利用Python通过telnet、ssh连接交换机、路由器等设备配置备份 第2版(粗略版)
2015-09-05 16:58
761 查看
数据库设计:(主要存储设备信息如IP等)
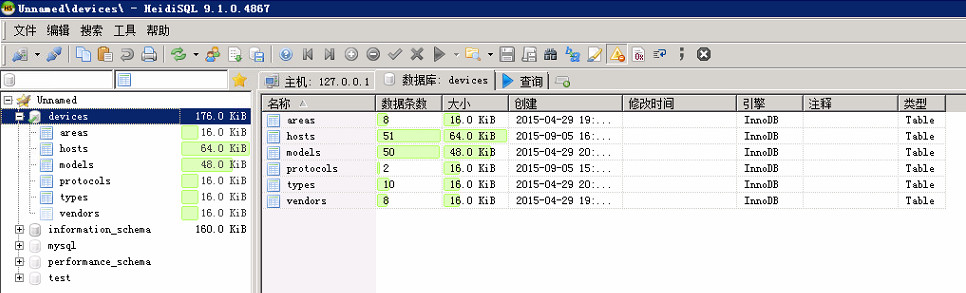
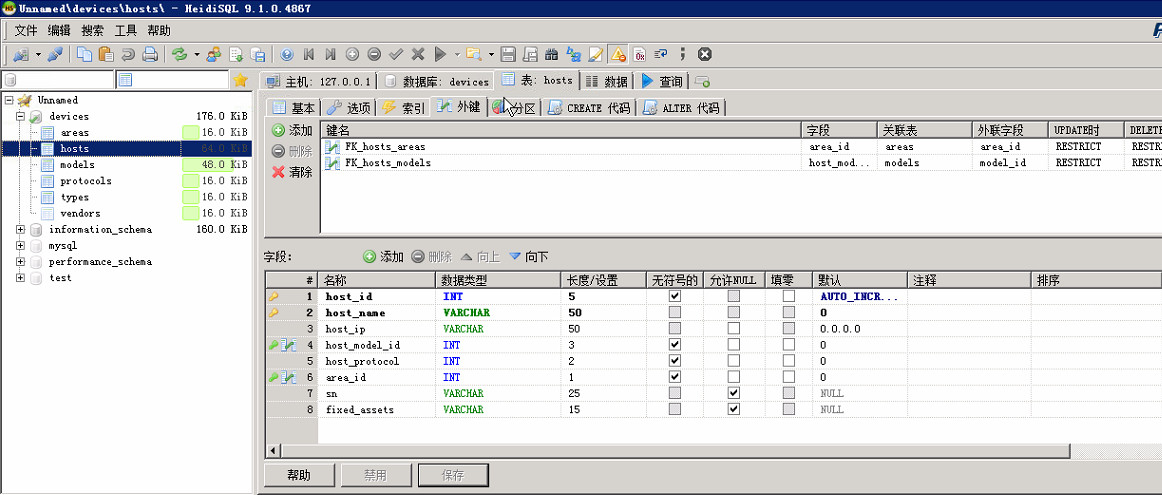
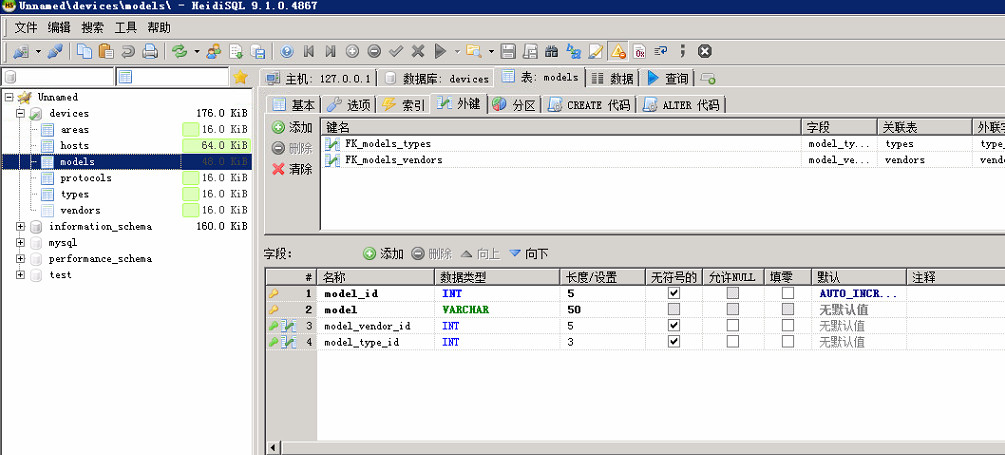
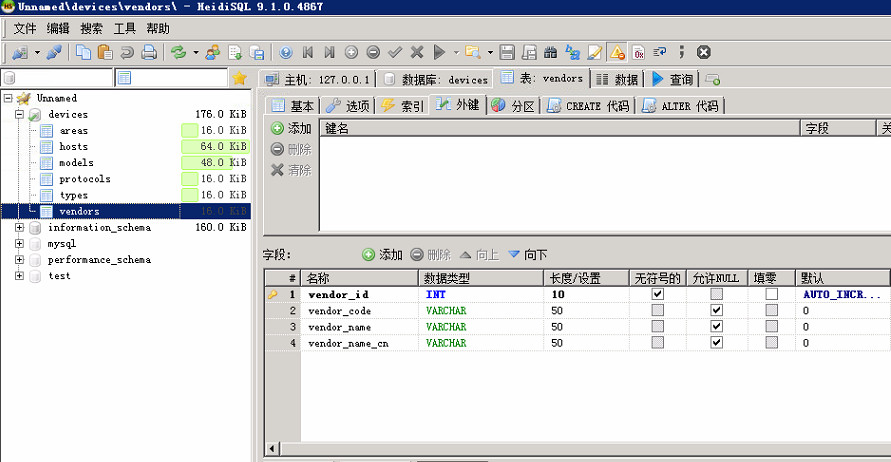
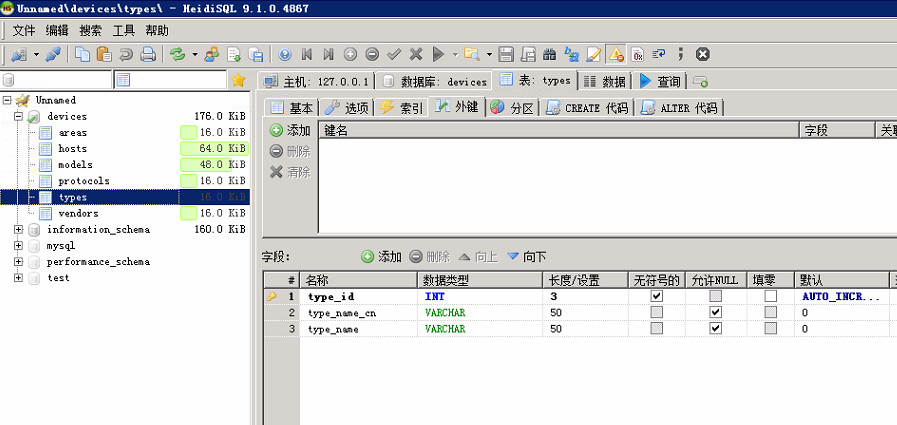
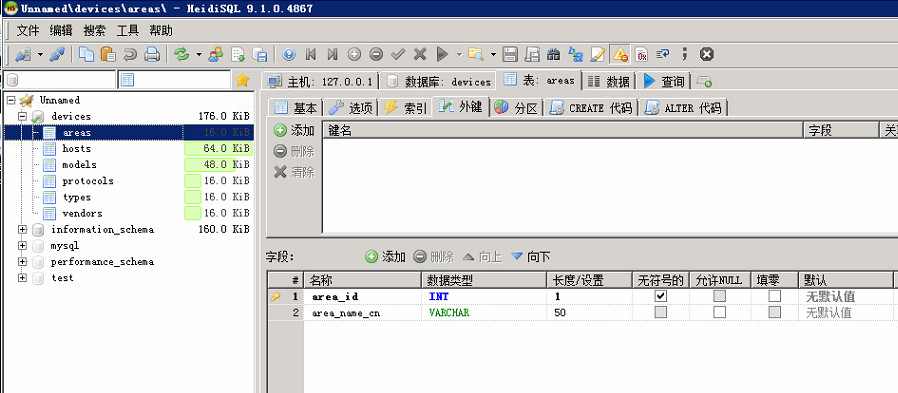
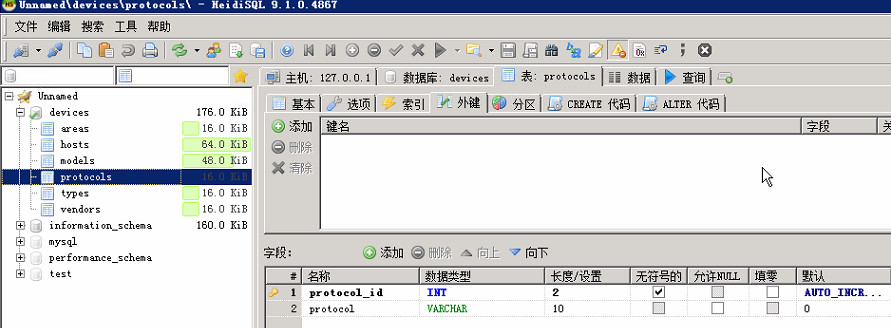
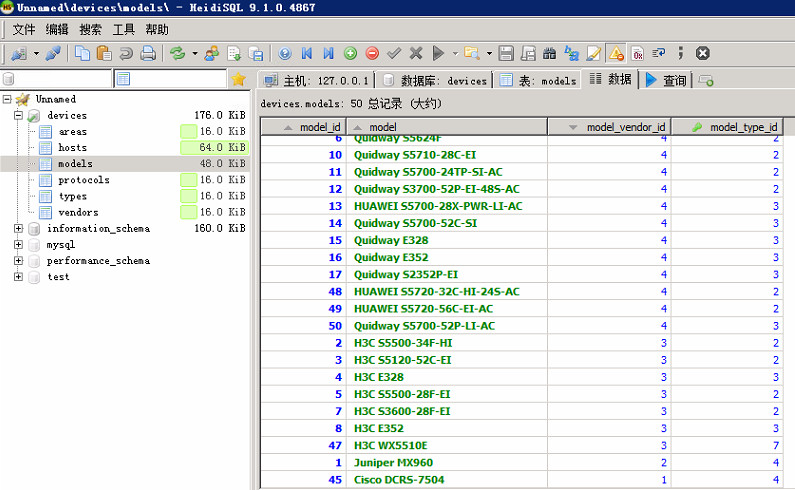
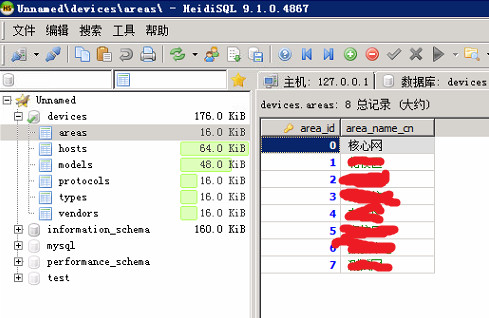
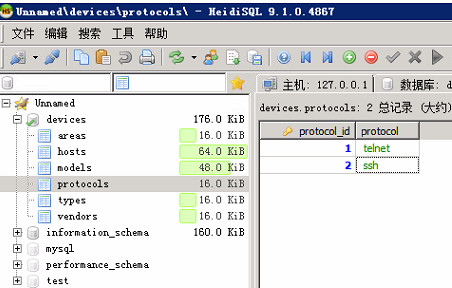
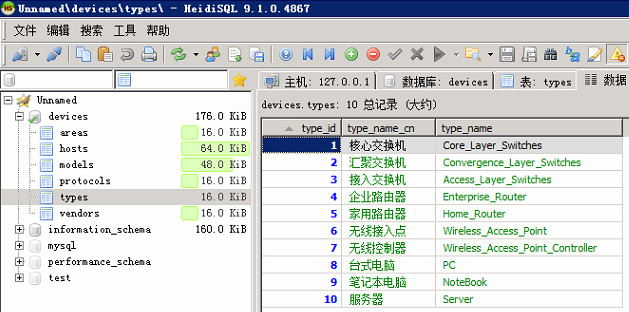
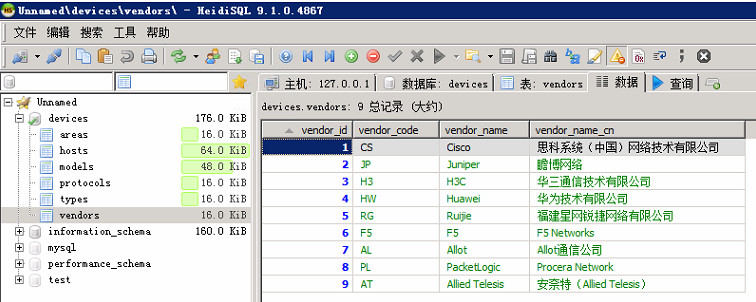
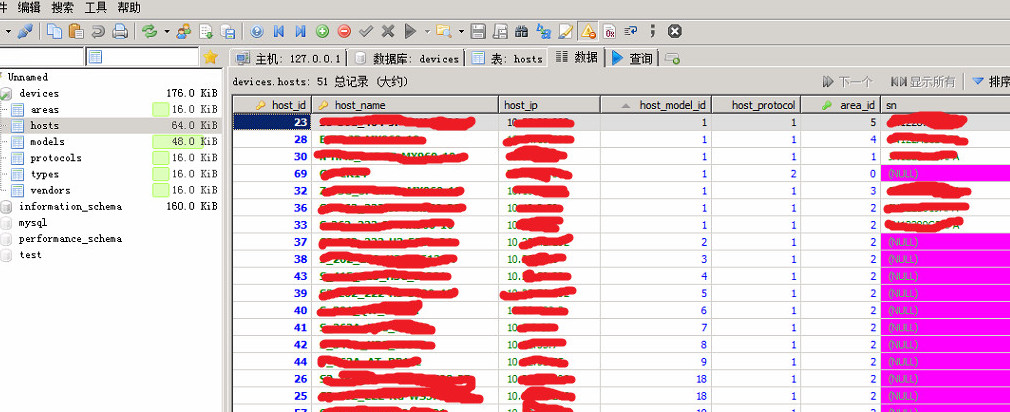
TFTP服务器准备:
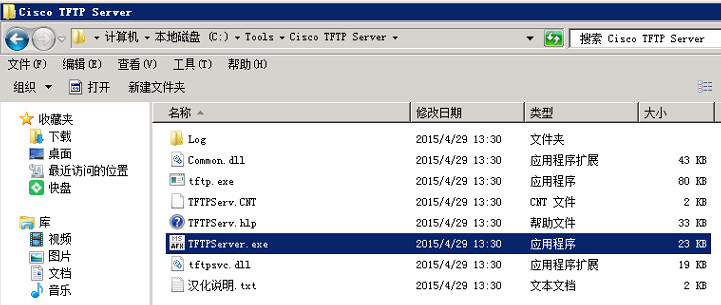
备份连接设备备份配置的代码:
转载请注明原文链接:http://my.oschina.net/caiyuanbo/blog/501358
暂时没时间添加注解及详细解释说明,代码比较简单,可能有些纰漏,如果有问题可以跟我一起研究。
PS:其实是人懒不想注释&优化。
QQ:384152164
E-mail:384152164@qq.com
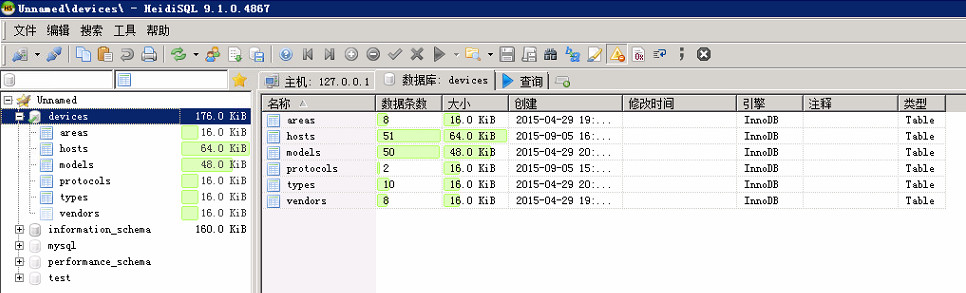
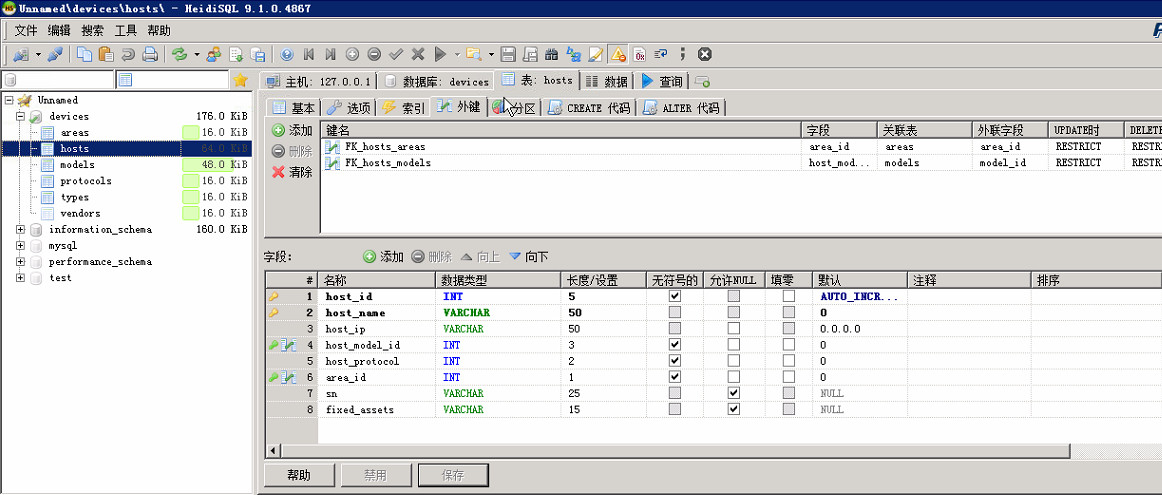
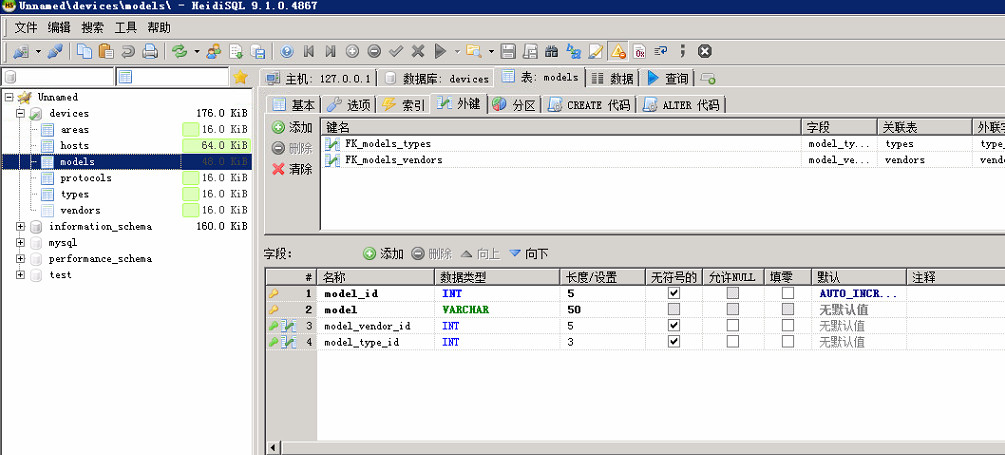
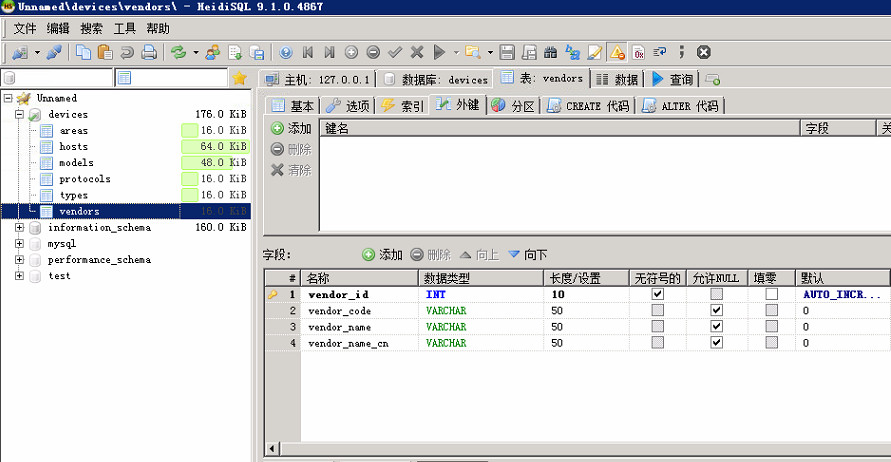
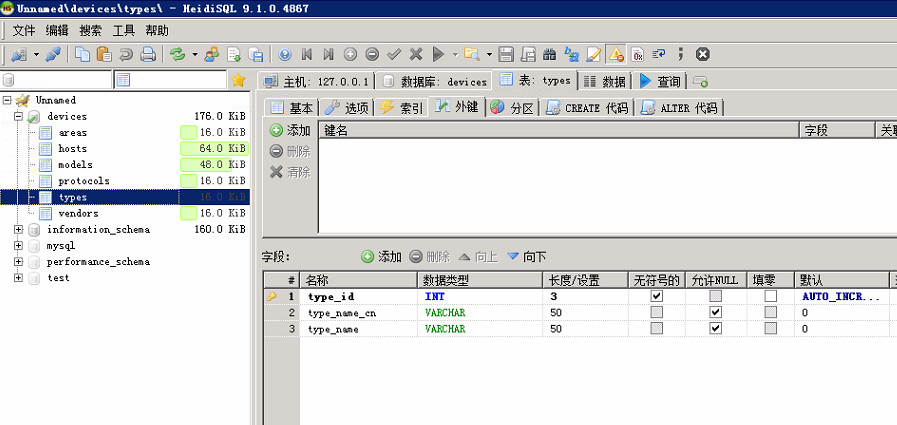
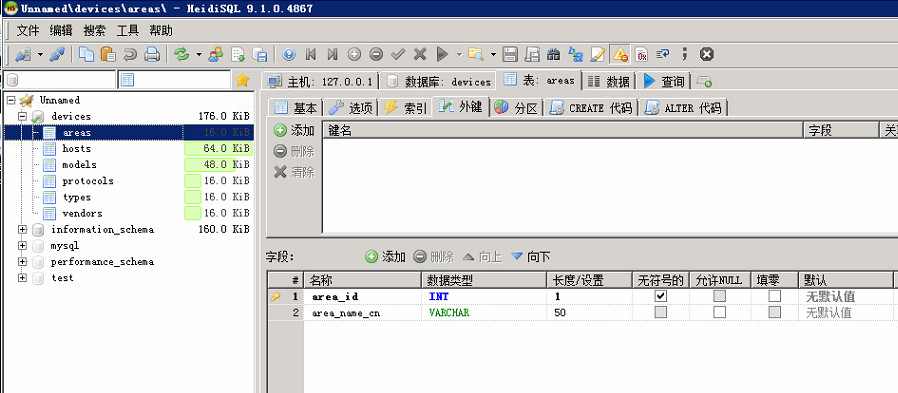
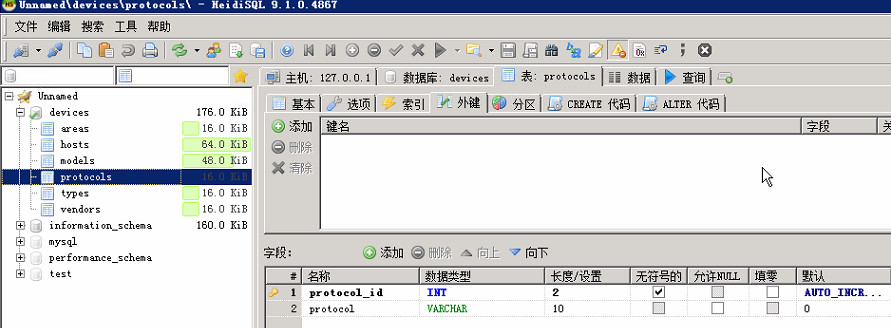
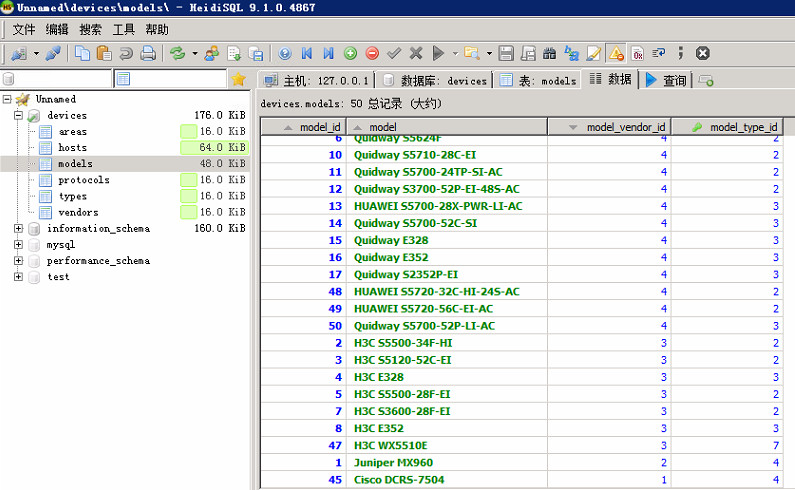
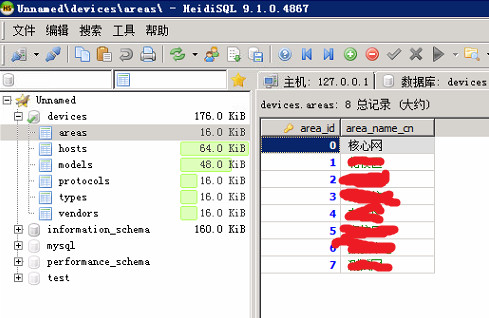
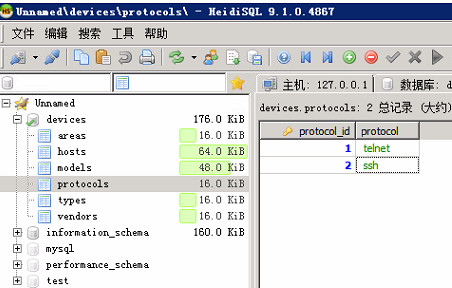
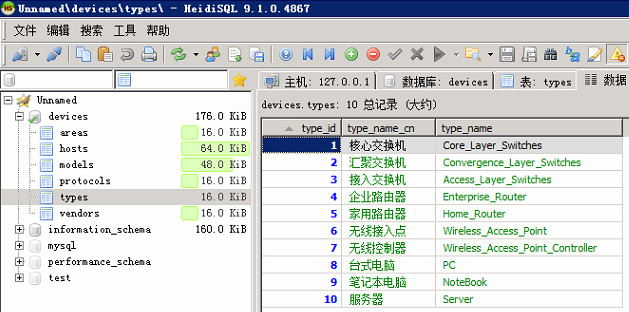
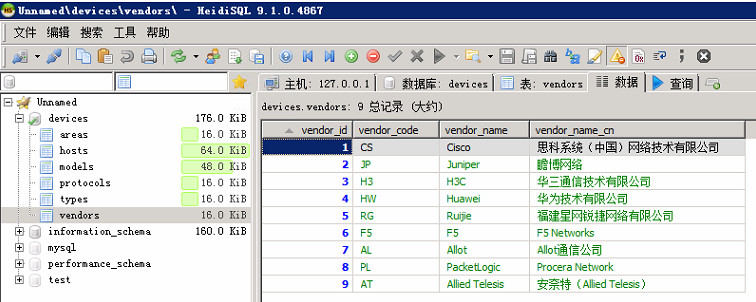
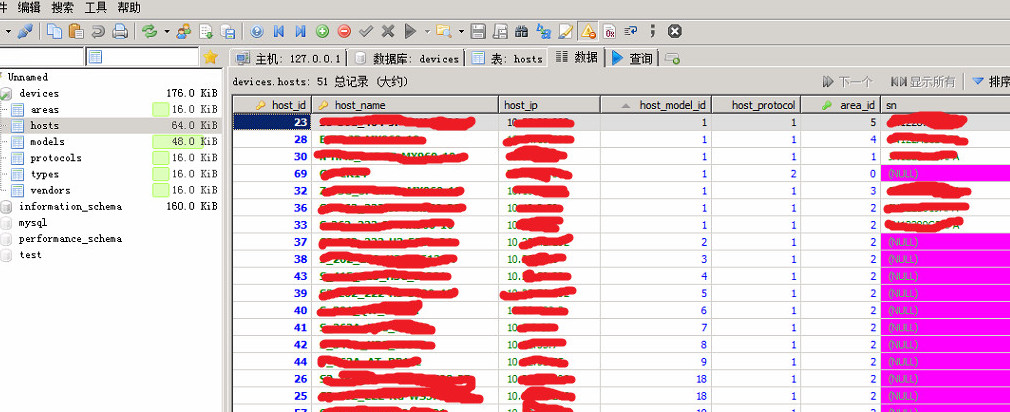
TFTP服务器准备:
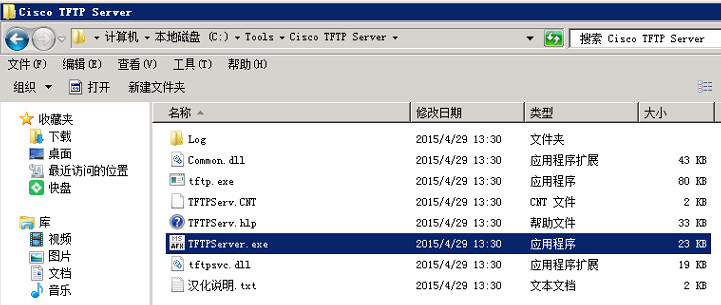
备份连接设备备份配置的代码:
import sys import telnetlib import time import pymysql import os import shutil import logging import paramiko def sqlconnect(): cur_host = conn.cursor() sql_host = "select host_name,host_ip,area_id,host_model_id,host_protocol from hosts" n_host = cur_host.execute(sql_host) for row_host in cur_host.fetchall(): host_name = row_host[0] host_ip = row_host[1] host_area = row_host[2] host_model_id = row_host[3] host_protocol = row_host[4] if host_protocol == 1: backup_telnet(host_name, host_ip, host_area, host_model_id) elif host_protocol == 2: backup_ssh(host_name, host_ip, host_area, host_model_id) def backup_telnet(b_name, b_ip, b_area, b_model): if 2 <= b_model <= 3 or b_model == 5 or b_model == 47: backup_telnet_h3_1(b_name, b_ip, b_area) elif b_model == 4 or 7 <= b_model <= 8: backup_telnet_h3_2(b_name, b_ip, b_area) elif 10 <= b_model <= 14 or b_model == 17 or 48 <= b_model <=50: backup_telnet_hw_1(b_name, b_ip, b_area) elif b_model == 6 or 15 <= b_model <= 16: backup_telnet_hw_2(b_name, b_ip, b_area) elif 19 <= b_model <= 38 or 40 <= b_model <= 44 or b_model == 46: backup_telnet_rg_1(b_name, b_ip, b_area) elif b_model == 18: backup_telnet_rg_2(b_name, b_ip, b_area) elif b_model == 39: backup_telnet_rg_3(b_name, b_ip, b_area) elif b_model == 1: backup_telnet_jp_1(b_name, b_ip, b_area) elif b_model ==9 : backup_telnet_at_1(b_name, b_ip, b_area) else: logging.warning(b_name + "(" + b_ip + ") 暂不支持该设备!") def backup_ssh(b_name, b_ip, b_area, b_model): if 2 <= b_model <= 3 or b_model == 5 or b_model == 47: logging.warning(b_name + "(" + b_ip + ") 暂不支持该设备!") elif b_model == 4 or 7 <= b_model <= 8: logging.warning(b_name + "(" + b_ip + ") 暂不支持该设备!") elif 10 <= b_model <= 14 or b_model == 17 or 48 <= b_model <=50: logging.warning(b_name + "(" + b_ip + ") 暂不支持该设备!") elif b_model == 6 or 15 <= b_model <= 16: logging.warning(b_name + "(" + b_ip + ") 暂不支持该设备!") elif 19 <= b_model <= 38 or 40 <= b_model <= 44 or b_model == 46: logging.warning(b_name + "(" + b_ip + ") 暂不支持该设备!") elif b_model == 18: logging.warning(b_name + "(" + b_ip + ") 暂不支持该设备!") elif b_model == 39: logging.warning(b_name + "(" + b_ip + ") 暂不支持该设备!") elif b_model == 1: backup_ssh_jp_1(b_name, b_ip, b_area) elif b_model ==9 : logging.warning(b_name + "(" + b_ip + ") 暂不支持该设备!") else: logging.warning(b_name + "(" + b_ip + ") 暂不支持该设备!") def backup_telnet_jp_1(name, ip, area): host = {} host['name'] = name.encode(encoding="utf-8") host['ip'] = ip.encode(encoding="utf-8") host['user'] = b"用户名" host['password'] = b"密码" host['configure'] = b"configure" host['show_config'] = b"show | display set" try: tn = telnetlib.Telnet(host['ip']) tn.set_debuglevel(2) tn.read_until(b"login:", timeout=2) tn.write(host['user']+b"\n") tn.read_until(b"Password:", timeout=2) tn.write(host['password']+b"\n") tn.read_until(b">", timeout=2) tn.write(host['configure']+b"\n") tn.read_until(b"#", timeout=2) tn.write(host['show_config']+b"\n ") time.sleep(6) read_show = tn.read_very_eager() if str(read_show).index("[edit]")>0: read_file_temp = open(tftp_dir + 'config-tmp.txt','wb') read_file_temp.write(read_show) read_file_temp.close() replace_1 = b"---(more)---" read_file_input = open(tftp_dir + 'config-tmp.txt','rb') read_file_output = open(tftp_dir + 'config.txt','wb') for read_line in read_file_input: read_file_output.write((read_line.replace(replace_1, b"").lstrip())) read_file_input.close() os.remove(tftp_dir + "config-tmp.txt") read_file_output.close() movefile_txt(name, ip, area) except TimeoutError: logging.warning(name + "(" + ip + ") TimeoutError: [WinError 10060] 由于连接方在一段时间后没有正确答复或连接的主机没有反应,连接尝试失败。") except FileNotFoundError: logging.error(name + "(" + ip + ") FileNotFoundError: 未成功备份 show | display set !") except ConnectionResetError: logging.warning(name + "(" + ip + ") ConnectionResetError: [WinError 10054] 远程主机强迫关闭了一个现有的连接。") except ValueError: logging.warning(name + "(" + ip + ") ValueError: substring not found 备份失败,可能是由于密码错误导致。") except: logging.error(name + "(" + ip + ") 发生未知错误!") def backup_ssh_jp_1(name, ip, area): host = {} host['name'] = name.encode(encoding="utf-8") host['ip'] = ip.encode(encoding="utf-8") host['user'] = b"用户名" host['password'] = b"密码" host['cmd'] = b"configure;show | display set" try: ssh = paramiko.SSHClient() ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) ssh.connect(host['ip'], 22, host['user'], host['password'], timeout=3) stdin, stdout, stderr = ssh.exec_command(host['cmd']) read_show = stdout.read() ssh.close() if str(read_show).index("host-name")>0: read_file_temp = open(tftp_dir + 'config-tmp.txt','wb') read_file_temp.write(read_show) read_file_temp.close() replace_1 = b"---(more)---" read_file_input = open(tftp_dir + 'config-tmp.txt','rb') read_file_output = open(tftp_dir + 'config.txt','wb') for read_line in read_file_input: read_file_output.write((read_line.replace(replace_1, b"").lstrip())) read_file_input.close() os.remove(tftp_dir + "config-tmp.txt") read_file_output.close() movefile_txt(name, ip, area) except TimeoutError: logging.warning(name + "(" + ip + ") TimeoutError: [WinError 10060] 由于连接方在一段时间后没有正确答复或连接的主机没有反应,连接尝试失败。") except FileNotFoundError: logging.error(name + "(" + ip + ") FileNotFoundError: 未成功备份 show | display set !") except ConnectionResetError: logging.warning(name + "(" + ip + ") ConnectionResetError: [WinError 10054] 远程主机强迫关闭了一个现有的连接。") except ValueError: logging.warning(name + "(" + ip + ") ValueError: substring not found 备份失败,可能是由于密码错误导致。") except: logging.error(name + "(" + ip + ") 发生未知错误!") def backup_telnet_at_1(name, ip, area): host = {} host['name'] = name.encode(encoding="utf-8") host['ip'] = ip.encode(encoding="utf-8") host['user'] = b"用户名" host['password'] = b"密码" host['show_config'] = b"show config dynamic" try: tn = telnetlib.Telnet(host['ip']) tn.set_debuglevel(2) tn.read_until(b"login:", timeout=2) tn.write(host['user']+b"\n") tn.read_until(b"Password:", timeout=2) tn.write(host['password']+b"\n") tn.read_until(b">", timeout=2) tn.write(host['show_config']+b"\n ") time.sleep(6) read_show = tn.read_very_eager() if str(read_show).index(">")>0: read_file_temp = open(tftp_dir + 'config-tmp.txt','wb') read_file_temp.write(read_show) read_file_temp.close() replace_1 = b"---(more)---" read_file_input = open(tftp_dir + 'config-tmp.txt','rb') read_file_output = open(tftp_dir + 'config.txt','wb') for read_line in read_file_input: read_file_output.write((read_line.replace(replace_1, b"").lstrip())) read_file_input.close() os.remove(tftp_dir + "config-tmp.txt") read_file_output.close() movefile_txt(name, ip, area) except TimeoutError: logging.warning(name + "(" + ip + ") TimeoutError: [WinError 10060] 由于连接方在一段时间后没有正确答复或连接的主机没有反应,连接尝试失败。") except FileNotFoundError: logging.error(name + "(" + ip + ") FileNotFoundError: 未成功备份 show | display set !") except ConnectionResetError: logging.warning(name + "(" + ip + ") ConnectionResetError: [WinError 10054] 远程主机强迫关闭了一个现有的连接。") except ValueError: logging.warning(name + "(" + ip + ") ValueError: substring not found 备份失败,可能是由于密码错误导致。") except: logging.error(name + "(" + ip + ") 发生未知错误!") def backup_telnet_h3_1(name, ip, area): host = {} host['name'] = name.encode(encoding="utf-8") host['ip'] = ip.encode(encoding="utf-8") host['user'] = b"用户名" host['password'] = b"密码" host['tftp'] = b"tftp <TFTP服务器IP> put startup.cfg config.txt" try: tn = telnetlib.Telnet(host['ip']) tn.set_debuglevel(2) tn.read_until(b"Username:", timeout=2) tn.write(host['user']+b"\n") tn.read_until(b"Password:", timeout=2) tn.write(host['password']+b"\n") tn.read_until(b">", timeout=2) tn.write(host['tftp']+b"\n") tn.read_until(b"successfully", timeout=6) movefile_txt(name, ip, area) except TimeoutError: logging.warning(name + "(" + ip + ") TimeoutError: [WinError 10060] 由于连接方在一段时间后没有正确答复或连接的主机没有反应,连接尝试失败。") except FileNotFoundError: logging.error(name + "(" + ip + ") FileNotFoundError: 未成功备份startup.cfg!") except ConnectionResetError: logging.warning(name + "(" + ip + ") ConnectionResetError: [WinError 10054] 远程主机强迫关闭了一个现有的连接。") except: logging.error(name + "(" + ip + ") 发生未知错误!") def backup_telnet_h3_2(name, ip, area): host = {} host['name'] = name.encode(encoding="utf-8") host['ip'] = ip.encode(encoding="utf-8") host['user'] = b"用户名" host['password'] = b"密码" host['tftp'] = b"tftp <TFTP服务器IP> put config.cfg config.txt" try: tn = telnetlib.Telnet(host['ip']) tn.set_debuglevel(2) tn.read_until(b"Username:", timeout=2) tn.write(host['user']+b"\n") tn.read_until(b"Password:", timeout=2) tn.write(host['password']+b"\n") tn.read_until(b">", timeout=2) tn.write(host['tftp']+b"\n") tn.read_until(b"successfully", timeout=6) movefile_txt(name, ip, area) except TimeoutError: logging.warning(name + "(" + ip + ") TimeoutError: [WinError 10060] 由于连接方在一段时间后没有正确答复或连接的主机没有反应,连接尝试失败。") except FileNotFoundError: logging.error(name + "(" + ip + ") FileNotFoundError: 未成功备份config.cfg!") except ConnectionResetError: logging.warning(name + "(" + ip + ") ConnectionResetError: [WinError 10054] 远程主机强迫关闭了一个现有的连接。") except: logging.error(name + "(" + ip + ") 发生未知错误!") def backup_telnet_hw_1(name, ip, area): host = {} host['name'] = name.encode(encoding="utf-8") host['ip'] = ip.encode(encoding="utf-8") host['user'] = b"用户名" host['password'] = b"密码" host['tftp'] = b"tftp <TFTP服务器IP> put vrpcfg.zip config.zip" try: tn = telnetlib.Telnet(host['ip']) tn.set_debuglevel(2) tn.read_until(b"Username:", timeout=2) tn.write(host['user']+b"\n") tn.read_until(b"Password:", timeout=2) tn.write(host['password']+b"\n") tn.read_until(b">", timeout=2) tn.write(host['tftp']+b"\n") tn.read_until(b"successfully", timeout=6) movefile_zip(name, ip, area) except TimeoutError: logging.warning(name + "(" + ip + ") TimeoutError: [WinError 10060] 由于连接方在一段时间后没有正确答复或连接的主机没有反应,连接尝试失败。") except FileNotFoundError: logging.error(name + "(" + ip + ") FileNotFoundError: 未成功备份config.txt!") except ConnectionResetError: logging.warning(name + "(" + ip + ") ConnectionResetError: [WinError 10054] 远程主机强迫关闭了一个现有的连接。") except: logging.error(name + "(" + ip + ") 发生未知错误!") def backup_telnet_hw_2(name, ip, area): host = {} host['name'] = name.encode(encoding="utf-8") host['ip'] = ip.encode(encoding="utf-8") host['user'] = b"用户名" host['password'] = b"密码" host['tftp'] = b"tftp <TFTP服务器IP> put vrpcfg.cfg config.txt" try: tn = telnetlib.Telnet(host['ip']) tn.set_debuglevel(2) tn.read_until(b"Username:", timeout=2) tn.write(host['user']+b"\n") tn.read_until(b"Password:", timeout=2) tn.write(host['password']+b"\n") tn.read_until(b">", timeout=2) tn.write(host['tftp']+b"\n") tn.read_until(b"successfully", timeout=6) movefile_txt(name, ip, area) except TimeoutError: logging.warning(name + "(" + ip + ") TimeoutError: [WinError 10060] 由于连接方在一段时间后没有正确答复或连接的主机没有反应,连接尝试失败。") except FileNotFoundError: logging.error(name + "(" + ip + ") FileNotFoundError: 未成功备份vrpcfg.cfg!") except ConnectionResetError: logging.warning(name + "(" + ip + ") ConnectionResetError: [WinError 10054] 远程主机强迫关闭了一个现有的连接。") except: logging.error(name + "(" + ip + ") 发生未知错误!") def backup_telnet_rg_1(name, ip, area): host = {} host['name'] = name.encode(encoding="utf-8") host['ip'] = ip.encode(encoding="utf-8") host['user'] = b"用户名" host['password'] = b"密码" host['tftp'] = b"copy flash:config.text tftp://<TFTP服务器IP>/config.txt" host['config'] = b"configure terminal" host['user_config'] = b"no username heqijun" host['exit'] = b"exit" host['wr'] = b"wr" try: tn = telnetlib.Telnet(host['ip']) tn.set_debuglevel(2) tn.read_until(b"Username:", timeout=2) tn.write(host['user']+b"\n") tn.read_until(b"Password:", timeout=2) tn.write(host['password']+b"\n") tn.read_until(b"#", timeout=2) tn.write(host['tftp']+b"\n") tn.read_until(b"length", timeout=6) movefile_txt(name, ip, area) except TimeoutError: logging.warning(name + "(" + ip + ") TimeoutError: [WinError 10060] 由于连接方在一段时间后没有正确答复或连接的主机没有反应,连接尝试失败。") except FileNotFoundError: logging.error(name + "(" + ip + ") FileNotFoundError: 未成功备份config.txt!") except ConnectionResetError: logging.warning(name + "(" + ip + ") ConnectionResetError: [WinError 10054] 远程主机强迫关闭了一个现有的连接。") except: logging.error(name + "(" + ip + ") 发生未知错误!") def backup_telnet_rg_2(name, ip, area): host = {} host['name'] = name.encode(encoding="utf-8") host['ip'] = ip.encode(encoding="utf-8") host['user'] = b"用户名" host['password'] = b"密码" host['tftp'] = b"copy flash:ap-config.text tftp://<TFTP服务器IP>/ap-config.txt" try: tn = telnetlib.Telnet(host['ip']) tn.set_debuglevel(2) tn.read_until(b"Username:", timeout=2) tn.write(host['user']+b"\n") tn.read_until(b"Password:", timeout=2) tn.write(host['password']+b"\n") tn.read_until(b"#", timeout=2) tn.write(host['tftp']+b"\n") tn.read_until(b"length", timeout=6) except TimeoutError: logging.warning(name + "(" + ip + ") TimeoutError: [WinError 10060] 由于连接方在一段时间后没有正确答复或连接的主机没有反应,连接尝试失败。") except FileNotFoundError: logging.error(name + "(" + ip + ") FileNotFoundError: 未成功备份ap-config.txt!") except ConnectionResetError: logging.warning(name + "(" + ip + ") ConnectionResetError: [WinError 10054] 远程主机强迫关闭了一个现有的连接。") except: logging.error(name + "(" + ip + ") 发生未知错误!") backup_telnet_rg_1(name, ip, area) def backup_telnet_rg_3(name, ip, area): host = {} host['name'] = name.encode(encoding="utf-8") host['ip'] = ip.encode(encoding="utf-8") host['user'] = b"用户名" host['password'] = b"密码" host['tftp'] = b"copy flash:config.text tftp://<TFTP服务器IP>/config.txt" try: tn = telnetlib.Telnet(host['ip']) tn.set_debuglevel(2) tn.read_until(b"Username:", timeout=2) tn.write(host['user']+b"\n") tn.read_until(b"Password:", timeout=2) tn.write(host['password']+b"\n") tn.read_until(b"#", timeout=2) tn.write(host['tftp']+b"\n") tn.read_until(b"length", timeout=6) movefile_txt(name, ip, area) except TimeoutError: logging.warning(name + "(" + ip + ") TimeoutError: [WinError 10060] 由于连接方在一段时间后没有正确答复或连接的主机没有反应,连接尝试失败。") except FileNotFoundError: logging.error(name + "(" + ip + ") FileNotFoundError: 未成功备份config.txt!") except ConnectionResetError: logging.warning(name + "(" + ip + ") ConnectionResetError: [WinError 10054] 远程主机强迫关闭了一个现有的连接。") except: logging.error(name + "(" + ip + ") 发生未知错误!") def movefile_zip(file_name, file_ip, file_area): cur_file_area = conn.cursor() sql_file_area = "select area_name_cn from areas where area_id=" + str(file_area) n_file_area = cur_file_area.execute(sql_file_area) for row_area in cur_file_area.fetchall(): file_area_name = row_area[0] move_dir = backup_dir + file_area_name + "\\" + file_year + "\\" + file_mon + "\\" + file_mday if not os.path.isdir(move_dir): os.makedirs(move_dir) shutil.move(tftp_dir + "config.zip", move_dir + "\\" + file_name + "(" + file_ip + ")_" + file_time + ".zip") logging.info(file_name + "(" + file_ip + "): *.zip 配置备份成功!") def movefile_txt(file_name, file_ip, file_area): cur_file_area = conn.cursor() sql_file_area = "select area_name_cn from areas where area_id=" + str(file_area) n_file_area = cur_file_area.execute(sql_file_area) for row_area in cur_file_area.fetchall(): file_area_name = row_area[0] move_dir = backup_dir + file_area_name + "\\" + file_year + "\\" + file_mon + "\\" + file_mday if not os.path.isdir(move_dir): os.makedirs(move_dir) shutil.move(tftp_dir + "config.txt", move_dir + "\\" + file_name + "(" + file_ip + ")_" + file_time + ".txt") logging.info(file_name + "(" + file_ip + "): *.txt 配置备份成功!") try: shutil.move(tftp_dir + "ap-config.txt", move_dir + "\\" + file_name + "(" + file_ip + ")_AP_" + file_time + ".txt") logging.info(file_name + "(" + file_ip + "): ap-config.text 配置备份成功!") except: print() conn = pymysql.connect(host='127.0.0.1', user='数据库用户名', passwd='数据库密码', db='devices', charset='utf8') file_year = time.strftime("%Y", time.localtime()) file_mon = time.strftime("%m", time.localtime()) file_mday = time.strftime("%d", time.localtime()) file_time = time.strftime("%Y%m%d", time.localtime()) backup_dir = os.getcwd() + "\\备份路径\\" tftp_dir = os.getcwd() + "\\TFTP\\Root\\" logging_dir = os.getcwd() + "\\备份路径\\备份程序执行日志" logging_name = "log_" + file_time + ".txt" if not os.path.isdir(logging_dir): os.makedirs(logging_dir) logging.basicConfig(filename = os.path.join(logging_dir, logging_name), level = logging.INFO, filemode = "a", format = "%(asctime)s - %(levelname)s: %(message)s") #logging.debug("debug") #logging.info("info") #logging.warning("warn") #logging.error("error") sqlconnect() logging.shutdown()
转载请注明原文链接:http://my.oschina.net/caiyuanbo/blog/501358
暂时没时间添加注解及详细解释说明,代码比较简单,可能有些纰漏,如果有问题可以跟我一起研究。
PS:其实是人懒不想注释&优化。
QQ:384152164
E-mail:384152164@qq.com
相关文章推荐
- 测试比json更快更小的二进制数据传输格式Msgpack [pythono MessagePack 版本] - 峰云,就她了。 - 51CTO技术博客
- 分享一个分布式定时任务系统 ( python) - V2EX
- python 应用xml.dom.minidom读xml
- python 安装包error: Unable to find vcvarsall.bat
- 卸载使用“python setup.py install”安装的包
- python 递归遍历文件夹
- Python-selenium进阶操作
- python第二天 - 异常处理
- 使用python绘制4个相切的圆形
- Python Wheel (.whl)文件安装实践
- 用Python制作新浪微博爬虫
- 零基础学python-11.3 代码分隔符
- 零基础学python-11.3 代码分隔符
- 当Python和R遇上北京二手房(下)
- 2015/9/4 Python基础(8):映射和集合类型
- 2小时玩转python基础(上)
- mac多版本python安装 pymysql
- 零基础学python-11.2 python语法规则
- 零基础学python-11.2 python语法规则
- Python中模块和包的概念