hdoj 1541 Stars 【树状数组 线段树】【单点更新 区间求和】
2015-05-17 22:13
423 查看
Stars
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)Total Submission(s): 5640 Accepted Submission(s): 2235
Problem Description
Astronomers often examine star maps where stars are represented by points on a plane and each star has Cartesian coordinates. Let the level of a star be an amount of the stars that are not higher and not to the right of the given
star. Astronomers want to know the distribution of the levels of the stars.
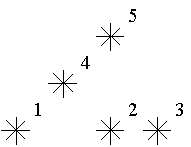
For example, look at the map shown on the figure above. Level of the star number 5 is equal to 3 (it's formed by three stars with a numbers 1, 2 and 4). And the levels of the stars numbered by 2 and 4 are 1. At this map there are only one star of the level
0, two stars of the level 1, one star of the level 2, and one star of the level 3.
You are to write a program that will count the amounts of the stars of each level on a given map.
Input
The first line of the input file contains a number of stars N (1<=N<=15000). The following N lines describe coordinates of stars (two integers X and Y per line separated by a space, 0<=X,Y<=32000). There can be only one star at one
point of the plane. Stars are listed in ascending order of Y coordinate. Stars with equal Y coordinates are listed in ascending order of X coordinate.
Output
The output should contain N lines, one number per line. The first line contains amount of stars of the level 0, the second does amount of stars of the level 1 and so on, the last line contains amount of stars of the level N-1.
Sample Input
5 1 1 5 1 7 1 3 3 5 5
Sample Output
1 2 1 1 0
题目大意:有n个星星,每一个星星都有自己的坐标。我们定义一个星星的等级为 该星星左下方所有的星星数目(和它有相同x坐标但是比它低的也算)。
然后输出0——n-1个等级所对应的星星数目(比如说等级为0的星星有两个,那么输出等级0时就输出2)。
是不是很难? 其实不然,因为星星的插入过程给了我们很好的提示:按y坐标从小到大插入,若星星有相同的y坐标,按x坐标从小到大插入。
这样就保证了我们在求当前星星的等级时,不用考虑其他未插入的星星,因为那些没有插入的星星要么在它上方,要么在它右方,不会影响该星星的等级。
所以问题就转化成了 一个二叉树的单点更新 区间求和问题。
树状数组:62ms
#include <cstdio> #include <cstring> #include <algorithm> #define MAX 32000+1 using namespace std; int c[MAX<<2]; int rec[15000];//记录等级对应的星星数目 int lowbit(int x) { return x&(-x); } void update(int x) { while(x <= MAX) { c[x] += 1; x += lowbit(x); } } int sum(int x) { int s = 0; while(x > 0) { s += c[x]; x -= lowbit(x); } return s; } int main() { int n, i; int x, y; int level; while(~scanf("%d", &n)) { memset(c, 0, sizeof(c)); memset(rec, 0, sizeof(rec)); for(i = 0; i < n; i++) { scanf("%d%d", &x, &y); level = sum(x+1);//插入当前星星时记录其等级 也是数组所对应的下标 rec[level]++;// 自增一 update(x+1);//让坐标从1开始更新 } for(i = 0; i < n; i++) printf("%d\n", rec[i]); } return 0; }
线段树:109ms
#include <cstdio> #include <cstring> #include <algorithm> #define MAX 32000+1 using namespace std; int sum[MAX<<2]; int rec[15000];//记录等级对应的星星数目 void PushUp(int o) { sum[o] = sum[o<<1] + sum[o<<1|1]; } void build(int o, int l, int r) { sum[o] = 0; if(l == r) return ; int mid = (l+r) >> 1; build(o<<1, l, mid); build(o<<1|1, mid+1, r); PushUp(o); } void update(int o, int l, int r, int L) { if(l == r) { sum[o] += 1; return ; } int mid = (l+r) >> 1; if(L <= mid) update(o<<1, l, mid, L); else update(o<<1|1, mid+1, r, L); PushUp(o); } int query(int o, int l, int r, int L, int R) { if(L <= l && R >= r) { return sum[o]; } int mid = (l+r) >> 1; int res = 0; if(L <= mid) res += query(o<<1, l ,mid, L, R); if(R > mid) res += query(o<<1|1, mid+1, r, L, R); return res; } int main() { int n, x, y; int i, j; int level; while(~scanf("%d", &n)) { build(1, 1, MAX); memset(rec, 0, sizeof(rec)); for(i = 0; i < n; i++) { scanf("%d%d", &x, &y); level = query(1, 1, MAX, 1, x+1); rec[level]++; update(1, 1, MAX, x+1); } for(i = 0; i < n; i++) printf("%d\n", rec[i]); } return 0; }
相关文章推荐
- 【HDU - 1541 - Stars 【 区间求和 单点更新 ==树状数组】
- hdoj 1166 敌兵布阵 【单点更新+区间求和】 【线段树】 【树状数组】
- Hdu 1166 敌兵布阵 树状数组 或 线段树 单点更新,区间求和
- 【树状数组--单点更新区间求和】 hdu1541 Stars
- HDOJ题目1541 Stars(树状数组单点更新)
- 线段树(单点更新)/树状数组 HDOJ 1166 敌兵布阵
- hdoj 3874 —— 树状数组单点修改+区间求和
- poj1195Mobile phones【二维树状数组。单点更新/区间求和】
- scu 2057 树状数组 单点更新区间求和问题
- 敌兵布阵------树状数组(单点更新与区间求和)
- poj-3468 线段树和树状数组的区间更新及求和
- POJ 2352 Stars(树状数组||线段树单点更新)
- NBOJv2 1050 Just Go(线段树/树状数组区间更新单点查询)
- hdu 1541 Stars(线段树单点更新,区间查询)
- NYOJ 116 树状数组 基本用法(单点更新,区间求和)
- hdu4893 Wow! Such Sequence!,树状数组,线段树,单点修改,区间更新
- POJ 2352 Stars + HDU 1556 Color the ball(树状数组单点更新及伪区间更新)
- POJ - 2155 Matrix (二维树状数组 + 区间修改 + 单点求值 或者 二维线段树 + 区间更新 + 单点求值)
- HDOJ-1556(线段树||树状数组,区间更新+点查询)
- HDU1556:Color the ball(线段树区间更新单点求值)&&树状数组解法