UI动图的制作
2015-08-28 20:09
387 查看
创建一个协议文件,把screen的width,height用宏定义
{
UIView *animationView;
}
@end
然后初始化animationView对象
@implementation ViewController
(void)viewDidLoad {
[super viewDidLoad];
animationView = [[UIView alloc] initWithFrame:CGRectMake(20, 20, 80, 80)];
animationView.backgroundColor = [UIColor redColor];
[self.view addSubview:animationView];
//layer那点事,layer相当于图片的图层
// CALayer *layer1 = [CALayer layer];
// layer1.frame = CGRectMake(0, 0, 40, 40);
// layer1.backgroundColor = [UIColor yellowColor].CGColor;
// [animationView.layer addSublayer:layer1];
// 接着初始化layerView
//让layer本身做动画
layerView = [[UIView alloc] initWithFrame:CGRectMake(Screen_Width/2-100, Screen_Height/2-100, 200, 200)];
[self.view addSubview:layerView];
//导入图片”1”
//初始化keyframeView,不过后面那个调用是什么意思?
keyframeView = [[UIView alloc] initWithFrame:CGRectMake(100, 120, 60, 30)];
keyframeView.backgroundColor = [UIColor clearColor];
keyframeView.layer.borderColor = [UIColor grayColor].CGColor;
keyframeView.layer.borderWidth = 1.0;
[self.view insertSubview:keyframeView aboveSubview:button];
//创建一个按钮,关联的方法为CATransactionLayerAnimation
button = [UIButton buttonWithType:UIButtonTypeSystem];
[button addTarget:self action:@selector(CATransactionLayerAnimation:) forControlEvents:UIControlEventTouchUpInside];
[button setTitle:@”做动画” forState:0];
button.frame = CGRectMake(Screen_Width-80, 180, 60, 30);
[self.view addSubview:button];
//初始化CATransactionLayer
CATransactionLayer = [CALayer layer];
CATransactionLayer.frame = CGRectMake(100, 100, 50, 50);
CATransactionLayer.backgroundColor = [UIColor yellowColor].CGColor;
[self.view.layer addSublayer:CATransactionLayer];
}
//下面是按钮方法的实现
-(void)buttonAction:(UIButton *)sender{
/*
// CABasicAnimation* fadeAnim = [CABasicAnimation animationWithKeyPath:@”opacity”];
// fadeAnim.fromValue = [NSNumber numberWithFloat:1.0];
// fadeAnim.toValue = [NSNumber numberWithFloat:0.0];
// fadeAnim.duration = 1.0;
// [animationView.layer addAnimation:fadeAnim forKey:@”opacity”];
CABasicAnimation *xRotation = [CABasicAnimation animationWithKeyPath:@”transform”];
xRotation.fromValue = [NSValue valueWithCATransform3D:CATransform3DIdentity];
xRotation.toValue = [NSValue valueWithCATransform3D:CATransform3DMakeRotation(M_PI, 1, 1, 1)];
xRotation.duration = 1.f;
xRotation.repeatCount = FLT_MAX;
xRotation.timingFunction = [CAMediaTimingFunction functionWithName:kCAMediaTimingFunctionLinear];
[animationView.layer addAnimation:xRotation forKey:@”vincent”];}
//改变大白的形态
-(void)CATransactionbuttonAction:(UIButton *)sender{
//实现大白跳的速度的改变
-(void)changeDabai:(NSTimer *)sender{
UIImage *image = [UIImage imageNamed:@”1”];
CGRect rect = CGRectZero;
if (CATransactionCount > 5) {
CATransactionCount = 0;
}
switch (CATransactionCount) {
case 0:
{
rect = CGRectMake(0, 0, 1, 0.75);
}
break;
case 1:
{
rect = CGRectMake(0, 0, 1, 0.5);
}
break;
case 2:
{
rect = CGRectMake(0, 0.25, 1, 0.5);
}
break;
case 3:
{
rect = CGRectMake(0, 0.25, 1, 0.5);
}
break;
case 4:
{
rect = CGRectMake(0, 0.5, 1, 1);
}
break;
case 5:
{
rect = CGRectMake(0, 0.25, 1, 1);
}
break;
}
-(void)keyframeAnimation:(UIButton *)sender{
CAKeyframeAnimation *keyAnimation = [CAKeyframeAnimation animationWithKeyPath:@”transform.scale.y”];
keyAnimation.values = @[@0,@-10,@10,@-10,@0];
// keyAnimation.path
keyAnimation.duration = 0.4;
keyAnimation.additive = YES;
[keyframeView.layer addAnimation:keyAnimation forKey:@”shake”];
}
-(void)CATransactionLayerAnimation:(UIButton *)sender{
[CATransaction begin];
//设置动画时间
[CATransaction setValue:@1.0f forKey:kCATransactionAnimationDuration];
//改变layer位置
CGFloat pointY = CATransactionLayer.position.y> 250 ? 125:425;
CATransactionLayer.position = CGPointMake(CATransactionLayer.position.x, pointY);
}
(void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
// Dispose of a
4000
ny resources that can be recreated.
}
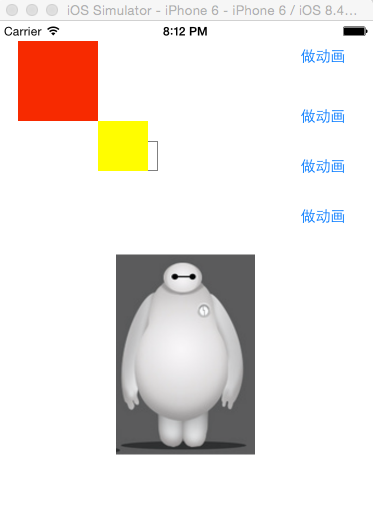
@end
效果如图
ifndef UI_05_02_PrefixHeader_pch
define UI_05_02_PrefixHeader_pch
define Screen_Width [[UIScreen mainScreen] bounds].size.width
define Screen_Height [[UIScreen mainScreen] bounds].size.height
endif
接着在ViewController.m文件中的interface.h声明如下对象的属性import “ViewController.h”
@interface ViewController (){
UIView *animationView;
UIView *layerView; int CATransactionCount; UIView *keyframeView; CALayer *CATransactionLayer;
}
@end
然后初始化animationView对象
@implementation ViewController
(void)viewDidLoad {
[super viewDidLoad];
animationView = [[UIView alloc] initWithFrame:CGRectMake(20, 20, 80, 80)];
animationView.backgroundColor = [UIColor redColor];
[self.view addSubview:animationView];
//layer那点事,layer相当于图片的图层
// CALayer *layer1 = [CALayer layer];
// layer1.frame = CGRectMake(0, 0, 40, 40);
// layer1.backgroundColor = [UIColor yellowColor].CGColor;
// [animationView.layer addSublayer:layer1];
// 接着初始化layerView
//让layer本身做动画
layerView = [[UIView alloc] initWithFrame:CGRectMake(Screen_Width/2-100, Screen_Height/2-100, 200, 200)];
[self.view addSubview:layerView];
//导入图片”1”
UIImage *image = [UIImage imageNamed:@"1"]; layerView.layer.contents = (__bridge id)(image.CGImage); layerView.layer.contentsGravity = kCAGravityResizeAspect; layerView.layer.contentsScale = image.scale; CATransactionCount = 0; //关于核心动画编程,就是给layer的属性做动画 //设置三个按钮 UIButton *button = [UIButton buttonWithType:UIButtonTypeSystem]; [button addTarget:self action:@selector(buttonAction:) forControlEvents:UIControlEventTouchUpInside]; [button setTitle:@"做动画" forState:0]; button.frame = CGRectMake(Screen_Width-80, 20, 60, 30); [self.view addSubview:button]; button = [UIButton buttonWithType:UIButtonTypeSystem]; [button addTarget:self action:@selector(CATransactionbuttonAction:) forControlEvents:UIControlEventTouchUpInside]; [button setTitle:@"做动画" forState:0]; button.frame = CGRectMake(Screen_Width-80, 80, 60, 30); [self.view addSubview:button]; button = [UIButton buttonWithType:UIButtonTypeSystem]; [button addTarget:self action:@selector(keyframeAnimation:) forControlEvents:UIControlEventTouchUpInside]; [button setTitle:@"做动画" forState:0]; button.frame = CGRectMake(Screen_Width-80, 130, 60, 30); [self.view addSubview:button];
//初始化keyframeView,不过后面那个调用是什么意思?
keyframeView = [[UIView alloc] initWithFrame:CGRectMake(100, 120, 60, 30)];
keyframeView.backgroundColor = [UIColor clearColor];
keyframeView.layer.borderColor = [UIColor grayColor].CGColor;
keyframeView.layer.borderWidth = 1.0;
[self.view insertSubview:keyframeView aboveSubview:button];
//创建一个按钮,关联的方法为CATransactionLayerAnimation
button = [UIButton buttonWithType:UIButtonTypeSystem];
[button addTarget:self action:@selector(CATransactionLayerAnimation:) forControlEvents:UIControlEventTouchUpInside];
[button setTitle:@”做动画” forState:0];
button.frame = CGRectMake(Screen_Width-80, 180, 60, 30);
[self.view addSubview:button];
//初始化CATransactionLayer
CATransactionLayer = [CALayer layer];
CATransactionLayer.frame = CGRectMake(100, 100, 50, 50);
CATransactionLayer.backgroundColor = [UIColor yellowColor].CGColor;
[self.view.layer addSublayer:CATransactionLayer];
}
//下面是按钮方法的实现
-(void)buttonAction:(UIButton *)sender{
/*
// CABasicAnimation* fadeAnim = [CABasicAnimation animationWithKeyPath:@”opacity”];
// fadeAnim.fromValue = [NSNumber numberWithFloat:1.0];
// fadeAnim.toValue = [NSNumber numberWithFloat:0.0];
// fadeAnim.duration = 1.0;
// [animationView.layer addAnimation:fadeAnim forKey:@”opacity”];
CGMutablePathRef thePath = CGPathCreateMutable(); CGPathMoveToPoint(thePath,NULL,74.0,74.0); CGPathAddCurveToPoint(thePath,NULL,74.0,500.0, 320.0,500.0, 320.0,74.0); CGPathAddCurveToPoint(thePath,NULL,320.0,500.0, 566.0,500.0, 566.0,74.0); CAKeyframeAnimation * theAnimation; // Create the animation object, specifying the position property as the key path. theAnimation=[CAKeyframeAnimation animationWithKeyPath:@"position"]; theAnimation.path=thePath; theAnimation.duration=5.0; // Add the animation to the layer. [animationView.layer addAnimation:theAnimation forKey:@"position"]; */ /* transform transform.rotation transform.translation transform.scale */ //旋转 /* CABasicAnimation *rotation = [CABasicAnimation animationWithKeyPath:@"transform.rotation"]; rotation.fromValue = @0; rotation.toValue = @(M_PI/2); rotation.duration = 1.f; rotation.repeatCount = FLT_MAX; rotation.timingFunction = [CAMediaTimingFunction functionWithName:kCAMediaTimingFunctionLinear]; [animationView.layer addAnimation:rotation forKey:@"vincent"]; */
CABasicAnimation *xRotation = [CABasicAnimation animationWithKeyPath:@”transform”];
xRotation.fromValue = [NSValue valueWithCATransform3D:CATransform3DIdentity];
xRotation.toValue = [NSValue valueWithCATransform3D:CATransform3DMakeRotation(M_PI, 1, 1, 1)];
xRotation.duration = 1.f;
xRotation.repeatCount = FLT_MAX;
xRotation.timingFunction = [CAMediaTimingFunction functionWithName:kCAMediaTimingFunctionLinear];
[animationView.layer addAnimation:xRotation forKey:@”vincent”];}
//改变大白的形态
-(void)CATransactionbuttonAction:(UIButton *)sender{
[NSTimer scheduledTimerWithTimeInterval:0.1 target:self selector:@selector(changeDabai:) userInfo:nil repeats:YES];}
//实现大白跳的速度的改变
-(void)changeDabai:(NSTimer *)sender{
UIImage *image = [UIImage imageNamed:@”1”];
CGRect rect = CGRectZero;
if (CATransactionCount > 5) {
CATransactionCount = 0;
}
switch (CATransactionCount) {
case 0:
{
rect = CGRectMake(0, 0, 1, 0.75);
}
break;
case 1:
{
rect = CGRectMake(0, 0, 1, 0.5);
}
break;
case 2:
{
rect = CGRectMake(0, 0.25, 1, 0.5);
}
break;
case 3:
{
rect = CGRectMake(0, 0.25, 1, 0.5);
}
break;
case 4:
{
rect = CGRectMake(0, 0.5, 1, 1);
}
break;
case 5:
{
rect = CGRectMake(0, 0.25, 1, 1);
}
break;
default: break; } CATransactionCount++; layerView.layer.contents = (__bridge id)image.CGImage; layerView.layer.contentsCenter = rect;
}
-(void)keyframeAnimation:(UIButton *)sender{
CAKeyframeAnimation *keyAnimation = [CAKeyframeAnimation animationWithKeyPath:@”transform.scale.y”];
keyAnimation.values = @[@0,@-10,@10,@-10,@0];
// keyAnimation.path
keyAnimation.duration = 0.4;
keyAnimation.additive = YES;
[keyframeView.layer addAnimation:keyAnimation forKey:@”shake”];
}
-(void)CATransactionLayerAnimation:(UIButton *)sender{
[CATransaction begin];
//设置动画时间
[CATransaction setValue:@1.0f forKey:kCATransactionAnimationDuration];
//改变layer位置
CGFloat pointY = CATransactionLayer.position.y> 250 ? 125:425;
CATransactionLayer.position = CGPointMake(CATransactionLayer.position.x, pointY);
[CATransaction commit];
}
(void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
// Dispose of a
4000
ny resources that can be recreated.
}
@end
效果如图
相关文章推荐
- 简述UIView、UIWindow和CALayer的理解
- 【UI初级 连载一】------iPhone开发入门
- 详解CALayer 和 UIView的区别和联系
- 创建一个可重用的UITableViewCell代码
- iOS:选择器控件UIPickerView的详解和演示
- value="hello boy"
- 【codechef】Chef and the Number Sequence(构成最长公共子序列为L的可能性)
- Android UI设计:自定义Dialog
- UI初级连载九----------UITableView的使用
- iOS 笔记五:手势识别 UIGestureRecognizer
- flume+kafka+Druid 流数据查询聚合工具
- UI初级连载八------------标签控制器
- ueditor配置
- easyui-treegrid篇
- UITableView
- UIControl
- POJ 题目3581 Sequence(后缀数组+离散化)
- NGUI
- UIDatePicker
- UIImagePicker照片选择器