五,一个可以左右滑动的关卡选择界面
2015-11-03 23:51
465 查看
依旧使用stage,因为关卡的总数可能变化,所以写了这个自适应关卡数的关卡选择界面。
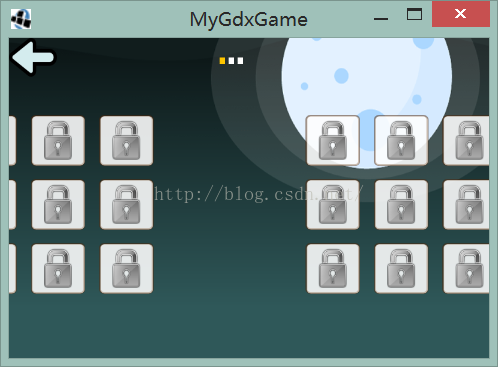
package com.hj.lee.game.screens;
import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.InputMultiplexer;
import com.badlogic.gdx.InputProcessor;
import com.badlogic.gdx.graphics.Color;
import com.badlogic.gdx.graphics.GL20;
import com.badlogic.gdx.graphics.OrthographicCamera;
import com.badlogic.gdx.graphics.g2d.BitmapFont;
import com.badlogic.gdx.graphics.g2d.SpriteBatch;
import com.badlogic.gdx.input.GestureDetector;
import com.badlogic.gdx.input.GestureDetector.GestureListener;
import com.badlogic.gdx.math.Interpolation;
import com.badlogic.gdx.math.Vector2;
import com.badlogic.gdx.math.Vector3;
import com.badlogic.gdx.scenes.scene2d.InputEvent;
import com.badlogic.gdx.scenes.scene2d.Stage;
import com.badlogic.gdx.scenes.scene2d.ui.Image;
import com.badlogic.gdx.scenes.scene2d.utils.ActorGestureListener;
import com.badlogic.gdx.scenes.scene2d.utils.TextureRegionDrawable;
import com.badlogic.gdx.utils.Array;
import com.badlogic.gdx.utils.viewport.FitViewport;
import com.badlogic.gdx.utils.viewport.StretchViewport;
import com.badlogic.gdx.utils.viewport.Viewport;
import com.hj.lee.game.DirectedGame;
import com.hj.lee.game.assets.Assets;
import com.hj.lee.game.assets.Constants;
import com.hj.lee.game.assets.GamePreferences;
import com.hj.lee.game.screens.actor.ButtonActor;
import com.hj.lee.game.screens.actor.ImagesActor;
import com.hj.lee.game.screens.transitions.ScreenTransition;
import com.hj.lee.game.screens.transitions.ScreenTransitionFade;
import com.hj.lee.game.screens.transitions.ScreenTransitionSlide;
import com.hj.lee.game.utils.CameraHelper;
import com.hj.lee.game.utils.GameObject;
import com.hj.lee.game.utils.RunTimeData;
public class LevelScreen extends AbstractGameScreen implements GestureListener {
public static final String TAG = LevelScreen.class.getName();
Stage stage;
Viewport viewport;//视图
Image bgImage;//背景图片
ButtonActor preScreenButton;
GestureDetector gestureDetector;
InputMultiplexer multiplexer;//这个屏幕的输入接收,如果使用libgdx自带的输入可能会出现屏幕间的串屏点击事件
Array<ImagesActor> levelIcons;
SpriteBatch batch;
Vector2 lockSize;
BitmapFont fnt;
CameraHelper camHelper;//屏幕可以左右移动,所以使用了自定义的相机帮助类,同样的,相机类也可以用在游戏的主界面
Array<Vector2> levelDotsInfo;
Color color;
float camBasePositionX;
boolean isPanned = false;
// row length
int row = 3;
// column length
int column = 5;
// pages number
int pages;
// current page
int currentPage = 1;
public LevelScreen(DirectedGame game) {
super(game);
// TODO Auto-generated constructor stub
initMembers();
initPages();
buildStage();
initLevelIcon();
initCameraHelper();
}
private void initMembers() {
multiplexer = new InputMultiplexer();
viewport = new StretchViewport(Constants.SCREEN_PIXEL_WIDTH, Constants.SCREEN_PIXEL_HEIGHT);
stage = new Stage(viewport);
batch = new SpriteBatch();
gestureDetector = new GestureDetector(this);
multiplexer.addProcessor(gestureDetector);
multiplexer.addProcessor(stage);
levelIcons = new Array<ImagesActor>();
lockSize = new Vector2(48, 60);
fnt = Assets.instance.fnt.bitmapFont;
levelDotsInfo = new Array<Vector2>();
color = new Color();
}
private void initCameraHelper() {
camHelper = new CameraHelper();
camHelper.setPosition(Constants.SCREEN_PIXEL_WIDTH / 2, Constants.SCREEN_PIXEL_HEIGHT / 2);
camHelper.applyTo((OrthographicCamera) stage.getCamera());
}
private void initLevelIcon() {
// TODO Auto-generated method stub
float column_height = Constants.SCREEN_PIXEL_HEIGHT / (row + 2);
float row_width = Constants.SCREEN_PIXEL_WIDTH / (column + 2);
// System.out.println("column_height:" + column_height + "row_width:" + row_width + TAG);
float x = 0;
float y = 0;
for (int p = 1; p <= pages; p++) {
for (int i = 0; i < row; i++) {
for (int j = 0; j < column; j++) {
if (i * column + j + 1 + (p - 1) * row * column > Constants.LEVEL_NUMBERS) {
return;
}
x = row_width + (j + 1) * row_width - row_width / 2 + (p - 1) * Constants.SCREEN_PIXEL_WIDTH;
y = Constants.SCREEN_PIXEL_HEIGHT - (column_height + (i + 1) * column_height - column_height / 2);
// System.out.println("x:" + x + " y:" + y + " " + (i * column + j + 1 + (p - 1) * row * column));
final ImagesActor ia = new ImagesActor(new TextureRegionDrawable(Assets.instance.atlas_ui.findRegion(Assets.levelBorad)), new GameObject(x
- row_width / 2, y - column_height / 2, (float) (row_width * 0.8), (float) (column_height * 0.8)), 1.2f);
ia.id = i * column + j + 1 + (p - 1) * row * column;
ia.addListener(new ActorGestureListener() {
public void touchDown(InputEvent event, float x, float y, int pointer, int button) {
ia.touchDown();
}
public void touchUp(InputEvent event, float x, float y, int pointer, int button) {
ia.touchUp();
if (!isPanned && ia.id <= GamePreferences.instance.levelCompleted) {
ScreenTransition transition = ScreenTransitionFade.init(0.3f);
RunTimeData.choosenLevel = ia.id;
game.setScreen(new GameScreen(game), transition);
}
}
});
levelIcons.add(ia);
stage.addActor(ia);
}
}
}
}
private void initPages() {
// TODO Auto-generated method stub
if (Constants.LEVEL_NUMBERS % (row * column) > 0) {
pages = Constants.LEVEL_NUMBERS / (row * column) + 1;
} else
pages = Constants.LEVEL_NUMBERS / (row * column);
for (int i = 0; i < pages; i++) {
Vector2 vec = new Vector2();
levelDotsInfo.add(vec);
}
}
private void buildStage() {
// TODO Auto-generated method stub
bgImage = new Image(new TextureRegionDrawable(Assets.instance.atlas_ui.findRegion(Assets.background)));
preScreenButton = new ButtonActor(new TextureRegionDrawable(Assets.instance.atlas_ui.findRegion(Assets.back)), new GameObject(0, 0, 80, 58), 1.2f);
preScreenButton.addListener(new ActorGestureListener() {
public void touchDown(InputEvent event, float x, float y, int pointer, int button) {
preScreenButton.touchDown();
}
public void touchUp(InputEvent event, float x, float y, int pointer, int button) {
preScreenButton.touchUp();
// if (!isPanned) {
// onPreScreenButtonClicked();
// }
onPreScreenButtonClicked();
}
});
stage.addActor(bgImage);
stage.addActor(preScreenButton);
}
protected void onPreScreenButtonClicked() {
// TODO Auto-generated method stub
ScreenTransition transition = ScreenTransitionSlide.init(0.75f, ScreenTransitionSlide.LEFT, false, Interpolation.bounceOut);
game.setScreen(new MenuScreen(game), transition);
}
@Override
public void render(float deltaTime) {
// TODO Auto-generated method stub
updateInfo();
updateButton();
Gdx.gl.glClearColor(0.0f, 0.0f, 0.0f, 1.0f);
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
stage.act(deltaTime);
stage.draw();
batch.setProjectionMatrix(stage.getCamera().combined);
batch.begin();
renderLevelIconsNumber();
drawInfo();
batch.end();
camHelper.update(deltaTime);
}
private void drawInfo() {
// TODO Auto-generated method stub
for (int i = 0; i < pages; i++) {
if (i + 1 == currentPage) {
color = fnt.getColor();
fnt.setColor(Color.ORANGE);
fnt.draw(batch, ".", stage.getCamera().position.x - 50 + i * 15, stage.getCamera().position.y + stage.getCamera().viewportHeight / 2);
fnt.setColor(color);
} else {
fnt.draw(batch, ".", stage.getCamera().position.x - 50 + i * 15, stage.getCamera().position.y + stage.getCamera().viewportHeight / 2);
}
}
}
private void updateInfo() {
// TODO Au
4000
to-generated method stub
}
private void renderLevelIconsNumber() {
// TODO Auto-generated method stub
color = fnt.getColor();
fnt.setColor(Color.OLIVE);
for (ImagesActor ia : levelIcons) {
if (ia.id <= GamePreferences.instance.levelCompleted) {
if (ia.id < 10) {
fnt.draw(batch, String.valueOf(ia.id), ia.gameObject.position.x + ia.gameObject.size.x / 2 - 15, ia.gameObject.position.y
+ ia.gameObject.size.y / 2 + 20);
} else {
fnt.draw(batch, String.valueOf(ia.id), ia.gameObject.position.x + ia.gameObject.size.x / 2 - 25, ia.gameObject.position.y
+ ia.gameObject.size.y / 2 + 20);
}
} else {
batch.draw(Assets.instance.atlas_ui.findRegion(Assets.levelLock), ia.gameObject.position.x + ia.gameObject.size.x / 2 - lockSize.x / 2,
ia.gameObject.position.y + ia.gameObject.size.y / 2 - lockSize.y / 2);
}
}
fnt.setColor(color);
}
private void updateButton() {
// TODO Auto-generated method stub
preScreenButton.updatePosition(stage.getCamera().position.x - stage.getCamera().viewportWidth / 2, stage.getCamera().position.y
+ stage.getCamera().viewportHeight / 2 - preScreenButton.gameObject.size.y);
bgImage.setPosition(stage.getCamera().position.x - bgImage.getWidth() / 2, stage.getCamera().position.y - bgImage.getHeight() / 2);
}
@Override
public void resize(int width, int height) {
// TODO Auto-generated method stub
viewport.setScreenSize(width, height);
}
@Override
public void show() {
// TODO Auto-generated method stub
}
@Override
public void hide() {
// TODO Auto-generated method stub
}
@Override
public void pause() {
// TODO Auto-generated method stub
}
@Override
public void dispose() {
// TODO Auto-generated method stub
stage.dispose();
}
@Override
public InputProcessor getInputProcessor() {
// TODO Auto-generated method stub
return multiplexer;
}
@Override
public boolean touchDown(float x, float y, int pointer, int button) {
// TODO Auto-generated method stub
System.out.println("gesture detector x:" + x + " y:" + y + "touchDown" + TAG);
isPanned = false;
return false;
}
@Override
public boolean tap(float x, float y, int count, int button) {
// TODO Auto-generated method stub
return false;
}
@Override
public boolean longPress(float x, float y) {
// TODO Auto-generated method stub
System.out.println("long pressed");
return false;
}
@Override
public boolean fling(float velocityX, float velocityY, int button) {
// TODO Auto-generated method stub
System.out.println("gesture detector velocityX:" + velocityX + "velocityY:" + velocityY + "fling" + TAG);
return false;
}
@Override
public boolean pan(float x, float y, float deltaX, float deltaY) {
// TODO Auto-generated method stub
System.out.println("gesture detector deltaX:" + deltaX + "pan" + TAG);
camHelper.disposeTarget();
deltaX = deltaX / (viewport.getScreenWidth() / viewport.getWorldWidth());
camHelper.getCamera().position.set(stage.getCamera().position.x - deltaX, stage.getCamera().position.y, 0);
camBasePositionX += deltaX;
isPanned = true;
return true;
}
@Override
public boolean panStop(float x, float y, int pointer, int button) {
// TODO Auto-generated method stub
System.out.println("gesture detector x:" + x + " y:" + y + "panStop" + TAG);
if (currentPage == 1) {
if (camBasePositionX < -100) {
camHelper.setTarget(new Vector3(currentPage * Constants.SCREEN_PIXEL_WIDTH + Constants.SCREEN_PIXEL_WIDTH / 2, 240, 0));
currentPage++;
} else {
camHelper.setTarget(new Vector3(Constants.SCREEN_PIXEL_WIDTH / 2, 240, 0));
}
} else if (currentPage < pages) {
if (camBasePositionX < -100) {
camHelper.setTarget(new Vector3(currentPage * Constants.SCREEN_PIXEL_WIDTH + Constants.SCREEN_PIXEL_WIDTH / 2, 240, 0));
currentPage++;
} else if (camBasePositionX > 100) {
camHelper.setTarget(new Vector3((currentPage - 2) * Constants.SCREEN_PIXEL_WIDTH + Constants.SCREEN_PIXEL_WIDTH / 2, 240, 0));
currentPage--;
} else {
camHelper.setTarget(new Vector3((currentPage - 1) * Constants.SCREEN_PIXEL_WIDTH + Constants.SCREEN_PIXEL_WIDTH / 2, 240, 0));
}
} else if (currentPage == pages) {
if (camBasePositionX < -100) {
camHelper.setTarget(new Vector3(pages * Constants.SCREEN_PIXEL_WIDTH - Constants.SCREEN_PIXEL_WIDTH / 2, 240, 0));
} else {
camHelper.setTarget(new Vector3((currentPage - 2) * Constants.SCREEN_PIXEL_WIDTH + Constants.SCREEN_PIXEL_WIDTH / 2, 240, 0));
currentPage--;
}
}
camBasePositionX = 0;
return false;
}
@Override
public boolean zoom(float initialDistance, float distance) {
// TODO Auto-generated method stub
return false;
}
@Override
public boolean pinch(Vector2 initialPointer1, Vector2 initialPointer2, Vector2 pointer1, Vector2 pointer2) {
// TODO Auto-generated method stub
return false;
}
}
package com.hj.lee.game.screens;
import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.InputMultiplexer;
import com.badlogic.gdx.InputProcessor;
import com.badlogic.gdx.graphics.Color;
import com.badlogic.gdx.graphics.GL20;
import com.badlogic.gdx.graphics.OrthographicCamera;
import com.badlogic.gdx.graphics.g2d.BitmapFont;
import com.badlogic.gdx.graphics.g2d.SpriteBatch;
import com.badlogic.gdx.input.GestureDetector;
import com.badlogic.gdx.input.GestureDetector.GestureListener;
import com.badlogic.gdx.math.Interpolation;
import com.badlogic.gdx.math.Vector2;
import com.badlogic.gdx.math.Vector3;
import com.badlogic.gdx.scenes.scene2d.InputEvent;
import com.badlogic.gdx.scenes.scene2d.Stage;
import com.badlogic.gdx.scenes.scene2d.ui.Image;
import com.badlogic.gdx.scenes.scene2d.utils.ActorGestureListener;
import com.badlogic.gdx.scenes.scene2d.utils.TextureRegionDrawable;
import com.badlogic.gdx.utils.Array;
import com.badlogic.gdx.utils.viewport.FitViewport;
import com.badlogic.gdx.utils.viewport.StretchViewport;
import com.badlogic.gdx.utils.viewport.Viewport;
import com.hj.lee.game.DirectedGame;
import com.hj.lee.game.assets.Assets;
import com.hj.lee.game.assets.Constants;
import com.hj.lee.game.assets.GamePreferences;
import com.hj.lee.game.screens.actor.ButtonActor;
import com.hj.lee.game.screens.actor.ImagesActor;
import com.hj.lee.game.screens.transitions.ScreenTransition;
import com.hj.lee.game.screens.transitions.ScreenTransitionFade;
import com.hj.lee.game.screens.transitions.ScreenTransitionSlide;
import com.hj.lee.game.utils.CameraHelper;
import com.hj.lee.game.utils.GameObject;
import com.hj.lee.game.utils.RunTimeData;
public class LevelScreen extends AbstractGameScreen implements GestureListener {
public static final String TAG = LevelScreen.class.getName();
Stage stage;
Viewport viewport;//视图
Image bgImage;//背景图片
ButtonActor preScreenButton;
GestureDetector gestureDetector;
InputMultiplexer multiplexer;//这个屏幕的输入接收,如果使用libgdx自带的输入可能会出现屏幕间的串屏点击事件
Array<ImagesActor> levelIcons;
SpriteBatch batch;
Vector2 lockSize;
BitmapFont fnt;
CameraHelper camHelper;//屏幕可以左右移动,所以使用了自定义的相机帮助类,同样的,相机类也可以用在游戏的主界面
Array<Vector2> levelDotsInfo;
Color color;
float camBasePositionX;
boolean isPanned = false;
// row length
int row = 3;
// column length
int column = 5;
// pages number
int pages;
// current page
int currentPage = 1;
public LevelScreen(DirectedGame game) {
super(game);
// TODO Auto-generated constructor stub
initMembers();
initPages();
buildStage();
initLevelIcon();
initCameraHelper();
}
private void initMembers() {
multiplexer = new InputMultiplexer();
viewport = new StretchViewport(Constants.SCREEN_PIXEL_WIDTH, Constants.SCREEN_PIXEL_HEIGHT);
stage = new Stage(viewport);
batch = new SpriteBatch();
gestureDetector = new GestureDetector(this);
multiplexer.addProcessor(gestureDetector);
multiplexer.addProcessor(stage);
levelIcons = new Array<ImagesActor>();
lockSize = new Vector2(48, 60);
fnt = Assets.instance.fnt.bitmapFont;
levelDotsInfo = new Array<Vector2>();
color = new Color();
}
private void initCameraHelper() {
camHelper = new CameraHelper();
camHelper.setPosition(Constants.SCREEN_PIXEL_WIDTH / 2, Constants.SCREEN_PIXEL_HEIGHT / 2);
camHelper.applyTo((OrthographicCamera) stage.getCamera());
}
private void initLevelIcon() {
// TODO Auto-generated method stub
float column_height = Constants.SCREEN_PIXEL_HEIGHT / (row + 2);
float row_width = Constants.SCREEN_PIXEL_WIDTH / (column + 2);
// System.out.println("column_height:" + column_height + "row_width:" + row_width + TAG);
float x = 0;
float y = 0;
for (int p = 1; p <= pages; p++) {
for (int i = 0; i < row; i++) {
for (int j = 0; j < column; j++) {
if (i * column + j + 1 + (p - 1) * row * column > Constants.LEVEL_NUMBERS) {
return;
}
x = row_width + (j + 1) * row_width - row_width / 2 + (p - 1) * Constants.SCREEN_PIXEL_WIDTH;
y = Constants.SCREEN_PIXEL_HEIGHT - (column_height + (i + 1) * column_height - column_height / 2);
// System.out.println("x:" + x + " y:" + y + " " + (i * column + j + 1 + (p - 1) * row * column));
final ImagesActor ia = new ImagesActor(new TextureRegionDrawable(Assets.instance.atlas_ui.findRegion(Assets.levelBorad)), new GameObject(x
- row_width / 2, y - column_height / 2, (float) (row_width * 0.8), (float) (column_height * 0.8)), 1.2f);
ia.id = i * column + j + 1 + (p - 1) * row * column;
ia.addListener(new ActorGestureListener() {
public void touchDown(InputEvent event, float x, float y, int pointer, int button) {
ia.touchDown();
}
public void touchUp(InputEvent event, float x, float y, int pointer, int button) {
ia.touchUp();
if (!isPanned && ia.id <= GamePreferences.instance.levelCompleted) {
ScreenTransition transition = ScreenTransitionFade.init(0.3f);
RunTimeData.choosenLevel = ia.id;
game.setScreen(new GameScreen(game), transition);
}
}
});
levelIcons.add(ia);
stage.addActor(ia);
}
}
}
}
private void initPages() {
// TODO Auto-generated method stub
if (Constants.LEVEL_NUMBERS % (row * column) > 0) {
pages = Constants.LEVEL_NUMBERS / (row * column) + 1;
} else
pages = Constants.LEVEL_NUMBERS / (row * column);
for (int i = 0; i < pages; i++) {
Vector2 vec = new Vector2();
levelDotsInfo.add(vec);
}
}
private void buildStage() {
// TODO Auto-generated method stub
bgImage = new Image(new TextureRegionDrawable(Assets.instance.atlas_ui.findRegion(Assets.background)));
preScreenButton = new ButtonActor(new TextureRegionDrawable(Assets.instance.atlas_ui.findRegion(Assets.back)), new GameObject(0, 0, 80, 58), 1.2f);
preScreenButton.addListener(new ActorGestureListener() {
public void touchDown(InputEvent event, float x, float y, int pointer, int button) {
preScreenButton.touchDown();
}
public void touchUp(InputEvent event, float x, float y, int pointer, int button) {
preScreenButton.touchUp();
// if (!isPanned) {
// onPreScreenButtonClicked();
// }
onPreScreenButtonClicked();
}
});
stage.addActor(bgImage);
stage.addActor(preScreenButton);
}
protected void onPreScreenButtonClicked() {
// TODO Auto-generated method stub
ScreenTransition transition = ScreenTransitionSlide.init(0.75f, ScreenTransitionSlide.LEFT, false, Interpolation.bounceOut);
game.setScreen(new MenuScreen(game), transition);
}
@Override
public void render(float deltaTime) {
// TODO Auto-generated method stub
updateInfo();
updateButton();
Gdx.gl.glClearColor(0.0f, 0.0f, 0.0f, 1.0f);
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
stage.act(deltaTime);
stage.draw();
batch.setProjectionMatrix(stage.getCamera().combined);
batch.begin();
renderLevelIconsNumber();
drawInfo();
batch.end();
camHelper.update(deltaTime);
}
private void drawInfo() {
// TODO Auto-generated method stub
for (int i = 0; i < pages; i++) {
if (i + 1 == currentPage) {
color = fnt.getColor();
fnt.setColor(Color.ORANGE);
fnt.draw(batch, ".", stage.getCamera().position.x - 50 + i * 15, stage.getCamera().position.y + stage.getCamera().viewportHeight / 2);
fnt.setColor(color);
} else {
fnt.draw(batch, ".", stage.getCamera().position.x - 50 + i * 15, stage.getCamera().position.y + stage.getCamera().viewportHeight / 2);
}
}
}
private void updateInfo() {
// TODO Au
4000
to-generated method stub
}
private void renderLevelIconsNumber() {
// TODO Auto-generated method stub
color = fnt.getColor();
fnt.setColor(Color.OLIVE);
for (ImagesActor ia : levelIcons) {
if (ia.id <= GamePreferences.instance.levelCompleted) {
if (ia.id < 10) {
fnt.draw(batch, String.valueOf(ia.id), ia.gameObject.position.x + ia.gameObject.size.x / 2 - 15, ia.gameObject.position.y
+ ia.gameObject.size.y / 2 + 20);
} else {
fnt.draw(batch, String.valueOf(ia.id), ia.gameObject.position.x + ia.gameObject.size.x / 2 - 25, ia.gameObject.position.y
+ ia.gameObject.size.y / 2 + 20);
}
} else {
batch.draw(Assets.instance.atlas_ui.findRegion(Assets.levelLock), ia.gameObject.position.x + ia.gameObject.size.x / 2 - lockSize.x / 2,
ia.gameObject.position.y + ia.gameObject.size.y / 2 - lockSize.y / 2);
}
}
fnt.setColor(color);
}
private void updateButton() {
// TODO Auto-generated method stub
preScreenButton.updatePosition(stage.getCamera().position.x - stage.getCamera().viewportWidth / 2, stage.getCamera().position.y
+ stage.getCamera().viewportHeight / 2 - preScreenButton.gameObject.size.y);
bgImage.setPosition(stage.getCamera().position.x - bgImage.getWidth() / 2, stage.getCamera().position.y - bgImage.getHeight() / 2);
}
@Override
public void resize(int width, int height) {
// TODO Auto-generated method stub
viewport.setScreenSize(width, height);
}
@Override
public void show() {
// TODO Auto-generated method stub
}
@Override
public void hide() {
// TODO Auto-generated method stub
}
@Override
public void pause() {
// TODO Auto-generated method stub
}
@Override
public void dispose() {
// TODO Auto-generated method stub
stage.dispose();
}
@Override
public InputProcessor getInputProcessor() {
// TODO Auto-generated method stub
return multiplexer;
}
@Override
public boolean touchDown(float x, float y, int pointer, int button) {
// TODO Auto-generated method stub
System.out.println("gesture detector x:" + x + " y:" + y + "touchDown" + TAG);
isPanned = false;
return false;
}
@Override
public boolean tap(float x, float y, int count, int button) {
// TODO Auto-generated method stub
return false;
}
@Override
public boolean longPress(float x, float y) {
// TODO Auto-generated method stub
System.out.println("long pressed");
return false;
}
@Override
public boolean fling(float velocityX, float velocityY, int button) {
// TODO Auto-generated method stub
System.out.println("gesture detector velocityX:" + velocityX + "velocityY:" + velocityY + "fling" + TAG);
return false;
}
@Override
public boolean pan(float x, float y, float deltaX, float deltaY) {
// TODO Auto-generated method stub
System.out.println("gesture detector deltaX:" + deltaX + "pan" + TAG);
camHelper.disposeTarget();
deltaX = deltaX / (viewport.getScreenWidth() / viewport.getWorldWidth());
camHelper.getCamera().position.set(stage.getCamera().position.x - deltaX, stage.getCamera().position.y, 0);
camBasePositionX += deltaX;
isPanned = true;
return true;
}
@Override
public boolean panStop(float x, float y, int pointer, int button) {
// TODO Auto-generated method stub
System.out.println("gesture detector x:" + x + " y:" + y + "panStop" + TAG);
if (currentPage == 1) {
if (camBasePositionX < -100) {
camHelper.setTarget(new Vector3(currentPage * Constants.SCREEN_PIXEL_WIDTH + Constants.SCREEN_PIXEL_WIDTH / 2, 240, 0));
currentPage++;
} else {
camHelper.setTarget(new Vector3(Constants.SCREEN_PIXEL_WIDTH / 2, 240, 0));
}
} else if (currentPage < pages) {
if (camBasePositionX < -100) {
camHelper.setTarget(new Vector3(currentPage * Constants.SCREEN_PIXEL_WIDTH + Constants.SCREEN_PIXEL_WIDTH / 2, 240, 0));
currentPage++;
} else if (camBasePositionX > 100) {
camHelper.setTarget(new Vector3((currentPage - 2) * Constants.SCREEN_PIXEL_WIDTH + Constants.SCREEN_PIXEL_WIDTH / 2, 240, 0));
currentPage--;
} else {
camHelper.setTarget(new Vector3((currentPage - 1) * Constants.SCREEN_PIXEL_WIDTH + Constants.SCREEN_PIXEL_WIDTH / 2, 240, 0));
}
} else if (currentPage == pages) {
if (camBasePositionX < -100) {
camHelper.setTarget(new Vector3(pages * Constants.SCREEN_PIXEL_WIDTH - Constants.SCREEN_PIXEL_WIDTH / 2, 240, 0));
} else {
camHelper.setTarget(new Vector3((currentPage - 2) * Constants.SCREEN_PIXEL_WIDTH + Constants.SCREEN_PIXEL_WIDTH / 2, 240, 0));
currentPage--;
}
}
camBasePositionX = 0;
return false;
}
@Override
public boolean zoom(float initialDistance, float distance) {
// TODO Auto-generated method stub
return false;
}
@Override
public boolean pinch(Vector2 initialPointer1, Vector2 initialPointer2, Vector2 pointer1, Vector2 pointer2) {
// TODO Auto-generated method stub
return false;
}
}
相关文章推荐
- 我是运营,我没有假期
- 每个 Linux 游戏玩家都绝不想要的恼人体验
- 在 Fedora 上使用 Steam play 和 Proton 来玩 Windows 游戏
- Steam 让我们在 Linux 上玩 Windows 的游戏更加容易
- 如何使用 Steam Play 在 Linux 上玩仅限 Windows 的游戏
- 新一代iPad适配应用之游戏篇
- VB实现的《QQ美女找茬游戏》作弊器实例
- C#实现洗牌游戏实例
- C#实现的算24点游戏算法实例分析
- C#实现简单的井字游戏实例
- C++编写简单的打靶游戏
- C++实现基于控制台界面的吃豆子游戏
- winform异型不规则界面设计的实现方法
- 纯javascript实现的小游戏《Flappy Pig》实例
- JavaScript实现俄罗斯方块游戏过程分析及源码分享
- JS小游戏之仙剑翻牌源码详解
- JS小游戏之宇宙战机源码详解
- Android基本游戏循环实例分析
- JavaScript游戏之优化篇
- js实现俄罗斯方块小游戏分享