Office 365 Code Snippets for Windows
2015-11-17 16:25
489 查看
The Office 365 Windows Snippets project contains a repository of code snippets that show you how to use the client libraries from the Office 365 API tools to interact with Office 365 objects, including users, groups, calendars, contacts, mail, files,
and folders.
These snippets are simple and self-contained, and you can copy and paste them into your own code, whenever appropriate, or use them as a resource for learning how to use the client libraries.
The image below shows what you'll see when you launch the app.
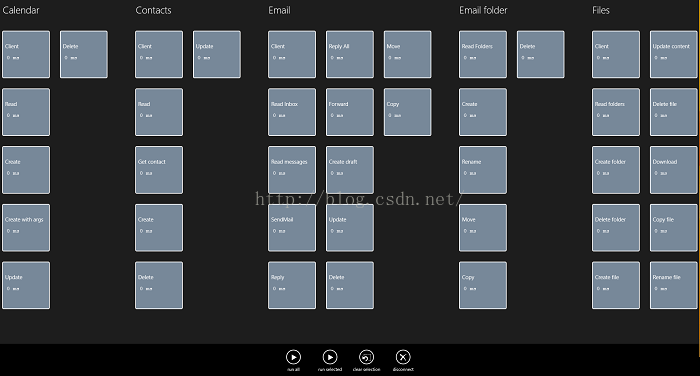
You can choose to run all of the snippets, or just the ones you select. After you choose to run, you’ll be prompted to authenticate with your Office 365 account credentials, and the snippets will run.
Note: This project contains code that authenticates and connects a user to Office 365, but if you want to learn about authentication specifically, look at the Connecting
to Office 365 in Windows Store, Phone, and universal apps.
Visual Studio 2013 with Update 4.
Office 365 API Tools version 1.4.50428.2.
An Office 365 account. You can sign up for an Office 365 Developer subscription that
includes the resources that you need to start building Office 365 apps.
Register
You can register the app with the Office 365 API Tools for Visual Studio. Be sure to download and install the Office
365 API tools from the Visual Studio Gallery.
Note: If you see any errors while installing packages (for example, Unable to find "Microsoft.IdentityModel.Clients.ActiveDirectory") make sure the local path
where you placed the solution is not too long/deep. Moving the solution closer to the root of your drive resolves this issue.
Open the O365-Win-Snippets.sln file using Visual Studio 2013.
Build the solution. The NuGet Package Restore feature will load the assemblies listed in the packages.config file. You should do this before adding connected services in the following steps so that you don't get older versions of some assemblies.
In the Solution Explorer window, right-click each project name and select Add -> Connected Service.
A Services Manager dialog box will appear. Choose Office 365 and then Register your app.
On the sign-in dialog box, enter the user name and password for your Office 365 tenant. This user name will often follow the pattern @.onmicrosoft.com. If you don't already have an Office 365 tenant, you can get a free Developer Site
as part of your MSDN Benefits or sign up for a free trial. After you're signed in, you will see a list of all the services. No permissions will be selected, since the app is not registered to use any services yet.
To register for the services used in this sample, choose the following services and permissions:
Calendar - Read and write to your calendars.
Contacts - Read and write to your contacts.
Mail - Read and write to your mail. Send mail as you.
My Files - Read and write your files.
Users and Groups - Sign you in and read your profile. Access your organization's directory.
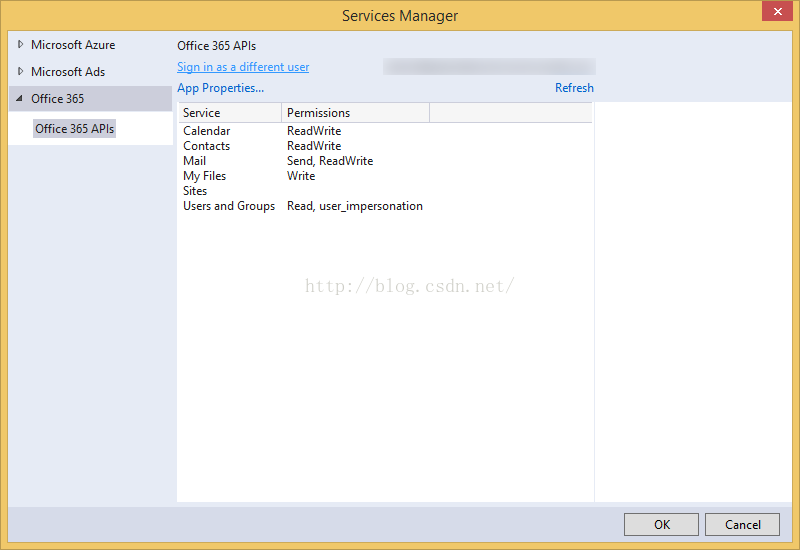
After you click OK in the Services Manager dialog box, you can select Build Solution from the Build
menu to load the Microsoft.IdentityModel.Clients.ActiveDirectory assembly, or you can wait until you debug.
Build
After you've loaded the solution in Visual Studio, press F5 to build and debug. Run the solution and sign in with your organizational account to Office 365.
How
This sample runs REST commands that create, read, update, or delete data. When running commands that delete or edit data, the sample creates fake entities. The fake entities are deleted or edited so that your actual tenant data is unaffected. The
sample will leave behind fake entities on your tenant.
Add
This project includes five snippets files: Calendar\CalendarSnippets.cs, Contacts\ContactsSnippets.cs, Email\EmailSnippets.cs, Files\FilesSnippets.cs, and UsersAndGroups\UsersAndGroupsSnippets.cs.
If you have a snippet of your own and you would like to run it in this project, just follow these three steps:
Add your snippet to the snippets file. Be sure to include a try/catch block. The snippet below is an example of a simple snippet that gets one page of calendar events:
Create a story that uses your snippet and add it to the associated stories file. For example, the
uses the
file:
Sometimes your story will need to run snippets in addition to the one that you're implementing. For example, if you want to update an event, you first need to use the
to create an event. Then you can update it. Always be sure to use snippets that already exist in the snippets file. If the operation you need doesn't exist, you'll have to create it and then include it in your story. It's a best practice to delete any entities
that you create in a story, especially if you're working on anything other than a test or developer tenant.
Add your story to the story collection in MainPageXaml.cs (inside the
Now you can test your snippet. When you run the app, your snippet will appear as a new box in the grid. Select the box for your snippet, and then run it. Use this as an opportunity to debug your snippet.
The Office 365 Windows Snippets project contains a repository of code snippets that show you how to use the client libraries from the Office 365 API tools to interact with Office 365 objects, including users, groups, calendars, contacts, mail, files,
and folders.
These snippets are simple and self-contained, and you can copy and paste them into your own code, whenever appropriate, or use them as a resource for learning how to use the client libraries.
The image below shows what you'll see when you launch the app.
You can choose to run all of the snippets, or just the ones you select. After you choose to run, you’ll be prompted to authenticate with your Office 365 account credentials, and the snippets will run.
Note: This project contains code that authenticates and connects a user to Office 365, but if you want to learn about authentication specifically, look at the Connecting
to Office 365 in Windows Store, Phone, and universal apps.
Prerequisites
This sample requires the following:Visual Studio 2013 with Update 4.
Office 365 API Tools version 1.4.50428.2.
An Office 365 account. You can sign up for an Office 365 Developer subscription that
includes the resources that you need to start building Office 365 apps.
Register
and configure the app
You can register the app with the Office 365 API Tools for Visual Studio. Be sure to download and install the Office365 API tools from the Visual Studio Gallery.
Note: If you see any errors while installing packages (for example, Unable to find "Microsoft.IdentityModel.Clients.ActiveDirectory") make sure the local path
where you placed the solution is not too long/deep. Moving the solution closer to the root of your drive resolves this issue.
Open the O365-Win-Snippets.sln file using Visual Studio 2013.
Build the solution. The NuGet Package Restore feature will load the assemblies listed in the packages.config file. You should do this before adding connected services in the following steps so that you don't get older versions of some assemblies.
In the Solution Explorer window, right-click each project name and select Add -> Connected Service.
A Services Manager dialog box will appear. Choose Office 365 and then Register your app.
On the sign-in dialog box, enter the user name and password for your Office 365 tenant. This user name will often follow the pattern @.onmicrosoft.com. If you don't already have an Office 365 tenant, you can get a free Developer Site
as part of your MSDN Benefits or sign up for a free trial. After you're signed in, you will see a list of all the services. No permissions will be selected, since the app is not registered to use any services yet.
To register for the services used in this sample, choose the following services and permissions:
Calendar - Read and write to your calendars.
Contacts - Read and write to your contacts.
Mail - Read and write to your mail. Send mail as you.
My Files - Read and write your files.
Users and Groups - Sign you in and read your profile. Access your organization's directory.
After you click OK in the Services Manager dialog box, you can select Build Solution from the Build
menu to load the Microsoft.IdentityModel.Clients.ActiveDirectory assembly, or you can wait until you debug.
Build
and debug
After you've loaded the solution in Visual Studio, press F5 to build and debug. Run the solution and sign in with your organizational account to Office 365.How
the sample affects your tenant data
This sample runs REST commands that create, read, update, or delete data. When running commands that delete or edit data, the sample creates fake entities. The fake entities are deleted or edited so that your actual tenant data is unaffected. Thesample will leave behind fake entities on your tenant.
Add
a snippet
This project includes five snippets files: Calendar\CalendarSnippets.cs, Contacts\ContactsSnippets.cs, Email\EmailSnippets.cs, Files\FilesSnippets.cs, and UsersAndGroups\UsersAndGroupsSnippets.cs.If you have a snippet of your own and you would like to run it in this project, just follow these three steps:
Add your snippet to the snippets file. Be sure to include a try/catch block. The snippet below is an example of a simple snippet that gets one page of calendar events:
public static async Task<List<IEvent>> GetCalendarEventsAsync() { try { // Make sure we have a reference to the Exchange client OutlookServicesClient client = await GetOutlookClientAsync(); IPagedCollection<IEvent> eventsResults = await client.Me.Calendar.Events.ExecuteAsync(); // You can access each event as follows. if (eventsResults.CurrentPage.Count > 0) { string eventId = eventsResults.CurrentPage[0].Id; Debug.WriteLine("First event:" + eventId); } return eventsResults.CurrentPage.ToList(); } catch { return null; } }
Create a story that uses your snippet and add it to the associated stories file. For example, the
TryCreateCalendarEventAsync()story
uses the
AddCalendarEventAsync ()snippet inside the Calendar\CalendarStories.cs
file:
public static async Task<bool> TryCreateCalendarEventAsync() { var newEventId = await CalendarSnippets.AddCalendarEventAsync(); if (newEventId == null) return false; //Cleanup await CalendarSnippets.DeleteCalendarEventAsync(newEventId); return true; }
Sometimes your story will need to run snippets in addition to the one that you're implementing. For example, if you want to update an event, you first need to use the
AddCalendarEventAsync()method
to create an event. Then you can update it. Always be sure to use snippets that already exist in the snippets file. If the operation you need doesn't exist, you'll have to create it and then include it in your story. It's a best practice to delete any entities
that you create in a story, especially if you're working on anything other than a test or developer tenant.
Add your story to the story collection in MainPageXaml.cs (inside the
CreateTestList()method):
StoryCollection.Add(new StoryDefinition() { GroupName = "Calendar", Title = "Create", RunStoryAsync = CalendarStories.TryCreateCalendarEventAsync });
Now you can test your snippet. When you run the app, your snippet will appear as a new box in the grid. Select the box for your snippet, and then run it. Use this as an opportunity to debug your snippet.
相关文章推荐
- Android Manifest 用法
- Spark RDD API详解(一) Map和Reduce
- 如何重装TCP/IP协议
- Windows 8 官方高清壁纸欣赏与下载
- 谁是桌面王者?Win PK Linux三大镇山之宝
- 对《大家都在点赞 Windows Terminal,我决定给你泼一盆冷水》一文的商榷
- Windows Clang开发环境备忘
- 从Windows系统下访问Linux分区相关软件
- 对《大家都在点赞 Windows Terminal,我决定给你泼一盆冷水》一文的商榷
- Windows下搭建本地SVN服务器
- Visual Studio 2012 示例代码浏览器 - 数以千计的开发示例近在手边,唾手可得
- Visual Studio 2012 示例代码浏览器 - 数以千计的开发示例近在手边,唾手可得
- 微软镜像下载
- windows server域用户提升到本地更高权限组中的方法
- 使用命令修改注册表键值及权限
- 通过手机、电脑远程开关机,Windows和linux机手机,电脑相互控制
- Windows XP最新应用技巧大荟萃
- Windows 系统组策略应用全攻略(上)第1/2页