uva_658_It's not a Bug, it's a Feature!(最短路)
2015-08-16 17:04
447 查看
It's not a Bug, it's a Feature!
SubmitStatus
Description
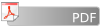
It is a curious fact that consumers buying a new software product generally donot expect the software to be bug-free. Can you imagine buying a car whose steering wheel only turns to the right? Or a CD-player that plays only CDs with country music
on them? Probably not. But for software systems it seems to be acceptable if they do not perform as they should do. In fact, many software companies have adopted the habit of sending out patches to fix bugs every few weeks after a new product is released (and
even charging money for the patches).
Tinyware Inc. is one of those companies. After releasing a new word processing software this summer, they have been producing patches ever since. Only this weekend they have realized a big problem with the patches they released. While all patches fix some
bugs, they often rely on other bugs to be present to be installed. This happens because to fix one bug, the patches exploit the special behavior of the program due to another bug.
More formally, the situation looks like this. Tinyware has found a total of
n bugs

in their software. And they have releasedm patches

. To apply patchpi to the software, the bugs

have to be present in the software, and the bugs

must be absent (of course

holds). The patch then fixes the bugs

(if they have been present) and introduces the new bugs

(where, again,

).
Tinyware's problem is a simple one. Given the original version of their software, which contains all the bugs inB, it is possible to apply a sequence of patches to the software which results in a bug- free version of the software? And if so, assuming
that every patch takes a certain time to apply, how long does the fastest sequence take?
m, the number of bugs and patches, respectively. These values satisfy

and

.
This is followed bym lines describing the m patches in order. Each line contains an integer, the time in seconds it takes to apply the patch, and two strings ofn characters each.
The first of these strings describes the bugs that have to be present or absent before the patch can be applied. Thei-th position of that string is a ``+'' if bug
bi has to be present, a ``-'' if bugbi has to be absent, and a `` 0'' if it doesn't matter whether the bug is present or not.
The second string describes which bugs are fixed and introduced by the patch. Thei-th position of that string is a ``+'' if bug
bi is introduced by the patch, a ``-'' if bugbi is removed by the patch (if it was present), and a ``0'' if bugbi is not affected by the patch (if it was
present before, it still is, if it wasn't, is still isn't).
The input is terminated by a description starting with n = m = 0. This test case should not be processed.
is such a sequence, output the time taken by the fastest sequence in the format shown in the sample output. If there is no such sequence, output ``Bugs cannot be fixed.''.
Print a blank line after each test case.
题意:补丁在修补bug时,有时候会引入新的bug。假定有n(n<=20)个潜在bug和m(m<=100)个补丁,每个补丁用两个长度为n的字符串表示,其中字符串的每个位置表示一个bug。第一个串表示打补丁前的状态(“-”表示该bug必须不存在,“+”表示必须存在,“0”表示无所谓),第二个串表示打补丁后的状态(“-”表示不存在,“+”表示存在,“0”表示不变)。每个补丁都有一个执行时间,你的任务是用最少的时间把一个所有bug都存在的软件通过打补丁的方式变得没有bug。一个补丁可以打多次。
分析:最短路。把状态看做结点,状态转移看做边,然后用spfa求解即可。结点数很多,多达2^n个,并且很多状态碰不到,所以不需要先把图存好,每次取出一个结点,直接枚举m个补丁,看是否能够打得上。
注:状态可以用二进制存。题目中巧妙运用位运算。
题目链接:http://acm.hust.edu.cn/vjudge/problem/viewProblem.action?id=22169
代码清单:
Time Limit: 3000MS | Memory Limit: Unknown | 64bit IO Format: %lld & %llu |
Description
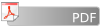
It is a curious fact that consumers buying a new software product generally donot expect the software to be bug-free. Can you imagine buying a car whose steering wheel only turns to the right? Or a CD-player that plays only CDs with country music
on them? Probably not. But for software systems it seems to be acceptable if they do not perform as they should do. In fact, many software companies have adopted the habit of sending out patches to fix bugs every few weeks after a new product is released (and
even charging money for the patches).
Tinyware Inc. is one of those companies. After releasing a new word processing software this summer, they have been producing patches ever since. Only this weekend they have realized a big problem with the patches they released. While all patches fix some
bugs, they often rely on other bugs to be present to be installed. This happens because to fix one bug, the patches exploit the special behavior of the program due to another bug.
More formally, the situation looks like this. Tinyware has found a total of
n bugs
in their software. And they have releasedm patches
. To apply patchpi to the software, the bugs
have to be present in the software, and the bugs
must be absent (of course
holds). The patch then fixes the bugs
(if they have been present) and introduces the new bugs
(where, again,
).
Tinyware's problem is a simple one. Given the original version of their software, which contains all the bugs inB, it is possible to apply a sequence of patches to the software which results in a bug- free version of the software? And if so, assuming
that every patch takes a certain time to apply, how long does the fastest sequence take?
Input
The input contains several product descriptions. Each description starts with a line containing two integersn andm, the number of bugs and patches, respectively. These values satisfy
and
.
This is followed bym lines describing the m patches in order. Each line contains an integer, the time in seconds it takes to apply the patch, and two strings ofn characters each.
The first of these strings describes the bugs that have to be present or absent before the patch can be applied. Thei-th position of that string is a ``+'' if bug
bi has to be present, a ``-'' if bugbi has to be absent, and a `` 0'' if it doesn't matter whether the bug is present or not.
The second string describes which bugs are fixed and introduced by the patch. Thei-th position of that string is a ``+'' if bug
bi is introduced by the patch, a ``-'' if bugbi is removed by the patch (if it was present), and a ``0'' if bugbi is not affected by the patch (if it was
present before, it still is, if it wasn't, is still isn't).
The input is terminated by a description starting with n = m = 0. This test case should not be processed.
Output
For each product description first output the number of the product. Then output whether there is a sequence of patches that removes all bugs from a product that has alln bugs. Note that in such a sequence a patch may be used multiple times. If thereis such a sequence, output the time taken by the fastest sequence in the format shown in the sample output. If there is no such sequence, output ``Bugs cannot be fixed.''.
Print a blank line after each test case.
Sample Input
3 3 1 000 00- 1 00- 0-+ 2 0-- -++ 4 1 7 0-0+ ---- 0 0
Sample Output
Product 1 Fastest sequence takes 8 seconds. Product 2 Bugs cannot be fixed.
题意:补丁在修补bug时,有时候会引入新的bug。假定有n(n<=20)个潜在bug和m(m<=100)个补丁,每个补丁用两个长度为n的字符串表示,其中字符串的每个位置表示一个bug。第一个串表示打补丁前的状态(“-”表示该bug必须不存在,“+”表示必须存在,“0”表示无所谓),第二个串表示打补丁后的状态(“-”表示不存在,“+”表示存在,“0”表示不变)。每个补丁都有一个执行时间,你的任务是用最少的时间把一个所有bug都存在的软件通过打补丁的方式变得没有bug。一个补丁可以打多次。
分析:最短路。把状态看做结点,状态转移看做边,然后用spfa求解即可。结点数很多,多达2^n个,并且很多状态碰不到,所以不需要先把图存好,每次取出一个结点,直接枚举m个补丁,看是否能够打得上。
注:状态可以用二进制存。题目中巧妙运用位运算。
题目链接:http://acm.hust.edu.cn/vjudge/problem/viewProblem.action?id=22169
代码清单:
#include<set> #include<map> #include<cmath> #include<queue> #include<stack> #include<ctime> #include<cctype> #include<string> #include<cstdio> #include<cstdlib> #include<cstring> #include<iostream> #include<algorithm> using namespace std; typedef long long ll; typedef unsigned int uint; typedef unsigned long long ull; const int maxs = 20 + 5; const int maxm = 100 + 5; const int maxn = (1<<20) + 5; const int max_dis = 0x7f7f7f7f; struct Patch{ int dis; char s1[maxs]; char s2[maxs]; }patch[maxm]; int n,m,cases; int dist[maxn]; bool vis[maxn]; void input(){ for(int i=1;i<=m;i++){ scanf("%d%s%s",&patch[i].dis,patch[i].s1,patch[i].s2); } } //判断该补丁是否可用 bool useful(int u,int idx){ for(int i=n-1;i>=0;i--){ int idd=(u>>(n-i-1))&1; if(patch[idx].s1[i]=='-'&&idd==1) return false; if(patch[idx].s1[i]=='+'&&idd==0) return false; }return true; } //得到打完补丁后的状态 int get_v(int u,int idx){ int vv=0; for(int i=n-1;i>=0;i--){ int idd=(u>>(n-i-1))&1; if(patch[idx].s2[i]=='0'){ if(idd==1) vv+=(1<<(n-i-1)); } if(patch[idx].s2[i]=='+'){ vv+=(1<<(n-i-1)); } }return vv; } int spfa(){ int s=(1<<n)-1; memset(vis,false,sizeof(vis)); memset(dist,max_dis,sizeof(dist)); //cout<<max_dis<<" "<<dist[0]<<endl; dist[s]=0; vis[s]=true; queue<int>que; while(!que.empty()) que.pop(); que.push(s); while(!que.empty()){ int u=que.front(); que.pop(); vis[u]=false; for(int i=1;i<=m;i++){ if(useful(u,i)){ int v=get_v(u,i); if(dist[v]>dist[u]+patch[i].dis){ dist[v]=dist[u]+patch[i].dis; if(!vis[v]){ vis[v]=true; que.push(v); } } } } } } void solve(){ spfa(); printf("Product %d\n",++cases); if(dist[0]==max_dis) printf("Bugs cannot be fixed.\n\n"); else printf("Fastest sequence takes %d seconds.\n\n",dist[0]); } int main(){ while(scanf("%d%d",&n,&m)!=EOF){ if(!n&&!m) break; input(); solve(); }return 0; }
相关文章推荐
- JavaScript数字字符转数据类型
- Delete Node in a Linked List
- 关于node 符号链接符号过多的问题
- Caffe源码(七):ReLU,Sigmoid and Tanh
- css滤镜实现页面灰色黑白色效果代码
- CSS中背景图像
- Java中的StringBuffer和数组Arrays以及常用类型的包装类
- Webfrom 生成流水号 组合查询 Repeater中单选与复选控件的使用 JS实战应用
- node Express 创建一个web应用
- JAVASCRIPT——图片滑动效果
- Jquery实现鼠标hover图片遮罩弹出提示层特效
- js 秒表计时器(开始,停止,清零)
- html5新特性总结
- CSS3 timing-function: steps()介绍
- 02---CSS整理
- Ubuntu14.04_X64 + ATI显卡 安装Caffe
- JS 实现继承静态属性
- jQuery.lazyload详解
- LeetCode(222) Count Complete Tree Nodes
- HTML5表单