C实现 LeetCode->Valid Parentheses
2015-06-17 09:51
447 查看
Given a string containing just the characters '(', ')', '{', '}', '[' and ']', determine if the input string is valid.
The brackets must close in the correct order, "()" and "()[]{}" are all valid but "(]" and "([)]" are not.
这一题是典型的使用压栈的方式解决的问题,题目中还有一种valid情况没有说明,需要我们自己考虑的,就是"({[]})"这种层层嵌套但可以完全匹配的,也是valid的一种。
解题思路:(5个case)
1:我们对字符串S中的每一个字符C,如果C是左括号,就压入栈stack中。
2:如果C是右括号,判断stack是不是空的,空则说明没有左括号,直接返回not valid
3:如果C是右括号, 并且 stack不为空,但是 左括号与 右括号不匹配 则直接返回not vaild
4:如果是匹配的,就弹出栈顶的字符,继续取S中的下一个字符;
5: 当我们遍历了一次字符串S后,注意这里还有一种情况,就是stack中还有残留的字符没有得到匹配,即此时stack不是空的,这时说明S不是valid的,因为只要valid,一定全都可以得到匹配使左括号弹出。
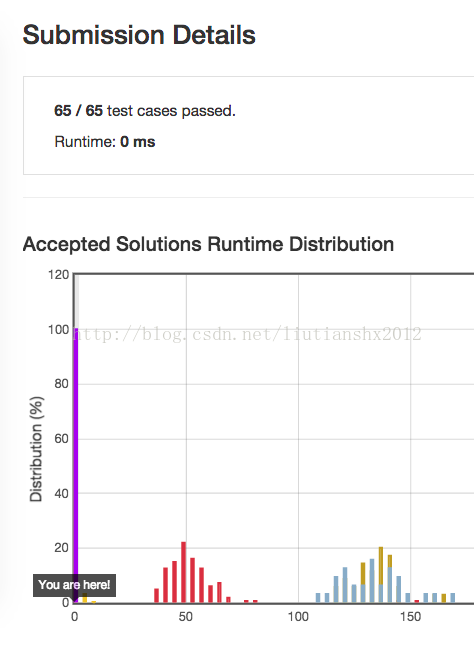
The brackets must close in the correct order, "()" and "()[]{}" are all valid but "(]" and "([)]" are not.
这一题是典型的使用压栈的方式解决的问题,题目中还有一种valid情况没有说明,需要我们自己考虑的,就是"({[]})"这种层层嵌套但可以完全匹配的,也是valid的一种。
解题思路:(5个case)
1:我们对字符串S中的每一个字符C,如果C是左括号,就压入栈stack中。
2:如果C是右括号,判断stack是不是空的,空则说明没有左括号,直接返回not valid
3:如果C是右括号, 并且 stack不为空,但是 左括号与 右括号不匹配 则直接返回not vaild
4:如果是匹配的,就弹出栈顶的字符,继续取S中的下一个字符;
5: 当我们遍历了一次字符串S后,注意这里还有一种情况,就是stack中还有残留的字符没有得到匹配,即此时stack不是空的,这时说明S不是valid的,因为只要valid,一定全都可以得到匹配使左括号弹出。
// // ValidParentheses.c // Algorithms // // Created by TTc on 15/6/17. // Copyright (c) 2015年 TTc. All rights reserved. // /** * Given a string containing just the characters '(', ')', '{', '}', '[' and ']', determine if the input string is valid. The brackets must close in the correct order, "()" and "()[]{}" are all valid but "(]" and "([)]" are not. */ #include "ValidParentheses.h" #include <stdlib.h> #include <stdbool.h> #include <string.h> bool isValid(char* s) { char *a; int top; //top of stack size_t n = strlen(s); a = (char*)malloc(sizeof(char*) * (n+1)); top = -1; int i; for( i = 0 ; i< n ; i++ ){ if (s[i]=='{' || s[i]== '[' || s[i] == '(') a[++top]=s[i]; else if (top==-1 && (s[i]=='}' || s[i]== ']' || s[i] == ')')) return 0; else if ( (s[i]==')'&& a[top]!='(') || (s[i]=='}'&& a[top]!='{') ||(s[i]==']'&&a[top]!='[') ) return 0; else if( (s[i]==')'&& a[top]=='(') || (s[i]=='}'&& a[top]=='{') || (s[i]==']'&& a[top]=='[') ) top--; } if(top == -1) return 1; else return 0; } void test_isValid(){ char *ss = "(1222,rrr(3))"; bool result = isValid(ss); printf("result ==%d",result); }
相关文章推荐
- 跳槽并不一定就是为了钱
- [ ArcGIS for Server 10.1 系列 ] - 重新创建Site
- PHP封装的HttpClient类用法实例
- Android Studio 简单介绍和使用问题小结
- Mybatis快速入门(OPT)
- 并发队列ConcurrentLinkedQueue和阻塞队列LinkedBlockingQueue
- 透过浏览器看HTTP缓存
- 将left join拆分成多条sql语句
- 同步和异步概念的解释
- #ifndef/#define/#endif使用详解
- oracle 中的 CONCAT,substring ,MINUS 用法 2009-03-25 10:45 4608人阅读 评论(0) 收藏 举报 oraclesql serversqlinterne
- SCSI驱动框架分析
- 程序员学习编程需要攻克的8个障碍
- 池化方法总结(Pooling)
- .OCX、.dll文件注册命令Regsvr32的使用
- ArcGIS JavaScript API本地部署离线开发环境[转]
- php数组全排列,元素所有组合
- android studio 更新 Gradle错误解决方法
- js判断字符串是否包含中文或英文
- 苹果Mac虚拟机装Windows哪家强?