Android程序:进度条(ProgressBar)的使用方法和案例
2015-04-23 18:32
423 查看
基本用法:
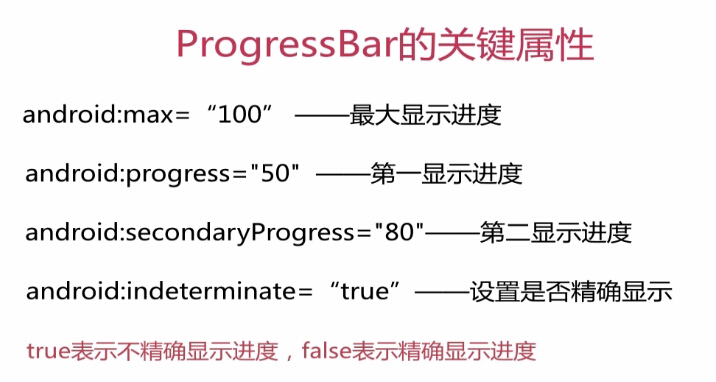
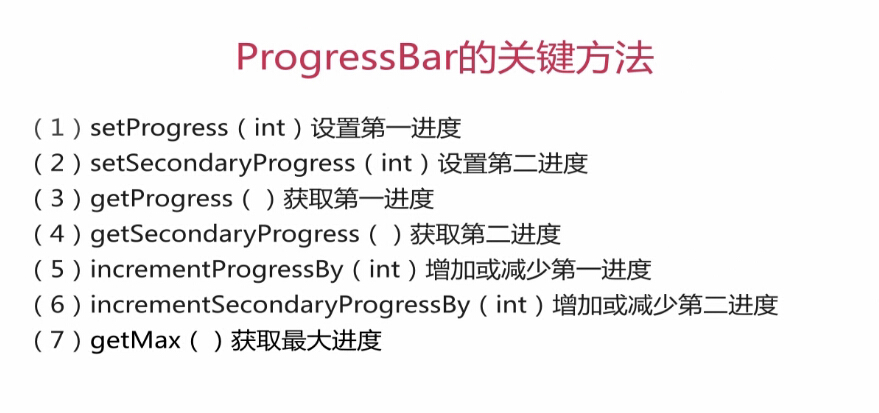
MainActivity :
main.xml:
MainActivity :
public class MainActivity extends Activity implements OnClickListener { private ProgressBar progressBar; private Button btAdd; private Button btReduce; private Button btReSet; private TextView text; private ProgressDialog progressDialog; private Button btShow; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); progressBar = (ProgressBar) findViewById(R.id.progressBar); btAdd = (Button) findViewById(R.id.add); btReduce = (Button) findViewById(R.id.reduce); btReSet = (Button) findViewById(R.id.reset); text = (TextView) findViewById(R.id.text); btShow = (Button) findViewById(R.id.show); // 获取第一条进度条的进度 int first = progressBar.getProgress(); // 获取第二条进度条的进度 int second = progressBar.getSecondaryProgress(); // 获取进度条的最大进度 int max = progressBar.getMax(); text.setText("第一进度百分比:" + (int) (first * 100.0 / max) + "%,第二进度百分比:" + (int) (second * 100.0 / max) + "%"); btAdd.setOnClickListener(this); btReduce.setOnClickListener(this); btReSet.setOnClickListener(this); btShow.setOnClickListener(this); } @Override public void onClick(View v) { switch (v.getId()) { case R.id.add: // 两个进度都增加10个刻度 progressBar.incrementProgressBy(10); progressBar.incrementSecondaryProgressBy(10); break; case R.id.reduce: progressBar.incrementProgressBy(-10); progressBar.incrementSecondaryProgressBy(-10); break; case R.id.reset: progressBar.setProgress(50); progressBar.setSecondaryProgress(80); break; case R.id.show: //新建ProgressDialog对象 progressDialog=new ProgressDialog(this); //设置显示风格 progressDialog.setProgressStyle(ProgressDialog.STYLE_HORIZONTAL); //设置标题 progressDialog.setTitle("对话框进度条"); //设置对话框中的文字 progressDialog.setMessage("这是对话框进度条"); //设置图标 progressDialog.setIcon(R.drawable.ic_launcher); /** * 设定关于ProgressBar的一些属性 */ //设定最大进度 progressDialog.setMax(100); //设定初始化的进度 progressDialog.incrementProgressBy(50); //进度条是明确显示进度的 progressDialog.setIndeterminate(false); /** * 设定一个确定按钮 */ progressDialog.setButton(DialogInterface.BUTTON_POSITIVE, "确定", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { Toast.makeText(MainActivity.this, "点了确定", Toast.LENGTH_SHORT).show(); } }); //是否可以通过返回按钮退出对话框 progressDialog.setCancelable(true); //显示ProgressDialog progressDialog.show(); break; } text.setText("第一进度百分比:" + (int) (progressBar.getProgress() * 100.0 / progressBar .getMax()) + "%,第二进度百分比:" + (int) (progressBar.getSecondaryProgress() * 100.0 / progressBar .getMax()) + "%"); } }
main.xml:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" > <!-- android:progressDrawable="" 可以定义配色方案 --> <ProgressBar android:id="@+id/progressBar" style="?android:attr/progressBarStyleHorizontal" android:layout_width="match_parent" android:layout_height="wrap_content" android:max="100" android:progress="50" android:secondaryProgress="80" /> <Button android:id="@+id/add" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/add" /> <Button android:id="@+id/reduce" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/reduce" /> <Button android:id="@+id/reset" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/reset" /> <TextView android:id="@+id/text" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <Button android:id="@+id/show" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/show" /> </LinearLayout>
相关文章推荐
- Android深入浅出系列之实例应用—程序运行进度提示条应用ProgressBar和Handler的使用
- Android深入浅出系列之实例应用—程序运行进度提示条应用ProgressBar和Handler的使用
- Android深入浅出系列之实例应用—程序运行进度提示条应用ProgressBar和Handler的使用
- Android程序解析XML文件的方法及使用PULL解析XML案例
- Android使用动画设置ProgressBar进度的方法
- Android程序解析XML文件的方法及使用PULL解析XML案例
- Android程序:checkbox的使用方法
- 谈使用Eclipse与DDMS调试Android程序的方法
- Android程序:使用SeekBar实现滑动进度条功能
- Android入门(15)——使用ProgressBar实现进度条
- 谈使用Eclipse与DDMS调试Android程序的方法
- Java如何操作Android的adb shell 之 我自己在程序中的使用方法
- 关于在android程序执行过程中使用Intent启动另一个活动后,同个方法未执行的代码是否会继续执行。
- Android 使用ProgressBar实现进度条
- Android自学笔记之ProgressBar进度条的属性、常用方法及使用
- Android中使用Makefile编译程序和库的方法
- 关于android程序中使用bitmap放大功能时的OOM问题解决方法
- 使用java传参调用exe并且获取程序进度和返回结果的一种方法
- 【Android】31、常见控件的使用方法——ProgressBar
- Android实用笔记——使用ProgressBar实现进度条