Unity3D之Legacy动画系统学习笔记
2015-09-24 17:14
736 查看
Unity3D的Mecanim动画系统是非常强大的,而且作为Unity推荐的动画系统,其未来会完全代替老的一套动画系统,即Legacy动画系统。目前的情况是Mecanim与Legacy两套动画系统同时共存,但是并不是说Legacy动画系统就没有任何价值了,作为Unity4.0以前使用的动画系统,我认为还是很有必要去了解和学习的,所以就有了这篇笔记。
我们可以使用Unity自带的资源来学习老版本的动画系统,新建Unity3D项目,选择菜单“Assets”->“Import Package”->“Character Controller”,导入的资源里的那个小人就是使用Legacy动画系统的模型,我们的学习可以基于他来进行。
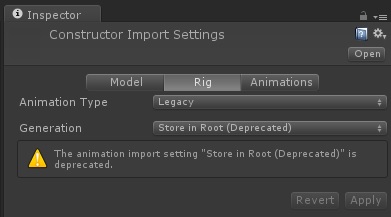
在骨骼这一项中,我们发现动画类型的设置就是Legacy,说明这个模型使用的动画类型为老版本的动画系统。
我们再看看动画页:

动画页中,我们可以对动画剪辑进行编辑。
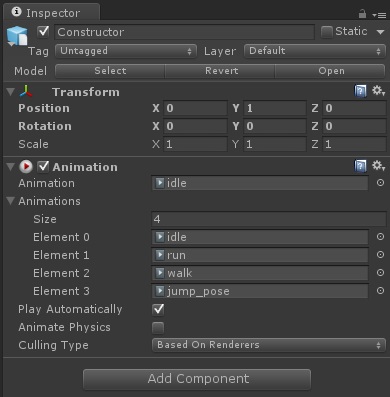
Animation组件的设置还是比较简单的:
Animation:当前播放的动画。
Animations:所有可以播放的动画。
Play Automatically:是否自动播放。
Animate Physics:动画是否和物理世界进行交互。
Culling Type:动画在不可见时是否还继续播放,优化选项默认即可。
点击播放按钮就可以看见动画正常播放了。
下面我们来看看如何使用脚本控制动画的播放,我们将下面的脚本绑定到人物身上即可。
运行程序,会看见两排按钮,其中第一排按钮使用Play方法来切换动画,而第二排按钮则使用CrossFade来播放动画。
Play:直接切换动画,如果人物之前处于倾斜跑步状态,则会立即变成站立状态,表现上比较不真实,特别是当两个动画姿势差别较大时。
CrossFade:通过动画融合来切换动画,第二个参数可以指定融合的时间,如果人物之前处于倾斜跑步状态,则会在指定的融合时间内逐渐变成站立状态,表现上接近真实的人物动作切换效果。
但是当使用CrossFade播放跳跃动画时会出现问题,主要问题是跳跃动画不是循环播放且其持续时间小于动画融合的时间,我们修改为下面的脚本指定融合时间短一点就可以正常进行跳跃的融合播放了:


虽然有一个Take 001的动画,但是实际上我们并不使用该动画,而是使用下面仅保存了动画的FBX文件:
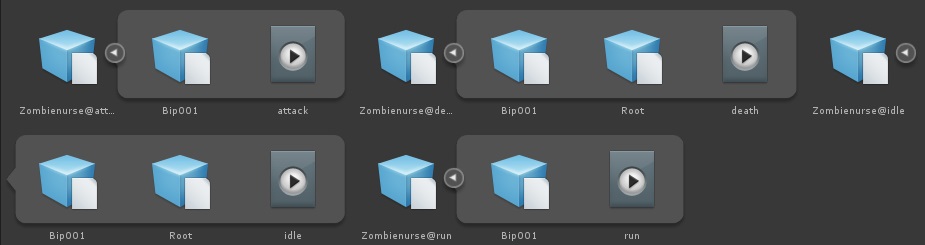
Legacy动画系统
http://docs.unity3d.com/Manual/Animations.html我们可以使用Unity自带的资源来学习老版本的动画系统,新建Unity3D项目,选择菜单“Assets”->“Import Package”->“Character Controller”,导入的资源里的那个小人就是使用Legacy动画系统的模型,我们的学习可以基于他来进行。
模型文件
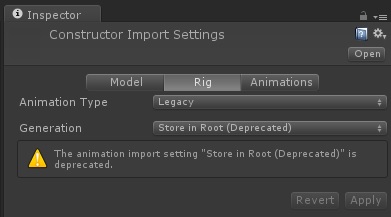
在骨骼这一项中,我们发现动画类型的设置就是Legacy,说明这个模型使用的动画类型为老版本的动画系统。
我们再看看动画页:

动画页中,我们可以对动画剪辑进行编辑。
控制动画
我们直接将FBX文件拖入场景,Unity会自动帮我们添加Transform和Animation两个组件(注意Mecanim动画系统使用的是Animator组件,Legacy动画系统使用的是Animation组件)。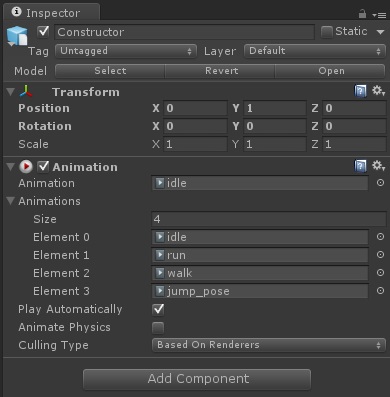
Animation组件的设置还是比较简单的:
Animation:当前播放的动画。
Animations:所有可以播放的动画。
Play Automatically:是否自动播放。
Animate Physics:动画是否和物理世界进行交互。
Culling Type:动画在不可见时是否还继续播放,优化选项默认即可。
点击播放按钮就可以看见动画正常播放了。
脚本控制
http://docs.unity3d.com/ScriptReference/Animation.html下面我们来看看如何使用脚本控制动画的播放,我们将下面的脚本绑定到人物身上即可。
using UnityEngine; using System.Collections; public class AnimationScript : MonoBehaviour { private Animation _animation; void Start() { _animation = this.animation; } void OnGUI() { //直接播放动画 if(GUI.Button(new Rect(0, 0, 100, 30), "idle")) { _animation.Play("idle"); } if(GUI.Button(new Rect(100, 0, 100, 30), "walk")) { _animation.Play("walk"); } if(GUI.Button(new Rect(200, 0, 100, 30), "run")) { _animation.Play("run"); } if(GUI.Button(new Rect(300, 0, 100, 30), "jump_pose")) { _animation.Play("jump_pose"); } //使用融合来播放动画 if(GUI.Button(new Rect(0, 30, 100, 30), "idle")) { _animation.CrossFade("idle"); } if(GUI.Button(new Rect(100, 30, 100, 30), "walk")) { _animation.CrossFade("walk"); } if(GUI.Button(new Rect(200, 30, 100, 30), "run")) { _animation.CrossFade("run"); } if(GUI.Button(new Rect(300, 30, 100, 30), "jump_pose")) { _animation.CrossFade("jump_pose"); } } }
运行程序,会看见两排按钮,其中第一排按钮使用Play方法来切换动画,而第二排按钮则使用CrossFade来播放动画。
Play与CrossFade的区别
以从跑步切换到站立动画为例来看:Play:直接切换动画,如果人物之前处于倾斜跑步状态,则会立即变成站立状态,表现上比较不真实,特别是当两个动画姿势差别较大时。
CrossFade:通过动画融合来切换动画,第二个参数可以指定融合的时间,如果人物之前处于倾斜跑步状态,则会在指定的融合时间内逐渐变成站立状态,表现上接近真实的人物动作切换效果。
但是当使用CrossFade播放跳跃动画时会出现问题,主要问题是跳跃动画不是循环播放且其持续时间小于动画融合的时间,我们修改为下面的脚本指定融合时间短一点就可以正常进行跳跃的融合播放了:
_animation.CrossFade("jump_pose", 0.1f);
PlayQueued
该方法可以指定当当前的动画播放完毕后接下来播放的动画,如下:_animation.PlayQueued("run", QueueMode.CompleteOthers, PlayMode.StopSameLayer);
文件格式和资源加载
我们的模型使用通用的FBX格式,那么动画文件的储存一般有两种情况,一是所有的动画和模型都一起存放到一个文件中,还有一种情况是模型单独一个文件而动画单独一个文件。模型动画都存放在一个文件中的情况
类似于Unity提供的Character Controller中的资源就是这样的结构,一个FBX文件保存了模型、骨骼和动画:
Resources加载
我们直接加载该资源就可以直接使用,将下面的脚本绑定到摄像机即可,脚本如下:using UnityEngine; using System.Collections; public class AllInOneResourcesLoad : MonoBehaviour { private Animation _animation; void Start() { GameObject go = Resources.Load<GameObject>("Standard Assets/Character Controllers/Sources/PrototypeCharacter/Constructor"); GameObject man = Instantiate(go) as GameObject; _animation = man.animation; } void OnGUI() { //直接播放动画 if(GUI.Button(new Rect(0, 0, 100, 30), "idle")) { _animation.Play("idle"); } if(GUI.Button(new Rect(100, 0, 100, 30), "walk")) { _animation.Play("walk"); } if(GUI.Button(new Rect(200, 0, 100, 30), "run")) { _animation.Play("run"); } if(GUI.Button(new Rect(300, 0, 100, 30), "jump_pose")) { _animation.Play("jump_pose"); } //使用融合来播放动画 if(GUI.Button(new Rect(0, 30, 100, 30), "idle")) { _animation.CrossFade("idle"); } if(GUI.Button(new Rect(100, 30, 100, 30), "walk")) { _animation.CrossFade("walk"); } if(GUI.Button(new Rect(200, 30, 100, 30), "run")) { _animation.CrossFade("run"); } if(GUI.Button(new Rect(300, 30, 100, 30), "jump_pose")) { _animation.CrossFade("jump_pose", 0.1f); } } }
AssetBundle加载
打包
使用下面的脚本打包:using UnityEditor; using UnityEngine; public class CreateAllInOneAB { [MenuItem("Tool/CreateAllInOneAB")] private static void Create() { BuildPipeline.BuildAssetBundle(null, new[] { AssetDatabase.LoadAssetAtPath("Assets/Resources/Standard Assets/Character Controllers/Sources/PrototypeCharacter/Constructor.FBX", typeof(GameObject)) }, Application.streamingAssetsPath + "/AllInOne.assetbundle", BuildAssetBundleOptions.CollectDependencies | BuildAssetBundleOptions.CompleteAssets | BuildAssetBundleOptions.UncompressedAssetBundle, BuildTarget.StandaloneWindows64); } }
加载
将下面的脚本绑定到摄像机即可:using UnityEngine; using System.Collections; public class AllInOneAssetBundleLoad : MonoBehaviour { private Animation _animation; void Start() { AssetBundle assetBundle = AssetBundle.CreateFromFile(Application.streamingAssetsPath + "/AllInOne.assetbundle"); GameObject go = assetBundle.Load("Constructor", typeof(GameObject)) as GameObject; GameObject man = Instantiate(go) as GameObject; _animation = man.animation; } void OnGUI() { //直接播放动画 if(GUI.Button(new Rect(0, 0, 100, 30), "idle")) { _animation.Play("idle"); } if(GUI.Button(new Rect(100, 0, 100, 30), "walk")) { _animation.Play("walk"); } if(GUI.Button(new Rect(200, 0, 100, 30), "run")) { _animation.Play("run"); } if(GUI.Button(new Rect(300, 0, 100, 30), "jump_pose")) { _animation.Play("jump_pose"); } //使用融合来播放动画 if(GUI.Button(new Rect(0, 30, 100, 30), "idle")) { _animation.CrossFade("idle"); } if(GUI.Button(new Rect(100, 30, 100, 30), "walk")) { _animation.CrossFade("walk"); } if(GUI.Button(new Rect(200, 30, 100, 30), "run")) { _animation.CrossFade("run"); } if(GUI.Button(new Rect(300, 30, 100, 30), "jump_pose")) { _animation.CrossFade("jump_pose", 0.1f); } } }
模型动画分开存放的情况
还有一种情况是模型和动画是分为多个FBX文件存放的,比如下面是模型文件:
虽然有一个Take 001的动画,但是实际上我们并不使用该动画,而是使用下面仅保存了动画的FBX文件:
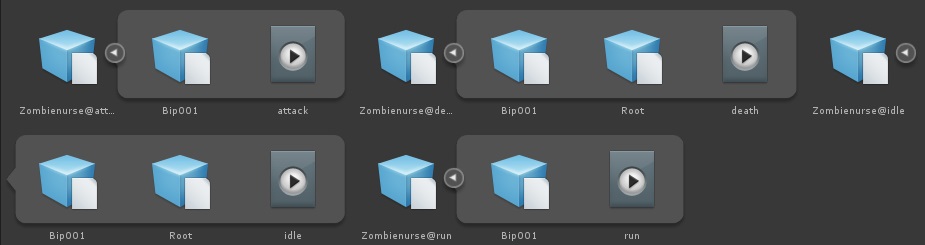
Resources加载
首先我们要清楚的是,无论是保存了模型还是保存了动画的FBX文件在Unity看来都是同样的一种类型,即添加了Animation组件的GameObject,下面我们看看如何在Resources中加载并显示这个角色,代码如下,挂载到主摄像机即可:using UnityEngine; using System.Collections; public class ResourcesLoad : MonoBehaviour { private Animation _animation; void Start() { GameObject go = Resources.Load<GameObject>("ZombieNurse/Zombienurse_Rig"); GameObject man = Instantiate(go) as GameObject; _animation = man.animation; //添加动画剪辑 _animation.AddClip(LoadAnimationClip("ZombieNurse/Animation/Zombienurse@attack"), "attack"); _animation.AddClip(LoadAnimationClip("ZombieNurse/Animation/Zombienurse@death"), "death"); _animation.AddClip(LoadAnimationClip("ZombieNurse/Animation/Zombienurse@idle"), "idle"); _animation.AddClip(LoadAnimationClip("ZombieNurse/Animation/Zombienurse@run"), "run"); _animation.Play("idle"); } private AnimationClip LoadAnimationClip(string path) { GameObject go = Resources.Load<GameObject>(path); return go.animation.clip; } void OnGUI() { if(GUI.Button(new Rect(0, 0, 100, 30), "idle")) { _animation.CrossFade("idle"); } if(GUI.Button(new Rect(100, 0, 100, 30), "run")) { _animation.CrossFade("run"); } if(GUI.Button(new Rect(200, 0, 100, 30), "attack")) { _animation.CrossFade("attack"); } if(GUI.Button(new Rect(300, 0, 100, 30), "death")) { _animation.CrossFade("death"); } } }
AssetBundle加载
打包
使用下面的脚本打包:using UnityEngine; using UnityEditor; public class CreateAB : MonoBehaviour { [MenuItem("Tool/CreateAB")] private static void Create() { BuildPipeline.BuildAssetBundle(null, new[] { AssetDatabase.LoadAssetAtPath("Assets/Resources/ZombieNurse/Zombienurse_Rig.FBX", typeof(GameObject)), AssetDatabase.LoadAssetAtPath("Assets/Resources/ZombieNurse/Animation/Zombienurse@attack.FBX", typeof(GameObject)), AssetDatabase.LoadAssetAtPath("Assets/Resources/ZombieNurse/Animation/Zombienurse@death.FBX", typeof(GameObject)), AssetDatabase.LoadAssetAtPath("Assets/Resources/ZombieNurse/Animation/Zombienurse@idle.FBX", typeof(GameObject)), AssetDatabase.LoadAssetAtPath("Assets/Resources/ZombieNurse/Animation/Zombienurse@run.FBX", typeof(GameObject)) }, Application.streamingAssetsPath + "/AB.assetbundle", BuildAssetBundleOptions.CollectDependencies | BuildAssetBundleOptions.CompleteAssets | BuildAssetBundleOptions.UncompressedAssetBundle, BuildTarget.StandaloneWindows64); } }
加载
将下面的脚本绑定到摄像机即可:using UnityEngine; using System.Collections; public class AssetBundleLoad : MonoBehaviour { private Animation _animation; void Start() { AssetBundle assetBundle = AssetBundle.CreateFromFile(Application.streamingAssetsPath + "/AB.assetbundle"); GameObject go = assetBundle.Load("Zombienurse_Rig", typeof(GameObject)) as GameObject; GameObject man = Instantiate(go) as GameObject; _animation = man.animation; //添加动画剪辑 _animation.AddClip(LoadAnimationClip(assetBundle, "Zombienurse@attack"), "attack"); _animation.AddClip(LoadAnimationClip(assetBundle, "Zombienurse@death"), "death"); _animation.AddClip(LoadAnimationClip(assetBundle, "Zombienurse@idle"), "idle"); _animation.AddClip(LoadAnimationClip(assetBundle, "Zombienurse@run"), "run"); _animation.Play("idle"); } private AnimationClip LoadAnimationClip(AssetBundle assetBundle, string path) { GameObject go = assetBundle.Load(path, typeof(GameObject)) as GameObject; return go.animation.clip; } void OnGUI() { if(GUI.Button(new Rect(0, 0, 100, 30), "idle")) { _animation.CrossFade("idle"); } if(GUI.Button(new Rect(100, 0, 100, 30), "run")) { _animation.CrossFade("run"); } if(GUI.Button(new Rect(200, 0, 100, 30), "attack")) { _animation.CrossFade("attack"); } if(GUI.Button(new Rect(300, 0, 100, 30), "death")) { _animation.CrossFade("death"); } } }
相关文章推荐
- Unity3D之Mecanim动画系统学习笔记(十一):高级功能应用
- Unity3d-内建编辑器样式
- unity3D第一天
- Unity3D之Mecanim动画系统学习笔记(十):Mecanim动画的资源加载相关
- UnityShader实例16:屏幕特效之径向模糊(Radial Blur)
- Unity的旋转问题
- Unity 加密解密那些事(转载)
- Unity3d开发(三)GameObject 作函数参数
- Unity游戏编程之游戏对象管理
- 辛巴学院-Unity-剑英陪你零基础学c#系列(三)计算与类型
- unity C#更改系统默认鼠标指针
- unity C#更改系统默认鼠标指针
- Unity - Camera
- 跳转场景的3种方式
- unity_NGUI动态字体制作与字体清晰的几点要素
- unity3d android打包中的class , .layout not found 等的问题研究
- Unity3D之Mecanim动画系统学习笔记(九):Blend Tree(混合树)
- unity3d 5.0以上打包安卓apk部分手机黑屏问题,尤其魅族手机
- Unity中的Material和Shader<坑待填>
- Unity中的Material和Shader<坑待填>