轮转调度算法(RR)
2015-12-03 01:00
330 查看
RR算法是使用非常广泛的一种调度算法。
首先将所有就绪的队列按FCFS策略排成一个就绪队列,然后系统设置一定的时间片,每次给队首作业分配时间片。如果此作业运行结束,即使时间片没用完,立刻从队列中去除此作业,并给下一个作业分配新的时间片;如果作业时间片用完没有运行结束,则将此作业重新加入就绪队列尾部等待调度。
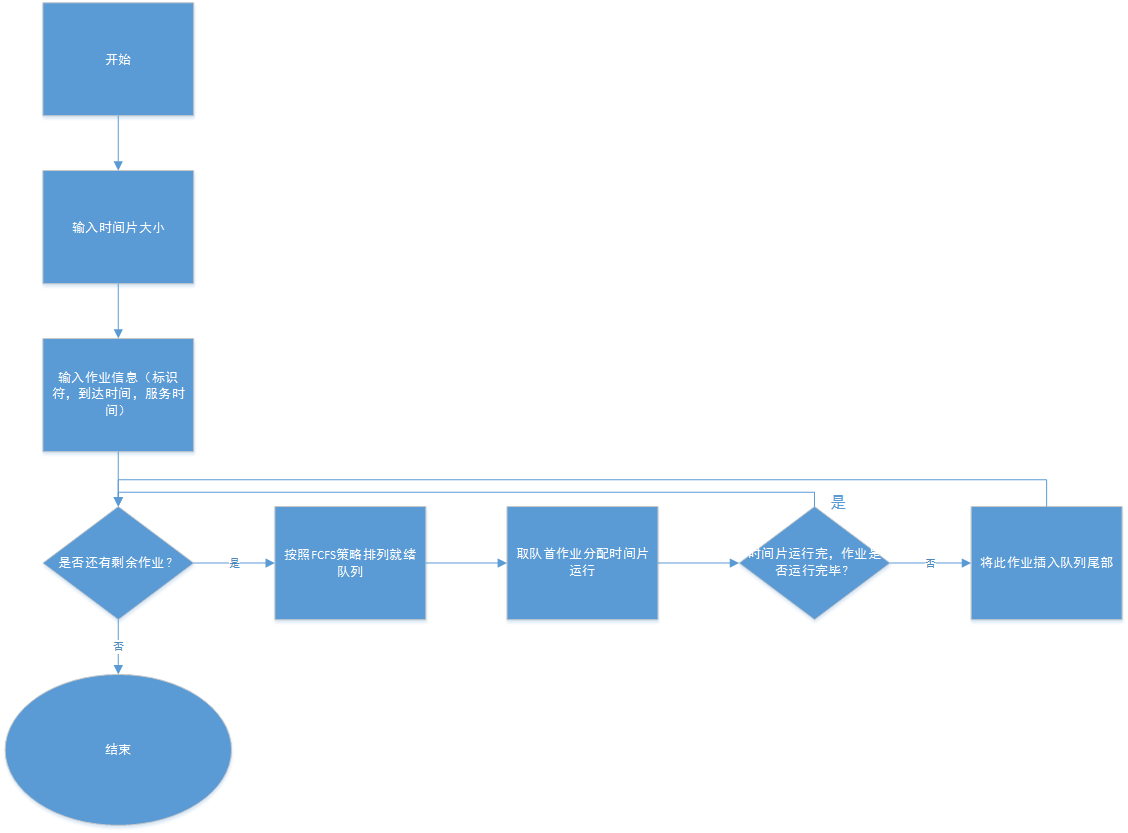
首先将所有就绪的队列按FCFS策略排成一个就绪队列,然后系统设置一定的时间片,每次给队首作业分配时间片。如果此作业运行结束,即使时间片没用完,立刻从队列中去除此作业,并给下一个作业分配新的时间片;如果作业时间片用完没有运行结束,则将此作业重新加入就绪队列尾部等待调度。
//main.cpp #include "RR.h" int main() { std::vector<PCB> PCBList; int timeslice; //输入时间片大小,作业信息 InputPCB(PCBList, timeslice); //RR算法 RR(PCBList, timeslice); //显示结果 show(PCBList); return 0; } //RR.h #ifndef RR_H_ #define RR_H_ #include <iostream> #include <algorithm> #include <iomanip> #include <vector> #include <queue> //作业结构体 typedef struct PCB { int ID; //标识符 int ComeTime; //到达时间 int ServerTime; //服务时间 int FinishTime; //完成时间 int TurnoverTime; //周转时间 double WeightedTurnoverTime; //带权周转时间 }PCB; /* 函数功能:输入作业信息 参数说明: PCBList std::vector<PCB>& PCB链 timeslice int 时间片 */ void InputPCB(std::vector<PCB> &PCBList, int ×lice); /* 函数功能:RR算法 参数说明: PCBList std::vector<PCB>& PCB链 */ void RR(std::vector<PCB> &PCBList, int timeslice); /* 函数功能:显示结果 参数说明: PCBList std::vector<PCB>& PCB链 */ void show(std::vector<PCB> &PCBList); /* 函数功能:比较函数,用于sort(),按ComeTime升序排列 参数说明: p1 const PCB& PCB p2 const PCB& PCB */ bool CmpByComeTime(const PCB &p1, const PCB &p2); #endif //RR.cpp #include "RR.h" //输入作业信息 void InputPCB(std::vector<PCB> &PCBList,int ×lice) { std::cout << "输入时间片大小: "; std::cin >> timeslice; do { PCB temp; std::cout << "输入标识符: "; std::cin >> temp.ID; std::cout << "输入到达时间: "; std::cin >> temp.ComeTime; std::cout << "输入服务时间: "; std::cin >> temp.ServerTime; temp.FinishTime = 0; //暂时存放运行了多少时间,来判断此作业是否运行结束 PCBList.push_back(temp); std::cout << "继续输入?Y/N: "; char ans; std::cin >> ans; if ('Y' == ans || 'y' == ans) continue; else break; } while (true); } //RR算法 void RR(std::vector<PCB> &PCBList, int timeslice) { std::sort(PCBList.begin(), PCBList.end(), CmpByComeTime); //按到达时间排序 std::vector<PCB> result; //保存结果 std::queue<PCB> Ready; //就绪队列 int BeginTime = (*PCBList.begin()).ComeTime; //第一个作业开始时间 Ready.push(*PCBList.begin()); PCBList.erase(PCBList.begin()); while (!PCBList.empty() || !Ready.empty()) { if (!PCBList.empty() && BeginTime >= (*PCBList.begin()).ComeTime) //有新作业到达,加入就绪队列 { Ready.push(*PCBList.begin()); PCBList.erase(PCBList.begin()); } if (Ready.front().FinishTime + timeslice < Ready.front().ServerTime) //时间片用完没运行完,加入队尾 { Ready.front().FinishTime += timeslice; Ready.push(Ready.front()); Ready.pop(); BeginTime += timeslice; } else //此作业运行完 { BeginTime += Ready.front().ServerTime - Ready.front().FinishTime; Ready.front().FinishTime = BeginTime; Ready.front().TurnoverTime = Ready.front().FinishTime - Ready.front().ComeTime; Ready.front().WeightedTurnoverTime = (double)Ready.front().TurnoverTime / Ready.front().ServerTime; //从就绪队列中移除作业 result.push_back(Ready.front()); Ready.pop(); } } //按ComeTime升序排序,便于显示结果 PCBList = result; std::sort(PCBList.begin(), PCBList.end(), CmpByComeTime); } //显示结果 void show(std::vector<PCB> &PCBList) { int SumTurnoverTime = 0; double SumWeightedTurnoverTime = 0; std::cout.setf(std::ios::left); std::cout << std::setw(20) << "标识符"; for (std::vector<PCB>::iterator it = PCBList.begin(); it < PCBList.end(); ++it) std::cout << std::setw(5) << (*it).ID; std::cout << std::endl; std::cout << std::setw(20) << "到达时间"; for (std::vector<PCB>::iterator it = PCBList.begin(); it < PCBList.end(); ++it) std::cout << std::setw(5) << (*it).ComeTime; std::cout << std::endl; std::cout << std::setw(20) << "服务时间"; for (std::vector<PCB>::iterator it = PCBList.begin(); it < PCBList.end(); ++it) std::cout << std::setw(5) << (*it).ServerTime; std::cout << std::endl; std::cout << std::setw(20) << "完成时间"; for (std::vector<PCB>::iterator it = PCBList.begin(); it < PCBList.end(); ++it) std::cout << std::setw(5) << (*it).FinishTime; std::cout << std::endl; std::cout << std::setw(20) << "周转时间"; for (std::vector<PCB>::iterator it = PCBList.begin(); it < PCBList.end(); ++it) { std::cout << std::setw(5) << (*it).TurnoverTime; SumTurnoverTime += (*it).TurnoverTime;; } std::cout << std::endl; std::cout << std::setw(20) << "带权周转时间"; for (std::vector<PCB>::iterator it = PCBList.begin(); it < PCBList.end(); ++it) { std::cout << std::setw(5) << (*it).WeightedTurnoverTime; SumWeightedTurnoverTime += (*it).WeightedTurnoverTime;; } std::cout << std::endl; std::cout << "平均周转时间: " << (double)SumTurnoverTime / PCBList.size() << std::endl; std::cout << "平均带权周转时间: " << SumWeightedTurnoverTime / PCBList.size() << std::endl; } //比较函数,按ComeTime升序排列 bool CmpByComeTime(const PCB &p1, const PCB &p2) { return p1.ComeTime < p2.ComeTime; }
相关文章推荐
- 关于java中的static
- UVa 11078 Ai-Aj(i<j)的最大值
- html css 内部有浮动元素的div的高度没有被撑开怎么办【转载】
- StrobeMediaPlayback的Javascript桥接
- 文件、目录和用户相关的一些shell命令学习
- 第一次开通技术博客,标记一下
- JavaScript中null和undefined的区别
- 知乎收藏夹
- db2像oracle一样使用hints(guidelines)
- python入门(5)
- Android自定义布局系列之一:流式布局(含TextView的点击事件)
- How do I get started with Node.js
- poj1840
- [知其然不知其所以然-12] intel_pstate忘记对cpu区别对待导致的问题
- 网页中加入VLC的播放RTSP流的控件(笔记)
- Ubuntu - Atom 编辑器 酷炫插件(activate-power-mode)
- 没有躲过的坑--std::string初始化、最快速判断字符串为空
- storm配置
- 没有躲过的坑--std::string初始化、最快速判断字符串为空
- 【C语言提高19】【字符串模型】两头堵模型