JavaScript集合
2015-12-05 00:00
253 查看
摘要: JavaScript集合
集合的概念
大家在高中数学都学过集合,在计算机科学中的集合数据结构也是一样的含义。集合是由一组无序且唯一的项组成的。与数学中的有限集合的概念一致。因此,联系数组这种数据结构,可以说,集合是一个既没有重复元素,也没有顺序概念的数组。
In computer science, a set is an abstract data type that can store certain values, without any particular order, and no repeated values. It is a computer implementation of the mathematical concept of a finite set. Unlike most other collection types, rather than retrieving a specific element from a set, one typically tests a value for membership in a set.
集合的实现
我们是使用对象来表示集合的。JavaScript的对象不允许一个键指向不同的属性,保证了集合里的元素是唯一(unique)的。接下来对集合进行测试。
测试的结果如下:
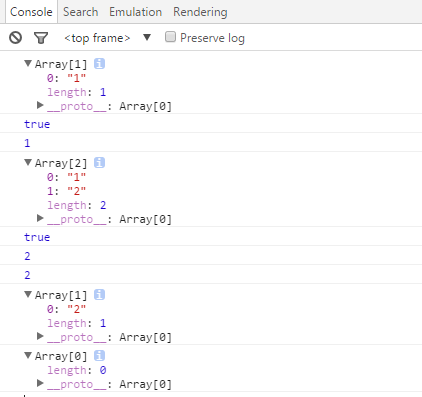
集合操作
并集(union):In set theory, the union (denoted by ∪) of a collection of sets is the set of all distinct elements in the collection. It is one of the fundamental operations through which sets can be combined and related to each other.
交集(intersection):In mathematics, the intersection A ∩ B of two sets A and B is the set that contains all elements of A that also belong to B (or equivalently, all elements of B that also belong to A), but no other elements.
差集(difference):The set difference (or simply difference) between and (in that order) is the set of all elements of that are not in . This set is denoted by or (or occasionally ).
子集(subset):In mathematics, especially in set theory, a set A is a subset of a set B, or equivalently B is a superset of A, if A is "contained" inside B, that is, all elements of A are also elements of B. A and B may coincide.
运行结果如下:
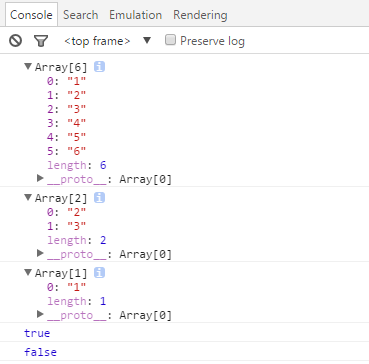
程序主要是实现一个与ECMAScript6定义类似的Set类。
ECMAScript 6, also known as ECMAScript 2015, is the latest version of the ECMAScript standard. ES6 is a significant update to the language, and the first update to the language since ES5 was standardized in 2009. Implementation of these features in major JavaScript engines is underway now.
集合的概念
大家在高中数学都学过集合,在计算机科学中的集合数据结构也是一样的含义。集合是由一组无序且唯一的项组成的。与数学中的有限集合的概念一致。因此,联系数组这种数据结构,可以说,集合是一个既没有重复元素,也没有顺序概念的数组。In computer science, a set is an abstract data type that can store certain values, without any particular order, and no repeated values. It is a computer implementation of the mathematical concept of a finite set. Unlike most other collection types, rather than retrieving a specific element from a set, one typically tests a value for membership in a set.
集合的实现
/** * ECMSCRIPT 6 already have a Set class implementation: * https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Set * We will try to copy the same functionalities * @constructor */ function Set() { var items = {}; this.add = function(value){ if (!this.has(value)){ items[value] = value; return true; } return false; }; this.remove = function(value){ if (this.has(value)){ delete items[value]; return true; } return false; }; this.has = function(value){ return items.hasOwnProperty(value); //return value in items; }; this.clear = function(){ items = {}; }; /** * Modern browsers function * IE9+, FF4+, Chrome5+, Opera12+, Safari5+ * @returns {Number} */ this.size = function(){ return Object.keys(items).length; }; /** * cross browser compatibility - legacy browsers * for modern browsers use size function * @returns {number} */ this.sizeLegacy = function(){ var count = 0; for(var prop in items) { if(items.hasOwnProperty(prop)) ++count; } return count; }; /** * Modern browsers function * IE9+, FF4+, Chrome5+, Opera12+, Safari5+ * @returns {Array} */ this.values = function(){ return Object.keys(items); }; this.valuesLegacy = function(){ var keys = []; for(var key in items){ keys.push(key); } return keys; }; this.getItems = function(){ return items; }; this.union = function(otherSet){ var unionSet = new Set(); //初始化一个新的集合,存放并集元素 var values = this.values(); for (var i=0; i<values.length; i++){ unionSet.add(values[i]); } values = otherSet.values(); for (var i=0; i<values.length; i++){ unionSet.add(values[i]);//并集只需要分别遍历两个集合即可 } return unionSet; }; this.intersection = function(otherSet){ var intersectionSet = new Set(); //{1} var values = this.values(); for (var i=0; i<values.length; i++){ //{2} if (otherSet.has(values[i])){ //{3} intersectionSet.add(values[i]); //{4} } } return intersectionSet; }; this.difference = function(otherSet){ var differenceSet = new Set(); var values = this.values(); for (var i=0; i<values.length; i++){ if (!otherSet.has(values[i])){ differenceSet.add(values[i]); } } return differenceSet; }; this.subset = function(otherSet){ if (this.size() > otherSet.size()){ //子集的元素个数一定小于超集 return false; } else { var values = this.values(); for (var i=0; i<values.length; i++){ //遍历 if (!otherSet.has(values[i])){ //判断otherSet中是否包含subset中的所有元素 return false; } } return true; } }; }
我们是使用对象来表示集合的。JavaScript的对象不允许一个键指向不同的属性,保证了集合里的元素是唯一(unique)的。接下来对集合进行测试。
测试集合
var set = new Set(); set.add(1); console.log(set.values()); console.log(set.has(1)); console.log(set.size()); set.add(2); console.log(set.values()); console.log(set.has(2)); console.log(set.size()); console.log(set.sizeLegacy()); set.remove(1); console.log(set.values()); set.remove(2); console.log(set.values());
测试的结果如下:
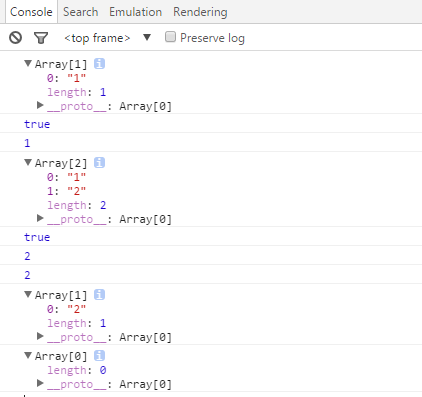
集合操作
并集(union):In set theory, the union (denoted by ∪) of a collection of sets is the set of all distinct elements in the collection. It is one of the fundamental operations through which sets can be combined and related to each other.交集(intersection):In mathematics, the intersection A ∩ B of two sets A and B is the set that contains all elements of A that also belong to B (or equivalently, all elements of B that also belong to A), but no other elements.
差集(difference):The set difference (or simply difference) between and (in that order) is the set of all elements of that are not in . This set is denoted by or (or occasionally ).
子集(subset):In mathematics, especially in set theory, a set A is a subset of a set B, or equivalently B is a superset of A, if A is "contained" inside B, that is, all elements of A are also elements of B. A and B may coincide.
测试集合操作
//--------- Union ---------- var setA = new Set(); setA.add(1); setA.add(2); setA.add(3); var setB = new Set(); setB.add(3); setB.add(4); setB.add(5); setB.add(6); var unionAB = setA.union(setB); console.log(unionAB.values()); //--------- Intersection ---------- var setA = new Set(); setA.add(1); setA.add(2); setA.add(3); var setB = new Set(); setB.add(2); setB.add(3); setB.add(4); var intersectionAB = setA.intersection(setB); console.log(intersectionAB.values()); //--------- Difference ---------- var setA = new Set(); setA.add(1); setA.add(2); setA.add(3); var setB = new Set(); setB.add(2); setB.add(3); setB.add(4); var differenceAB = setA.difference(setB); console.log(differenceAB.values()); //--------- Subset ---------- var setA = new Set(); setA.add(1); setA.add(2); var setB = new Set(); setB.add(1); setB.add(2); setB.add(3); var setC = new Set(); setC.add(2); setC.add(3); setC.add(4); console.log(setA.subset(setB)); console.log(setA.subset(setC));
运行结果如下:
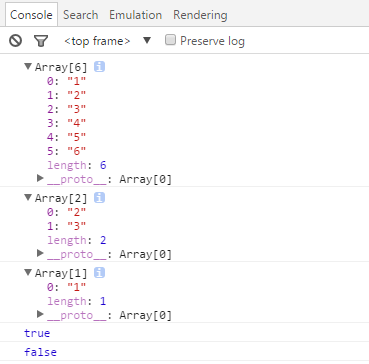
程序主要是实现一个与ECMAScript6定义类似的Set类。
ECMAScript 6, also known as ECMAScript 2015, is the latest version of the ECMAScript standard. ES6 is a significant update to the language, and the first update to the language since ES5 was standardized in 2009. Implementation of these features in major JavaScript engines is underway now.
相关文章推荐
- JavaScript数据结构与算法之集合(Set)
- JavaScript escape() 函数
- 分享10个原生JavaScript技巧
- JavaScript 调试建议和技巧
- 通过js获取cookie的实例及简单分析
- 几个学js经常看的网址
- JavaScript的应用
- JavaScript-undefined与null区别
- 在sublime text中添加JavaScript的build-system
- 40.JSON解析
- JSON
- 使用Gson解析json格式的字符串的正确方式
- html5中js添加下拉菜单
- javascript 如何定义一个多维数组
- Round() 四舍五入 js银行家算法
- Js获取当前时间、日期
- servlet&jsp 各种乱码问题
- js回到顶部,关闭一个模块的方法
- js超链接
- json的使用(js对象表示法)