JSP (2)内置对象
2015-06-08 11:56
741 查看
out 对象
向客户端浏览器输出各种数据
request对象
封装来自客户端浏览器的各种信息, 如cookie,客户端IP
request对象处理数组形式的表单信息
新建三个jsp文件

在register.jsp方法中,添加:
在doregister.jsp中添加
在welcome.jsp中添加
response对象
封装服务器的响应信息,用于对客户端的请求进行回应,处理http链接信息,设置http文件头,Cookie信息
用response实现页面跳转
用response访问Cookie
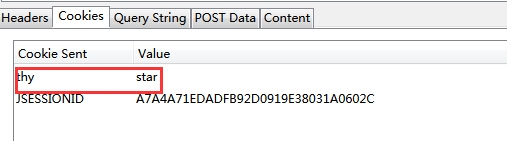
exception对象
jsp执行过程中发生的异常和错误信息
创建两个jsp文件
在throw_error.jsp中添加:
在handle.jsp中添加:
config对象
封装应用程序的配置信息
page对象
指向jsp程序本身
session对象
保存回话信息,可以在同一用户的不同请求之间共享数据
session的应用
用户登陆:
新建四个jsp文件
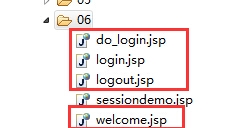
在login.jsp中添加代码:
在do_login.jsp
在welcome.jsp中添加代码
在logout.jsp中添加代码
application对象
代表当前应用程序的上下文,在不同的用户之间共享信息。相对于session对象而言,application对象的生命周期更长,为用户共享某些信息提供了方便
应用,记录页面的访问次数:
pageContext对象
提供对jsp所有对象及命名空间的访问
极客网址:http://www.jikexueyuan.com/course/520.html
向客户端浏览器输出各种数据
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ page buffer="10kb" %> <!-- 修改缓冲区大小 --> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <% out.println("thystar"); out.newLine(); out.println("123"); out.println("<br/>"); // 强制刷新服务器输出缓冲区里的数据,手动将缓冲区里的数据输出到客户端浏览器 //out.flush(); // 清空缓冲区; //out.clearBuffer(); // 清空数据,但是不能喝flash方法一起用,否则抛出异常 out.clear(); // 获取当前缓冲区的大小 out对象的默认缓冲区大小8KB out.println(out.getBufferSize()); out.println("<br/>"); // 获取当前缓冲区剩余字节的数目 out.println(out.getRemaining()); %> </body> </html>
request对象
封装来自客户端浏览器的各种信息, 如cookie,客户端IP
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <form> <input type="text" name="userName"/> <input type="submit" name="submit"/> </form> <!-- 获取请求的方法名 --> <%= request.getMethod()%><br/> <!-- 获取请求的URI字符串 --> <%= request.getRequestURI() %><br/> <!-- 获取请求时的协议 --> <%= request.getProtocol() %><br/> <!-- 获取请求的服务器IP --> <%= request.getServerName() %><br/> <!-- 获取请求服务器的端口号 --> <%= request.getServerPort() %><br/> <!-- 获取客户端IP --> <%= request.getRemoteAddr() %><br/> <!-- 获取客户端主机名 --> <%= request.getRemoteHost() %><br/> <!-- 获取提交表单的值 --> <%= request.getParameter("userName") %><br/> </body> </html>
request对象处理数组形式的表单信息
新建三个jsp文件

在register.jsp方法中,添加:
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>录入注册信息</title> </head> <body> <form action="doregister.jsp" method="post"> 用户名: <input type="text" name="userName"/><br/> 技能: <input type="checkbox" name="skills" value="java"/>java <input type="checkbox" name="skills" value="C"/>C <input type="checkbox" name="skills" value="python"/>python <input type="checkbox" name="skills" value="R"/>r <br/> <input type="submit" name="提交"/> <input type="reset" name="重置"/> </form> </body> </html>
在doregister.jsp中添加
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <% //getParameter方法用于获取客户端浏览器通过“GET”或“POST”方法获取的表单数据,没有setParameter这个方法 String userName = request.getParameter("userName"); String skills = ""; String[] skillArr = request.getParameterValues("skills"); if(skillArr != null && skillArr.length > 0){ for(String skill : skillArr){ skills = skills + skill + " "; } } //getAttribute和setAttribute用于在web组件之间共享信息 request.setAttribute("userName", userName); request.setAttribute("skills", skills); %> <jsp:forward page="welcome.jsp"></jsp:forward>
在welcome.jsp中添加
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> 用户名:<%= request.getAttribute("userName") %> 技能:<%= request.getAttribute("skills") %> </body> </html>
response对象
封装服务器的响应信息,用于对客户端的请求进行回应,处理http链接信息,设置http文件头,Cookie信息
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <% //设置头信息,Cache-Control用于设置缓存策略,no_cache表示数据内容不会被存储 response.setHeader("Cache-Control", "no_cache"); //设置整型的头信息, 网页每个2s刷新一次 response.setIntHeader("Refresh", 2); //日期类型的头信息 out.println(new java.util.Date().toString() + "<br/>"); %> </body> </html>
用response实现页面跳转
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <% //页面跳转 response.sendRedirect("http://www.baidu.com"); %>
用response访问Cookie
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <% //修改Cookie, cookie是"小甜品",其实是一段纯文本信息,由服务器发送到浏览器 // 当浏览器再次访问该网站的时候,浏览器会把请求的网址连同该Cookie一起提交给服务器 // 服务器检查Cookie用于识别用户状态 Cookie myCookie = new Cookie("thy", "star"); myCookie.setMaxAge(3600); response.addCookie(myCookie); %> </body> </html>
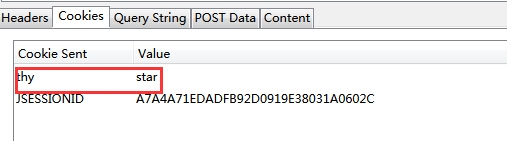
exception对象
jsp执行过程中发生的异常和错误信息
创建两个jsp文件
在throw_error.jsp中添加:
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ page errorPage="handle_error.jsp" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <% int a = 1/0; %> </body> </html>
在handle.jsp中添加:
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ page isErrorPage="true" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <% out.println(exception.getMessage()); //异常描述信息 %> <br/> <% out.println(exception.toString());//exception对象的字符串描述 %> <br/> <% exception.printStackTrace();//打印异常的堆栈轨迹,向标准输出流打印,不会输出在页面上 %> </body> </html>
config对象
封装应用程序的配置信息
page对象
指向jsp程序本身
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <% out.println(page.toString());//page对象的字符串 page.getClass(); //返回当前的object类 page.hashCode();//对象的HashCode值 //page.equals(obj);//对象是否与指定对象相等 %> </body> </html>
session对象
保存回话信息,可以在同一用户的不同请求之间共享数据
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <!-- session的唯一标识符 --> <%= session.getId() %><br/> <!-- session的创建时间 --> <%= session.getCreationTime() %><br/> <!-- session的最后访问时间 --> <%= session.getLastAccessedTime() %><br/> <!-- session的失效时间 --> <%= session.getMaxInactiveInterval() %><br/> <% %> </body> </html>
session的应用
用户登陆:
新建四个jsp文件
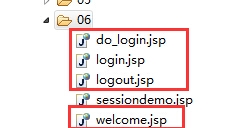
在login.jsp中添加代码:
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>登陆页面</title> </head> <body> <form action="do_login.jsp" method="post"> userName: <input type="text" name="userName"/><br/> password: <input type="password" name="password"/><br/> <input type="submit" value="submit"/> <input type="reset" value="reset"/> </form> </body> </html>
在do_login.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <% String userName = request.getParameter("userName"); String password = request.getParameter("password"); if(userName != null && password != null){ session.setAttribute("userName", userName); response.setHeader("refresh", "2;URL=welcome.jsp"); } %>
在welcome.jsp中添加代码
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>欢迎页面</title> </head> <body> <%if(session.getAttribute("userName") != null) {%> 欢迎<%=session.getAttribute("userName") %> <a href="logout.jsp">注销</a> <br/> <%}else{ %> 请先登陆 <a href="login.jsp">登陆</a> <%} %> <%if(session.isNew()){ %> <br/> 欢迎新用户! <%}else{ %> 欢迎老用户 <%} %> </body> </html>
在logout.jsp中添加代码
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <% session.invalidate();//清楚session对象 response.setHeader("refresh", "2;URL=welcome.jsp"); %>
application对象
代表当前应用程序的上下文,在不同的用户之间共享信息。相对于session对象而言,application对象的生命周期更长,为用户共享某些信息提供了方便
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <!-- 返回当前服务 器的信息 --> <%=application.getServerInfo() %><br/> <!-- 返回当前应用名称 --> <%=application.getServletContextName() %><br/> </body> </html>
应用,记录页面的访问次数:
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <% Object obj = application.getAttribute("counter"); if(obj==null){ application.setAttribute("counter", new Integer(1)); out.println("访问1次"); }else{ int counterValue=Integer.parseInt(obj.toString()); counterValue++; out.print(counterValue+"<br/>"); application.setAttribute("counter", counterValue); } %> </body> </html>
pageContext对象
提供对jsp所有对象及命名空间的访问
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <% //JspWriter myOut = pageContext.getOut(); //myOut.println("hello world"); pageContext.setAttribute("thy", "star", pageContext.SESSION_SCOPE); String value = session.getAttribute("thy").toString(); out.println(value); out.println("<br/>"); %> </body> </html>
极客网址:http://www.jikexueyuan.com/course/520.html
相关文章推荐
- java对世界各个时区(TimeZone)的通用转换处理方法(转载)
- java-注解annotation
- java-模拟tomcat服务器
- java-用HttpURLConnection发送Http请求.
- java-WEB中的监听器Lisener
- Android IPC进程间通讯机制
- Android Native 绘图方法
- Android java 与 javascript互访(相互调用)的方法例子
- GUI - Web前端开发框架
- 介绍一款信息管理系统的开源框架---jeecg
- Extjs4.0 最新最全视频教程
- 聚类算法之kmeans算法java版本
- java实现 PageRank算法
- PropertyChangeListener简单理解
- 插入排序
- 冒泡排序
- 堆排序