Android动画浅谈之Animation动画
2015-09-29 09:47
459 查看
这几天学习使用了Android的动画相关的知识,现在总结一下学到的关于Animation的知识,后面还会介绍一下更加好用的Android动画实现方法
Android中Animation动画一共有四种动画,分别为rotation(旋转)、translation(偏移)、alpha(透明度)、scale(缩放)主要就是这四种方法,我们可以通过xml文件来实现这几种动画,也可以通过代码来实现动画,下面会对两种实现方法做一个详细的介绍:
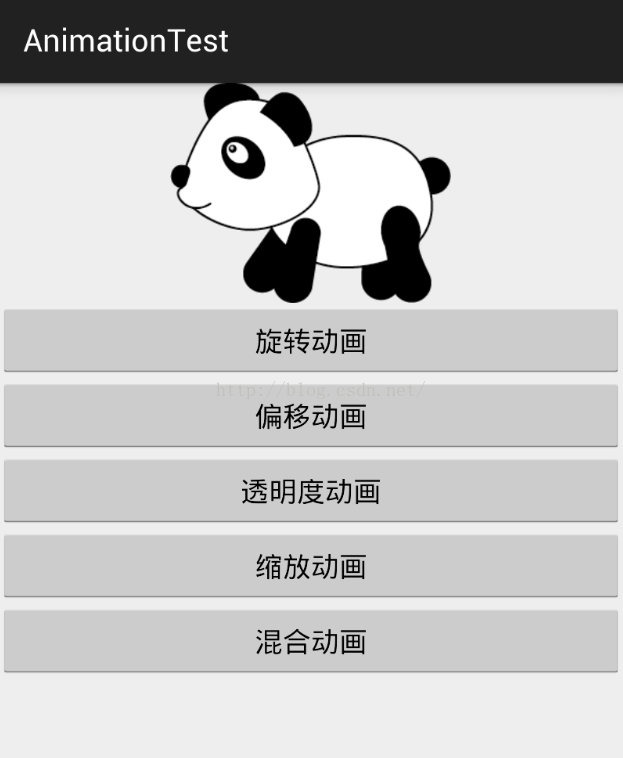
后面几种方法和此方法一样就不在粘贴上面的代码
其中的anim动画文件为anim文件夹下的myanimation.xml
点击打开链接
Android中Animation动画一共有四种动画,分别为rotation(旋转)、translation(偏移)、alpha(透明度)、scale(缩放)主要就是这四种方法,我们可以通过xml文件来实现这几种动画,也可以通过代码来实现动画,下面会对两种实现方法做一个详细的介绍:
1、旋转动画
a、通过代码实现
// 四个参数代表,旋转的起始角度、终止角度、旋转x点、旋转y点 RotateAnimation rotateAnimation = new RotateAnimation(0f, 360f, Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF, 0.5f); rotateAnimation.setDuration(2000);// 旋转时间为2秒 img.startAnimation(rotateAnimation);// 启动动画
b、通过xml文件来实现
Animation rotateAnimation2=(Animation) AnimationUtils.loadAnimation(this, R.anim.myanimation); img.startAnimation(rotateAnimation2);
后面几种方法和此方法一样就不在粘贴上面的代码
其中的anim动画文件为anim文件夹下的myanimation.xml
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android" > <rotate android:duration="2000" android:fromDegrees="0" android:pivotX="50%" android:pivotY="50%" android:toDegrees="360" /> </set>
2、偏移动画
a、通过代码实现
//四个参数代表,偏移的x起始点、x终止点、偏移的y起始点、y终止点 TranslateAnimation translateAnimation=new TranslateAnimation(0f, 200f, 0f, 200f); translateAnimation.setDuration(2000); img.startAnimation(translateAnimation);
b、通过xml文件来实现
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android" > <translate android:duration="2000" android:fromXDelta="0" android:toXDelta="200" android:fromYDelta="0" android:toYDelta="200" /> </set>
3、透明度动画
a、通过代码实现
// 两个参数代表,透明度从0升到1 AlphaAnimation alphaAnimation = new AlphaAnimation(0f, 1f); alphaAnimation.setDuration(2000); img.startAnimation(alphaAnimation);
b、通过xml文件来实现
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android" > <alpha android:duration="2000" android:fromAlpha="0" android:toAlpha="1" /> </set>
4、缩放动画
a、通过代码实现
// 六个参数代表,缩放的x、y的大小,x、y的起始和终止点 ScaleAnimation scaleAnimation = new ScaleAnimation(0f, 1f, 0f, 1f,Animation.RELATIVE_TO_SELF, 0.5f,Animation.RELATIVE_TO_SELF, 0.5f); scaleAnimation.setDuration(2000); img.startAnimation(scaleAnimation);
b、通过xml文件来实现
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android" > <scale android:duration="2000" android:fromXScale="0" android:fromYScale="0" android:pivotX="50%" android:pivotY="50%" android:toXScale="200" android:toYScale="200" /> </set>
5、四种动画同时进行
a、代码实现
RotateAnimation rotateAnimation1 = new RotateAnimation(0f, 360f, Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF, 0.5f); TranslateAnimation translateAnimation1 = new TranslateAnimation(0f, 200f, 0f, 200f); AlphaAnimation alphaAnimation1 = new AlphaAnimation(0f, 1f); ScaleAnimation scaleAnimation1 = new ScaleAnimation(0f, 1f, 0f, 1f, Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF, 0.5f); AnimationSet set=new AnimationSet(true); set.addAnimation(rotateAnimation1); set.addAnimation(translateAnimation1); set.addAnimation(alphaAnimation1); set.addAnimation(scaleAnimation1); set.setDuration(2000); img.startAnimation(set);
b、xml文件实现
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android" > <!-- 旋转 --> <rotate android:duration="2000" android:fromDegrees="0" android:pivotX="50%" android:pivotY="50%" android:toDegrees="360" /> <!-- 偏移 --> <translate android:duration="2000" android:fromXDelta="0" android:fromYDelta="0" android:toXDelta="200" android:toYDelta="200" /> <!-- 透明度 --> <alpha android:duration="2000" android:fromAlpha="0" android:toAlpha="1" /> <!-- 缩放 --> <scale android:duration="2000" android:fromXScale="0" android:fromYScale="0" android:pivotX="50%" android:pivotY="50%" android:toXScale="1" android:toYScale="1" /> </set>
最后附上项目的代码(粘贴就能用)
package com.example.animationtest;
import android.os.Bundle;
import android.support.v7.app.ActionBarActivity;
import android.view.View;
import android.view.animation.AlphaAnimation;
import android.view.animation.Animation;
import android.view.animation.AnimationSet;
import android.view.animation.AnimationUtils;
import android.view.animation.RotateAnimation;
import android.view.animation.ScaleAnimation;
import android.view.animation.TranslateAnimation;
import android.widget.ImageView;
public class MainActivity extends ActionBarActivity {
private ImageView img;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
img = (ImageView) findViewById(R.id.imageView);
}
public void myclick(View view) {
switch (view.getId()) {
case R.id.rotation_bt:
/*
* 通过代码实现
*/
// 四个参数代表,旋转的起始角度、终止角度、旋转x点、旋转y点 RotateAnimation rotateAnimation = new RotateAnimation(0f, 360f, Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF, 0.5f); rotateAnimation.setDuration(2000);// 旋转时间为2秒 img.startAnimation(rotateAnimation);// 启动动画
/*
* 通过xml文件来实现动画效果
*/
// Animation rotateAnimation2 = (Animation) AnimationUtils
// .loadAnimation(this, R.anim.myanimation);
// img.startAnimation(rotateAnimation2);
break;
case R.id.translation_bt:
/*
* 通过代码实现
*/
// 四个参数代表,偏移的x起始点、x终止点、偏移的y起始点、y终止点
TranslateAnimation translateAnimation = new TranslateAnimation(0f,
200f, 0f, 200f);
translateAnimation.setDuration(2000);
img.startAnimation(translateAnimation);
break;
case R.id.alpha_bt:
/*
* 通过代码实现
*/
// 两个参数代表,透明度从0升到1 AlphaAnimation alphaAnimation = new AlphaAnimation(0f, 1f); alphaAnimation.setDuration(2000); img.startAnimation(alphaAnimation);
break;
case R.id.scale_bt:
/*
* 通过代码实现
*/
// 六个参数代表,缩放的x、y的大小,x、y的起始和终止点
ScaleAnimation scaleAnimation = new ScaleAnimation(0f, 1f, 0f, 1f,
Animation.RELATIVE_TO_SELF, 0.5f,
Animation.RELATIVE_TO_SELF, 0.5f);
scaleAnimation.setDuration(2000);
img.startAnimation(scaleAnimation);
break;
case R.id.together_bt:
RotateAnimation rotateAnimation1 = new RotateAnimation(0f, 360f,
Animation.RELATIVE_TO_SELF, 0.5f,
Animation.RELATIVE_TO_SELF, 0.5f);
TranslateAnimation translateAnimation1 = new TranslateAnimation(0f,
200f, 0f, 200f);
AlphaAnimation alphaAnimation1 = new AlphaAnimation(0f, 1f);
ScaleAnimation scaleAnimation1 = new ScaleAnimation(0f, 1f, 0f, 1f,
Animation.RELATIVE_TO_SELF, 0.5f,
Animation.RELATIVE_TO_SELF, 0.5f);
AnimationSet set = new AnimationSet(true);
set.addAnimation(rotateAnimation1);
set.addAnimation(translateAnimation1);
set.addAnimation(alphaAnimation1);
set.addAnimation(scaleAnimation1);
set.setDuration(2000);
img.startAnimation(set);
break;
}
}
}
布局文件代码
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/LinearLayout1" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center_horizontal" android:orientation="vertical" > <ImageView android:id="@+id/imageView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/run1" /> <Button android:id="@+id/rotation_bt" android:onClick="myclick" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="旋转动画" /> <Button android:id="@+id/translation_bt" android:onClick="myclick" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="偏移动画" /> <Button android:id="@+id/alpha_bt" android:onClick="myclick" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="透明度动画" /> <Button android:id="@+id/scale_bt" android:onClick="myclick" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="缩放动画" /> <Button android:id="@+id/together_bt" android:onClick="myclick" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="混合动画" /> </LinearLayout>
还有anim文件下的myanimation.xml文件代码
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android" > <!-- 旋转 --> <rotate android:duration="2000" android:fromDegrees="0" android:pivotX="50%" android:pivotY="50%" android:toDegrees="360" /> <!-- 偏移 --> <translate android:duration="2000" android:fromXDelta="0" android:fromYDelta="0" android:toXDelta="200" android:toYDelta="200" /> <!-- 透明度 --> <alpha android:duration="2000" android:fromAlpha="0" android:toAlpha="1" /> <!-- 缩放 --> <scale android:duration="2000" android:fromXScale="0" android:fromYScale="0" android:pivotX="50%" android:pivotY="50%" android:toXScale="1" android:toYScale="1" /> </set>
源码:
http://download.csdn.net/detail/jl_stone/9518252点击打开链接
相关文章推荐
- 使用C++实现JNI接口需要注意的事项
- Android IPC进程间通讯机制
- Android Manifest 用法
- [转载]Activity中ConfigChanges属性的用法
- Android之获取手机上的图片和视频缩略图thumbnails
- Android之使用Http协议实现文件上传功能
- Android学习笔记(二九):嵌入浏览器
- android string.xml文件中的整型和string型代替
- i-jetty环境搭配与编译
- android之定时器AlarmManager
- android wifi 无线调试
- Android Native 绘图方法
- Android java 与 javascript互访(相互调用)的方法例子
- android 代码实现控件之间的间距
- android FragmentPagerAdapter的“标准”配置
- Android"解决"onTouch和onClick的冲突问题
- android:installLocation简析
- android searchView的关闭事件
- SourceProvider.getJniDirectories