];
cell.userbrowseLabel.text=dict[@"userbrowseLabel"];
cell.userPhoneSource.text=dict[@"userPhoneSource"];
cell.userAppreciateNumbers.text=dict[@"userAppreciateNumbers"];
cell.userContentImage.image=[UIImage imageNamed:dict[@"userContentImage"]];
return cell;
}
NSString* cellID=@"cell";
gameCell* cell=[tableView dequeueReusableCellWithIdentifier:cellID];
if (cell==nil) {
cell=[[[NSBundle mainBundle]loadNibNamed:@"gameCell" owner:nil options:nil] lastObject];
}
NSDictionary* dict=self.playArray[indexPath.row];
cell.gameIcon.image=[UIImage imageNamed:dict[@"image"]];
cell.gameCount.text=dict[@"peopleCount"];
cell.gameTitle.text=dict[@"title"];
cell.gameType.text=dict[@"type"];
return cell;
}
-(CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath
{
//第三组的高度
if (indexPath.section == 2) {
return 92;
}
else if (indexPath.section==1){
return 320;
}
else{
//第一组的高度
return 50;
}
}
@end
第一部分 要实现效果
先来看看真实QQ空间的效果吧:

从这张截图中,可以看到两种自定义的cell,其实在QQ空间的我的空间中有三种自定义的cell,那么这就是我们要实现的效果。
第二部分 实现的思路
第一步(由于没有数据源,我们只好操作plist文件):
创建我们所需要的plist文件,如下图:
这是表格样式三所需的plist文件
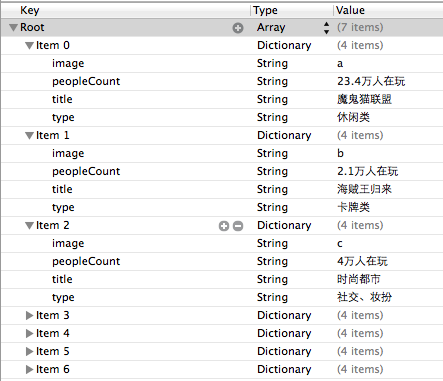
这是我们表格样式一所需的plist文件
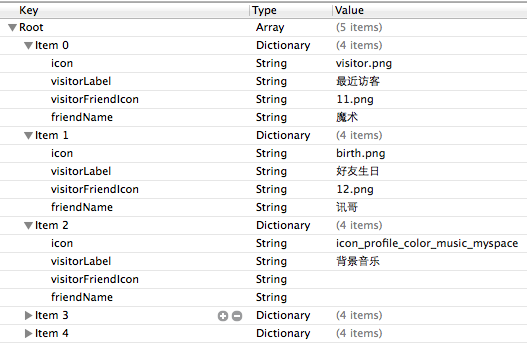
这是表格样式二所需要的文件
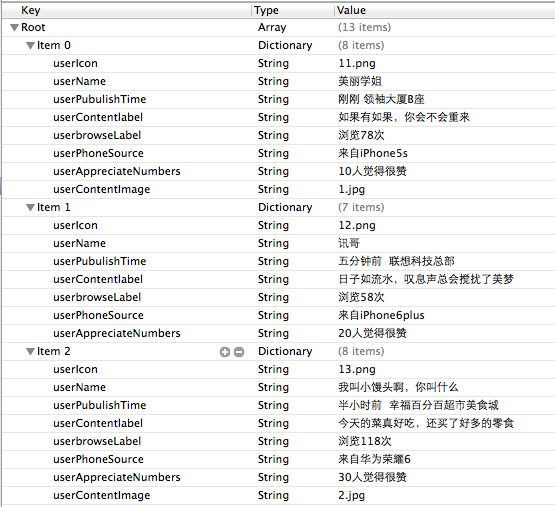
第二步 有了素材文件之后,就好办了,我们需要把它解析出来,由于这些plist文件都是以数组形式包装的,所以在解析出来的时候,我们要用数组给他们保存起来,所以我们在头文件中声明了这三个数组
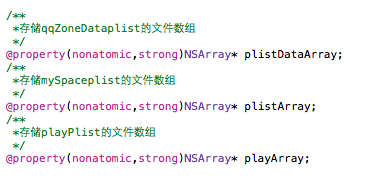
第三步 加载并保存plist文件
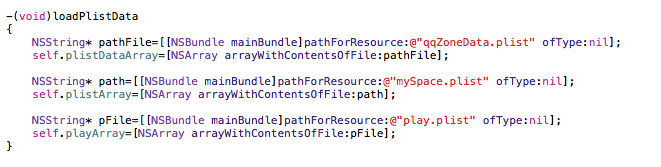
第四步 控制器成为表格的代理和数据源,并实现数据源相应的方法

第五步 实现表格的返回section的分区数的这个方法,这个很重要,也是实现我们今天的这个效果的关键部分之一
由于我们需要实现三个自定义的cell,所以在这里返回3就行了,如果你想返回很多个分区,你在这里写上你想要的分区数就行了
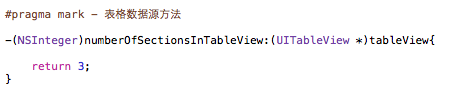
第六步 返回每个分区表格的行数,由于有三个分区,需要作相应的判断,方法如下
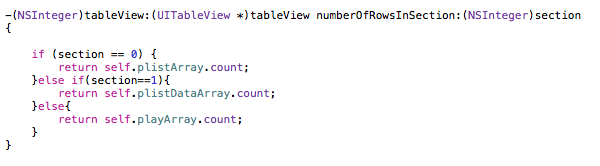
第七步 返回每个分区中表格中每行返回的要显示的cell
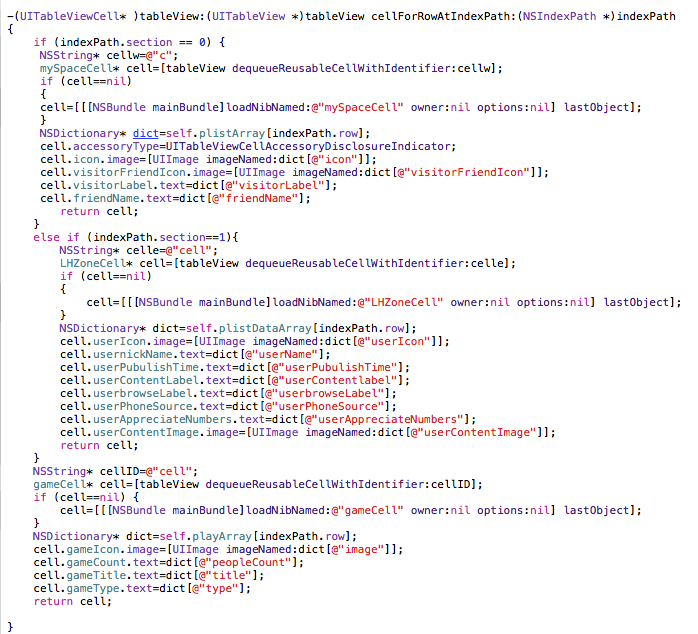
第八步 返回分区中每行表格需要显示的行高,不要忘了这个方法哦,不然表格不会正常的显示出来,而是显示默认的44的高度
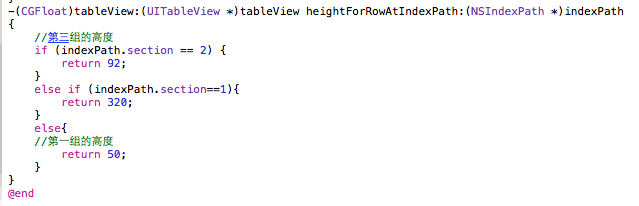
第三部分 程序实现的效果
好了,思路大概就是那样的了,现在来看看我们的结果吧

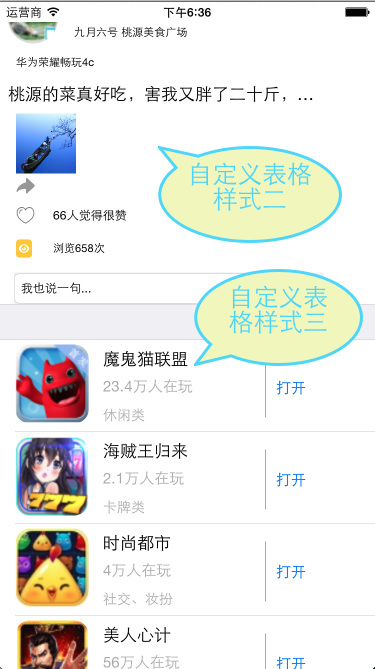
第四部分 源码
为了方便大家学习,我把源码复制上来
// 多样式的表格
//
// Created by 妖精的尾巴 on 15-10-16.
// Copyright (c) 2015年 妖精的尾巴. All rights reserved.
//
#import "ViewController.h"
#import "LHZoneCell.h"
#import "mySpaceCell.h"
#import "gameCell.h"
@interface ViewController ()<UITableViewDataSource,UITableViewDelegate>
/**
*存储qqZoneDataplist的文件数组
*/
@property(nonatomic,strong)NSArray*
plistDataArray;
/**
*存储mySpaceplist的文件数组
*/
@property(nonatomic,strong)NSArray*
plistArray;
/**
*存储playPlist的文件数组
*/
@property(nonatomic,strong)NSArray*
playArray;
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
[self createTable];
[self loadPlistData];
}
-(void)loadPlistData
{
NSString* pathFile=[[NSBundle mainBundle]pathForResource:@"qqZoneData.plist" ofType:nil];
self.plistDataArray=[NSArray arrayWithContentsOfFile:pathFile];
NSString* path=[[NSBundle mainBundle]pathForResource:@"mySpace.plist" ofType:nil];
self.plistArray=[NSArray arrayWithContentsOfFile:path];
NSString* pFile=[[NSBundle mainBundle]pathForResource:@"play.plist" ofType:nil];
self.playArray=[NSArray arrayWithContentsOfFile:pFile];
}
-(void)createTable
{
UITableView* tableView=[[UITableView alloc]initWithFrame:CGRectMake(0, 20, self.view.frame.size.width, self.view.frame.size.height) style:UITableViewStyleGrouped];
tableView.delegate=self;
tableView.dataSource=self;
[self.view addSubview:tableView];
}
#pragma mark - 表格数据源方法
-(NSInteger)numberOfSectionsInTableView:(UITableView *)tableView{
return 3;
}
-(NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
if (section == 0) {
return self.plistArray.count;
}else if(section==1){
return self.plistDataArray.count;
}else{
return self.playArray.count;
}
}
-(UITableViewCell* )tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
if (indexPath.section == 0) {
NSString* cellw=@"c";
mySpaceCell* cell=[tableView dequeueReusableCellWithIdentifier:cellw];
if (cell==nil)
{
cell=[[[NSBundle mainBundle]loadNibNamed:@"mySpaceCell" owner:nil options:nil] lastObject];
}
NSDictionary* dict=self.plistArray[indexPath.row];
cell.accessoryType=UITableViewCellAccessoryDisclosureIndicator;
cell.icon.image=[UIImage imageNamed:dict[@"icon"]];
cell.visitorFriendIcon.image=[UIImage imageNamed:dict[@"visitorFriendIcon"]];
cell.visitorLabel.text=dict[@"visitorLabel"];
cell.friendName.text=dict[@"friendName"];
return cell;
}
else if (indexPath.section==1){
NSString* celle=@"cell";
LHZoneCell* cell=[tableView dequeueReusableCellWithIdentifier:celle];
if (cell==nil)
{
cell=[[[NSBundle mainBundle]loadNibNamed:@"LHZoneCell" owner:nil options:nil] lastObject];
}
NSDictionary* dict=self.plistDataArray[indexPath.row];
cell.userIcon.image=[UIImage imageNamed:dict[@"userIcon"]];
cell.usernickName.text=dict[@"userName"];
cell.userPubulishTime.text=dict[@"userPubulishTime"];
cell.userContentLabel.text=dict[@"userContentlabel"];
cell.userbrowseLabel.text=dict[@"userbrowseLabel"];
cell.userPhoneSource.text=dict[@"userPhoneSource"];
cell.userAppreciateNumbers.text=dict[@"userAppreciateNumbers"];
cell.userContentImage.image=[UIImage imageNamed:dict[@"userContentImage"]];
return cell;
}
NSString* cellID=@"cell";
gameCell* cell=[tableView dequeueReusableCellWithIdentifier:cellID];
if (cell==nil) {
cell=[[[NSBundle mainBundle]loadNibNamed:@"gameCell" owner:nil options:nil] lastObject];
}
NSDictionary* dict=self.playArray[indexPath.row];
cell.gameIcon.image=[UIImage imageNamed:dict[@"image"]];
cell.gameCount.text=dict[@"peopleCount"];
cell.gameTitle.text=dict[@"title"];
cell.gameType.text=dict[@"type"];
return cell;
}
-(CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath
{
//第三组的高度
if (indexPath.section == 2) {
return 92;
}
else if (indexPath.section==1){
return 320;
}
else{
//第一组的高度
return 50;
}
}
@end
相关文章推荐
- Animation动画的解析
- HTML 颜色名
- iOS耗电测试工具--batterydetective
- 使用git 新建分支以及管理分支
- activiti笔记三 Activiti问题重现
- PInvoke复习之深入理解char*与wchar_t*与string以及wstring之间的相互转换
- UITextField总结
- BZOJ2208 [JSOI2010] 连通数
- 关于项目的构建与打包
- IOS TableView的Cell高度自适应,UILabel自动换行适应
- javascript正则表达式
- HTML 颜色
- 第三个Sprint ------第一天
- 【第14周-查找项目1-4——验证平衡二叉树相关算法】
- shell之RDS备份+判断是否传输完成
- 第14周 项目1 - 验证算法 - 二叉排序树相关算法
- JSTL标签库核心标签C标签的使用
- javascript实现URL不缓存的方法
- 合成模式(Composite)-山下的养牛场
- 关闭Android/iPhone浏览器自动识别数字为电话号码