自定义安卓的组合控件
2015-06-24 19:08
309 查看
自定义组合控件:将一些常用布局抽取出来,抽取出来后可以使用<include layout="@layout/bottom_item" />直接引用,
但是这样引用里面的属性值是静态的.我们自己定义一个view对象,并将这个布局文件加载关联进去,
这样就可以动态设置自己view布局的属性值了.
自定义组合控件和自定义控件的区别:自定义控件要重写onMeasure() onDraw(Canvas)方法,相当于自己创建一个唯一的view
而组合控件只是将系统提供的控件进行提取,组合,并向外提供一些设置每个控件的方法
例子:像系统的设置页面的条目,每个设置都是由标题,备注,单选框和一条线条组成的。
将其自定义为自己的控件自定义组合控件的过程。
效果图:
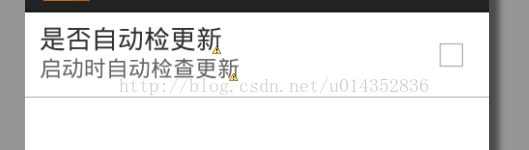
1:先创建一个自定义的布局文件
3.实现父类的构造方法。一般来说,需要在构造方法里初始化自定义的布局文件;
4.根据一些需要或者需求,定义一些API方法;
5:布局文件中使用自定定的view
以上步骤完成了自定义控件
-------------------添加自定义属性--------------------------------------
根据需要,自定义控件的属性,可以参照TextView属性;
5.要使用控件的布局文件中添加 自定义命名空间,例如:
// xmlns:itheima="http://schemas.android.com/apk/res/《包名》"
如: xmlns:zhong="http://schemas.android.com/apk/res/com.zhong.mobilephonetools"
6.自定义我们的属性,在Res/values/attrs.xml
7.使用我们自定义的属性
例如:
但是这样引用里面的属性值是静态的.我们自己定义一个view对象,并将这个布局文件加载关联进去,
这样就可以动态设置自己view布局的属性值了.
自定义组合控件和自定义控件的区别:自定义控件要重写onMeasure() onDraw(Canvas)方法,相当于自己创建一个唯一的view
而组合控件只是将系统提供的控件进行提取,组合,并向外提供一些设置每个控件的方法
例子:像系统的设置页面的条目,每个设置都是由标题,备注,单选框和一条线条组成的。
将其自定义为自己的控件自定义组合控件的过程。
效果图:
1:先创建一个自定义的布局文件
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" > <TextView android:id="@+id/tv_content_setting_item" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="10dip" android:layout_marginTop="8dip" android:text="是否自动检更新" android:textSize="18sp" /> <TextView android:textSize="15sp" android:textColor="#99000000" android:layout_marginLeft="10dip" android:id="@+id/tv_description_setting_item" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@id/tv_content_setting_item" android:text="启动时自动检查更新" /> <CheckBox android:id="@+id/cb_status" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentRight="true" android:layout_centerInParent="true" android:layout_marginRight="10dp" /> <View android:layout_width="wrap_content" android:layout_height="0.5sp" android:layout_below="@id/tv_description_setting_item" android:layout_marginTop="10dp" android:background="#55000000" /> </RelativeLayout>2.自定义一个View 一般来说,继承相对布局,或者线性布局 ViewGroup(根据布局文件的根接点);
3.实现父类的构造方法。一般来说,需要在构造方法里初始化自定义的布局文件;
4.根据一些需要或者需求,定义一些API方法;
public class SettingItemView extends RelativeLayout { private TextView tv_content; private TextView tv_description; private CheckBox cb_status; private void iniView(Context context) { View.inflate(context, R.layout.setting_item, this); tv_content = (TextView) findViewById(R.id.tv_content_setting_item); tv_description = (TextView) findViewById(R.id.tv_description_setting_item); cb_status = (CheckBox) findViewById(R.id.cb_status); } public SettingItemView(Context context, AttributeSet attrs, int defStyle) { super(context, attrs, defStyle); iniView(context); } public SettingItemView(Context context, AttributeSet attrs) { super(context, attrs); iniView(context); } public SettingItemView(Context context) { super(context); iniView(context); } //--------------------API方法; /** * 校验组合控件是否选中,单选框的状态就是这个自定义组件的状态 */ public boolean isChecked() { return cb_status.isChecked(); } /** * 设置组合控件的状态,单选框的状态就是这个自定义组件的状态 */ public void setCheck(boolean b) { cb_status.setChecked(b); } /** * 设置 组合控件的描述信息 */ public void setDescription(String description) { tv_description.setText(description); } }
5:布局文件中使用自定定的view
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="wrap_content" android:layout_height="wrap_content" > <com.zhong.mobilephonetools.ui.SettingItemView android:id="@+id/siv_update" android:layout_width="wrap_content" android:layout_height="wrap_content" > </com.zhong.mobilephonetools.ui.SettingItemView> </RelativeLayout>
以上步骤完成了自定义控件
-------------------添加自定义属性--------------------------------------
根据需要,自定义控件的属性,可以参照TextView属性;
5.要使用控件的布局文件中添加 自定义命名空间,例如:
// xmlns:itheima="http://schemas.android.com/apk/res/《包名》"
如: xmlns:zhong="http://schemas.android.com/apk/res/com.zhong.mobilephonetools"
6.自定义我们的属性,在Res/values/attrs.xml
<?xml version="1.0" encoding="utf-8"?> <resources> <declare-styleable name="TextView"> <attr name="title" format="string" /> <attr name="desc_on" format="string" /> <attr name="desc_off" format="string" /> </declare-styleable> </resources>
7.使用我们自定义的属性
例如:
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:zhong="http://schemas.android.com/apk/res/com.zhong.mobilephonetools" android:layout_width="wrap_content" android:layout_height="wrap_content" > <com.zhong.mobilephonetools.ui.SettingItemView android:id="@+id/siv_update" android:layout_width="wrap_content" android:layout_height="wrap_content" zhong:desc_off="设置自动更新已经关闭" zhong:desc_on="设置自动更新已经开启" zhong:title="设置是否自动更新" > </com.zhong.mobilephonetools.ui.SettingItemView> </RelativeLayout>8.在我们自定义控件的带有两个参数的构造方法里AttributeSet attrs 取出我们的属性值,关联自定义布局文件对应的控件;
public class SettingItemView extends RelativeLayout { private TextView tv_content; private TextView tv_description; private CheckBox cb_status; private String desc_on; private String desc_off; private void iniView(Context context) { View.inflate(context, R.layout.setting_item, this); tv_content = (TextView) findViewById(R.id.tv_content_setting_item); tv_description = (TextView) findViewById(R.id.tv_description_setting_item); cb_status = (CheckBox) findViewById(R.id.cb_status); } /***AttributeSet attrs 取出我们的属性值,关联自定义布局文件对应的控件;***/ public SettingItemView(Context context, AttributeSet attrs) { super(context, attrs); iniView(context); String namespace = "http://schemas.android.com/apk/res/com.zhong.mobilephonetools"; String title = attrs.getAttributeValue(namespace, "title"); desc_on = attrs.getAttributeValue(namespace, "desc_on"); desc_off = attrs.getAttributeValue(namespace, "desc_off"); tv_content.setText(title); } public SettingItemView(Context context, AttributeSet attrs, int defStyle) { super(context, attrs, defStyle); iniView(context); } public SettingItemView(Context context) { super(context); iniView(context); } /** * 校验组合控件是否选中,单选框的状态就是这个自定义组件的状态 */ public boolean isChecked() { return cb_status.isChecked(); } /** * 设置组合控件的状态,单选框的状态就是这个自定义组件的状态 */ public void setCheck(boolean b) { if (b) { setDescription(desc_on); } else { setDescription(desc_off); } cb_status.setChecked(b); } /** * 设置 组合控件的描述信息 */ public void setDescription(String description) { tv_description.setText(description); } }
相关文章推荐
- Java中的Object类
- 对IO的初步理解与使用
- 黑马程序员--java 类的继承
- 细谈colorPrimary用于控制ActionBar背景色的好处
- 写给开发者:记录日志的10个建议
- validation插件
- html笔记01:顺序和无序列表
- 后台自动启动appium
- sql中的group by 和 having 用法解析
- Android开发之控件属性
- 设计模式那点事--策略模式
- 解析Cron表达式
- select resharper shortcuts scheme
- Sharepoint学习笔记—Site Definition系列--9、如何在Site Definition中整合Bing Map
- leetcode-12Integer to Roman
- php(扩展到其他变成语言) self:: const static private protected public $this 函数参数访问类变量相关
- zynq虐我千百遍——第0篇 环境搭建--smb与nfs
- 22款 思维导图软件开源软件
- 一篇故事讲述了计算机网络里的基本概念:网关,DHCP,IP寻址,ARP欺骗,路由,DDOS等
- 关于腾讯应用管理中心,认领应用