带头结点的单链表( 冒泡排序 一次遍历求中间结点的值) 链表 就地反转 倒数第k个结点
2014-04-14 22:06
471 查看
Source Code:
Result:
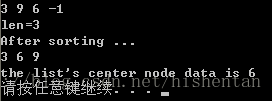
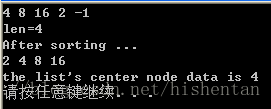
就地反转
倒数第k个结点:
注意程序的鲁棒性
#include <iostream> using namespace std; typedef struct student { int num; struct student *next; }node; node *create() {//with head node node *head,*p,*s;//head -->head p-->end s -->middle node int num; head = new node[sizeof(struct student)]; p=head; cin>>num; while(num!=-1) { s = new node[sizeof(struct student)]; s->num= num; p->next = s; p = s; cin>>num; } p->next = NULL; return head; } int print(node *l)//return the len of the linklist { node *p = l; int count = 0; p = p->next; while(p) { cout<<p->num<<" "; p= p->next; count++; } cout<<endl; return count; } int GetLen(node *l) { node *p = l; int count = 0; p = p->next; while(p) { p= p->next; count++; } return count; } node *sort(node *l) {//sort AES int len = GetLen(l); node *head = l; node *p;//move forward int temp; for(int i = 0;i<len-1;i++) { p = head->next; for(int j = 0;j<len-i-1;j++) { if(p->num>p->next->num) { temp = p->num; p->num = p->next->num; p->next->num = temp; } p = p->next; } } return head; } int GetCenterNode(node *l) {//一次遍历求中间结点的值 node *p2 = l;//two step node *p1 = l;//one step while(1) { p2 = p2->next->next; p1 = p1->next; if(p2->next == NULL)//len %2 ==0 break; if(p2->next->next == NULL)//len %2 == 1 { p1 = p1->next; break; } } return p1->num; } int main() { node *StudentLink = create(); int len= GetLen(StudentLink); cout<<"len="<<len<<endl; node *Sorted_StudetLink = sort(StudentLink);// O(n) + O(n*n) cout<<"After sorting ..."<<endl; print(Sorted_StudetLink); cout<<"the list's center node data is "<<GetCenterNode(StudentLink)<<endl;//O(n) return 0; }
Result:
就地反转
ListNode* ReverseList(ListNode* pHead) { ListNode* pNode=pHead;//当前结点 ListNode* pPrev=NULL;//当前结点的前一个结点 while(pNode!=NULL) { ListNode* pNext=pNode->m_pNext; pNode->m_pNext=pPrev;//当前结点指向前一个结点 pPrev=pNode;//pPrev和pNode往前移动。 pNode=pNext;//这里要使用前面保存下来的pNext,不能使用pNode->m_pNext } return pPrev;//返回反转链表头指针。 }
倒数第k个结点:
注意程序的鲁棒性
ListNode* KthNodeFromEnd(ListNode* pHead,int k) { if(pHead==NULL||k==0) return NULL; ListNode* pNode=pHead;//当前结点 ListNode* pKthNode=pHead;// while(k-1>0) { if(pNode->m_pNext!=NULL) { pNode=pNode->m_pNext;//让pNode先走k-1步 --k; } else return NULL; } while(pNode->m_pNext!=NULL) { pNode=pNode->m_pNext; pKthNode=pKthNode->m_pNext; } return pKthNode; }
相关文章推荐
- 笔试面试,单链表相关(3)遍历一次找中间结点、倒数第K个结点
- 单链表遍历一次求倒数第k个结点和中间结点
- 笔试面试,单链表相关(3)遍历一次找中间结点、倒数第K个结点
- 链表的倒数第K个结点(一次遍历)
- 逆置/反转单链表+查找单链表的倒数第k个节点,要求只能遍历一次链表
- 【链表】查找链表倒数第k个结点,要求只能遍历一次
- 有关单链表的两个问题【遍历一次求中间节点,倒数第K个结点】
- C实现简单单向链表,一次遍历查找倒数第k个节点的值
- 逆置/反转单链表+查找单链表的倒数第k个节点,要求只能遍历一次链表
- 查找单链表的倒数第k个节点,要求只能遍历一次链表(C语言)
- 只遍历链表一次求链表中间结点
- C语言:【单链表】查找单链表的倒数第k个节点,要求只能遍历一次
- 建立一个带附加头结点的单链表.实现测长/打印/删除结点/插入结点/逆置/查找中间节点/查找倒数第k个节点/判断是否有环
- 找链表的中间结点和倒数第k个结点(链表笔试题面试题)
- 查找单链表的倒数第k个节点,要求只能遍历一次链表
- 查找单链表的倒数第k个节点,要求只能遍历一次链表
- 巧妙利用两个指针遍历链表——链表中倒数第k个结点
- 带头结点的单链表和不带头结点的单链表的倒数第K个节点
- C语言:【单链表】查找单链表的倒数第k个节点,要求只能遍历一次
- 《剑指offer》--- 链表中的倒数第k个结点 和 反转链表