UISegmentedControl用法详解
2015-11-25 14:34
411 查看
1、基本用法:
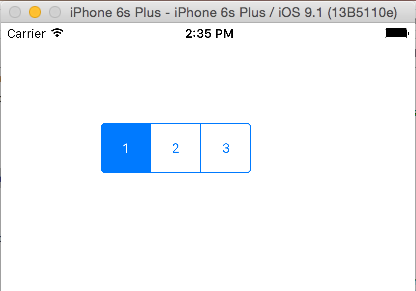
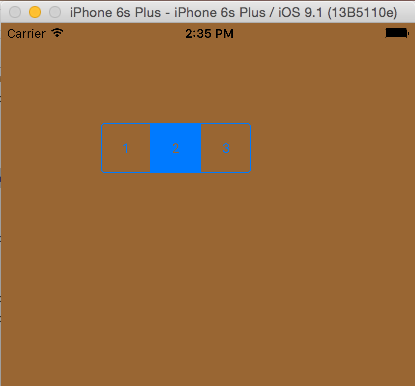
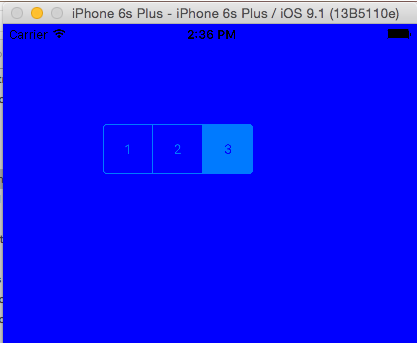
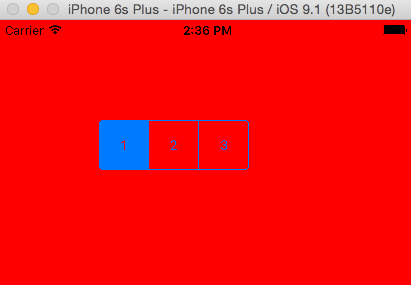
2、设置选中部分的颜色
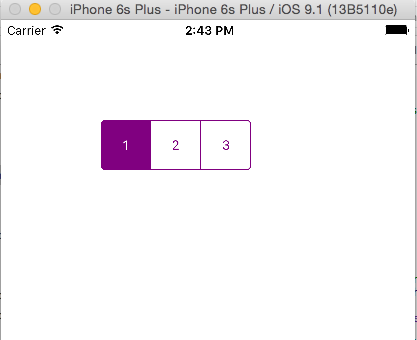
3、注:ios7后segmentedControlStyle属性不再有任何影响
4、不显示选中部分状态
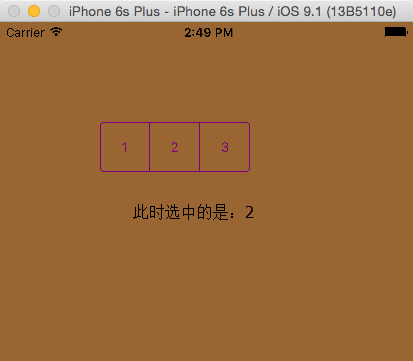
5、使用图片
使用图片前要先说一下,并不是所有的图片都可以,需要透明全品质图片。如果没有,可以有简单的方法自己制作一些透明全品质图片做测试用,下面给出制作方法:
(当然,如果你是PS大神,那就不用看了,那种图片还不是手到擒来)
首先,进入在线PS:http://www.webps.cn/
创建一张新图片:
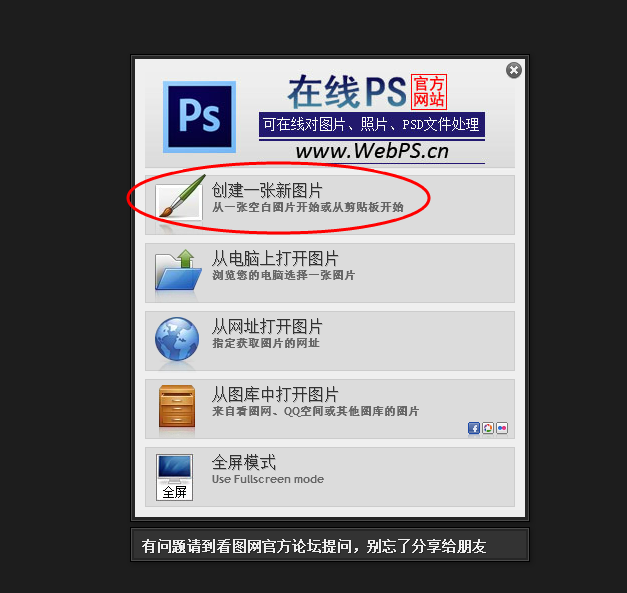
设置一下图片大小:
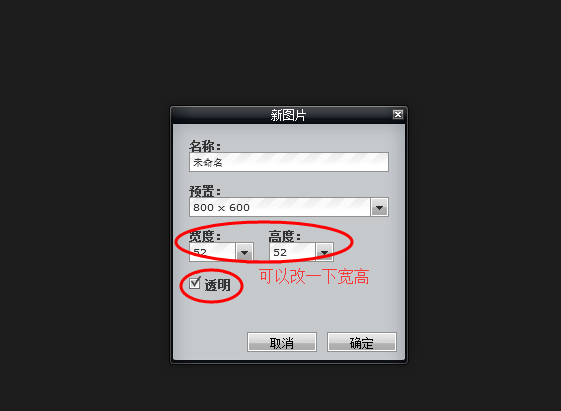
在图片上随便加一些文字,然后保存为透明,全品质图像:
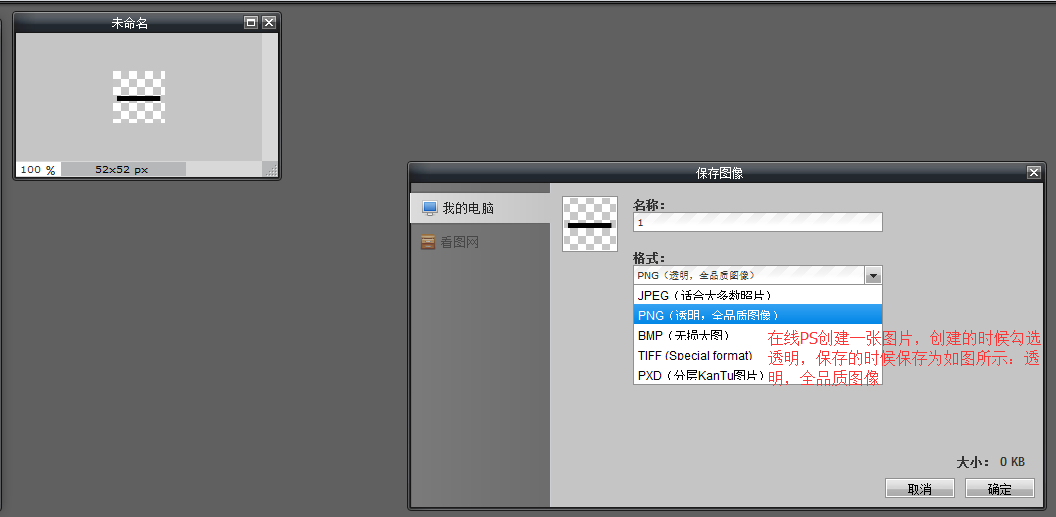
这样测试图片就有了。
将UISegmentControl的每一部分用图片表示:
测试代码:
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
self.view.backgroundColor = [UIColor whiteColor];
UIImage * pic1Image = [UIImage imageNamed:@"my1.png"];
UIImage * pic2Image = [UIImage imageNamed:@"my2.png"];
UIImage * pic3Image = [UIImage imageNamed:@"my3.png"];
NSArray * images = @[pic1Image, pic2Image, pic3Image];
UISegmentedControl * segmented = [[UISegmentedControl alloc] initWithItems:images];
segmented.frame = CGRectMake(100, 100, 150, 50);
segmented.selectedSegmentIndex = 0; //设置默认选中项
[segmented addTarget:self action:@selector(segmentedAction:) forControlEvents:UIControlEventValueChanged];
[self.view addSubview:segmented];
// NSUInteger numbers = segmented.numberOfSegments; // 返回总共有几个部分,只读属性
// segmented.tintColor = [UIColor purpleColor]; //设置选中部分的颜色
// segmented.momentary = YES; //默认为NO YES表示按钮按下后很快恢复
}
- (void) segmentedAction:(id)sender {
if ([sender isKindOfClass:[UISegmentedControl class]]) {
UISegmentedControl * segment = sender;
if (segment.selectedSegmentIndex == 0) {
//点击换为另外的图片
UIImage * image = [UIImage imageNamed:@"yuan.png"];
[segment setImage:image forSegmentAtIndex:0];
// self.view.backgroundColor = [UIColor redColor];
}else if (segment.selectedSegmentIndex == 1) {
//点击换为文字
[segment setTitle:@"second" forSegmentAtIndex:1];
// self.view.backgroundColor = [UIColor brownColor];
}else if (segment.selectedSegmentIndex == 2) {
// self.view.backgroundColor = [UIColor blueColor];
}
}
}初始:
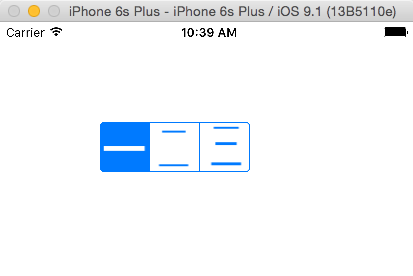
点击二,变为文字second:
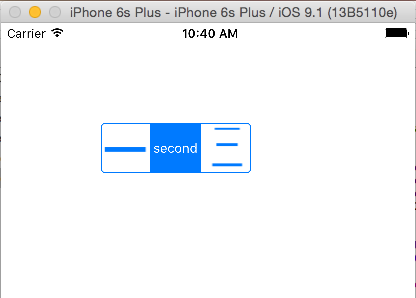
点击三,无变化:
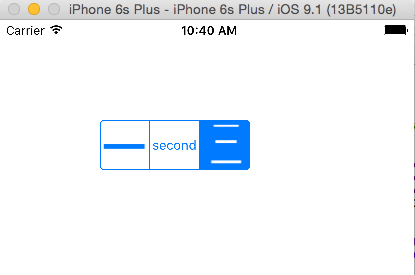
点击一,变为另外的图片:
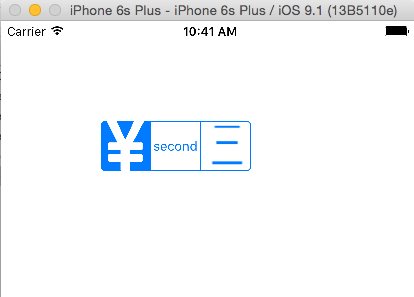
6、某一选项设置为不可选择:
[segmented setEnabled:NO forSegmentAtIndex:1];设置为NO即为不可选择
7、插入删除:
#import "ViewController.h"
@interface ViewController ()
{
UISegmentedControl * segmented;
}
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
self.view.backgroundColor = [UIColor whiteColor];
segmented = [[UISegmentedControl alloc] initWithItems:@[@"1", @"2", @"3"]];
segmented.frame = CGRectMake(100, 100, 150, 50);
segmented.selectedSegmentIndex = 0; //设置默认选中项
[self.view addSubview:segmented];
UIButton * btn1 = [UIButton buttonWithType:UIButtonTypeRoundedRect];
btn1.frame = CGRectMake(100, 200, 50, 30);
[btn1 setTitle:@"插入" forState:UIControlStateNormal];
[btn1 setBackgroundColor:[UIColor brownColor]];
[self.view addSubview:btn1];
[btn1 addTarget:self action:@selector(insertSeg) forControlEvents:UIControlEventTouchUpInside];
UIButton * btn2 = [UIButton buttonWithType:UIButtonTypeRoundedRect];
btn2.frame = CGRectMake(180, 200, 50, 30);
[btn2 setTitle:@"删除" forState:UIControlStateNormal];
[btn2 setBackgroundColor:[UIColor brownColor]];
[self.view addSubview:btn2];
[btn2 addTarget:self action:@selector(deleteSeg) forControlEvents:UIControlEventTouchUpInside];
// NSUInteger numbers = segmented.numberOfSegments; // 返回总共有几个部分,只读属性
// segmented.tintColor = [UIColor purpleColor]; //设置选中部分的颜色
// segmented.momentary = YES; //默认为NO YES表示按钮按下后很快恢复
// [segmented setEnabled:NO forSegmentAtIndex:1];
}
- (void) insertSeg {
[segmented insertSegmentWithTitle:@"insert" atIndex:segmented.selectedSegmentIndex animated:YES];
}
- (void) deleteSeg {
[segmented removeSegmentAtIndex:segmented.selectedSegmentIndex animated:YES];
}初始:
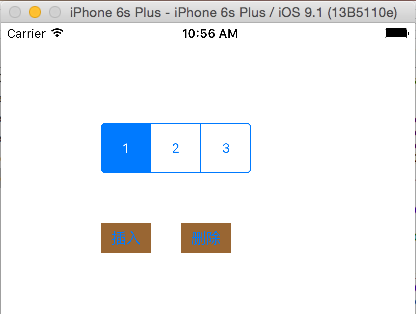
点击插入:
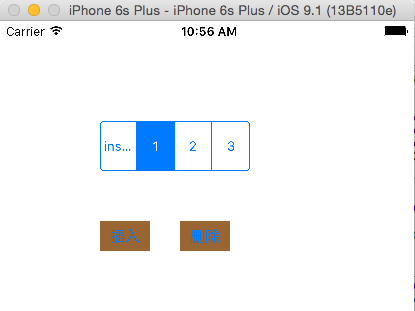
点击删除:
- (void)viewDidLoad { [super viewDidLoad]; // Do any additional setup after loading the view, typically from a nib. self.view.backgroundColor = [UIColor whiteColor]; UISegmentedControl * segmented = [[UISegmentedControl alloc] initWithItems:@[@"1", @"2", @"3"]]; segmented.frame = CGRectMake(100, 100, 150, 50); segmented.selectedSegmentIndex = 0; //设置默认选中项 [segmented addTarget:self action:@selector(segmentedAction:) forControlEvents:UIControlEventValueChanged]; //添加事件 [self.view addSubview:segmented]; } - (void) segmentedAction:(id)sender { if ([sender isKindOfClass:[UISegmentedControl class]]) { UISegmentedControl * segment = sender; if (segment.selectedSegmentIndex == 0) { self.view.backgroundColor = [UIColor redColor]; }else if (segment.selectedSegmentIndex == 1) { self.view.backgroundColor = [UIColor brownColor]; }else if (segment.selectedSegmentIndex == 2) { self.view.backgroundColor = [UIColor blueColor]; } } }
2、设置选中部分的颜色
NSUInteger numbers = segmented.numberOfSegments; // 返回总共有几个部分,只读属性 segmented.tintColor = [UIColor purpleColor]; //设置选中部分的颜色
3、注:ios7后segmentedControlStyle属性不再有任何影响
4、不显示选中部分状态
segmented.momentary = YES; //默认为NO
5、使用图片
使用图片前要先说一下,并不是所有的图片都可以,需要透明全品质图片。如果没有,可以有简单的方法自己制作一些透明全品质图片做测试用,下面给出制作方法:
(当然,如果你是PS大神,那就不用看了,那种图片还不是手到擒来)
首先,进入在线PS:http://www.webps.cn/
创建一张新图片:
设置一下图片大小:
在图片上随便加一些文字,然后保存为透明,全品质图像:
这样测试图片就有了。
将UISegmentControl的每一部分用图片表示:
测试代码:
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
self.view.backgroundColor = [UIColor whiteColor];
UIImage * pic1Image = [UIImage imageNamed:@"my1.png"];
UIImage * pic2Image = [UIImage imageNamed:@"my2.png"];
UIImage * pic3Image = [UIImage imageNamed:@"my3.png"];
NSArray * images = @[pic1Image, pic2Image, pic3Image];
UISegmentedControl * segmented = [[UISegmentedControl alloc] initWithItems:images];
segmented.frame = CGRectMake(100, 100, 150, 50);
segmented.selectedSegmentIndex = 0; //设置默认选中项
[segmented addTarget:self action:@selector(segmentedAction:) forControlEvents:UIControlEventValueChanged];
[self.view addSubview:segmented];
// NSUInteger numbers = segmented.numberOfSegments; // 返回总共有几个部分,只读属性
// segmented.tintColor = [UIColor purpleColor]; //设置选中部分的颜色
// segmented.momentary = YES; //默认为NO YES表示按钮按下后很快恢复
}
- (void) segmentedAction:(id)sender {
if ([sender isKindOfClass:[UISegmentedControl class]]) {
UISegmentedControl * segment = sender;
if (segment.selectedSegmentIndex == 0) {
//点击换为另外的图片
UIImage * image = [UIImage imageNamed:@"yuan.png"];
[segment setImage:image forSegmentAtIndex:0];
// self.view.backgroundColor = [UIColor redColor];
}else if (segment.selectedSegmentIndex == 1) {
//点击换为文字
[segment setTitle:@"second" forSegmentAtIndex:1];
// self.view.backgroundColor = [UIColor brownColor];
}else if (segment.selectedSegmentIndex == 2) {
// self.view.backgroundColor = [UIColor blueColor];
}
}
}初始:
点击二,变为文字second:
点击三,无变化:
点击一,变为另外的图片:
6、某一选项设置为不可选择:
[segmented setEnabled:NO forSegmentAtIndex:1];设置为NO即为不可选择
7、插入删除:
#import "ViewController.h"
@interface ViewController ()
{
UISegmentedControl * segmented;
}
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
self.view.backgroundColor = [UIColor whiteColor];
segmented = [[UISegmentedControl alloc] initWithItems:@[@"1", @"2", @"3"]];
segmented.frame = CGRectMake(100, 100, 150, 50);
segmented.selectedSegmentIndex = 0; //设置默认选中项
[self.view addSubview:segmented];
UIButton * btn1 = [UIButton buttonWithType:UIButtonTypeRoundedRect];
btn1.frame = CGRectMake(100, 200, 50, 30);
[btn1 setTitle:@"插入" forState:UIControlStateNormal];
[btn1 setBackgroundColor:[UIColor brownColor]];
[self.view addSubview:btn1];
[btn1 addTarget:self action:@selector(insertSeg) forControlEvents:UIControlEventTouchUpInside];
UIButton * btn2 = [UIButton buttonWithType:UIButtonTypeRoundedRect];
btn2.frame = CGRectMake(180, 200, 50, 30);
[btn2 setTitle:@"删除" forState:UIControlStateNormal];
[btn2 setBackgroundColor:[UIColor brownColor]];
[self.view addSubview:btn2];
[btn2 addTarget:self action:@selector(deleteSeg) forControlEvents:UIControlEventTouchUpInside];
// NSUInteger numbers = segmented.numberOfSegments; // 返回总共有几个部分,只读属性
// segmented.tintColor = [UIColor purpleColor]; //设置选中部分的颜色
// segmented.momentary = YES; //默认为NO YES表示按钮按下后很快恢复
// [segmented setEnabled:NO forSegmentAtIndex:1];
}
- (void) insertSeg {
[segmented insertSegmentWithTitle:@"insert" atIndex:segmented.selectedSegmentIndex animated:YES];
}
- (void) deleteSeg {
[segmented removeSegmentAtIndex:segmented.selectedSegmentIndex animated:YES];
}初始:
点击插入:
点击删除:
相关文章推荐
- 设定UISegmentControl的文字属性
- UISegmentControl的用法
- iOS基础 -- UISegmentControl
- IOS学习笔记(七)之UISegmentedControl分段控件的基本概念和使用方法
- IOS学习笔记(七)之UISegmentedControl分段控件的基本概念和使用方法
- UISegmentedControl的详细使用
- UISegmentedControl在storyboard下的使用,支持横评
- iOS——UISegmentControl
- easyui tree 默认选中第一个节点
- CF 559B Equivalent Strings
- Android UI ------ActionBar
- UIImage 和 UIColor 互转
- UISwitch用法详解
- poj-2749 Building roads
- MIUI7的优化。
- Excel表格自动生成GUID(宏)
- Machine Learning week 5 quiz: programming assignment-Multi-Neural Network Learning
- UIView中间透明周围半透明(四种方法)
- easyUI级联
- UITableView相关