hihoCoder #1199 Tower Defense Game
2015-10-27 22:06
344 查看
Description
There is a tower defense game with n levels(missions). The n levels form a tree whose root is level 1.In the i-th level, you have to spend pi units of money buying towers and after the level, you can sell the towers so that you have qi units of money back.
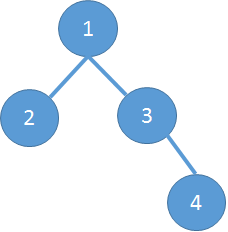
Each level is played once. You can choose any order to complete all the levels, but the order should follow the following rules:
1: A level can be played when all its ancestors are completed.
2: The levels which form a subtree of the original tree should be completed consecutively in your order.
You want to know in which order of completion you can bring the minimum money before starting the first level.
Input
The first line contains one single integers, n - the number of levels. (1<=n<=10000)The next n lines describes the levels. Each line contains 2 integers, pi and qi which are described in the statement. (0<=qi<=pi<=20000)
The next n-1 lines describes the tree. Each line contains 2 integers, ai and bi which means there is an edge between level ai and level bi.
For 30% of the data, n<=100.
For 60% of the data, n<=1000.
Output
Print one line with an single integer representing the minimum cost.Sample Hint
There are two orders of completing all levels which are: 1234 and 1342.In the order 1234, the minimum beginning money is 5.
In the order 1342, the minimum beginning money is 7.
1324 is not a valid order because level 3 and level 4 are not completed consecutively.
Sample Input
4 2 1 4 3 2 1 2 1 1 2 1 3 3 4
Sample Output
5
Solution:
#include <cstdio> #include <algorithm> #include <vector> #include <cstring> using namespace std; #define max(a,b) (a>b?a:b) #define MAXN 10010 int p[MAXN]; int q[MAXN]; vector<int> edges[MAXN]; void addEdge(int u, int v) { edges[u].push_back(v); edges[v].push_back(u); } int in[MAXN]; int out[MAXN]; int vis[MAXN] = { 0 }; struct unit { int input; int output; }; int dfs(int u, int *vis) { vis[u] = 1; if (in[u] >= 0) { return in[u]; } vector<unit> ch; for (int i = 0; i < edges[u].size(); ++i) { int v = edges[u][i]; if (!vis[v]) { dfs(v, vis); ch.push_back({ in[v], out[v] }); } } if (ch.size() == 0) { out[u] = q[u]; return in[u] = p[u]; } sort(ch.begin(), ch.end(), [](const unit &u1, const unit &u2) { return max(u1.input, u2.input + u1.input - u1.output) < max(u2.input, u1.input + u2.input - u2.output); }); int a = ch.back().input; int d = ch.back().input - ch.back().output; for (int i = ch.size() - 2; i >= 0; --i) { a = max(ch[i].input, ch[i].input - ch[i].output + a); d += ch[i].input - ch[i].output; } in[u] = max(p[u], a + p[u] - q[u]); out[u] = in[u] - d - (p[u] - q[u]); return in[u]; } int main() { int N; scanf("%d", &N); for (int i = 1; i <= N; ++i) { scanf("%d %d", p + i, q + i); } for (int i = 0; i < N - 1; ++i) { int u, v; scanf("%d %d", &u, &v); addEdge(u, v); } memset(in, -1, sizeof in); printf("%d\n", dfs(1,vis)); }
相关文章推荐
- 【JavaScript】 splice & slice
- 重要的JAVA-API StringBuffer类
- javascript方法--bind()
- jQuery之表单验证
- JQuery中$.ajax()方法参数详解
- 不解析html?
- javascript文件
- jsonp跨域
- 高性能JavaScript--数据存储(简要学习笔记二)
- 如何循序渐进有效学习 JavaScript
- ajax 的前进 后退 问题 jquery.history
- web开发学习笔记(2):HTML注释<!--..-->与JavaScript注释
- javascript Date format(js日期格式化)
- HTML5用audio标签做一个最简单的音频播放器
- 4.json解析
- JS 正则表达式验证帐号/手机号/电话号/邮箱
- 在网页加载时替换JS文件
- 迁移学习 transfer learning
- JS小函数
- A Bug's Life 【POJ--2492】【带权的并查集】