jquery通过ajax在jsp中局部刷新页面
2015-06-01 18:23
656 查看
在jquery中ajax的调用已经非常方便了。也提供了一些新的调用方式。
这里有两个 在jsp中局部刷新页面 的例子。
一种是json返回内容再拼接 html
一种是直接返回 一个jsp 到另一个jsp中
这里的框架用的是springMVC
其它框架 类推
IndexController.java
content.jsp
IndexController.java
content.jsp
IndexController.java
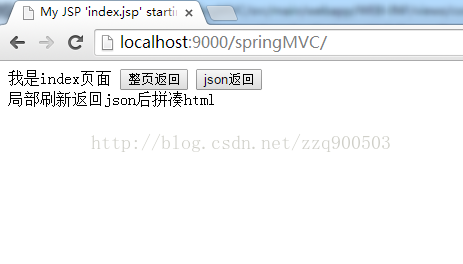
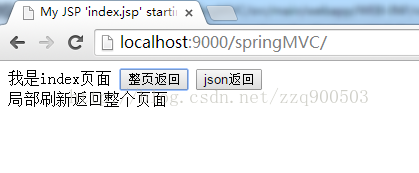
这里有两个 在jsp中局部刷新页面 的例子。
一种是json返回内容再拼接 html
一种是直接返回 一个jsp 到另一个jsp中
这里的框架用的是springMVC
其它框架 类推
json返回内容再拼接 html
index.jsp页面<div id="showdiv"> </div> </body> <script type="text/javascript"> function jsonhtml() { var successUUID = function(data){ if(data.data.detailOk=='ok'){ document.getElementById("showdiv").innerHTML =data.data.content; } }; $.ajax({ dataType: "json", url: "showcontentjson", success: successUUID, cache:false }); } </script>
IndexController.java
public class IndexController { @RequestMapping("/showcontentjson") @ResponseBody public Object contentjson() { JSONObject json = new JSONObject(); json.put("content", "局部刷新返回json后拼凑html"); String detailOk = "ok"; json.put("detailOk", detailOk); return JSONResult.success(json); } }
直接返回一个jsp到另一个jsp中
index.jsp<div id="showdiv"> </div> </body> <script type="text/javascript"> function allhtml() { document.getElementById("showdiv").innerHTML =""; $.ajax({ type: 'post', //可选get url: 'showcontent', //这里是接收数据的程序 data: 'data=2', //传给后台的数据,多个参数用&连接 dataType: 'html', //服务器返回的数据类型 可选XML ,Json jsonp script html text等 success: function(msg) { //这里是ajax提交成功后,程序返回的数据处理函数。msg是返回的数据,数据类型在dataType参数里定义! document.getElementById("showdiv").innerHTML = msg; //$("#duoduo").innerHTML = msg; }, error: function() { alert('对不起失败了'); } }) } </script>
content.jsp
<%@ page language="java" import="java.util.*" pageEncoding="utf-8"%> <% String path = request.getContextPath(); String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/"; %> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <base href="<%=basePath%>"> <title>My JSP 'content.jsp' starting page</title> <meta http-equiv="pragma" content="no-cache"> <meta http-equiv="cache-control" content="no-cache"> <meta http-equiv="expires" content="0"> <meta http-equiv="keywords" content="keyword1,keyword2,keyword3"> <meta http-equiv="description" content="This is my page"> <!-- <link rel="stylesheet" type="text/css" href="styles.css"> --> </head> <body>${content} </body> </html>
IndexController.java
public class IndexController { @RequestMapping("/showcontent") public String content(Model model) throws IOException { model.addAttribute("content", "局部刷新返回整个页面"); return "/content"; } @RequestMapping("/") public String index(Model model) throws IOException { return "/index"; }
完整例子
index.jsp<%@ page language="java" import="java.util.*" pageEncoding="utf-8"%> <% String path = request.getContextPath(); String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/"; %> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <base href="<%=basePath%>"> <title>My JSP 'index.jsp' starting page</title> <meta http-equiv="pragma" content="no-cache"> <meta http-equiv="cache-control" content="no-cache"> <meta http-equiv="expires" content="0"> <meta http-equiv="keywords" content="keyword1,keyword2,keyword3"> <meta http-equiv="description" content="This is my page"> <!-- <link rel="stylesheet" type="text/css" href="styles.css"> --> <script type="text/javascript" src="<%=basePath%>/res/plugin/jquery.min.js"></script></head> <body > 我是index页面 <input type="button" onclick="allhtml()" value="整页返回"> <input type="button" onclick="jsonhtml()" value="json返回"> <div id="showdiv"> </div> </body> <script type="text/javascript"> function allhtml() { document.getElementById("showdiv").innerHTML =""; $.ajax({ type: 'post', //可选get url: 'showcontent', //这里是接收数据的程序 data: 'data=2', //传给后台的数据,多个参数用&连接 dataType: 'html', //服务器返回的数据类型 可选XML ,Json jsonp script html text等 success: function(msg) { //这里是ajax提交成功后,程序返回的数据处理函数。msg是返回的数据,数据类型在dataType参数里定义! document.getElementById("showdiv").innerHTML = msg; //$("#duoduo").innerHTML = msg; }, error: function() { alert('对不起失败了'); } }) } function jsonhtml() { var successUUID = function(data){ if(data.data.detailOk=='ok'){ document.getElementById("showdiv").innerHTML =data.data.content; } }; $.ajax({ dataType: "json", url: "showcontentjson", success: successUUID, cache:false }); } </script> </html>
content.jsp
<%@ page language="java" import="java.util.*" pageEncoding="utf-8"%> <% String path = request.getContextPath(); String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/"; %> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <base href="<%=basePath%>"> <title>My JSP 'content.jsp' starting page</title> <meta http-equiv="pragma" content="no-cache"> <meta http-equiv="cache-control" content="no-cache"> <meta http-equiv="expires" content="0"> <meta http-equiv="keywords" content="keyword1,keyword2,keyword3"> <meta http-equiv="description" content="This is my page"> <!-- <link rel="stylesheet" type="text/css" href="styles.css"> --> </head> <body>${content} </body> </html>
IndexController.java
package com.mofang.web.controller; import java.io.IOException; import org.apache.shiro.SecurityUtils; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.ResponseBody; import com.alibaba.fastjson.JSONObject; import com.mofang.web.message.response.JSONResult; /** * IndexController * * */ @Controller public class IndexController { @RequestMapping("/showcontentjson") @ResponseBody public Object contentjson() { JSONObject json = new JSONObject(); json.put("content", "局部刷新返回json后拼凑html"); String detailOk = "ok"; json.put("detailOk", detailOk); return JSONResult.success(json); } @RequestMapping("/showcontent") public String content(Model model) throws IOException { model.addAttribute("content", "局部刷新返回整个页面"); return "/content"; } @RequestMapping("/") public String index(Model model) throws IOException { return "/index"; } }
结果图
例子下载
springMVC框架相关文章推荐
- jquery通过ajax在jsp中局部刷新页面
- Jquery autocomplete插件的使用
- jquery实现checkbox全选,反选,取消选择,对象存在
- Flexslider图片轮播、文字图片相结合滑动切换效果HTML 首先在页面head部位载入jquery库文件和Flexslider插件,以及Flexslider所需的基本css样式文件。
- jquery 增加 tr 增加 li
- 表单验证信息jQuery代码
- Jquery 常用方法经典总结
- jquery trigger 用法实例
- jquery select 上移下移
- JQuery实现商品列表
- jQuery Mobile和jQuery的使用简记
- jquery正则表达式匹配
- jQuery插件制作之参数用法实例分析
- JQuery动态添加Select的Option元素
- jQuery(htmlString) versus jQuery(selectorString)
- jquery中判断选择器,找没找到元素用$().size()==0
- jquery里面.length和.size()有什么区别(转)
- trick:CSS 3+checkbox实现JQuery的6个基本动画效果
- 可将canvas图像导出为多种格式图片的jQuery插件
- jQuery里的mouseover与mouseenter事件类型区别