Python 解析xml文件
2015-01-25 13:29
281 查看
python有三种方法解析XML,分别是SAX,DOM,以及ElementTree,其中ElmentTree比较容易使用,其API比较方便友好。代码可用性好,速度快,消耗内存少。
xml中的元素主要有:tag,value,attribute
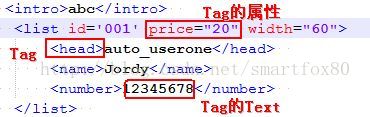
一个简单的python 解析xml的例子如下:
Xml文件为:
<?xml version="1.0" encoding="utf-8"?>
<info>
<list id='001' price="20" width="60">
<head>auto_userone</head>
<name>Jordy</name>
<number>12345678</number>
</list>
<list id='002' price="20" width="60">
<head>auto_usertwo</head>
<name>tester</name>
<number>34443678</number>
</list>
</info>
Python代码为:
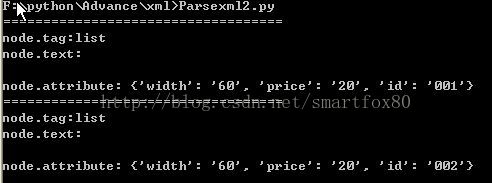
我们可以看到 xml的Attribute是一个字典,因此也可以用字典的方法遍历Attribute,获取具体属性的值。
def print_node(node):
print "==================================="
print "node.tag:%s" % node.tag
print "node.text:%s" % node.text
#print "node.attr:%s" % node.attrib
mapAttrib=node.attrib
for key in mapAttrib:
print "%s=%s" %(key,mapAttrib[key])
如果知道具体attribute 的名字,也可以用get方法获取属性的值:
如何遍历xml的元素呢? ElementTree提供了四种方法,这四种方法分别是getiterator,getchildren,find 和findall,具体使用方法如下:
# -*- coding: utf-8 -*-
import xml.etree.ElementTree as et
def print_node(node): print "===================================" print "node.tag:%s" % node.tag print "node.text:%s" % node.text print "id:%s" % node.get("id")
#读取xml文件
def load_xml_file(fileName):
root =et.parse(fileName)
#使用getiterator
nodes = root.getiterator("list")
for node in nodes:
print_node(node)
#使用getchildren()
children = nodes[0].getchildren()
for child in children:
print_node(child)
#使用find方法
specNode =root.find("list")
if specNode is not None:
print_node(specNode)
#使用findall方法
node_findall = root.findall("list/name")[1]
print_node(node_findall)
if __name__ == '__main__':
load_xml_file(r'F:\python\Advance\xml\sample.xml')
xml中的元素主要有:tag,value,attribute
一个简单的python 解析xml的例子如下:
Xml文件为:
<?xml version="1.0" encoding="utf-8"?>
<info>
<list id='001' price="20" width="60">
<head>auto_userone</head>
<name>Jordy</name>
<number>12345678</number>
</list>
<list id='002' price="20" width="60">
<head>auto_usertwo</head>
<name>tester</name>
<number>34443678</number>
</list>
</info>
Python代码为:
# -*- coding: utf-8 -*- import xml.etree.ElementTree as et def print_node(node): print "===================================" print "node.tag:%s" % node.tag print "node.text:%s" % node.text print "node.attribute: %s" %node.attrib #读取xml文件 def load_xml_file(fileName): root =et.parse(fileName) nodes = root.getiterator("list") for node in nodes: print_node(node) if __name__ == '__main__': load_xml_file(r'sample.xml')
我们可以看到 xml的Attribute是一个字典,因此也可以用字典的方法遍历Attribute,获取具体属性的值。
def print_node(node):
print "==================================="
print "node.tag:%s" % node.tag
print "node.text:%s" % node.text
#print "node.attr:%s" % node.attrib
mapAttrib=node.attrib
for key in mapAttrib:
print "%s=%s" %(key,mapAttrib[key])
如果知道具体attribute 的名字,也可以用get方法获取属性的值:
def print_node(node): print "===================================" print "node.tag:%s" % node.tag print "node.text:%s" % node.text print "id:%s" % node.get("id")
如何遍历xml的元素呢? ElementTree提供了四种方法,这四种方法分别是getiterator,getchildren,find 和findall,具体使用方法如下:
# -*- coding: utf-8 -*-
import xml.etree.ElementTree as et
def print_node(node): print "===================================" print "node.tag:%s" % node.tag print "node.text:%s" % node.text print "id:%s" % node.get("id")
#读取xml文件
def load_xml_file(fileName):
root =et.parse(fileName)
#使用getiterator
nodes = root.getiterator("list")
for node in nodes:
print_node(node)
#使用getchildren()
children = nodes[0].getchildren()
for child in children:
print_node(child)
#使用find方法
specNode =root.find("list")
if specNode is not None:
print_node(specNode)
#使用findall方法
node_findall = root.findall("list/name")[1]
print_node(node_findall)
if __name__ == '__main__':
load_xml_file(r'F:\python\Advance\xml\sample.xml')
相关文章推荐
- Python 解析XML文件
- 用python解析xml文件
- Python解析XML文件
- python lxml包——解析xml文件遇到的问题处理
- 使用python3.4解析xml文件(sax、dom、etree)
- 用python来解析xml文件(简单情况)
- python解析xml文件实例分享
- python解析xml文件实例分享
- python解析GBK格式xml文件
- Python SAX模块对大xml文件解析的错误认识
- python语言解析xml文件
- python 利用lxml 解析xml文件
- 用python来解析xml文件(简单情况)
- 解析XML文件总结 分类: python基础学习 python 2013-06-17 12:04 232人阅读 评论(0) 收藏
- python 学习 (二) 解析xml文件
- 利用python的xmllib2实现XML文件解析
- Python 解析XML文件
- Python基础(十一) 使用xml.dom 创建XML文件与解析
- python 解析xml文件
- python3解析XML文件