Unique Paths 算法详解
2015-06-25 15:42
423 查看
Unique Paths I
算法题目:A robot is located at the top-left corner of a m x n grid (marked ‘Start’ in the diagram below).
The robot can only move either down or right at any point in time. The robot is trying to reach the bottom-right corner of the grid (marked ‘Finish’ in the diagram below).
How many possible unique paths are there?
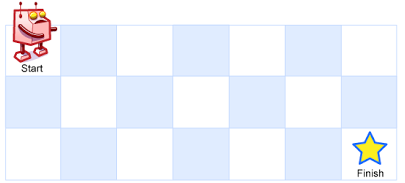
Above is a 3 x 7 grid. How many possible unique paths are there?
Note: m and n will be at most 100.
大致意思:如上图所示,robot从左上角走到右下角有多少种走法?
思路:F(i,j)=F(i-1,j)+F(i,j-1)
Unique Paths II
算法题目:Follow up for “Unique Paths”:
Now consider if some obstacles are added to the grids. How many unique paths would there be?
An obstacle and empty space is marked as 1 and 0 respectively in the grid.
For example,
There is one obstacle in the middle of a 3x3 grid as illustrated below.
[
[0,0,0],
[0,1,0],
[0,0,0]
]
The total number of unique paths is 2.
Note: m and n will be at most 100.
思路:和I思路一样,若为障碍,则相应设置F[i][j]=0;
算法题目:A robot is located at the top-left corner of a m x n grid (marked ‘Start’ in the diagram below).
The robot can only move either down or right at any point in time. The robot is trying to reach the bottom-right corner of the grid (marked ‘Finish’ in the diagram below).
How many possible unique paths are there?
Above is a 3 x 7 grid. How many possible unique paths are there?
Note: m and n will be at most 100.
大致意思:如上图所示,robot从左上角走到右下角有多少种走法?
思路:F(i,j)=F(i-1,j)+F(i,j-1)
int uniquePaths(int m, int n) { if(m<=0||n<=0)return 0; vector<vector<int>> ret(m,vector<int>(n,1)); for(int i=1;i<m;i++) { for(int j=1;j<n;j++) { ret[i][j]=ret[i][j-1]+ret[i-1][j]; } } return ret[m-1][n-1]; }
Unique Paths II
算法题目:Follow up for “Unique Paths”:
Now consider if some obstacles are added to the grids. How many unique paths would there be?
An obstacle and empty space is marked as 1 and 0 respectively in the grid.
For example,
There is one obstacle in the middle of a 3x3 grid as illustrated below.
[
[0,0,0],
[0,1,0],
[0,0,0]
]
The total number of unique paths is 2.
Note: m and n will be at most 100.
思路:和I思路一样,若为障碍,则相应设置F[i][j]=0;
int uniquePathsWithObstacles(vector<vector<int>>& obstacleGrid) { int m=obstacleGrid.size(); int n=obstacleGrid[0].size(); vector<vector<int>> ret(m,vector<int>(n,0)); for(int i=0;i<m;i++) { for(int j=0;j<n;j++) { if(obstacleGrid[i][j]) { ret[i][j]=0; } else { if(i==0&&j==0) { ret[i][j]=1; } else if(i==0&&j>0) { ret[i][j]=ret[i][j-1]; } else if(i>0&&j==0) { ret[i][j]=ret[i-1][j]; } else { ret[i][j]=ret[i-1][j]+ret[i][j-1]; } } } } return ret[m-1][n-1]; }
相关文章推荐
- 基于unique与primary约束的区别分析
- leetcode题目解答--Max Points on a Line
- java 唯一生成码
- php二维数组去重
- unique() 去重函数
- STL中transform ,erase,sort,unique的使用
- OCP-1Z0-051 补充题库 第20题 约束的注意事项
- OCP-1Z0-051 补充题库 第36题 约束
- Vertica修改带UNIQUE约束字段的长度
- BestCoder #Valentine's Day Round 1001 || hdu 5174
- STL algorithm 之 unique 函数的用法
- Java的一些高级特性(六)——Java7中的目录和文件管理
- SQLite学习笔记六: 约束NOT NULL,DEFAULT,UNIQUE,PRIMARY KEY, CHECK
- oracle_约束constraint
- 1041. Be Unique (20)
- 数据的离散化 STL用法
- STL之unique
- oracle 11g初学—sql基础(三)
- 关于EXCEL重复数据筛选
- 走火入魔.NET从C/S单点登录到B/S系统的例子,SUID(System Unique Identification)