用JWidow写一个可以在桌面上拖动的小时钟
2015-01-10 00:00
387 查看
很多人可能对JWidow的使用不太清楚,下面就对JWidow写的一个小例子供参考!
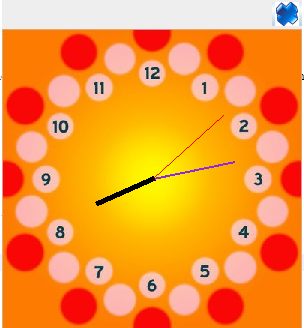
import java.awt.*;
这里用到了其他的一些类,由于篇幅的原因,就不往上贴了。源代码下载地址:
http://download.csdn.net/download/luoweifu/4590710
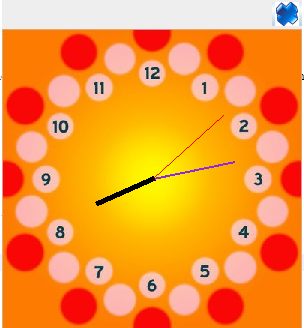
import java.awt.*;
import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import java.net.URL; import java.util.Calendar; import javax.swing.*; /** * 用于显示时钟 * @author 罗伟富 * */ public class ShowClock extends JWindow implements Runnable, ActionListener{ /** * 窗口的宽度 */ public static final int WIDTH = 300; /** * 窗口的宽度 */ public static final int HEIGHT = 330; /** * 时钟的宽度 */ public static final int CWIDTH = 300; /** *时钟的高度 */ public static final int CHEIGHT = 300; /** * 关闭按钮的宽度 */ public static final int CLOSEWIDTH = 30; /** * 关闭按钮的宽度 */ public static final int CLOSEHEIGHT = 30; /** * 度数转换成弧度的比例 */ final double RAD = Math.PI / 180; /** * 时针、分针、秒针的参考半径 */ public static final int R = 120; private CalendarTime calTime; private Image img; private JPanel clockPanel; private JButton closeButton; private Thread thread; private boolean isWorking = true; //原点,半径(时、分、秒),角度, private int ox, oy, r, h, m, s, hh, mm, ss; private int X = 0, Y = 0; /** * 构造函数 * @param calTime Calendar对象,用于计时 * @param img 用于作为背景的图像 */ public ShowClock(CalendarTime calTime, Image img) { this.calTime = calTime; this.img = img; thread = new Thread(this); thread.start(); initialize(); } /** * 为true,则计时器在计时,否则不计时 * @return 为true,则计时器在计时,否则不计时 */ public boolean isWorking() { return isWorking; } /** * 设置isWork的状态 * @param isWorking */ public void setWorking(boolean isWorking) { this.isWorking = isWorking; } /** * 初始化组件 */ public void initialize() { ox = 150; oy = 150; final ImageIcon originalImg = new ImageIcon(this.getClass().getResource("/Images/close1.gif")); final ImageIcon rolloverImg = new ImageIcon(this.getClass().getResource("/Images/close2.gif")); closeButton = new ImageButton(originalImg); closeButton.setRolloverIcon(rolloverImg); closeButton.addActionListener(this); clockPanel = new PaintPanel(); clockPanel.setPreferredSize(new Dimension(CWIDTH, CHEIGHT)); JLabel emptyLabel = new JLabel(); emptyLabel.setPreferredSize(new Dimension(WIDTH-CLOSEWIDTH, CLOSEHEIGHT)); Container c = getContentPane(); c.setLayout(new GridBagLayout()); GridBagConstraints gbc = new GridBagConstraints(); gbc.gridx = 0; gbc.gridy = 0; gbc.gridwidth = 1; gbc.gridheight = 1; gbc.fill = gbc.HORIZONTAL; gbc.anchor = gbc.CENTER; c.add(emptyLabel, gbc); gbc.gridx = 1; gbc.gridy = 0; c.add(closeButton, gbc); gbc.gridx = 0; gbc.gridy = 1; gbc.gridwidth = 2; c.add(clockPanel, gbc); setSize(WIDTH, HEIGHT); addMouseListener(new MouseAdapter() { public void mousePressed(MouseEvent e) { X = e.getX(); Y = e.getY(); } } ); addMouseMotionListener(new MouseAdapter() { public void mouseDragged(MouseEvent e) { setLocation(getLocation().x + (e.getX()-X),getLocation().y + (e.getY()-Y)); } } ); } /** * 每隔一秒中刷新一次显示时钟的屏幕 */ public void run() { try { while(isWorking) { Thread.sleep(1000); clockPanel.repaint(); } } catch (InterruptedException e) { e.printStackTrace(); } } /** * */ public void actionPerformed(ActionEvent e) { if(e.getSource() == closeButton) { setVisible(false); } } public static void main(String[] args) { 7fe8 URL urlImg = ShowClock.class.getResource("/Images/clock.jpg"); Image imgClock = Toolkit.getDefaultToolkit().getImage(urlImg); ShowClock showclock = new ShowClock(new CalendarTime(), imgClock); showclock.setLocationRelativeTo(null); showclock.setVisible(true); } /** * 用于画时钟的JPanel面板 */ class PaintPanel extends JPanel { public void paint(Graphics g) { super.paint(g); Graphics2D g2 = (Graphics2D)g; g2.drawImage(img, 0, 0, CWIDTH, CHEIGHT, this); h = calTime.get(Calendar.HOUR); m = calTime.get(Calendar.MINUTE); s = calTime.get(Calendar.SECOND); ss = 90-6*s; mm = 90-(6*m+s/10); //即 mm = 90-(6*m+6*(s/60)); hh = 90-(30*h+m/2); //即hh = 90-30*(m/60); g2.setStroke(new BasicStroke(1.0f)); g2.setColor(Color.red); int x1 = (int)(0.8*R*Math.cos(ss*RAD)) + ox; int y1 = (int)(0.8*R*Math.sin(ss*RAD)) + oy; g2.drawLine(ox, oy, x1, CHEIGHT-y1); g2.setStroke(new BasicStroke(2.0f)); g2.setColor(new Color(138, 43, 226)); int x2 = (int)(0.7*R*Math.cos(mm*RAD)) + ox; int y2 = (int)(0.7*R*Math.sin(mm*RAD)) + oy; g2.drawLine(ox, oy, x2, CHEIGHT-y2); g2.setStroke(new BasicStroke(5.0f)); g2.setColor(Color.black); int x3 = (int)(0.5*R*Math.cos(hh*RAD)) + ox; int y3 = (int)(0.5*R*Math.sin(hh*RAD)) + oy; g2.drawLine(ox, oy, x3, CHEIGHT-y3); } } }
这里用到了其他的一些类,由于篇幅的原因,就不往上贴了。源代码下载地址:
http://download.csdn.net/download/luoweifu/4590710
相关文章推荐
- 用JWidow写一个可以在桌面上拖动的小时钟
- 用JWidow写一个可以在桌面上拖动的小时钟
- 发现一个很有意思的事情,可以改变显示桌面的图标
- 在vs2010中可以很方便的打包桌面程序和同一解决方案中一个项目引用另外一个项目的问题
- 一个可以拖动指针设置hour,minute的钟表,开源框架TimePickerDialog改动eclipse project
- 自定义画一个可以拖动的圆形或图片
- 一个可以拖动的自定义Gridview代码
- 使用API实现的一个增加系统桌面,并且可以任意切换的小程序.
- js实现一个可以兼容PC端和移动端的div拖动效果
- Qt中,当QDockWidget的父窗口是一个不可以拖动的QTabWidget的时候实现拖动的方法
- js实现一个可以兼容PC端和移动端的div拖动效果实例
- 最近由于要用到分隔条,找到了一篇讲原理的, 实现了一个。可以双击缩到左边,可以左右拖动
- linux下如何为刚安装好的Eclipse在桌面建一个启动图标???(QtCreator 也可以类似去做)
- 自定义一个可以随着手指拖动的按钮
- 编写一个单文档界面的应用程序,程序启动后在用户区显示一个圆,用鼠标可以拖动这个圆。
- 用几十行代码写一个可以在PC Web,PC桌面,安卓,iOS上运行的程序
- 本来从动态壁纸预览页面设置一个动态壁纸回到桌面便可以看到桌面动态壁纸,可以观察得到自己的动态壁纸是否设置成功了(必须知道设置是否成功的结构,因为还有一些操作需要完成)! 但是现在是要在自己的应用中进入
- 发现一个好东东,可以让浏览器跟本地桌面交互,哈哈
- 一个可以拖动的自定义Gridview代码
- js实现一个可以兼容PC端和移动端的div拖动效果