用imageswitcher和gallery实现壁纸切换效果
2015-08-14 15:42
525 查看
用imageswitcher和gallery实现壁纸切换效果
先来张效果图
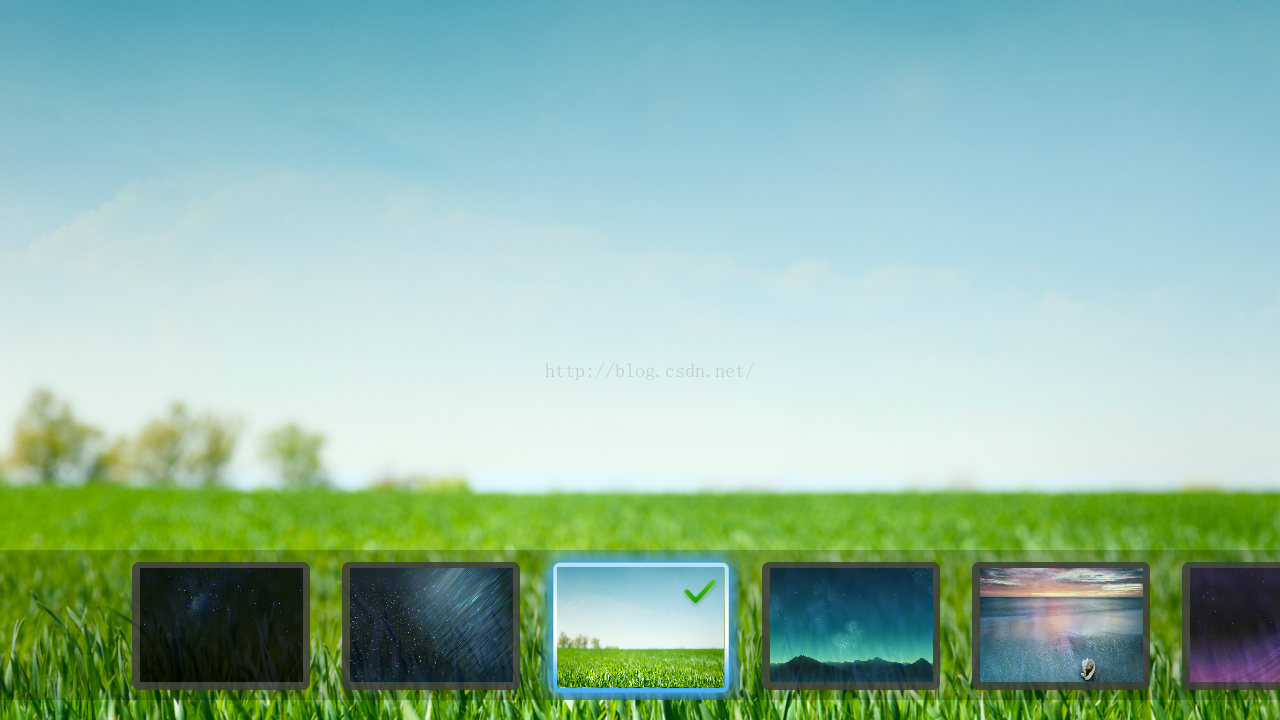
首先介绍下实现的效果:
底部图片左右滑动切换,每个item有选中效果,当item被选中后背景图跟着切换
当点击后,item上显示选择标记,并记录当前选中壁纸,当退出后,重新进入,焦点在前一次选中项上
整体思路:
1、背景用imageswitcher控件,滑动浏览图片的用gallery控件,整体用framelayout布局
2、图片源有两种模式:1.直接将图片放在drawable文件夹中,程序中用int数组就下所有图片的ID
2.从内存文件夹中获取,首先得在文件夹中扫描所有文件,将所有图片转换成bitmap格式,记录在一个
ArrayList,这样方便引用,而不用每次去内存中取
3、sharepreference记录下每次选中的结果,再次进入选中值保持为上次选中值
接下来上代码:
xml文件
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<ImageSwitcher
android:id="@+id/imgswitcher"
android:layout_width="fill_parent"
android:layout_height="fill_parent"/>
<Gallery
android:id="@+id/gallery"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_marginTop="550dp"
android:spacing="10dp"
android:background="@color/transparent_65" />
</FrameLayout>
每个gallery item布局文件
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<ImageView
android:id="@+id/imageview"
android:layout_width="fill_parent"
android:layout_height="fill_parent"/>
<ImageView
android:id="@+id/check"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/select"
android:layout_marginLeft="120dp"
/>
</FrameLayout>
activity文件
package com.lee.gallery3d;
import android.app.Activity;
import android.content.Context;
import android.content.res.TypedArray;
import android.graphics.Bitmap;
import android.graphics.drawable.BitmapDrawable;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.View.OnClickListener;
import android.view.View.OnFocusChangeListener;
import android.view.ViewGroup;
import android.view.ViewGroup.LayoutParams;
import android.view.animation.AnimationUtils;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemClickListener;
import android.widget.AdapterView.OnItemSelectedListener;
import android.widget.BaseAdapter;
import android.widget.Gallery;
import android.widget.ImageSwitcher;
import android.widget.ImageView;
import android.widget.ViewSwitcher.ViewFactory;
public class wallpaperSetActivity extends Activity implements OnItemSelectedListener,ViewFactory,OnItemClickListener{
private Gallery gallery;
private ImageSwitcher imageSwitcher;
private ImageAdapter imageAdapter;
private int[] resIds = new int[]
{ R.drawable.wallpaper1,
R.drawable.wallpaper2,
R.drawable.wallpaper3,
R.drawable.wallpaper4,
R.drawable.wallpaper5,
R.drawable.wallpaper6};
public class ImageAdapter extends BaseAdapter
{
private int select;
private int ischeck;
int mGalleryItemBackground;
private Context mContext;
private LayoutInflater mInflater;
public ImageAdapter(Context context)
{
mContext = context;
//Bitmap bmp = ((BitmapDrawable) getResources().getDrawable(R.drawable.c)).getBitmap();
TypedArray typedArray = obtainStyledAttributes(R.styleable.Gallery);
mGalleryItemBackground = typedArray.getResourceId(
R.styleable.Gallery_android_galleryItemBackground, 0);
typedArray.recycle();
mInflater = LayoutInflater.from(context);
}
// 第1点改进,返回一个很大的值,例如,Integer.MAX_VALUE
public int getCount()
{
return resIds.length;
}
public Object getItem(int position)
{
return position;
}
public long getItemId(int position)
{
return position;
}
public View getView(int position, View view, ViewGroup parent)
{
/* ImageView imageView = new ImageView(mContext);
// 第2点改进,通过取余来循环取得resIds数组中的图像资源ID
//imageView.setImageResource(resIds[position % resIds.length]);
imageView.setImageResource(resIds[position]);
imageView.setScaleType(ImageView.ScaleType.FIT_XY);
imageView.setLayoutParams(new Gallery.LayoutParams(200, 150));
if(select == position){
imageView.setBackgroundResource(R.drawable.focus);
}else{
imageView.setBackgroundResource(R.drawable.grey_focus);
}
return imageView;*/
//自定义layout
ViewHolder holder;
if(view==null){
view = mInflater.inflate(R.layout.gallery_tiem, null);
holder = new ViewHolder();
holder.imageview = (ImageView)view.findViewById(R.id.imageview);
holder.check = (ImageView)view.findViewById(R.id.check);
view.setTag(holder);
}else{
holder = (ViewHolder)view.getTag();
}
holder.imageview.setImageResource(resIds[position]);
holder.imageview.setScaleType(ImageView.ScaleType.FIT_XY);
view.setLayoutParams(new Gallery.LayoutParams(200, 150));
if(select == position){
// holder.imageview.setBackgroundResource(R.drawable.focus);
view.setBackgroundResource(R.drawable.focus);
}else{
//holder.imageview.setBackgroundResource(R.drawable.grey_focus);
view.setBackgroundResource(R.drawable.grey_focus);
}
if(ischeck==position){
holder.check.setVisibility(View.VISIBLE);
}else{
holder.check.setVisibility(View.INVISIBLE);
}
return view;
}
public class ViewHolder {
ImageView imageview;
ImageView check;
}
public void setSelectItem(int selectItem) {
if (this.select != selectItem) {
this.select = selectItem;
notifyDataSetChanged();
}
}
public void setCheckItem(int checkItem) {
if (this.ischeck!= checkItem) {
this.ischeck = checkItem;
notifyDataSetChanged();
}
}
}
@Override
public void onItemSelected(AdapterView<?> parent, View view, int position,long id)
{
// 选中Gallery中某个图像时,在ImageSwitcher组件中放大显示该图像
imageSwitcher.setImageResource(resIds[position]);
imageAdapter.setSelectItem(position);
}
@Override
public void onNothingSelected(AdapterView<?> parent)
{
}
@Override
// ImageSwitcher组件需要这个方法来创建一个View对象(一般为ImageView对象)
// 来显示图像
public View makeView()
{
ImageView imageView = new ImageView(this);
imageView.setBackgroundColor(0xFF000000);
imageView.setScaleType(ImageView.ScaleType.FIT_XY);
imageView.setLayoutParams(new ImageSwitcher.LayoutParams(
LayoutParams.FILL_PARENT, LayoutParams.FILL_PARENT));
return imageView;
}
@Override
public void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_bg_set);
gallery = (Gallery) findViewById(R.id.gallery);
imageAdapter = new ImageAdapter(this);
gallery.setAdapter(imageAdapter);
gallery.setOnItemSelectedListener(this);
gallery.setOnItemClickListener(this);
imageSwitcher = (ImageSwitcher) findViewById(R.id.imgswitcher);
// 设置ImageSwitcher组件的工厂对象
imageSwitcher.setFactory(this);
// 设置ImageSwitcher组件显示图像的动画效果
imageSwitcher.setInAnimation(AnimationUtils.loadAnimation(this,android.R.anim.fade_in));
imageSwitcher.setOutAnimation(AnimationUtils.loadAnimation(this,android.R.anim.fade_out));
}
@Override
public void onItemClick(AdapterView<?> arg0, View arg1, int position, long arg3) {
imageAdapter.setCheckItem(position);
}
}
源码地址:http://download.csdn.net/detail/u011403718/9005397
先来张效果图
首先介绍下实现的效果:
底部图片左右滑动切换,每个item有选中效果,当item被选中后背景图跟着切换
当点击后,item上显示选择标记,并记录当前选中壁纸,当退出后,重新进入,焦点在前一次选中项上
整体思路:
1、背景用imageswitcher控件,滑动浏览图片的用gallery控件,整体用framelayout布局
2、图片源有两种模式:1.直接将图片放在drawable文件夹中,程序中用int数组就下所有图片的ID
2.从内存文件夹中获取,首先得在文件夹中扫描所有文件,将所有图片转换成bitmap格式,记录在一个
ArrayList,这样方便引用,而不用每次去内存中取
3、sharepreference记录下每次选中的结果,再次进入选中值保持为上次选中值
接下来上代码:
xml文件
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<ImageSwitcher
android:id="@+id/imgswitcher"
android:layout_width="fill_parent"
android:layout_height="fill_parent"/>
<Gallery
android:id="@+id/gallery"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_marginTop="550dp"
android:spacing="10dp"
android:background="@color/transparent_65" />
</FrameLayout>
每个gallery item布局文件
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<ImageView
android:id="@+id/imageview"
android:layout_width="fill_parent"
android:layout_height="fill_parent"/>
<ImageView
android:id="@+id/check"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/select"
android:layout_marginLeft="120dp"
/>
</FrameLayout>
activity文件
package com.lee.gallery3d;
import android.app.Activity;
import android.content.Context;
import android.content.res.TypedArray;
import android.graphics.Bitmap;
import android.graphics.drawable.BitmapDrawable;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.View.OnClickListener;
import android.view.View.OnFocusChangeListener;
import android.view.ViewGroup;
import android.view.ViewGroup.LayoutParams;
import android.view.animation.AnimationUtils;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemClickListener;
import android.widget.AdapterView.OnItemSelectedListener;
import android.widget.BaseAdapter;
import android.widget.Gallery;
import android.widget.ImageSwitcher;
import android.widget.ImageView;
import android.widget.ViewSwitcher.ViewFactory;
public class wallpaperSetActivity extends Activity implements OnItemSelectedListener,ViewFactory,OnItemClickListener{
private Gallery gallery;
private ImageSwitcher imageSwitcher;
private ImageAdapter imageAdapter;
private int[] resIds = new int[]
{ R.drawable.wallpaper1,
R.drawable.wallpaper2,
R.drawable.wallpaper3,
R.drawable.wallpaper4,
R.drawable.wallpaper5,
R.drawable.wallpaper6};
public class ImageAdapter extends BaseAdapter
{
private int select;
private int ischeck;
int mGalleryItemBackground;
private Context mContext;
private LayoutInflater mInflater;
public ImageAdapter(Context context)
{
mContext = context;
//Bitmap bmp = ((BitmapDrawable) getResources().getDrawable(R.drawable.c)).getBitmap();
TypedArray typedArray = obtainStyledAttributes(R.styleable.Gallery);
mGalleryItemBackground = typedArray.getResourceId(
R.styleable.Gallery_android_galleryItemBackground, 0);
typedArray.recycle();
mInflater = LayoutInflater.from(context);
}
// 第1点改进,返回一个很大的值,例如,Integer.MAX_VALUE
public int getCount()
{
return resIds.length;
}
public Object getItem(int position)
{
return position;
}
public long getItemId(int position)
{
return position;
}
public View getView(int position, View view, ViewGroup parent)
{
/* ImageView imageView = new ImageView(mContext);
// 第2点改进,通过取余来循环取得resIds数组中的图像资源ID
//imageView.setImageResource(resIds[position % resIds.length]);
imageView.setImageResource(resIds[position]);
imageView.setScaleType(ImageView.ScaleType.FIT_XY);
imageView.setLayoutParams(new Gallery.LayoutParams(200, 150));
if(select == position){
imageView.setBackgroundResource(R.drawable.focus);
}else{
imageView.setBackgroundResource(R.drawable.grey_focus);
}
return imageView;*/
//自定义layout
ViewHolder holder;
if(view==null){
view = mInflater.inflate(R.layout.gallery_tiem, null);
holder = new ViewHolder();
holder.imageview = (ImageView)view.findViewById(R.id.imageview);
holder.check = (ImageView)view.findViewById(R.id.check);
view.setTag(holder);
}else{
holder = (ViewHolder)view.getTag();
}
holder.imageview.setImageResource(resIds[position]);
holder.imageview.setScaleType(ImageView.ScaleType.FIT_XY);
view.setLayoutParams(new Gallery.LayoutParams(200, 150));
if(select == position){
// holder.imageview.setBackgroundResource(R.drawable.focus);
view.setBackgroundResource(R.drawable.focus);
}else{
//holder.imageview.setBackgroundResource(R.drawable.grey_focus);
view.setBackgroundResource(R.drawable.grey_focus);
}
if(ischeck==position){
holder.check.setVisibility(View.VISIBLE);
}else{
holder.check.setVisibility(View.INVISIBLE);
}
return view;
}
public class ViewHolder {
ImageView imageview;
ImageView check;
}
public void setSelectItem(int selectItem) {
if (this.select != selectItem) {
this.select = selectItem;
notifyDataSetChanged();
}
}
public void setCheckItem(int checkItem) {
if (this.ischeck!= checkItem) {
this.ischeck = checkItem;
notifyDataSetChanged();
}
}
}
@Override
public void onItemSelected(AdapterView<?> parent, View view, int position,long id)
{
// 选中Gallery中某个图像时,在ImageSwitcher组件中放大显示该图像
imageSwitcher.setImageResource(resIds[position]);
imageAdapter.setSelectItem(position);
}
@Override
public void onNothingSelected(AdapterView<?> parent)
{
}
@Override
// ImageSwitcher组件需要这个方法来创建一个View对象(一般为ImageView对象)
// 来显示图像
public View makeView()
{
ImageView imageView = new ImageView(this);
imageView.setBackgroundColor(0xFF000000);
imageView.setScaleType(ImageView.ScaleType.FIT_XY);
imageView.setLayoutParams(new ImageSwitcher.LayoutParams(
LayoutParams.FILL_PARENT, LayoutParams.FILL_PARENT));
return imageView;
}
@Override
public void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_bg_set);
gallery = (Gallery) findViewById(R.id.gallery);
imageAdapter = new ImageAdapter(this);
gallery.setAdapter(imageAdapter);
gallery.setOnItemSelectedListener(this);
gallery.setOnItemClickListener(this);
imageSwitcher = (ImageSwitcher) findViewById(R.id.imgswitcher);
// 设置ImageSwitcher组件的工厂对象
imageSwitcher.setFactory(this);
// 设置ImageSwitcher组件显示图像的动画效果
imageSwitcher.setInAnimation(AnimationUtils.loadAnimation(this,android.R.anim.fade_in));
imageSwitcher.setOutAnimation(AnimationUtils.loadAnimation(this,android.R.anim.fade_out));
}
@Override
public void onItemClick(AdapterView<?> arg0, View arg1, int position, long arg3) {
imageAdapter.setCheckItem(position);
}
}
源码地址:http://download.csdn.net/detail/u011403718/9005397
相关文章推荐
- Ubuntu 默认壁纸历代记
- 使用C++实现JNI接口需要注意的事项
- Android IPC进程间通讯机制
- Android Manifest 用法
- [转载]Activity中ConfigChanges属性的用法
- Android之获取手机上的图片和视频缩略图thumbnails
- Android之使用Http协议实现文件上传功能
- Android学习笔记(二九):嵌入浏览器
- android string.xml文件中的整型和string型代替
- i-jetty环境搭配与编译
- android之定时器AlarmManager
- android wifi 无线调试
- Android Native 绘图方法
- Android java 与 javascript互访(相互调用)的方法例子
- android 代码实现控件之间的间距
- android FragmentPagerAdapter的“标准”配置
- Android"解决"onTouch和onClick的冲突问题
- android:installLocation简析
- android searchView的关闭事件