JsonModel&AFNetWorking
2015-07-21 20:47
639 查看
// HttpManager.h // JsonModel&AFNetWorking // // Created by qianfeng on 15/7/21. // Copyright (c) 2015年 张国锋. All rights reserved. // #import <Foundation/Foundation.h> #import "AFNetworking.h" typedef void (^sucessBlock)(AFHTTPRequestOperation *operation, id responseObject); typedef void (^faileBlock)(AFHTTPRequestOperation *operation, NSError *error); @interface HttpManager : NSObject + (HttpManager *)shareManager; - (void)requestWithUrl:(NSString *)url withDic:(NSDictionary *)dic WithSuccess:(sucessBlock)sucBlock WithFailure:(faileBlock) failBlock; @end // // HttpManager.m // JsonModel&AFNetWorking // // Created by qianfeng on 15/7/21. // Copyright (c) 2015年 张国锋. All rights reserved. // #import "HttpManager.h" @implementation HttpManager + (HttpManager *)shareManager { static HttpManager *manager; static dispatch_once_t once; dispatch_once(&once,^{ if(manager ==nil) { manager =[[HttpManager alloc]init]; } }); return manager; // static dispatch_once_t *once; 错误 // dispatch_once(once,^{ } - (void)requestWithUrl:(NSString *)url withDic:(NSDictionary *)dic WithSuccess:(sucessBlock)sucBlock WithFailure:(faileBlock) failBlock { AFHTTPRequestOperationManager *manager =[AFHTTPRequestOperationManager manager]; //manager.responseSerializer.acceptableContentTypes =[NSSet setWithObject:@"application/json"]; manager.responseSerializer.acceptableContentTypes =[NSSet setWithObjects:@"application/json",@"text/text", nil]; [manager POST:url parameters:dic success:^(AFHTTPRequestOperation *operation, id responseObject) { sucBlock(operation,responseObject); } failure:^(AFHTTPRequestOperation *operation, NSError *error) { NSLog(@"error:%@",error); failBlock(operation,error); }]; } @end
// // GFControl.h // JsonModel&AFNetWorking // // Created by qianfeng on 15/7/21. // Copyright (c) 2015年 张国锋. All rights reserved. // #import <Foundation/Foundation.h> #import <UIKit/UIKit.h> @interface GFControl : NSObject + (UILabel *)createLabelWithFrame:(CGRect)frame WithFont:(UIFont *)font; @end // // GFControl.m // JsonModel&AFNetWorking // // Created by qianfeng on 15/7/21. // Copyright (c) 2015年 张国锋. All rights reserved. // #import "GFControl.h" @implementation GFControl + (UILabel *)createLabelWithFrame:(CGRect)frame WithFont:(UIFont *)font { UILabel *label =[[UILabel alloc]initWithFrame:frame]; label.textColor=[UIColor blackColor]; label.font=font; label.textAlignment=NSTextAlignmentLeft; return label; } @end
// // MyModel.h // JsonModel&AFNetWorking // // Created by qianfeng on 15/7/21. // Copyright (c) 2015年 张国锋. All rights reserved. // #import "JSONModel.h" //创建的时候继承至JSONModel //#import "JSONModelLib.h" //@protocol CreateDateModel //@end @protocol ObjModel @end @interface CreateDateModel : JSONModel @property (nonatomic,strong) NSString <Optional> *date; @property (nonatomic,strong) NSString <Optional> *day; @property (nonatomic,strong) NSString <Optional> *hours; @property (nonatomic,strong) NSString <Optional> *minutes; @property (nonatomic,strong) NSString <Optional> *month; @property (nonatomic,strong) NSString <Optional> *seconds; @property (nonatomic,strong) NSString <Optional> *time; @property (nonatomic,strong) NSString <Optional> *timezoneOffset; @property (nonatomic,strong) NSString <Optional> *year; @end @interface ObjModel : JSONModel @property (nonatomic,strong) NSString <Optional> *descriptionOne; @property (nonatomic,strong) NSString <Optional> *isNew; @property (nonatomic,strong) NSString <Optional> *isRecommend; @property (nonatomic,strong) NSString <Optional> *isSubscibe; @property (nonatomic,strong) NSString <Optional> *itemID; @property (nonatomic,strong) NSString <Optional> *itemName; @property (nonatomic,strong) NSString <Optional> *picUrl; @property (nonatomic,strong) NSString <Optional> *readNum; //@property (nonatomic,strong) NSString <Optional> *numRows; //@property (nonatomic,strong) NSArray <Optional,CreateDateModel> *obj; @property (nonatomic,strong) CreateDateModel <Optional> *createDate; @end @interface MyModel : JSONModel @property (nonatomic,strong) NSString <Optional> *numRows; @property (nonatomic,strong) NSDictionary <Optional> *resultObj; @property (nonatomic,strong) NSArray <Optional,ObjModel> *obj; @end // // MyModel.m // JsonModel&AFNetWorking // // Created by qianfeng on 15/7/21. // Copyright (c) 2015年 张国锋. All rights reserved. // #import "MyModel.h" @implementation CreateDateModel @end @implementation ObjModel +(JSONKeyMapper *)keyMapper { // NSMutableDictionary *dic =[[NSMutableDictionary alloc]init]; // [dic setObject:@"description" forKey:@"description1"]; // return [[JSONKeyMapper alloc]initWithDictionary:dic]; return [[JSONKeyMapper alloc]initWithDictionary:@{@"description":@"descriptionOne"}]; } @end @implementation MyModel @end
// MyCell.h // JsonModel&AFNetWorking // // Created by qianfeng on 15/7/21. // Copyright (c) 2015年 张国锋. All rights reserved. // #import <Foundation/Foundation.h> #import <UIKit/UIKit.h> @interface MyCell : UITableViewCell @property (nonatomic,strong)UILabel *itemNameLabel; @property (nonatomic,strong)UILabel *desLabel; @property (nonatomic,strong)UILabel *timeLabel; @property (nonatomic,strong)UIImageView *psImage; @end // // MyCell.m // JsonModel&AFNetWorking // // Created by qianfeng on 15/7/21. // Copyright (c) 2015年 张国锋. All rights reserved. // #import "MyCell.h" #import "GFControl.h" @implementation MyCell - (instancetype)initWithStyle:(UITableViewCellStyle)style reuseIdentifier:(NSString *)reuseIdentifier { self=[super initWithStyle:style reuseIdentifier:reuseIdentifier]; if (self) { [self configCell]; } return self; } - (void)configCell { _psImage =[[UIImageView alloc]initWithFrame:CGRectMake(10, 10, 80, 90)]; [self addSubview:_psImage]; CGFloat witdth=[[UIScreen mainScreen] bounds].size.width; _itemNameLabel =[GFControl createLabelWithFrame:CGRectMake(110, 10, witdth-100-10, 30) WithFont:[UIFont systemFontOfSize:15]]; // NSLog(@"%@",_itemNameLabel.text); [self addSubview:_itemNameLabel]; _desLabel =[GFControl createLabelWithFrame:CGRectMake(110, 40, witdth-100-10, 30) WithFont:[UIFont systemFontOfSize:13]]; [self addSubview:_desLabel]; _timeLabel =[GFControl createLabelWithFrame:CGRectMake(110, 70, witdth-100-10, 30) WithFont:[UIFont systemFontOfSize:13]]; _timeLabel.textAlignment=NSTextAlignmentRight; [self addSubview:_timeLabel]; } @end
// ViewController.h // JsonModel&AFNetWorking // // Created by qianfeng on 15/7/21. // Copyright (c) 2015年 张国锋. All rights reserved. // #import <UIKit/UIKit.h> @interface ViewController : UIViewController @end // // ViewController.m // JsonModel&AFNetWorking // // Created by qianfeng on 15/7/21. // Copyright (c) 2015年 张国锋. All rights reserved. // #import "ViewController.h" #import "HttpManager.h" #import "MyModel.h" #import "MyCell.h" #import "UIImageView+WebCache.h" #define KPostUrl @"http://services.baidu.com/ApricotForestWirelessServiceForLiterature/LiteratureDataServlet" @interface ViewController () <UITableViewDataSource,UITableViewDelegate> { MyModel *_myModel; UITableView *_tabelView; } @end @implementation ViewController - (void)viewDidLoad { [super viewDidLoad]; // self.view.backgroundColor=[UIColor whiteColor]; // // [[HttpManager shareManager] requestWithUrl:@"" withDic:nil WithSuccess:^(AFHTTPRequestOperation *operation, id responseObject) { // // } WithFailure:^(AFHTTPRequestOperation *operation, NSError *error) { // // }]; [self configUI]; [self requestdata]; } - (void)configUI { _tabelView =[[UITableView alloc]initWithFrame:CGRectMake(0, 0, self.view.frame.size.width, self.view.frame.size.height)]; [self.view addSubview:_tabelView]; _tabelView.delegate=self; _tabelView.dataSource=self; [_tabelView registerClass:[MyCell class] forCellReuseIdentifier:@"MyCell"]; } - (void)requestdata { NSMutableDictionary *dic =[[NSMutableDictionary alloc]init]; [dic setObject:@"7B63373363386530373034366164393262633633373039326138356238366366307D2C7B336464363563386338626263653130663531623832616632343963363832323065363964656161627D2C7B66616C73657D2C7B307D2C7B307D2C7B66373539333863642D326162352D346232342D616336622D3132386538626434663366397D2C7B37363033396665333533326461623034656561353162363734643131636532617D2C7B323031352D30332D30372031313A31383A33337D2C7B312E382E367D2C7B696F736C6974657261747572657D2C7B494F536C6974657261747572655F312E382E365F696F73382E312E335F6950686F6E65352D327D" forKey:@"sessionKey"]; [dic setObject:@"10" forKey:@"pageSize"]; [dic setObject:@"2" forKey:@"rtype"]; [dic setObject:@"0" forKey:@"pageIndex"]; [dic setObject:@"getLiteraturegroupBySpecialty" forKey:@"m"]; [[HttpManager shareManager] requestWithUrl:KPostUrl withDic:dic WithSuccess:^(AFHTTPRequestOperation *operation, id responseObject) { _myModel =[[MyModel alloc] initWithDictionary:responseObject error:nil]; //NSLog(@"%@",responseObject); [_tabelView reloadData]; NSLog(@"%@",_myModel.numRows); } WithFailure:^(AFHTTPRequestOperation *operation, NSError *error) { NSLog(@"%@",error); }]; } - (CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath { return 110; } - (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section { return _myModel.obj.count; } - (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath { // MyCell *cell =[tableView dequeueReusableCellWithIdentifier:@"MyCell"]; MyCell *cell =[tableView dequeueReusableCellWithIdentifier:@"MyCell" forIndexPath:indexPath]; ObjModel *obj=_myModel.obj[indexPath.row]; [cell.psImage sd_setImageWithURL:[NSURL URLWithString:obj.picUrl]]; cell.desLabel.text =obj.descriptionOne; cell.itemNameLabel.text=obj.itemName; cell.timeLabel.text=[NSString stringWithFormat:@"%@时%@分%@秒",obj.createDate.hours,obj.createDate.minutes,obj.createDate.seconds]; return cell; } - (void)didReceiveMemoryWarning { [super didReceiveMemoryWarning]; // Dispose of any resources that can be recreated. } @end
// AppDelegate.h // JsonModel&AFNetWorking // // Created by qianfeng on 15/7/21. // Copyright (c) 2015年 张国锋. All rights reserved. // //Utility:自己封装的类 //libs:第3方库 #import <UIKit/UIKit.h> #import <CoreData/CoreData.h> @interface AppDelegate : UIResponder <UIApplicationDelegate> @property (strong, nonatomic) UIWindow *window; @property (readonly, strong, nonatomic) NSManagedObjectContext *managedObjectContext; @property (readonly, strong, nonatomic) NSManagedObjectModel *managedObjectModel; @property (readonly, strong, nonatomic) NSPersistentStoreCoordinator *persistentStoreCoordinator; - (void)saveContext; - (NSURL *)applicationDocumentsDirectory; @end // AppDelegate.m // JsonModel&AFNetWorking // // Created by qianfeng on 15/7/21. // Copyright (c) 2015年 张国锋. All rights reserved. // #import "AppDelegate.h" #import "ViewController.h" @interface AppDelegate () @end @implementation AppDelegate - (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions { // Override point for customization after application launch. CGRect frame =[[UIScreen mainScreen] bounds];//获取屏幕尺寸 self.window =[[UIWindow alloc]initWithFrame: frame]; self.window.backgroundColor =[UIColor clearColor]; [self.window makeKeyAndVisible]; //让window可见 //以上三句,创建空模板 ViewController *vc =[[ViewController alloc]init]; self.window.rootViewController=vc; return YES; }
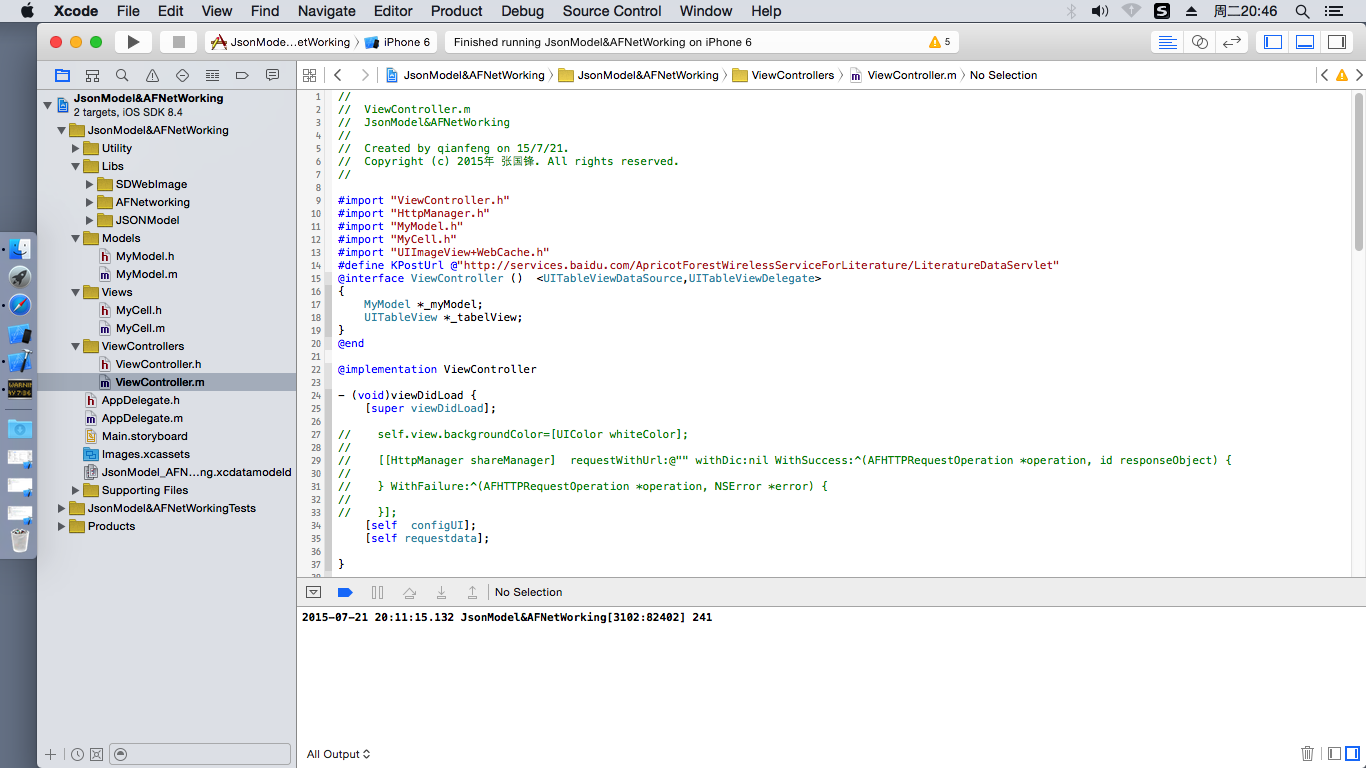
相关文章推荐
- JavaScript学习要点(五)
- 服务器采用JSON格式返回数据给安卓客户端
- JSONModel 简单例子
- js刷新窗口 (父窗口,以及点确定后的当前窗口) 一般用于页面数据刷新重新加载
- 实时显示裁剪的DEM生成的等高线
- javascript笔试面试题
- JavaScript继承方式详解
- avalon.js 多级下拉框实现
- JS的事件监听机制
- Extjs-Grid-动态生成columns
- [JSON] JSON入门指南
- maven 加入json-lib.jar 报错 Missing artifact net.sf.json-lib:json-lib:jar:2.4:compile
- js获取各种宽高方法
- Js_日期格式化
- 用UglifyJS2合并压缩混淆JS代码
- 详解js跨域问题
- javascript中的“向量”
- AgularJS中Unknown provider: $routeProvider解决方案
- javascript实现网页屏蔽Backspace事件,输入框不屏蔽
- angular js自学笔记(二)——作用域1.0