Spring AOP + AspectJ annotation example
2015-09-17 12:52
609 查看
In this tutorial, we show you how to integrate AspectJ annotation with Spring AOP framework. In simple, Spring AOP + AspectJ allow you to intercept method easily.
Common AspectJ annotations :
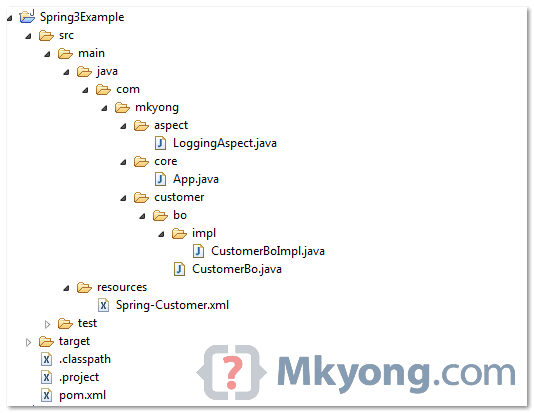
AspectJ supported since Spring 2.0
This example is using Spring 3, but the AspectJ features are supported since Spring 2.0.
File :
File :
4. AspectJ
In below example, the
10228
e,
Note
AspectJ “pointcuts” is used to declare which method is going to intercept.
Run it
Output
5. AspectJ
In below example, the
File :
Run it
Output
6. AspectJ
In below example, the
To intercept returned value, the value of the “
File :
Run it
Output
7. AspectJ
In below example, the
File :
Run it
Output
8. AspectJ
In below example, the
File :
Run it
Output
Common AspectJ annotations :
@Before– Run before the method execution
@After– Run after the method returned a result
@AfterReturning– Run after the method returned a result, intercept the returned result as well.
@AfterThrowing– Run after the method throws an exception
@Around– Run around the method execution, combine all three advices above.
1. Directory Structure
See directory structure of this example.2. Project Dependencies
To enable AspectJ, you needaspectjrt.jar,
aspectjweaver.jarand
spring-aop.jar. See following Maven
pom.xmlfile.
AspectJ supported since Spring 2.0
This example is using Spring 3, but the AspectJ features are supported since Spring 2.0.
File : pom.xml
<project ...> <properties> <spring.version>3.0.5.RELEASE</spring.version> </properties> <dependencies> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${spring.version}</version> </dependency> <!-- Spring AOP + AspectJ --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-aop</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjrt</artifactId> <version>1.6.11</version> </dependency> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjweaver</artifactId> <version>1.6.11</version> </dependency> </dependencies> </project>
3. Spring Beans
Normal bean, with few methods, later intercept it via AspectJ annotation.package com.mkyong.customer.bo; public interface CustomerBo { void addCustomer(); String addCustomerReturnValue(); void addCustomerThrowException() throws Exception; void addCustomerAround(String name); }
package com.mkyong.customer.bo.impl; import com.mkyong.customer.bo.CustomerBo; public class CustomerBoImpl implements CustomerBo { public void addCustomer(){ System.out.println("addCustomer() is running "); } public String addCustomerReturnValue(){ System.out.println("addCustomerReturnValue() is running "); return "abc"; } public void addCustomerThrowException() throws Exception { System.out.println("addCustomerThrowException() is running "); throw new Exception("Generic Error"); } public void addCustomerAround(String name){ System.out.println("addCustomerAround() is running, args : " + name); } }
4. Enable AspectJ
In Spring configuration file, put “<aop:aspectj-autoproxy />“, and define your Aspect (interceptor) and normal bean.
File :Spring-Customer.xml
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.0.xsd "> <aop:aspectj-autoproxy /> <bean id="customerBo" class="com.mkyong.customer.bo.impl.CustomerBoImpl" /> <!-- Aspect --> <bean id="logAspect" class="com.mkyong.aspect.LoggingAspect" /> </beans>
4. AspectJ @Before
In below example, the logBefore()method will be executed before the execution of
CustomerBointerfac
10228
e,
addCustomer()method.
Note
AspectJ “pointcuts” is used to declare which method is going to intercept.
File : LoggingAspect.java
package com.mkyong.aspect; import org.aspectj.lang.JoinPoint; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Before; @Aspect public class LoggingAspect { @Before("execution(* com.mkyong.customer.bo.CustomerBo.addCustomer(..))") public void logBefore(JoinPoint joinPoint) { System.out.println("logBefore() is running!"); System.out.println("hijacked : " + joinPoint.getSignature().getName()); System.out.println("******"); } }
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo"); customer.addCustomer();
Output
logBefore() is running! hijacked : addCustomer ****** addCustomer() is running
5. AspectJ @After
In below example, the logAfter()method will be executed after the execution of
CustomerBointerface,
addCustomer()method.
File : LoggingAspect.java
package com.mkyong.aspect; import org.aspectj.lang.JoinPoint; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.After; @Aspect public class LoggingAspect { @After("execution(* com.mkyong.customer.bo.CustomerBo.addCustomer(..))") public void logAfter(JoinPoint joinPoint) { System.out.println("logAfter() is running!"); System.out.println("hijacked : " + joinPoint.getSignature().getName()); System.out.println("******"); } }
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo"); customer.addCustomer();
Output
addCustomer() is running logAfter() is running! hijacked : addCustomer ******
6. AspectJ @AfterReturning
In below example, the logAfterReturning()method will be executed after the execution of
CustomerBointerface,
addCustomerReturnValue()method. In addition, you can intercept the returned value with the “
returning” attribute.
To intercept returned value, the value of the “
returning” attribute (result) need to be same with the method parameter (
result).
File : LoggingAspect.java
package com.mkyong.aspect; import org.aspectj.lang.JoinPoint; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.AfterReturning; @Aspect public class LoggingAspect { @AfterReturning( pointcut = "execution(* com.mkyong.customer.bo.CustomerBo.addCustomerReturnValue(..))", returning= "result") public void logAfterReturning(JoinPoint joinPoint, Object result) { System.out.println("logAfterReturning() is running!"); System.out.println("hijacked : " + joinPoint.getSignature().getName()); System.out.println("Method returned value is : " + result); System.out.println("******"); } }
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo"); customer.addCustomerReturnValue();
Output
addCustomerReturnValue() is running logAfterReturning() is running! hijacked : addCustomerReturnValue Method returned value is : abc ******
7. AspectJ @AfterReturning
In below example, the logAfterThrowing()method will be executed if the customerBo interface,
addCustomerThrowException()method is throwing an exception.
File : LoggingAspect.java
package com.mkyong.aspect; import org.aspectj.lang.JoinPoint; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.AfterThrowing; @Aspect public class LoggingAspect { @AfterThrowing( pointcut = "execution(* com.mkyong.customer.bo.CustomerBo.addCustomerThrowException(..))", throwing= "error") public void logAfterThrowing(JoinPoint joinPoint, Throwable error) { System.out.println("logAfterThrowing() is running!"); System.out.println("hijacked : " + joinPoint.getSignature().getName()); System.out.println("Exception : " + error); System.out.println("******"); } }
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo"); customer.addCustomerThrowException();
Output
addCustomerThrowException() is running logAfterThrowing() is running! hijacked : addCustomerThrowException Exception : java.lang.Exception: Generic Error ****** Exception in thread "main" java.lang.Exception: Generic Error //...
8. AspectJ @Around
In below example, the logAround()method will be executed before the
customerBointerface,
addCustomerAround()method, and you have to define the “
joinPoint.proceed();” to control when should the interceptor return the control to the original
addCustomerAround()method.
File : LoggingAspect.java
package com.mkyong.aspect; import org.aspectj.lang.ProceedingJoinPoint; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Around; @Aspect public class LoggingAspect { @Around("execution(* com.mkyong.customer.bo.CustomerBo.addCustomerAround(..))") public void logAround(ProceedingJoinPoint joinPoint) throws Throwable { System.out.println("logAround() is running!"); System.out.println("hijacked method : " + joinPoint.getSignature().getName()); System.out.println("hijacked arguments : " + Arrays.toString(joinPoint.getArgs())); System.out.println("Around before is running!"); joinPoint.proceed(); //continue on the intercepted method System.out.println("Around after is running!"); System.out.println("******"); } }
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo"); customer.addCustomerAround("mkyong");
Output
logAround() is running! hijacked method : addCustomerAround hijacked arguments : [mkyong] Around before is running! addCustomerAround() is running, args : mkyong Around after is running! ******
Conclusion
It’s always recommended to apply the least power AsjectJ annotation.相关文章推荐
- 一个jar包里的网站
- 一个jar包里的网站之文件上传
- 一个jar包里的网站之返回对媒体类型
- yui3的AOP(面向切面编程)和OOP(面向对象编程)
- JavaScript AOP编程实例
- 使用AOP改善javascript代码
- 初识SmartJS - AOP三剑客
- spring+html5实现安全传输随机数字密码键盘
- Spring中属性注入详解
- struts2 spring整合fieldError问题
- spring的jdbctemplate的crud的基类dao
- java使用动态代理来实现AOP(日志记录)的实例代码
- 读取spring配置文件的方法(spring读取资源文件)
- java实现简单美女拼图游戏
- java基本教程之线程休眠 java多线程教程
- JSP开发中在spring mvc项目中实现登录账号单浏览器登录
- JavaScript之AOP编程实例
- Javascript aop(面向切面编程)之around(环绕)分析
- php笔记之:AOP的应用
- 基于Spring框架的Shiro配置方法