使用uploadify实现文件上传
2015-09-06 00:00
441 查看
摘要: 使用uploadify上传文件
使用uploadify实现文件上传
导入需要的js,css等文件
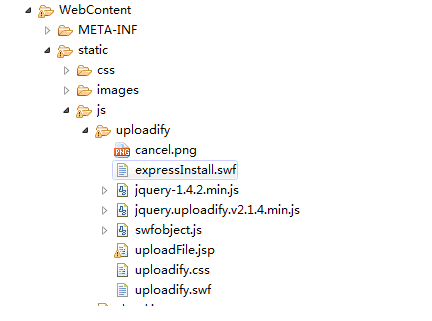
添加uploadify.jsp文件
//代码
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<base href="<%=basePath%>">
<link href="<%=basePath%>static/js/uploadify/uploadify.css" rel="stylesheet" type="text/css">
<script type="text/javascript" src="<%=basePath%>static/js/uploadify/jquery-1.4.2.min.js"></script>
<script type="text/javascript" src="<%=basePath%>static/js/uploadify/swfobject.js"></script>
<script type="text/javascript" src="<%=basePath%>static/js/uploadify/jquery.uploadify.v2.1.4.min.js"></script>
</head>
<body>
<div id="fileQueue"></div>
<label>图片: </label>
<div class="showImg">
<img class="showImgs" width="100" height="100" alt="" src="easy/js/uploadify/default_image.gif">
</div>
<input type="file" name="uploadify" id="uploadify" style="width:200px;"/>
<p><a href="javascript: jQuery('#uploadify').uploadifyUpload()">开始上传</a></p>
</body>
<script type="text/javascript">
//官方网址:http://www.uploadify.com/
$(document).ready(function(){
$("#uploadify").uploadify({
'uploader' : "<%=basePath%>static/js/uploadify/uploadify.swf",
'script' : "<%=basePath%>uploadFile",//此处是servlet请求路径;如果请求使用uploadFile.jsp,此处就是uploadFile.jsp的路径"<%=basePath%>static/js/uploadify/uploadFile.jsp"
'cancelImg' : "<%=basePath%>static/js/uploadify/cancel.png",
'folder' : "static/uploads",//上传文件存放的路径,请保持与uploadFile.jsp中PATH的值相同
'queueId' : "fileQueue",
'queueSizeLimit' : 10,//限制上传文件的数量
'fileExt' : "*.rar,*.zip",
//'fileDesc' : "RAR *.rar",//限制文件类型
'auto' : false,
'multi' : true,//是否允许多文件上传
'simUploadLimit': 2,//同时运行上传的进程数量
'buttonText': " select files",
'onComplete': function(event, ID, fileObj, response, data) {
//response返回值:重命名后的文件名称,文件保存路径
var resultArray = response.split(",");
var realName = resultArray[0];//图片现在的名称,前面加上时间的图片名称,带扩展名
realName = $.trim(realName);
var p = "<%=basePath%>static/uploads/"+realName;
$(".showImgs").attr("src",p);
}
});
});
</script>
</html>
上传文件:添加servlet方法(或者使用uploadFile.jsp)
(1)//servlet方法代码
@RequestMapping("uploadFile")
@ResponseBody
public String uploadifyStart(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException{
String savePath = request.getSession().getServletContext().getRealPath("/");
String PATH = "/static/uploads/";
savePath = savePath + PATH;
File f1 = new File(savePath);
//System.out.println(savePath);
if (!f1.exists()) {
f1.mkdirs();
}
DiskFileItemFactory fac = new DiskFileItemFactory();
ServletFileUpload upload = new ServletFileUpload(fac);
upload.setHeaderEncoding("utf-8");
List fileList = null;
try {
fileList = upload.parseRequest(request);
} catch (FileUploadException ex) {
return "";
}
Iterator<FileItem> it = fileList.iterator();
String name = "";
String extName = "";
while (it.hasNext()) {
FileItem item = it.next();
if (!item.isFormField()) {
name = item.getName();
long size = item.getSize();
String type = item.getContentType();
//System.out.println(size + " " + type);
if (name == null || name.trim().equals("")) {
continue;
}
// 扩展名格式:
if (name.lastIndexOf(".") >= 0) {
extName = name.substring(name.lastIndexOf("."));
}
File file = null;
do {
// 生成文件名:
name = UUID.randomUUID().toString();
file = new File(savePath + name + extName);
} while (file.exists());
File saveFile = new File(savePath + name + extName);
try {
item.write(saveFile);
} catch (Exception e) {
e.printStackTrace();
}
}
}
return name + extName;
}
(2)uploadFile.jsp实现上传
//uploadFile.jsp代码
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ page import="java.io.*, java.util.*, org.apache.commons.fileupload.*" %>
<%@ page import="org.apache.commons.fileupload.disk.*, org.apache.commons.fileupload.servlet.*" %>
<%!
String PATH = "/static/uploads/";
public void upload(HttpServletRequest request, HttpServletResponse response)throws ServletException, IOException {
String savePath = this.getServletConfig().getServletContext().getRealPath("");
savePath = savePath + PATH;
File f1 = new File(savePath);
System.out.println(savePath);
if (!f1.exists()) {
f1.mkdirs();
}
DiskFileItemFactory fac = new DiskFileItemFactory();
ServletFileUpload upload = new ServletFileUpload(fac);
upload.setHeaderEncoding("utf-8");
List fileList = null;
try {
fileList = upload.parseRequest(request);
} catch (FileUploadException ex) {
return;
}
Iterator<FileItem> it = fileList.iterator();
String name = "";
String extName = "";
while (it.hasNext()) {
FileItem item = it.next();
if (!item.isFormField()) {
name = item.getName();
long size = item.getSize();
String type = item.getContentType();
System.out.println(size + " " + type);
if (name == null || name.trim().equals("")) {
continue;
}
// 扩展名格式:
if (name.lastIndexOf(".") >= 0) {
extName = name.substring(name.lastIndexOf("."));
}
File file = null;
do {
// 生成文件名:
name = UUID.randomUUID().toString();
file = new File(savePath + name + extName);
} while (file.exists());
File saveFile = new File(savePath + name + extName);
try {
item.write(saveFile);
} catch (Exception e) {
e.printStackTrace();
}
}
}
response.getWriter().print(name + extName);
}
%>
<%
upload(request, response);
%>
4.uploadify文件下载地址:http://download.csdn.net/detail/lychaox/3677400
使用uploadify实现文件上传
导入需要的js,css等文件
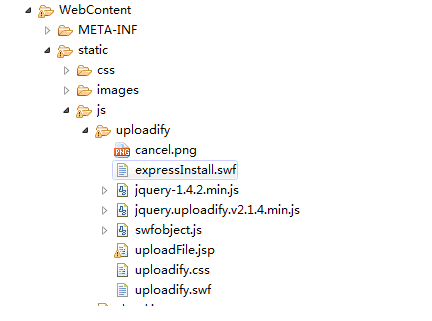
添加uploadify.jsp文件
//代码
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<base href="<%=basePath%>">
<link href="<%=basePath%>static/js/uploadify/uploadify.css" rel="stylesheet" type="text/css">
<script type="text/javascript" src="<%=basePath%>static/js/uploadify/jquery-1.4.2.min.js"></script>
<script type="text/javascript" src="<%=basePath%>static/js/uploadify/swfobject.js"></script>
<script type="text/javascript" src="<%=basePath%>static/js/uploadify/jquery.uploadify.v2.1.4.min.js"></script>
</head>
<body>
<div id="fileQueue"></div>
<label>图片: </label>
<div class="showImg">
<img class="showImgs" width="100" height="100" alt="" src="easy/js/uploadify/default_image.gif">
</div>
<input type="file" name="uploadify" id="uploadify" style="width:200px;"/>
<p><a href="javascript: jQuery('#uploadify').uploadifyUpload()">开始上传</a></p>
</body>
<script type="text/javascript">
//官方网址:http://www.uploadify.com/
$(document).ready(function(){
$("#uploadify").uploadify({
'uploader' : "<%=basePath%>static/js/uploadify/uploadify.swf",
'script' : "<%=basePath%>uploadFile",//此处是servlet请求路径;如果请求使用uploadFile.jsp,此处就是uploadFile.jsp的路径"<%=basePath%>static/js/uploadify/uploadFile.jsp"
'cancelImg' : "<%=basePath%>static/js/uploadify/cancel.png",
'folder' : "static/uploads",//上传文件存放的路径,请保持与uploadFile.jsp中PATH的值相同
'queueId' : "fileQueue",
'queueSizeLimit' : 10,//限制上传文件的数量
'fileExt' : "*.rar,*.zip",
//'fileDesc' : "RAR *.rar",//限制文件类型
'auto' : false,
'multi' : true,//是否允许多文件上传
'simUploadLimit': 2,//同时运行上传的进程数量
'buttonText': " select files",
'onComplete': function(event, ID, fileObj, response, data) {
//response返回值:重命名后的文件名称,文件保存路径
var resultArray = response.split(",");
var realName = resultArray[0];//图片现在的名称,前面加上时间的图片名称,带扩展名
realName = $.trim(realName);
var p = "<%=basePath%>static/uploads/"+realName;
$(".showImgs").attr("src",p);
}
});
});
</script>
</html>
上传文件:添加servlet方法(或者使用uploadFile.jsp)
(1)//servlet方法代码
@RequestMapping("uploadFile")
@ResponseBody
public String uploadifyStart(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException{
String savePath = request.getSession().getServletContext().getRealPath("/");
String PATH = "/static/uploads/";
savePath = savePath + PATH;
File f1 = new File(savePath);
//System.out.println(savePath);
if (!f1.exists()) {
f1.mkdirs();
}
DiskFileItemFactory fac = new DiskFileItemFactory();
ServletFileUpload upload = new ServletFileUpload(fac);
upload.setHeaderEncoding("utf-8");
List fileList = null;
try {
fileList = upload.parseRequest(request);
} catch (FileUploadException ex) {
return "";
}
Iterator<FileItem> it = fileList.iterator();
String name = "";
String extName = "";
while (it.hasNext()) {
FileItem item = it.next();
if (!item.isFormField()) {
name = item.getName();
long size = item.getSize();
String type = item.getContentType();
//System.out.println(size + " " + type);
if (name == null || name.trim().equals("")) {
continue;
}
// 扩展名格式:
if (name.lastIndexOf(".") >= 0) {
extName = name.substring(name.lastIndexOf("."));
}
File file = null;
do {
// 生成文件名:
name = UUID.randomUUID().toString();
file = new File(savePath + name + extName);
} while (file.exists());
File saveFile = new File(savePath + name + extName);
try {
item.write(saveFile);
} catch (Exception e) {
e.printStackTrace();
}
}
}
return name + extName;
}
(2)uploadFile.jsp实现上传
//uploadFile.jsp代码
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ page import="java.io.*, java.util.*, org.apache.commons.fileupload.*" %>
<%@ page import="org.apache.commons.fileupload.disk.*, org.apache.commons.fileupload.servlet.*" %>
<%!
String PATH = "/static/uploads/";
public void upload(HttpServletRequest request, HttpServletResponse response)throws ServletException, IOException {
String savePath = this.getServletConfig().getServletContext().getRealPath("");
savePath = savePath + PATH;
File f1 = new File(savePath);
System.out.println(savePath);
if (!f1.exists()) {
f1.mkdirs();
}
DiskFileItemFactory fac = new DiskFileItemFactory();
ServletFileUpload upload = new ServletFileUpload(fac);
upload.setHeaderEncoding("utf-8");
List fileList = null;
try {
fileList = upload.parseRequest(request);
} catch (FileUploadException ex) {
return;
}
Iterator<FileItem> it = fileList.iterator();
String name = "";
String extName = "";
while (it.hasNext()) {
FileItem item = it.next();
if (!item.isFormField()) {
name = item.getName();
long size = item.getSize();
String type = item.getContentType();
System.out.println(size + " " + type);
if (name == null || name.trim().equals("")) {
continue;
}
// 扩展名格式:
if (name.lastIndexOf(".") >= 0) {
extName = name.substring(name.lastIndexOf("."));
}
File file = null;
do {
// 生成文件名:
name = UUID.randomUUID().toString();
file = new File(savePath + name + extName);
} while (file.exists());
File saveFile = new File(savePath + name + extName);
try {
item.write(saveFile);
} catch (Exception e) {
e.printStackTrace();
}
}
}
response.getWriter().print(name + extName);
}
%>
<%
upload(request, response);
%>
4.uploadify文件下载地址:http://download.csdn.net/detail/lychaox/3677400
相关文章推荐
- viewpager 简介及大量页面加载的方法推荐
- 学习nodejs之hello world
- 学习nodejs之restful
- restful阶段性理解
- scala使用多线程示例
- 中文验证码
- Android UI之Notification
- BestCoder Round #54 (div.2) HDOJ 5427 A problem of sorting(模拟)
- 获取沙盒路径,Documents目录路径,Caches目录路径,tmp目录路径的方法
- hdu 5428 The Factor(唯一分解定理)
- 生成浮点型随机数相加
- error: allocating an object of abstract class type
- 手工删除Oracle 12C数据库实例
- hdu4961 Boring Sum(数学)
- spring使用aop
- linux 第二天
- 单用户模式下修改root用户密码并设置系统默认进入多用户模式
- HDU1045 - Fire Net (深搜)
- 自学QT之字体选择对话框
- OGRE渲染过程