Android异步处理二:AsynTask介绍和使用AsyncTask异步更新UI界面
2015-04-25 13:14
501 查看
在上一篇(http://blog.csdn.net/xlgen157387/article/details/45269389)中介绍了使用Thread+Handler实现非UI线程更新UI界面的方法步骤,下边做一下如何同构AsyncTask异步任务来更新UI界面。
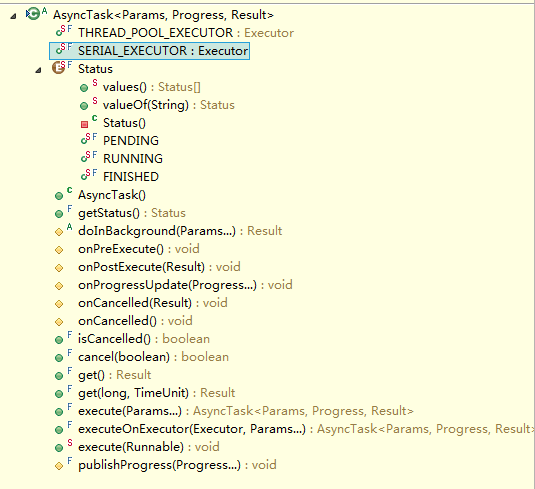
通过上图中的AsyncTask的源码结构图可以看到,主要用于重载的方法是doInBackground(),onPreExecute()、onPostExecute()、onProgressUpdate()、onCancelled()、publishProgress()等前边是”黄色的菱形“图标的,下边详细介绍这些方法的功能:
AsyncTask的构造函数有三个模板参数:
1.Params,传递给后台任务的参数类型。
2.Progress,后台计算执行过程中,进步单位(progress units)的类型。(就是后台程序已经执行了百分之几了。)
3.Result, 后台执行返回的结果的类型。
AsyncTask并不总是需要使用上面的全部3种类型。标识不使用的类型很简单,只需要使用Void类型即可。
正在后台运行:doInBackground(Params…),该回调函数由后台线程在onPreExecute()方法执行结束后立即调用。通常在这里执行耗时的后台计算。计算的结果必须由该函数返回,并被传递到onPostExecute()中。在该函数内也可以使用publishProgress(Progress…)来发布一个或多个进度单位(unitsof progress)。这些值将会在onProgressUpdate(Progress…)中被发布到UI线程。
准备运行:onPreExecute(),该回调函数在任务被执行之后立即由UI线程调用。这个步骤通常用来建立任务,在用户接口(UI)上显示进度条。
完成后台任务:onPostExecute(Result),当后台计算结束后调用。后台计算的结果会被作为参数传递给这一函数。
完成后台任务:onPostExecute(Result),当后台计算结束后调用。后台计算的结果会被作为参数传递给这一函数。
主要用于显示进度。
布局文件:
同样需要开启访问网络的权限和设置启动界面
另外我们使用AsynTask的时候,需要注意的是
(1)AsyncTask的介绍
通过上图中的AsyncTask的源码结构图可以看到,主要用于重载的方法是doInBackground(),onPreExecute()、onPostExecute()、onProgressUpdate()、onCancelled()、publishProgress()等前边是”黄色的菱形“图标的,下边详细介绍这些方法的功能:
AsyncTask的构造函数有三个模板参数:
1.Params,传递给后台任务的参数类型。
2.Progress,后台计算执行过程中,进步单位(progress units)的类型。(就是后台程序已经执行了百分之几了。)
3.Result, 后台执行返回的结果的类型。
AsyncTask并不总是需要使用上面的全部3种类型。标识不使用的类型很简单,只需要使用Void类型即可。
doInBackground(Params…)
源码注释:/** * Override this method to perform a computation on a background thread. The * specified parameters are the parameters passed to {@link #execute} * by the caller of this task. * * This method can call {@link #publishProgress} to publish updates * on the UI thread. * * @param params The parameters of the task. * * @return A result, defined by the subclass of this task. * * @see #onPreExecute() * @see #onPostExecute * @see #publishProgress */ protected abstract Result doInBackground(Params... params);
正在后台运行:doInBackground(Params…),该回调函数由后台线程在onPreExecute()方法执行结束后立即调用。通常在这里执行耗时的后台计算。计算的结果必须由该函数返回,并被传递到onPostExecute()中。在该函数内也可以使用publishProgress(Progress…)来发布一个或多个进度单位(unitsof progress)。这些值将会在onProgressUpdate(Progress…)中被发布到UI线程。
onPreExecute()
/** * Runs on the UI thread before {@link #doInBackground}. * * @see #onPostExecute * @see #doInBackground */ protected void onPreExecute() { }
准备运行:onPreExecute(),该回调函数在任务被执行之后立即由UI线程调用。这个步骤通常用来建立任务,在用户接口(UI)上显示进度条。
onPostExecute()
/** * <p>Runs on the UI thread after {@link #doInBackground}. The * specified result is the value returned by {@link #doInBackground}.</p> * * <p>This method won't be invoked if the task was cancelled.</p> * * @param result The result of the operation computed by {@link #doInBackground}. * * @see #onPreExecute * @see #doInBackground * @see #onCancelled(Object) */ @SuppressWarnings({"UnusedDeclaration"}) protected void onPostExecute(Result result) { }
完成后台任务:onPostExecute(Result),当后台计算结束后调用。后台计算的结果会被作为参数传递给这一函数。
onProgressUpdate()
/** * Runs on the UI thread after {@link #publishProgress} is invoked. * The specified values are the values passed to {@link #publishProgress}. * * @param values The values indicating progress. * * @see #publishProgress * @see #doInBackground */ @SuppressWarnings({"UnusedDeclaration"}) protected void onProgressUpdate(Progress... values) { }
完成后台任务:onPostExecute(Result),当后台计算结束后调用。后台计算的结果会被作为参数传递给这一函数。
onCancelled()
取消任务:onCancelled (),在调用AsyncTask的cancel()方法时调用publishProgress()
/** * This method can be invoked from {@link #doInBackground} to * publish updates on the UI thread while the background computation is * still running. Each call to this method will trigger the execution of * {@link #onProgressUpdate} on the UI thread. * * {@link #onProgressUpdate} will note be called if the task has been * canceled. * * @param values The progress values to update the UI with. * * @see #onProgressUpdate * @see #doInBackground */ protected final void publishProgress(Progress... values) { if (!isCancelled()) { sHandler.obtainMessage(MESSAGE_POST_PROGRESS, new AsyncTaskResult<Progress>(this, values)).sendToTarget(); } }
主要用于显示进度。
(2)使用AsyncTask异步更新UI界面
AsyncTaskActivity .java作为启动页面的文件public class AsyncTaskActivity extends Activity { private ImageView mImageView; private Button mButton; private ProgressBar mProgressBar; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.asyntask_main); mImageView= (ImageView) findViewById(R.id.imageView); mButton = (Button) findViewById(R.id.button); mProgressBar = (ProgressBar) findViewById(R.id.progressBar); mButton.setOnClickListener(new OnClickListener() { public void onClick(View v) { GetImageTask task = new GetImageTask(); task.execute("http://g.hiphotos.baidu.com/image/pic/item/b3119313b07eca80d340a3e0932397dda1448374.jpg"); } }); } class GetImageTask extends AsyncTask<String,Integer,Bitmap> {//继承AsyncTask @Override protected Bitmap doInBackground(String... params) {//处理后台执行的任务,在后台线程执行 publishProgress(0);//将会调用onProgressUpdate(Integer... progress)方法 HttpClient httpClient = new DefaultHttpClient(); publishProgress(30); HttpGet httpGet = new HttpGet(params[0]);//获取csdn的logo final Bitmap bitmap; try { HttpResponse httpResponse = httpClient.execute(httpGet); bitmap = BitmapFactory.decodeStream(httpResponse.getEntity().getContent()); } catch (Exception e) { return null; } publishProgress(100); //mImageView.setImageBitmap(result); 不能在后台线程操作ui return bitmap; } protected void onProgressUpdate(Integer... progress) {//在调用publishProgress之后被调用,在ui线程执行 mProgressBar.setProgress(progress[0]);//更新进度条的进度 } protected void onPostExecute(Bitmap result) {//后台任务执行完之后被调用,在ui线程执行 if(result != null) { Toast.makeText(AsyncTaskActivity.this, "成功获取图片", Toast.LENGTH_LONG).show(); mImageView.setImageBitmap(result); }else { Toast.makeText(AsyncTaskActivity.this, "获取图片失败", Toast.LENGTH_LONG).show(); } } protected void onPreExecute () {//在 doInBackground(Params...)之前被调用,在ui线程执行 mImageView.setImageBitmap(null); mProgressBar.setProgress(0);//进度条复位 } protected void onCancelled () {//在ui线程执行 mProgressBar.setProgress(0);//进度条复位 } } }
布局文件:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <ProgressBar android:id="@+id/progressBar" style="?android:attr/progressBarStyleHorizontal" android:layout_width="fill_parent" android:layout_height="wrap_content" > </ProgressBar> <Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="下载图片" > </Button> <ImageView android:id="@+id/imageView" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </LinearLayout>
同样需要开启访问网络的权限和设置启动界面
另外我们使用AsynTask的时候,需要注意的是
相关文章推荐
- Android异步处理二:AsynTask介绍和使用AsyncTask异步更新UI界面
- Android异步处理二:使用AsyncTask异步更新UI界面
- Android异步处理二:使用AsyncTask异步更新UI界面
- Android异步处理二:使用AsyncTask异步更新UI界面
- Android异步处理二:使用AsyncTask异步更新UI界面
- Android异步处理二:使用AsyncTask异步更新UI界面
- Android异步处理二:使用AsyncTask异步更新UI界面
- Android异步处理二:使用AsyncTask异步更新UI界面 .
- Android异步处理二:使用AsyncTask异步更新UI界面
- Android异步处理二:使用AsyncTask异步更新UI界面
- Android异步处理二:使用AsyncTask异步更新UI界面
- Android异步处理二:使用AsyncTask异步更新UI界面
- Android异步处理二:使用AsyncTask异步更新UI界面
- Android异步处理二:使用AsyncTask异步更新UI界面
- Android异步处理二:使用AsyncTask异步更新UI界面
- Android异步处理二:使用AsyncTask异步更新UI界面
- Android异步处理二:使用AsyncTask异步更新UI界面
- Android异步处理二:使用AsyncTask异步更新UI界面 .
- Android异步处理二:使用AsyncTask异步更新UI界面
- Android异步处理二:使用AsyncTask异步更新UI界面