swift版的CircleView
2015-10-07 21:18
441 查看
swift版的CircleView
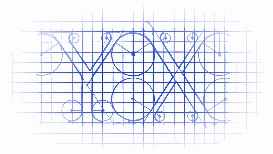
效果图

源码
说明
参数查看并没有OC那么直白.
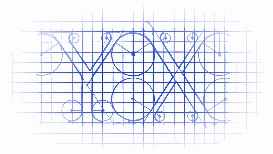
效果图

源码
// // CircleView.swift // CircleView // // Created by YouXianMing on 15/10/7. // Copyright © 2015年 YouXianMing. All rights reserved. // import UIKit class CircleView: UIView { // MARK: - 变量 var lineWidth : CGFloat = 1 var lineColor : UIColor = UIColor.blackColor() var clockWise : Bool = false var startAngle : CGFloat = 0 var duration : NSTimeInterval = 0.2 private var circleLayer : CAShapeLayer! // MARK: - Public Method /** 构建view,让参数生效 */ func buildView() { let size = bounds.size let point = CGPoint(x: size.height / 2, y: size.width / 2) let radius = size.width / 2 - lineWidth / 2 var tmpStartAngle : CGFloat = 0 var tmpEndAngle : CGFloat = 0 if (clockWise == true) { tmpStartAngle = -radian(Double(180 - startAngle)); tmpEndAngle = radian(Double(180 + self.startAngle)); } else { tmpStartAngle = radian(Double(180 - self.startAngle)); tmpEndAngle = -radian(Double(180 + self.startAngle)); } let circlePath = UIBezierPath(arcCenter: point, radius: radius, startAngle: tmpStartAngle, endAngle: tmpEndAngle, clockwise: clockWise) circleLayer.path = circlePath.CGPath circleLayer.strokeColor = lineColor.CGColor circleLayer.fillColor = UIColor.clearColor().CGColor circleLayer.lineWidth = lineWidth circleLayer.strokeEnd = 0 } /** 绘制圆形百分比 - parameter percent: 百分比 - parameter animated: 是否开启动画 */ func changeToPercent(var percent : CGFloat, animated : Bool) { if (percent <= 0) { percent = 0; } else if (percent >= 1) { percent = 1; } if (animated) { let basicAnimation : CABasicAnimation! = CABasicAnimation() basicAnimation.keyPath = "strokeEnd" basicAnimation.duration = (duration <= 0 ? 0.2 : duration) basicAnimation.fromValue = circleLayer.strokeEnd basicAnimation.toValue = percent circleLayer.strokeEnd = percent circleLayer.addAnimation(basicAnimation, forKey: nil) } else { CATransaction.setDisableActions(true) circleLayer.strokeEnd = percent CATransaction.setDisableActions(false) } } // MARK: - System Method override init(frame: CGRect) { super.init(frame: frame) createCircleLayer() } required init?(coder aDecoder: NSCoder) { fatalError("init(coder:) has not been implemented") } // MARK: - Private Method private func radian(degrees : Double) -> CGFloat { return CGFloat((M_PI * degrees) / 180) } private func createCircleLayer() { circleLayer = CAShapeLayer() circleLayer.frame = self.bounds self.layer.addSublayer(circleLayer) } }
// // ViewController.swift // CircleView // // Created by YouXianMing on 15/10/7. // Copyright © 2015年 YouXianMing. All rights reserved. // import UIKit class ViewController: UIViewController { var eventTimer : NSTimer! var circleView : CircleView! override func viewDidLoad() { super.viewDidLoad() eventTimer = NSTimer.scheduledTimerWithTimeInterval(1, target: self, selector: "timerEvent", userInfo: nil, repeats: true) circleView = CircleView(frame: CGRect(x: 0, y: 0, width: 100, height: 100)) circleView.lineWidth = 1 circleView.lineColor = UIColor.blackColor() circleView.duration = 0.25 circleView.clockWise = true circleView.startAngle = 90 circleView.center = view.center circleView.buildView() view.addSubview(circleView) } func timerEvent() { circleView.changeToPercent(CGFloat(arc4random() % 101) / 100, animated: true) } }
说明
参数查看并没有OC那么直白.
相关文章推荐
- 12.Swift JSON 和 PList
- 用drawAtPoint绘制文字(swift)
- swift开发笔记10 - 通过drawRect自定义控件外观
- Swift学习笔试19——扩展(Extension)
- Swift中的可选类型Optional与if let 语法
- 11.Swift XML解析
- swift开发的那些应用
- 10.Swift 数据存取
- Swift中Singleton的实现
- 基于iOS开发对Swift构造方法的感悟
- Swift中的构造方法
- Swift详解之NSPredicate
- Swift学习笔记20——协议(Protocols)
- 【Swift】学习笔记(一)——熟知 基础数据类型,编码风格,元组,主张
- Swift与OC代码转换实例
- swift中的as?和as!
- Swift - 表格图片加载优化(拖动表格时不加载,停止时只加载当前页图片)
- Swift - 给图片添加滤镜效果(棕褐色老照片滤镜,黑白滤镜)
- Swift - .plist文件数据的读取和存储
- Swift - 使用HTML5进行iOS开发(将HTML5打包成iOS应用)