Android-----自定义圆形的头像控件
2015-10-22 14:46
429 查看
在现在的网络上圆形头像是非常常见的,圆形头像大多数使用在显示个人信息中的头像信息,今天就试试实现一个圆形的头像。
自定义一个CircleImageView,并且继承ImageView,用于显示圆形的图片。
package com.gjg.circleimageviewdemo;
import android.content.Context;
import android.content.res.TypedArray;
import android.graphics.Bitmap;
import android.graphics.BitmapShader;
import android.graphics.Canvas;
import android.graphics.Matrix;
import android.graphics.Paint;
import android.graphics.RectF;
import android.graphics.Shader;
import android.graphics.drawable.BitmapDrawable;
import android.graphics.drawable.ColorDrawable;
import android.graphics.drawable.Drawable;
import android.net.Uri;
import android.util.AttributeSet;
import android.widget.ImageView;
public class CircleImageView extends ImageView{
//设置scaletype为ScaleType.CENTER_CROP(均衡缩放)
private static final ScaleType SCALE_TYPE = ScaleType.CENTER_CROP;
private static final Bitmap.Config BITMAP_CONFIG = Bitmap.Config.ARGB_8888;
private static final int COLORDRAWABLE_DIMENSION = 1;
private final RectF mDrawableRect = new RectF();
private final RectF mBorderRect = new RectF();
private final Matrix mShaderMatrix = new Matrix();
private final Paint mBitmapPaint = new Paint();//画整个圆形的画笔
private final Paint mBorderPaint = new Paint();//画边框的画笔
private int mBorderColor;//圆边框的颜色
private int mBorderWidth;//圆边框的宽度
private Bitmap mBitmap;
private BitmapShader mBitmapShader;
private int mBitmapWidth;
private int mBitmapHeight;
private float mDrawableRadius;
private float mBorderRadius;
private boolean mReady;
private boolean mSetupPending;
public CircleImageView(Context context) {
super(context);
init();
}
public CircleImageView(Context context, AttributeSet attrs) {
this(context, attrs, 0);
init();
}
public CircleImageView(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
//从attrs.xml中获取自定义的属性
TypedArray a = context.obtainStyledAttributes(attrs, R.styleable.CircleImageView, defStyle, 0);
mBorderWidth = a.getDimensionPixelSize(R.styleable.CircleImageView_border_width, 0);
mBorderColor = a.getColor(R.styleable.CircleImageView_border_color, 0);
//回收属性,避免对下次的使用造成影响
a.recycle();
init();
}
private void init() {
super.setScaleType(SCALE_TYPE);
mReady = true;
if (mSetupPending) {
setup();
mSetupPending = false;
}
}
@Override
public ScaleType getScaleType() {
return SCALE_TYPE;
}
@Override
public void setScaleType(ScaleType scaleType) {
if (scaleType != SCALE_TYPE) {
throw new IllegalArgumentException(String.format("ScaleType %s not supported.", scaleType));
}
}
@Override
protected void onDraw(Canvas canvas) {
if (getDrawable() == null) {
return;
}
//画圆(圆形头像部分)
canvas.drawCircle(getWidth() / 2, getHeight() / 2, mDrawableRadius, mBitmapPaint);
//若设置了圆的边框宽度,将边框画出来
if (mBorderWidth != 0) {
canvas.drawCircle(getWidth() / 2, getHeight() / 2, mBorderRadius, mBorderPaint);
}
}
@Override
protected void onSizeChanged(int w, int h, int oldw, int oldh) {
super.onSizeChanged(w, h, oldw, oldh);
setup();
}
public int getBorderColor() {
return mBorderColor;
}
public void setBorderColor(int borderColor) {
if (borderColor == mBorderColor) {
return;
}
mBorderColor = borderColor;
mBorderPaint.setColor(mBorderColor);
invalidate();
}
public int getBorderWidth() {
return mBorderWidth;
}
public void setBorderWidth(int borderWidth) {
if (borderWidth == mBorderWidth) {
return;
}
mBorderWidth = borderWidth;
setup();
}
@Override
public void setImageBitmap(Bitmap bm) {
super.setImageBitmap(bm);
mBitmap = bm;
setup();
}
@Override
public void setImageDrawable(Drawable drawable) {
super.setImageDrawable(drawable);
mBitmap = getBitmapFromDrawable(drawable);
setup();
}
@Override
public void setImageResource(int resId) {
super.setImageResource(resId);
mBitmap = getBitmapFromDrawable(getDrawable());
setup();
}
@Override
public void setImageURI(Uri uri) {
super.setImageURI(uri);
mBitmap = getBitmapFromDrawable(getDrawable());
setup();
}
/**
* 获取最后的圆形图片
* @param drawable
* @return Bitmap
*/
private Bitmap getBitmapFromDrawable(Drawable drawable) {
if (drawable == null) {
return null;
}
if (drawable instanceof BitmapDrawable) {
return ((BitmapDrawable) drawable).getBitmap();
}
try {
Bitmap bitmap;
if (drawable instanceof ColorDrawable) {
bitmap = Bitmap.createBitmap(COLORDRAWABLE_DIMENSION, COLORDRAWABLE_DIMENSION, BITMAP_CONFIG);
} else {
bitmap = Bitmap.createBitmap(drawable.getIntrinsicWidth(), drawable.getIntrinsicHeight(), BITMAP_CONFIG);
}
Canvas canvas = new Canvas(bitmap);
drawable.setBounds(0, 0, canvas.getWidth(), canvas.getHeight());
drawable.draw(canvas);
return bitmap;
} catch (OutOfMemoryError e) {
return null;
}
}
private void setup() {
if (!mReady) {
mSetupPending = true;
return;
}
if (mBitmap == null) {
return;
}
mBitmapShader = new BitmapShader(mBitmap, Shader.TileMode.CLAMP, Shader.TileMode.CLAMP);
mBitmapPaint.setAntiAlias(true);
mBitmapPaint.setShader(mBitmapShader);
mBorderPaint.setStyle(Paint.Style.STROKE);
mBorderPaint.setAntiAlias(true);
mBorderPaint.setColor(mBorderColor);
mBorderPaint.setStrokeWidth(mBorderWidth);
mBitmapHeight = mBitmap.getHeight();
mBitmapWidth = mBitmap.getWidth();
mBorderRect.set(0, 0, getWidth(), getHeight());
mBorderRadius = Math.min((mBorderRect.height() - mBorderWidth) / 2, (mBorderRect.width() - mBorderWidth) / 2);
mDrawableRect.set(mBorderWidth, mBorderWidth, mBorderRect.width() - mBorderWidth, mBorderRect.height() - mBorderWidth);
mDrawableRadius = Math.min(mDrawableRect.height() / 2, mDrawableRect.width() / 2);
updateShaderMatrix();
invalidate();
}
private void updateShaderMatrix() {
float scale;
float dx = 0;
float dy = 0;
mShaderMatrix.set(null);
if (mBitmapWidth * mDrawableRect.height() > mDrawableRect.width() * mBitmapHeight) {
scale = mDrawableRect.height() / (float) mBitmapHeight;
dx = (mDrawableRect.width() - mBitmapWidth * scale) * 0.5f;
} else {
scale = mDrawableRect.width() / (float) mBitmapWidth;
dy = (mDrawableRect.height() - mBitmapHeight * scale) * 0.5f;
}
mShaderMatrix.setScale(scale, scale);
mShaderMatrix.postTranslate((int) (dx + 0.5f) + mBorderWidth, (int) (dy + 0.5f) + mBorderWidth);
mBitmapShader.setLocalMatrix(mShaderMatrix);
}
}
上面的 代码需要注意的是:
public CircleImageView(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
//从attrs.xml中获取自定义的属性
TypedArray a = context.obtainStyledAttributes(attrs, R.styleable.CircleImageView, defStyle, 0);
mBorderWidth = a.getDimensionPixelSize(R.styleable.CircleImageView_border_width, 0);
mBorderColor = a.getColor(R.styleable.CircleImageView_border_color, 0);
//回收属性,避免对下次的使用造成影响
a.recycle();
init();
}从attrs.xml文件中获得自定义的属性后,需要调用recycle()方法。
布局文件:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
xmlns:myapp="http://schemas.android.com/apk/res/com.gjg.circleimageviewdemo"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity" >
<com.gjg.circleimageviewdemo.CircleImageView
android:id="@+id/head_icon2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/head_icon1"
android:layout_centerHorizontal="true"
android:layout_marginTop="30dp"
android:background="@drawable/headicon"
myapp:border_color="#328843"
myapp:border_width="2dp" />
<com.gjg.circleimageviewdemo.CircleImageView
android:id="@+id/head_icon1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/head_icon2"
android:layout_alignParentTop="true"
android:layout_marginTop="81dp"
android:src="@drawable/headicon"
myapp:border_color="#328843"
myapp:border_width="2dp" />
<Button
android:id="@+id/button1"
android:onClick="toOtherActivity"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="16dp"
android:text="Button" />
</RelativeLayout>
注意上面的图片引用应该是: android:src="@drawable/headicon" 不要写成这种:android:background="@drawable/headicon"
后面的哪种仍然是矩形显示,不能圆形显示。
布局文件中的 xmlns:myapp="http://schemas.android.com/apk/res/com.gjg.circleimageviewdemo"引用的自定义的属性,属性文件在values/attrs.xml
attrs.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<declare-styleable name="CircleImageView">
<attr name="border_width" format="dimension" />
<attr name="border_color" format="color" />
</declare-styleable>
</resources>引用的属性的用法:
myapp:border_color="#328843"
myapp:border_width="2dp"
以myapp:开头,因为布局文件中的
xmlns:myapp="http://schemas.android.com/apk/res/com.gjg.circleimageviewdemo"也是定义成myapp,后面跟的直接是attrs中定义的name。
在attrs.xml中,其中resource是根标签,可以在里面定义若干个declare-styleable,<declare-styleable name="CircleImageView">中name定义了变量的名称,下面可以再自定义多个属性,针对<attr name="border_width" format="dimension" />来说,其属性的名称为"border_width",format指定了该属性类型为dimension,只能表示字体的大小。
format还可以指定其他的类型比如;
reference 表示引用,参考某一资源ID
string 表示字符串
color 表示颜色值
dimension 表示尺寸值
boolean 表示布尔值
integer 表示整型值
float 表示浮点值
fraction 表示百分数
enum 表示枚举值
flag 表示位运算
效果如下:
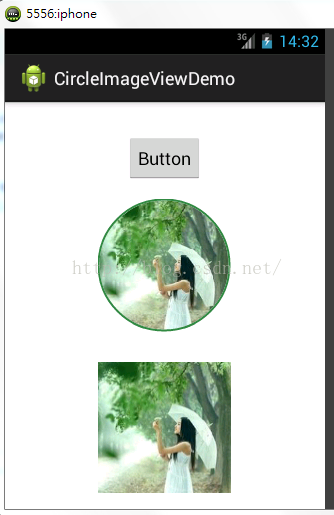
另外从网上搜了下,还可以用以下的方式直接将图裁剪成圆形图片,然后再显示。
/**
* 直接将bitmap裁剪成圆形
* @param v
*/
public void cropBitmap(View v){
Bitmap src=BitmapFactory.decodeResource(getResources(), R.drawable.headicon);
Bitmap bitmap=Bitmap.createBitmap(src);
Bitmap newbitmap=toRoundBitmap(bitmap);
ivHead.setImageBitmap(newbitmap);
}
点击“直接裁剪”按钮,效果如下:
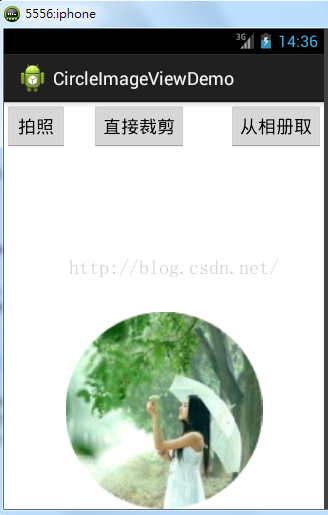
demo下载地址:http://download.csdn.net/detail/dangnianmingyue_gg/9203149
自定义一个CircleImageView,并且继承ImageView,用于显示圆形的图片。
package com.gjg.circleimageviewdemo;
import android.content.Context;
import android.content.res.TypedArray;
import android.graphics.Bitmap;
import android.graphics.BitmapShader;
import android.graphics.Canvas;
import android.graphics.Matrix;
import android.graphics.Paint;
import android.graphics.RectF;
import android.graphics.Shader;
import android.graphics.drawable.BitmapDrawable;
import android.graphics.drawable.ColorDrawable;
import android.graphics.drawable.Drawable;
import android.net.Uri;
import android.util.AttributeSet;
import android.widget.ImageView;
public class CircleImageView extends ImageView{
//设置scaletype为ScaleType.CENTER_CROP(均衡缩放)
private static final ScaleType SCALE_TYPE = ScaleType.CENTER_CROP;
private static final Bitmap.Config BITMAP_CONFIG = Bitmap.Config.ARGB_8888;
private static final int COLORDRAWABLE_DIMENSION = 1;
private final RectF mDrawableRect = new RectF();
private final RectF mBorderRect = new RectF();
private final Matrix mShaderMatrix = new Matrix();
private final Paint mBitmapPaint = new Paint();//画整个圆形的画笔
private final Paint mBorderPaint = new Paint();//画边框的画笔
private int mBorderColor;//圆边框的颜色
private int mBorderWidth;//圆边框的宽度
private Bitmap mBitmap;
private BitmapShader mBitmapShader;
private int mBitmapWidth;
private int mBitmapHeight;
private float mDrawableRadius;
private float mBorderRadius;
private boolean mReady;
private boolean mSetupPending;
public CircleImageView(Context context) {
super(context);
init();
}
public CircleImageView(Context context, AttributeSet attrs) {
this(context, attrs, 0);
init();
}
public CircleImageView(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
//从attrs.xml中获取自定义的属性
TypedArray a = context.obtainStyledAttributes(attrs, R.styleable.CircleImageView, defStyle, 0);
mBorderWidth = a.getDimensionPixelSize(R.styleable.CircleImageView_border_width, 0);
mBorderColor = a.getColor(R.styleable.CircleImageView_border_color, 0);
//回收属性,避免对下次的使用造成影响
a.recycle();
init();
}
private void init() {
super.setScaleType(SCALE_TYPE);
mReady = true;
if (mSetupPending) {
setup();
mSetupPending = false;
}
}
@Override
public ScaleType getScaleType() {
return SCALE_TYPE;
}
@Override
public void setScaleType(ScaleType scaleType) {
if (scaleType != SCALE_TYPE) {
throw new IllegalArgumentException(String.format("ScaleType %s not supported.", scaleType));
}
}
@Override
protected void onDraw(Canvas canvas) {
if (getDrawable() == null) {
return;
}
//画圆(圆形头像部分)
canvas.drawCircle(getWidth() / 2, getHeight() / 2, mDrawableRadius, mBitmapPaint);
//若设置了圆的边框宽度,将边框画出来
if (mBorderWidth != 0) {
canvas.drawCircle(getWidth() / 2, getHeight() / 2, mBorderRadius, mBorderPaint);
}
}
@Override
protected void onSizeChanged(int w, int h, int oldw, int oldh) {
super.onSizeChanged(w, h, oldw, oldh);
setup();
}
public int getBorderColor() {
return mBorderColor;
}
public void setBorderColor(int borderColor) {
if (borderColor == mBorderColor) {
return;
}
mBorderColor = borderColor;
mBorderPaint.setColor(mBorderColor);
invalidate();
}
public int getBorderWidth() {
return mBorderWidth;
}
public void setBorderWidth(int borderWidth) {
if (borderWidth == mBorderWidth) {
return;
}
mBorderWidth = borderWidth;
setup();
}
@Override
public void setImageBitmap(Bitmap bm) {
super.setImageBitmap(bm);
mBitmap = bm;
setup();
}
@Override
public void setImageDrawable(Drawable drawable) {
super.setImageDrawable(drawable);
mBitmap = getBitmapFromDrawable(drawable);
setup();
}
@Override
public void setImageResource(int resId) {
super.setImageResource(resId);
mBitmap = getBitmapFromDrawable(getDrawable());
setup();
}
@Override
public void setImageURI(Uri uri) {
super.setImageURI(uri);
mBitmap = getBitmapFromDrawable(getDrawable());
setup();
}
/**
* 获取最后的圆形图片
* @param drawable
* @return Bitmap
*/
private Bitmap getBitmapFromDrawable(Drawable drawable) {
if (drawable == null) {
return null;
}
if (drawable instanceof BitmapDrawable) {
return ((BitmapDrawable) drawable).getBitmap();
}
try {
Bitmap bitmap;
if (drawable instanceof ColorDrawable) {
bitmap = Bitmap.createBitmap(COLORDRAWABLE_DIMENSION, COLORDRAWABLE_DIMENSION, BITMAP_CONFIG);
} else {
bitmap = Bitmap.createBitmap(drawable.getIntrinsicWidth(), drawable.getIntrinsicHeight(), BITMAP_CONFIG);
}
Canvas canvas = new Canvas(bitmap);
drawable.setBounds(0, 0, canvas.getWidth(), canvas.getHeight());
drawable.draw(canvas);
return bitmap;
} catch (OutOfMemoryError e) {
return null;
}
}
private void setup() {
if (!mReady) {
mSetupPending = true;
return;
}
if (mBitmap == null) {
return;
}
mBitmapShader = new BitmapShader(mBitmap, Shader.TileMode.CLAMP, Shader.TileMode.CLAMP);
mBitmapPaint.setAntiAlias(true);
mBitmapPaint.setShader(mBitmapShader);
mBorderPaint.setStyle(Paint.Style.STROKE);
mBorderPaint.setAntiAlias(true);
mBorderPaint.setColor(mBorderColor);
mBorderPaint.setStrokeWidth(mBorderWidth);
mBitmapHeight = mBitmap.getHeight();
mBitmapWidth = mBitmap.getWidth();
mBorderRect.set(0, 0, getWidth(), getHeight());
mBorderRadius = Math.min((mBorderRect.height() - mBorderWidth) / 2, (mBorderRect.width() - mBorderWidth) / 2);
mDrawableRect.set(mBorderWidth, mBorderWidth, mBorderRect.width() - mBorderWidth, mBorderRect.height() - mBorderWidth);
mDrawableRadius = Math.min(mDrawableRect.height() / 2, mDrawableRect.width() / 2);
updateShaderMatrix();
invalidate();
}
private void updateShaderMatrix() {
float scale;
float dx = 0;
float dy = 0;
mShaderMatrix.set(null);
if (mBitmapWidth * mDrawableRect.height() > mDrawableRect.width() * mBitmapHeight) {
scale = mDrawableRect.height() / (float) mBitmapHeight;
dx = (mDrawableRect.width() - mBitmapWidth * scale) * 0.5f;
} else {
scale = mDrawableRect.width() / (float) mBitmapWidth;
dy = (mDrawableRect.height() - mBitmapHeight * scale) * 0.5f;
}
mShaderMatrix.setScale(scale, scale);
mShaderMatrix.postTranslate((int) (dx + 0.5f) + mBorderWidth, (int) (dy + 0.5f) + mBorderWidth);
mBitmapShader.setLocalMatrix(mShaderMatrix);
}
}
上面的 代码需要注意的是:
public CircleImageView(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
//从attrs.xml中获取自定义的属性
TypedArray a = context.obtainStyledAttributes(attrs, R.styleable.CircleImageView, defStyle, 0);
mBorderWidth = a.getDimensionPixelSize(R.styleable.CircleImageView_border_width, 0);
mBorderColor = a.getColor(R.styleable.CircleImageView_border_color, 0);
//回收属性,避免对下次的使用造成影响
a.recycle();
init();
}从attrs.xml文件中获得自定义的属性后,需要调用recycle()方法。
布局文件:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
xmlns:myapp="http://schemas.android.com/apk/res/com.gjg.circleimageviewdemo"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity" >
<com.gjg.circleimageviewdemo.CircleImageView
android:id="@+id/head_icon2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/head_icon1"
android:layout_centerHorizontal="true"
android:layout_marginTop="30dp"
android:background="@drawable/headicon"
myapp:border_color="#328843"
myapp:border_width="2dp" />
<com.gjg.circleimageviewdemo.CircleImageView
android:id="@+id/head_icon1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/head_icon2"
android:layout_alignParentTop="true"
android:layout_marginTop="81dp"
android:src="@drawable/headicon"
myapp:border_color="#328843"
myapp:border_width="2dp" />
<Button
android:id="@+id/button1"
android:onClick="toOtherActivity"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="16dp"
android:text="Button" />
</RelativeLayout>
注意上面的图片引用应该是: android:src="@drawable/headicon" 不要写成这种:android:background="@drawable/headicon"
后面的哪种仍然是矩形显示,不能圆形显示。
布局文件中的 xmlns:myapp="http://schemas.android.com/apk/res/com.gjg.circleimageviewdemo"引用的自定义的属性,属性文件在values/attrs.xml
attrs.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<declare-styleable name="CircleImageView">
<attr name="border_width" format="dimension" />
<attr name="border_color" format="color" />
</declare-styleable>
</resources>引用的属性的用法:
myapp:border_color="#328843"
myapp:border_width="2dp"
以myapp:开头,因为布局文件中的
xmlns:myapp="http://schemas.android.com/apk/res/com.gjg.circleimageviewdemo"也是定义成myapp,后面跟的直接是attrs中定义的name。
在attrs.xml中,其中resource是根标签,可以在里面定义若干个declare-styleable,<declare-styleable name="CircleImageView">中name定义了变量的名称,下面可以再自定义多个属性,针对<attr name="border_width" format="dimension" />来说,其属性的名称为"border_width",format指定了该属性类型为dimension,只能表示字体的大小。
format还可以指定其他的类型比如;
reference 表示引用,参考某一资源ID
string 表示字符串
color 表示颜色值
dimension 表示尺寸值
boolean 表示布尔值
integer 表示整型值
float 表示浮点值
fraction 表示百分数
enum 表示枚举值
flag 表示位运算
效果如下:
另外从网上搜了下,还可以用以下的方式直接将图裁剪成圆形图片,然后再显示。
/**
* 直接将bitmap裁剪成圆形
* @param v
*/
public void cropBitmap(View v){
Bitmap src=BitmapFactory.decodeResource(getResources(), R.drawable.headicon);
Bitmap bitmap=Bitmap.createBitmap(src);
Bitmap newbitmap=toRoundBitmap(bitmap);
ivHead.setImageBitmap(newbitmap);
}
/** * 转换图片成圆形 * * @param bitmap 传入Bitmap对象 * @return */ public Bitmap toRoundBitmap(Bitmap bitmap) { int width = bitmap.getWidth(); int height = bitmap.getHeight(); float roundPx; float left, top, right, bottom, dst_left, dst_top, dst_right, dst_bottom; if (width <= height) { roundPx = width / 2; top = 0; bottom = width; left = 0; right = width; height = width; dst_left = 0; dst_top = 0; dst_right = width; dst_bottom = width; } else { roundPx = height / 2; float clip = (width - height) / 2; left = clip; right = width - clip; top = 0; bottom = height; width = height; dst_left = 0; dst_top = 0; dst_right = height; dst_bottom = height; } Bitmap output = Bitmap.createBitmap(width, height, Config.ARGB_8888); Canvas canvas = new Canvas(output); final int color = 0xff424242; final Paint paint = new Paint(); final Rect src = new Rect((int) left, (int) top, (int) right, (int) bottom); final Rect dst = new Rect((int) dst_left, (int) dst_top, (int) dst_right, (int) dst_bottom); final RectF rectF = new RectF(dst); paint.setAntiAlias(true); canvas.drawARGB(0, 0, 0, 0); paint.setColor(color); canvas.drawRoundRect(rectF, roundPx, roundPx, paint); paint.setXfermode(new PorterDuffXfermode(Mode.SRC_IN)); canvas.drawBitmap(bitmap, src, dst, paint); return output; }
点击“直接裁剪”按钮,效果如下:
demo下载地址:http://download.csdn.net/detail/dangnianmingyue_gg/9203149
相关文章推荐
- 使用ImageMagick进行图片缩放、合成与裁剪(js+python)
- Android开发从相机或相册获取图片裁剪
- PHP 使用 Imagick 裁切/生成缩略图/添加水印自动检测和处理 GIF
- jQuery插件jcrop+Fileapi完美实现图片上传+裁剪+预览的代码分享
- Android实现GridView中ImageView动态变换的方法
- Android控件之ImageView用法实例分析
- Android UI之ImageView实现图片旋转和缩放
- android ImageView 的几点经验总结
- Android控件系列之ImageView使用方法
- Android中通过反射实现圆角ImageView代码实例
- Android中ImageView使用网络图片资源的方法
- Android实现本地上传图片并设置为圆形头像
- ASP.NET简单好用功能齐全图片上传工具类(水印、缩略图、裁剪等)
- 基于JQuery实现的图片自动进行缩放和裁剪处理
- MacOS 安装 PHP的图片裁剪扩展Tclip
- ImageView的属性android:scaleType的作用分析
- Android优秀开源库收集
- android 实现圆形imageView,Circle imageView.
- vtk中实现裁剪
- cropper图片裁剪上传