The DOM in JavaScript
2015-09-09 15:42
633 查看
DOM : Document Object Model
D is for document :
The DOM cant work without a document . When you create a web page and load it in a web browser, the DOM comes to life. It takes the document that you have written and turns it into
an object .
O is for Objects : Just as we see before.
M is for Model :
The M in DOM stands for Model, but it could just as easily stand for Map.
The DOM represents the web page thats currently loaded in the browser window. The browser
provides a map (or a model) of the page. You can use JavaScript to read this map.
The most important convention used by the DOM is the representation of a document as a tree.
More specifically, the document is represented as a family tree.

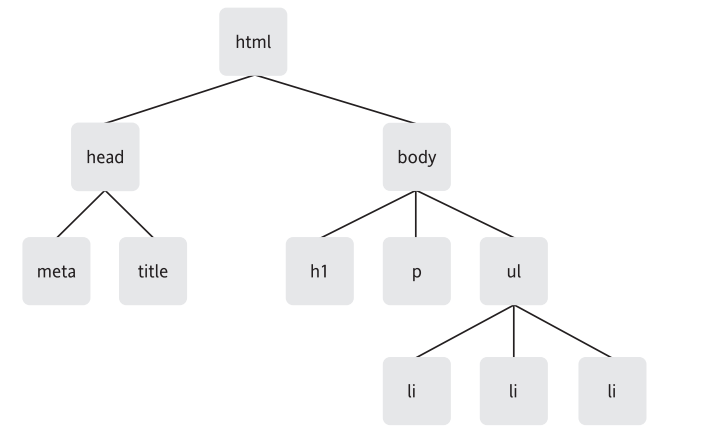
However, instead of using the term family tree, its more accurate to call a document a node tree.
Nodes :
A document is a collection of nodes, with nodes as the branches and leaves on the document tree.
There are a number of defferent types of nodes. Lets take a quick look at three of them :
element nodes, text nodes, attribute nodes.
Element nodes :
such as <body> <p> <ul> , Elemnets are the basic building blocks of documents on the Web, and
its the arrangement of these elements in a document that gives the document its structure.
Text nodes:
if a document consisted purely of empty elements, it would have a structure, but the document
itself wouldnt contain much content. On the web, most content is provided by text.
such as <p>Dont forget to buy this stuff</p> , "Dont forget to buy this stuff" is a text node.
In XHTML, text nodes are always enclosed within element nodes.
Attribute nodes:
Attributes are used to give more specific information about an element.
<p title = "a gentle reminder"> Dont forget to buy this stuff. </p>
In DOM, title = "a gentle reminder" is an attribute node.

Because attributes are always placed within opening tags, attribute nodes are always contained
within element nodes. Not all elements contain attribute, but all attributes are contained by elements.
In fact, each element in a document is an object. Using the DOM, you can "get" at all of these
elements .
Getting Elements :
Three DOM methods allow you to access element nodes by ID, tag name, and class name.
getElementById() :
usage : document.getElementById(id) :
this method will return a specific object in the document model.
you can use the typeof to test this : eg :
alert( typeof document.getElementById("purchases") ) ;
getElementsByTagName() :
usage : element.getElementByTagName( tag );
Even if there is only one element with the specified tag name, getElementByTagName still returns
an array. The length of the array will be simply 1.
you can also use a wildcard(通配符) with getElementByTagName, which means you can make an
array with every single element. eg :
alert ( document.getElementsByTagName("*").length ) ;
you can also combine getElementsByTagName with getElementById. eg :
var shopping = document.getElementById("purchases") ;
var items = shopping.getElementsByTagName("*") ;
alert( items.length );
getElementsByClassName()
It also behaves in the same way as getElementsByTagName by returning an array of elements with a common class name.The getElementsByClassName method is quite useful, but it’s supported by only modern browsers. To make up for this lack of support, DOM scripters have needed to roll their own getElementsByClassName function using existing DOM methods, sort of like a rite of passage.
Before you may write like this :
var shopping = document.getElementById("purchases");
var sales = shopping.getElementsByClassName("sale");
With this function, you can write the code like this :
var shopping = document.getElementById("purchases");
var sales = getElementsByClassName(shopping, "sale");
Here's a quick summary of what you've seen so far :
A document is a tree of nodes.
There are different types of nodes : elements, attributes, text, and so on
you can go straight to a specific element node using getElementById.
you can go directly to a collection of element nodes using getElementsByTagName or getElementsByClassName
Every one of these nodes is an object.
Getting and Setting Attributes :
getAttribute()
usage : object.getAttribute( attribute ) ;
Unlike the other methods you've seen before, you cant use getAttribute on the document object.
It can be used on only an element node object.
SetAttribute()
usage : object.setAttribute(attribute, value);
D is for document :
The DOM cant work without a document . When you create a web page and load it in a web browser, the DOM comes to life. It takes the document that you have written and turns it into
an object .
O is for Objects : Just as we see before.
M is for Model :
The M in DOM stands for Model, but it could just as easily stand for Map.
The DOM represents the web page thats currently loaded in the browser window. The browser
provides a map (or a model) of the page. You can use JavaScript to read this map.
The most important convention used by the DOM is the representation of a document as a tree.
More specifically, the document is represented as a family tree.

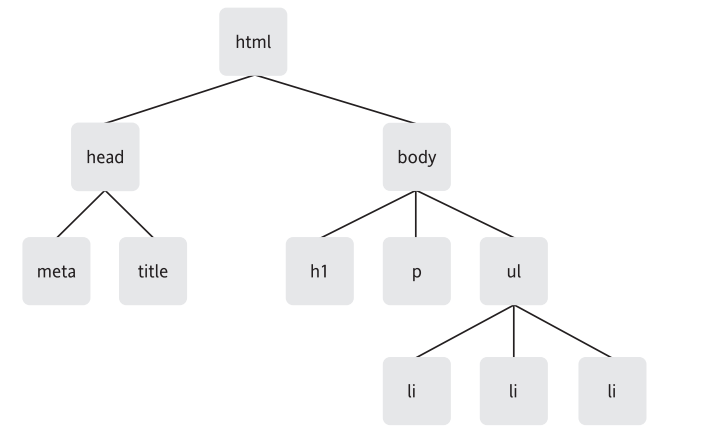
However, instead of using the term family tree, its more accurate to call a document a node tree.
Nodes :
A document is a collection of nodes, with nodes as the branches and leaves on the document tree.
There are a number of defferent types of nodes. Lets take a quick look at three of them :
element nodes, text nodes, attribute nodes.
Element nodes :
such as <body> <p> <ul> , Elemnets are the basic building blocks of documents on the Web, and
its the arrangement of these elements in a document that gives the document its structure.
Text nodes:
if a document consisted purely of empty elements, it would have a structure, but the document
itself wouldnt contain much content. On the web, most content is provided by text.
such as <p>Dont forget to buy this stuff</p> , "Dont forget to buy this stuff" is a text node.
In XHTML, text nodes are always enclosed within element nodes.
Attribute nodes:
Attributes are used to give more specific information about an element.
<p title = "a gentle reminder"> Dont forget to buy this stuff. </p>
In DOM, title = "a gentle reminder" is an attribute node.

Because attributes are always placed within opening tags, attribute nodes are always contained
within element nodes. Not all elements contain attribute, but all attributes are contained by elements.
In fact, each element in a document is an object. Using the DOM, you can "get" at all of these
elements .
Getting Elements :
Three DOM methods allow you to access element nodes by ID, tag name, and class name.
getElementById() :
usage : document.getElementById(id) :
this method will return a specific object in the document model.
you can use the typeof to test this : eg :
alert( typeof document.getElementById("purchases") ) ;
getElementsByTagName() :
usage : element.getElementByTagName( tag );
Even if there is only one element with the specified tag name, getElementByTagName still returns
an array. The length of the array will be simply 1.
you can also use a wildcard(通配符) with getElementByTagName, which means you can make an
array with every single element. eg :
alert ( document.getElementsByTagName("*").length ) ;
you can also combine getElementsByTagName with getElementById. eg :
var shopping = document.getElementById("purchases") ;
var items = shopping.getElementsByTagName("*") ;
alert( items.length );
getElementsByClassName()
It also behaves in the same way as getElementsByTagName by returning an array of elements with a common class name.The getElementsByClassName method is quite useful, but it’s supported by only modern browsers. To make up for this lack of support, DOM scripters have needed to roll their own getElementsByClassName function using existing DOM methods, sort of like a rite of passage.
function getElementsByClassName(node, classname) { if (node.getElementsByClassName) { // Use the existing method return node.getElementsByClassName(classname); } else { var results = new Array(); var elems = node.getElementsByTagName("*"); for (var i=0; i<elems.length; i++) { if (elems[i].className.indexOf(classname) != -1) { results[results.length] = elems[i]; } } return results; } }
Before you may write like this :
var shopping = document.getElementById("purchases");
var sales = shopping.getElementsByClassName("sale");
With this function, you can write the code like this :
var shopping = document.getElementById("purchases");
var sales = getElementsByClassName(shopping, "sale");
Here's a quick summary of what you've seen so far :
A document is a tree of nodes.
There are different types of nodes : elements, attributes, text, and so on
you can go straight to a specific element node using getElementById.
you can go directly to a collection of element nodes using getElementsByTagName or getElementsByClassName
Every one of these nodes is an object.
Getting and Setting Attributes :
getAttribute()
usage : object.getAttribute( attribute ) ;
Unlike the other methods you've seen before, you cant use getAttribute on the document object.
It can be used on only an element node object.
SetAttribute()
usage : object.setAttribute(attribute, value);
相关文章推荐
- JavaScript 弹出窗体点击按钮返回选择数据的实现
- 防止form重复提交
- JavaScript 弹出窗体点击按钮返回选择数据的实现
- js页面加载顺序
- JS,JQ点击事件
- js获取某个ID的class名称
- javascript小插件-多选框全选
- Json转译
- simplejson json
- prefixfree.js和依托应用之Animatable
- JS中的prototype
- EXTjs4 动态设置 button pressed 状态切换
- javascript中的split()和join()方法
- js禁止复制页面文字
- 利用js代码模拟浏览器后退、前进、刷新
- JSP---自定义标签库(TagLib)
- JavaScript并非“按值传递”
- 理解JavaScript中的原型
- javascript新手入门必读书籍推荐
- JS中的prototype