前端加密数据后台解密之RSA算法实现
2014-09-23 12:32
417 查看
1、概述
前端时间与前端人员配合开发一款移动端WEB-APP,在各种因素的限制下,数据的安全性,让我为难,目前最可行和最直接的方式就是对所有用户敏感数据进行加密处理,然后传输到后端,解析处理。事先我尝试fiddler工具,拦截了APP所有的请求,结果发现不得不做安全处理了,至少先对这些数据加密处理。本文将讲述如何使用前端加密后端解密的过程,以及途中遇到的问题,如何解决。
2、选择加密算法
由于前端各种数据很容易被其他人获取,自然而然的选择的非对称加密,个人选择的是RSA算法。在确定好算法之后。最重要的问题前端如何对数据加密。我选择了网络上的一些的前端加密JS插件,这里可以参考: http://www.oschina.net/code/snippet_1611_4789 (写的不错的)
3、遇到的问题及解决方案
1)前端加密方式是采用模数和指数加密,无法将后端的公用KEY放置到前端;
解决方式:
起初的想法是在需要加密前,发送请求到后端获取公用key的模数和指数,但是由于前端采用的jsonp方式,无法请求同步处理,因此方式了该处理方式,而且每次发送请求获取模数和指数并不是很好的选择,采用的方式是在后端确定好公用KEy和私用KEY,并将其模数和指数确定,直接写在前端代码中。这样前端可以更加高效的加密,并且后端也可以根据确定的私用KEy解密。
4、DEMO展示
相关包需求:
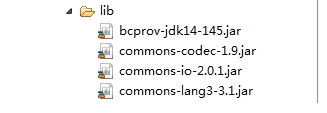
附件:http://down.51cto.com/data/2364889
前端时间与前端人员配合开发一款移动端WEB-APP,在各种因素的限制下,数据的安全性,让我为难,目前最可行和最直接的方式就是对所有用户敏感数据进行加密处理,然后传输到后端,解析处理。事先我尝试fiddler工具,拦截了APP所有的请求,结果发现不得不做安全处理了,至少先对这些数据加密处理。本文将讲述如何使用前端加密后端解密的过程,以及途中遇到的问题,如何解决。
2、选择加密算法
由于前端各种数据很容易被其他人获取,自然而然的选择的非对称加密,个人选择的是RSA算法。在确定好算法之后。最重要的问题前端如何对数据加密。我选择了网络上的一些的前端加密JS插件,这里可以参考: http://www.oschina.net/code/snippet_1611_4789 (写的不错的)
3、遇到的问题及解决方案
1)前端加密方式是采用模数和指数加密,无法将后端的公用KEY放置到前端;
解决方式:
起初的想法是在需要加密前,发送请求到后端获取公用key的模数和指数,但是由于前端采用的jsonp方式,无法请求同步处理,因此方式了该处理方式,而且每次发送请求获取模数和指数并不是很好的选择,采用的方式是在后端确定好公用KEy和私用KEY,并将其模数和指数确定,直接写在前端代码中。这样前端可以更加高效的加密,并且后端也可以根据确定的私用KEy解密。
4、DEMO展示
相关包需求:
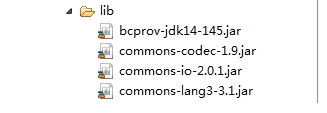
package com.rsa.codec; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; import java.math.BigInteger; import java.security.InvalidParameterException; import java.security.KeyFactory; import java.security.KeyPair; import java.security.KeyPairGenerator; import java.security.NoSuchAlgorithmException; import java.security.PrivateKey; import java.security.Provider; import java.security.PublicKey; import java.security.SecureRandom; import java.security.interfaces.RSAPrivateKey; import java.security.interfaces.RSAPublicKey; import java.security.spec.InvalidKeySpecException; import java.security.spec.RSAPrivateKeySpec; import java.security.spec.RSAPublicKeySpec; import javax.crypto.Cipher; import org.apache.commons.codec.DecoderException; import org.apache.commons.codec.binary.Hex; import org.apache.commons.io.FileUtils; import org.apache.commons.io.IOUtils; import org.apache.commons.lang3.StringUtils; import org.bouncycastle.jce.provider.BouncyCastleProvider; /** * RSA签名 * 注:原代码来源于http://www.oschina.net/code/snippet_1611_4789,自己稍做了一些修改 * @author wangzp */ public class RsaUtil { /** 算法名称 */ private static final String ALGORITHOM = "RSA"; /**保存生成的密钥对的文件名称。 */ private static final String RSA_PAIR_FILENAME = "/__RSA_PAIR.txt"; /** 密钥大小 */ private static final int KEY_SIZE = 1024; /** 默认的安全服务提供者 */ private static final Provider DEFAULT_PROVIDER = new BouncyCastleProvider(); private static KeyPairGenerator keyPairGen = null; private static KeyFactory keyFactory = null; /** 缓存的密钥对。 */ private static KeyPair oneKeyPair = null; private static File rsaPairFile = null; static { try { keyPairGen = KeyPairGenerator.getInstance(ALGORITHOM, DEFAULT_PROVIDER); keyFactory = KeyFactory.getInstance(ALGORITHOM, DEFAULT_PROVIDER); } catch (NoSuchAlgorithmException ex) { } rsaPairFile = new File(getRSAPairFilePath()); } private RsaUtil() { } /** * 生成并返回RSA密钥对。 */ private static synchronized KeyPair generateKeyPair() { try { keyPairGen.initialize(KEY_SIZE, new SecureRandom()); oneKeyPair = keyPairGen.generateKeyPair(); saveKeyPair(oneKeyPair); return oneKeyPair; } catch (InvalidParameterException ex) { } catch (NullPointerException ex) { } return null; } /** * 返回生成/读取的密钥对文件的路径。 */ private static String getRSAPairFilePath() { String urlPath = RsaUtil.class.getResource("/").getPath(); return (new File(urlPath).getParent() + RSA_PAIR_FILENAME); } /** * 若需要创建新的密钥对文件,则返回 {@code true},否则 {@code false}。 */ private static boolean isCreateKeyPairFile() { // 是否创建新的密钥对文件 boolean createNewKeyPair = false; if (!rsaPairFile.exists() || rsaPairFile.isDirectory()) { createNewKeyPair = true; } return createNewKeyPair; } /** * 将指定的RSA密钥对以文件形式保存。 * * @param keyPair 要保存的密钥对。 */ private static void saveKeyPair(KeyPair keyPair) { FileOutputStream fos = null; ObjectOutputStream oos = null; try { fos = FileUtils.openOutputStream(rsaPairFile); oos = new ObjectOutputStream(fos); oos.writeObject(keyPair); } catch (Exception ex) { ex.printStackTrace(); } finally { IOUtils.closeQuietly(oos); IOUtils.closeQuietly(fos); } } /** * 返回RSA密钥对。 */ public static KeyPair getKeyPair() { // 首先判断是否需要重新生成新的密钥对文件 if (isCreateKeyPairFile()) { // 直接强制生成密钥对文件,并存入缓存。 return generateKeyPair(); } if (oneKeyPair != null) { return oneKeyPair; } return readKeyPair(); } // 同步读出保存的密钥对 private static KeyPair readKeyPair() { FileInputStream fis = null; ObjectInputStream ois = null; try { fis = FileUtils.openInputStream(rsaPairFile); ois = new ObjectInputStream(fis); oneKeyPair = (KeyPair) ois.readObject(); return oneKeyPair; } catch (Exception ex) { ex.printStackTrace(); } finally { IOUtils.closeQuietly(ois); IOUtils.closeQuietly(fis); } return null; } /** * 根据给定的系数和专用指数构造一个RSA专用的公钥对象。 * * @param modulus 系数。 * @param publicExponent 专用指数。 * @return RSA专用公钥对象。 */ public static RSAPublicKey generateRSAPublicKey(byte[] modulus, byte[] publicExponent) { RSAPublicKeySpec publicKeySpec = new RSAPublicKeySpec(new BigInteger(modulus), new BigInteger(publicExponent)); try { return (RSAPublicKey) keyFactory.generatePublic(publicKeySpec); } catch (InvalidKeySpecException ex) { } catch (NullPointerException ex) { } return null; } /** * 根据给定的系数和专用指数构造一个RSA专用的私钥对象。 * * @param modulus 系数。 * @param privateExponent 专用指数。 * @return RSA专用私钥对象。 */ public static RSAPrivateKey generateRSAPrivateKey(byte[] modulus, byte[] privateExponent) { RSAPrivateKeySpec privateKeySpec = new RSAPrivateKeySpec(new BigInteger(modulus), new BigInteger(privateExponent)); try { return (RSAPrivateKey) keyFactory.generatePrivate(privateKeySpec); } catch (InvalidKeySpecException ex) { } catch (NullPointerException ex) { } return null; } /** * 根据给定的16进制系数和专用指数字符串构造一个RSA专用的私钥对象。 * * @param modulus 系数。 * @param privateExponent 专用指数。 * @return RSA专用私钥对象。 */ public static RSAPrivateKey getRSAPrivateKey(String hexModulus, String hexPrivateExponent) { if(StringUtils.isBlank(hexModulus) || StringUtils.isBlank(hexPrivateExponent)) { return null; } byte[] modulus = null; byte[] privateExponent = null; try { modulus = Hex.decodeHex(hexModulus.toCharArray()); privateExponent = Hex.decodeHex(hexPrivateExponent.toCharArray()); } catch(DecoderException ex) { } if(modulus != null && privateExponent != null) { return generateRSAPrivateKey(modulus, privateExponent); } return null; } /** * 根据给定的16进制系数和专用指数字符串构造一个RSA专用的公钥对象。 * * @param modulus 系数。 * @param publicExponent 专用指数。 * @return RSA专用公钥对象。 */ public static RSAPublicKey getRSAPublidKey(String hexModulus, String hexPublicExponent) { if(StringUtils.isBlank(hexModulus) || StringUtils.isBlank(hexPublicExponent)) { return null; } byte[] modulus = null; byte[] publicExponent = null; try { modulus = Hex.decodeHex(hexModulus.toCharArray()); publicExponent = Hex.decodeHex(hexPublicExponent.toCharArray()); } catch(DecoderException ex) { } if(modulus != null && publicExponent != null) { return generateRSAPublicKey(modulus, publicExponent); } return null; } /** * 使用指定的公钥加密数据。 * * @param publicKey 给定的公钥。 * @param data 要加密的数据。 * @return 加密后的数据。 */ public static byte[] encrypt(PublicKey publicKey, byte[] data) throws Exception { Cipher ci = Cipher.getInstance(ALGORITHOM, DEFAULT_PROVIDER); ci.init(Cipher.ENCRYPT_MODE, publicKey); return ci.doFinal(data); } /** * 使用指定的私钥解密数据。 * * @param privateKey 给定的私钥。 * @param data 要解密的数据。 * @return 原数据。 */ public static byte[] decrypt(PrivateKey privateKey, byte[] data) throws Exception { Cipher ci = Cipher.getInstance(ALGORITHOM, DEFAULT_PROVIDER); ci.init(Cipher.DECRYPT_MODE, privateKey); return ci.doFinal(data); } /** * 使用给定的公钥加密给定的字符串。 * <p /> * 若 {@code publicKey} 为 {@code null},或者 {@code plaintext} 为 {@code null} 则返回 {@code * null}。 * * @param publicKey 给定的公钥。 * @param plaintext 字符串。 * @return 给定字符串的密文。 */ public static String encryptString(PublicKey publicKey, String plaintext) { if (publicKey == null || plaintext == null) { return null; } byte[] data = plaintext.getBytes(); try { byte[] en_data = encrypt(publicKey, data); return new String(Hex.encodeHex(en_data)); } catch (Exception ex) { } return null; } /** * 使用默认的公钥加密给定的字符串。 * <p /> * 若{@code plaintext} 为 {@code null} 则返回 {@code null}。 * * @param plaintext 字符串。 * @return 给定字符串的密文。 */ public static String encryptString(String plaintext) { if(plaintext == null) { return null; } byte[] data = plaintext.getBytes(); KeyPair keyPair = getKeyPair(); try { byte[] en_data = encrypt((RSAPublicKey)keyPair.getPublic(), data); return new String(Hex.encodeHex(en_data)); } catch(NullPointerException ex) { } catch(Exception ex) { } return null; } /** * 使用给定的私钥解密给定的字符串。 * <p /> * 若私钥为 {@code null},或者 {@code encrypttext} 为 {@code null}或空字符串则返回 {@code null}。 * 私钥不匹配时,返回 {@code null}。 * * @param privateKey 给定的私钥。 * @param encrypttext 密文。 * @return 原文字符串。 */ public static String decryptString(PrivateKey privateKey, String encrypttext) { if (privateKey == null || StringUtils.isBlank(encrypttext)) { return null; } try { byte[] en_data = Hex.decodeHex(encrypttext.toCharArray()); byte[] data = decrypt(privateKey, en_data); return new String(data); } catch (Exception ex) { } return null; } /** * 使用默认的私钥解密给定的字符串。 * <p /> * 若{@code encrypttext} 为 {@code null}或空字符串则返回 {@code null}。 * 私钥不匹配时,返回 {@code null}。 * * @param encrypttext 密文。 * @return 原文字符串。 */ public static String decryptString(String encrypttext) { if(StringUtils.isBlank(encrypttext)) { return null; } KeyPair keyPair = getKeyPair(); try { byte[] en_data = Hex.decodeHex(encrypttext.toCharArray()); byte[] data = decrypt((RSAPrivateKey)keyPair.getPrivate(), en_data); return new String(data); } catch(NullPointerException ex) { } catch (Exception ex) { } return null; } /** * 使用默认的私钥解密由JS加密(使用此类提供的公钥加密)的字符串。 * * @param encrypttext 密文。 * @return {@code encrypttext} 的原文字符串。 */ public static String decryptStringByJs(String encrypttext) { String text = decryptString(encrypttext); if(text == null) { return null; } return StringUtils.reverse(text); } /** * 与文中参考的博客不同,添加了该方法 * @param privateKey * @param encrypttext * @return */ public static String decryptStringByJs(RSAPrivateKey privateKey, String encrypttext) { String text = decryptString(privateKey, encrypttext); if(text == null) { return null; } return StringUtils.reverse(text); } /** 返回已初始化的默认的公钥。*/ public static RSAPublicKey getDefaultPublicKey() { KeyPair keyPair = getKeyPair(); if(keyPair != null) { return (RSAPublicKey)keyPair.getPublic(); } return null; } /** 返回已初始化的默认的私钥。*/ public static RSAPrivateKey getDefaultPrivateKey() { KeyPair keyPair = getKeyPair(); if(keyPair != null) { return (RSAPrivateKey)keyPair.getPrivate(); } return null; } public static void main(String[] args) throws DecoderException { RSAPublicKey publicKey = getDefaultPublicKey(); RSAPrivateKey privateKey = getDefaultPrivateKey(); String data = "username=wangzp&admin=ok"; String codeString = encryptString(publicKey, data); System.out.println(codeString); System.out.println(decryptString(privateKey, codeString)); } }上述代码可以生成默认的KEy对文件,也可以生成指定的Key根据模数和指数。可以根据实际需求,来选择使用默认key对,还是定制的。
<%@ page language="java" import="java.util.*" pageEncoding="ISO-8859-1"%> <% String path = request.getContextPath(); String basePath = request.getScheme() + "://" + request.getServerName() + ":" + request.getServerPort() + path + "/"; %> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <base href="<%=basePath%>"> <title>My JSP 'index.jsp' starting page</title> <meta http-equiv="pragma" content="no-cache"> <meta http-equiv="cache-control" content="no-cache"> <meta http-equiv="expires" content="0"> <meta http-equiv="keywords" content="keyword1,keyword2,keyword3"> <meta http-equiv="description" content="This is my page"> <script type="text/javascript" src="./js/RSA.js"></script> <script type="text/javascript"> function rsalogin() { var thisPwd = document.getElementById("password").value; var result = encryptedString(getKey(), encodeURIComponent(thisPwd)); loginForm.action = "login.do?result=" + result; loginForm.submit(); } function getKey() { setMaxDigits(130); // 指数和模数可以根据需求,从后台获取,或者写在前端代码中 key = new RSAKeyPair( "<加密指数>", "<解密指数>", "<公Key模数>"); return key; } </script> </head> <body> <form method="post" name="loginForm" target=_blank> Password:<input type='text' name="password" id="password" style='width:400px' value="test" /> <input type="button" value="SUBMIT" onclick="rsalogin();" /> </form> <br> </body> </html>注:附件是RSA前端加密js文件
附件:http://down.51cto.com/data/2364889
相关文章推荐
- 加密传到后台的数据,使用RSA非对称加密,前端加密,后台java解密
- RSA AES 前端JS与后台JAVA的加密解密的是实现
- JAVA WEB实现前端加密后台解密
- AJAX+REA实现前后台数据交互的加密解密
- 关于使用JS前台加密、JAVA后台解密的RSA实现,RSA加密和签名
- ios下使用RSA算法加密与java后台解密配合demo
- 安卓Shareprefrence实现记住密码自动登录以及保存在shareprefrence里面数据如何加密解密
- ios下使用RSA算法加密与java后台解密配合demo
- 实现客户端加密,后台解密
- C# 实现 数据加密、解密方法
- js前端3des加密 后台java解密
- 数据的加密传输——单片机上实现TEA加密解密算法(转)
- JS前台加密,java后台解密实现
- VC实现数据的加密和解密(MD5加密/DES/RSA加密解密)
- JS前台加密,java后台解密实现
- JS前台加密,java后台解密实现
- RSA前端JS加密,后端JAVA解密实现
- java实现数据的加密解密
- 实现客户端加密,后台解密
- ios下使用RSA算法加密与java后台解密配合demo