CreateJS奥运五环动画
2014-02-26 14:04
393 查看
索契奥运会结束了,今天给大家看一个利用CreateJS实现的奥运五环动画,在线把玩请点这里,下载收藏请戳这里。
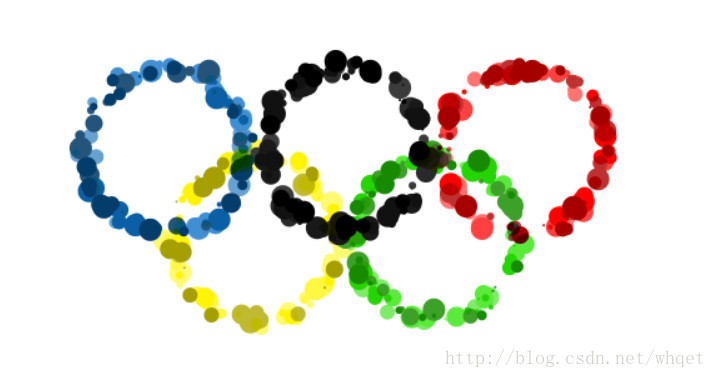
html文件非常简单,仅仅是弄了个canvas
[html]
view plaincopy


<canvas id="world"></canvas>
没有用到CSS,JS文件如下,在这个效果里面五环我们需要两个类,环类和粒子类。这里用到了CreateJS里面的easeljs。我们需要导入这个文件。 http://code.createjs.com/easeljs-0.7.1.min.js
[javascript]
view plaincopy


/*-----------------------------------
变量
-----------------------------------*/
var cj = createjs,
stage,
particles = [],
centerX,
centerY,
particleNum = window.innerWidth / 6,
color = ["blue","black","red","yellow","green"];
speed = Math.PI / 60,
RADIUS = window.innerWidth / 10, //圆的半径
margin = RADIUS / 10;
SPEED_MIN = RADIUS / 2,
SPEED_MAX = RADIUS;
/*-----------------------------------
初始化
-----------------------------------*/
function init(){
var rotateCenterX,
rotateCenterY,
circle,
radius = RADIUS;
//生成舞台
stage = new cj.Stage("world");
stage.canvas.width = window.innerWidth;
stage.canvas.height = window.innerHeight;
for(var i = 1;i <= 3;i++){
circle = new Circle(i,1,radius,color[i - 1]);
circle.create();
}
circle = new Circle(1,2,radius,color[3]);
circle.create();
circle = new Circle(2,2,radius,color[4]);
circle.create();
//渲染到舞台
stage.update();
}
cj.Ticker.timingMode = cj.Ticker.RAF;
cj.Ticker.addEventListener("tick",tick);
/*-----------------------------------
自動更新
-----------------------------------*/
function tick(){
for(var i = 0;i < particles.length;i++){
var particle = particles[i];
particle.move();
}
//渲染到舞台
stage.update();
}
/*-----------------------------------
粒子构造方法
-----------------------------------*/
function Particle(cx,cy,_angle,_radius,_color){
this.initialize();
//半径
this.radius = getRandomNum(5,10);
getColor(this,_color);
this.graphics.drawCircle(0,0,getRandomNum(1,10))
.endFill();
//中心位置
this.centerX = cx;
this.centerY = cy;
//角度
this.angle = _angle;
//角速度
if(getRandomNum(1,10) % 2 == 0){
this.speed = Math.PI / (getRandomNum(SPEED_MIN,SPEED_MAX));
}else{
this.speed = - Math.PI / (getRandomNum(SPEED_MIN,SPEED_MAX));
}
this.rotateCenterX = cx + _radius;
this.rotateCenterY = cy;
this.compositeOperation = "darker";
}
//継承 <
104f0
/span>
Particle.prototype = new cj.Shape();
/*-----------------------------------
粒子移动位置
-----------------------------------*/
Particle.prototype.move = function(){
this.angle += this.speed;
this.rotateCenterX = this.centerX + (RADIUS - margin) * Math.cos(this.angle / 5);
this.rotateCenterY = this.centerY + (RADIUS - margin) * Math.sin(this.angle / 5);
this.x = this.rotateCenterX + this.radius * Math.cos(this.angle / 360) * Math.cos(this.angle);
this.y = this.rotateCenterY + this.radius * Math.sin(this.angle / 360) * Math.sin(this.angle);
};
/*-----------------------------------
环类构造方法
-----------------------------------*/
function Circle(cx,cy,r,_color){
if(cy == 1){//上段
this.centerX = r + 2 * r * (cx - 1) + ((window.innerWidth / 2) - 3 * r);
this.centerY = r * cy + ((window.innerHeight / 2) - 1.5 * r);
}else{//下段
this.centerX = r + 2 * r * (cx - 1) + r + ((window.innerWidth / 2) - 3 * r);
this.centerY = r * cy + ((window.innerHeight / 2) - 1.5 * r);
}
this.radius = r;
this.color = _color;
}
/*-----------------------------------
环类的生成方法
-----------------------------------*/
Circle.prototype.create = function(){
var rotateCenterX = this.centerX + this.radius,
rotateCenterY = this.centerY;
for(var j = 1;j < particleNum;j++){
var angle = j * 15 * 10;
var particle = new Particle(this.centerX,this.centerY,angle,this.radius,this.color);
particles.push(particle);
stage.addChild(particle);
}
};
/*-----------------------------------
取得随机数
-----------------------------------*/
function getRandomNum( min, max ) {
return ( Math.random() * ( max - min ) + min ) | 0;
}
/*-----------------------------------
取得颜色
-----------------------------------*/
function getColor(obj,_color){
var fillColor;
switch(_color){
case "blue":
switch((Math.random() * 5 | 0 ) % 5){
case 0:
fillColor = obj.graphics.beginFill("#0B5FA5");
break;
case 1:
fillColor = obj.graphics.beginFill("#25547B");
break;
case 2:
fillColor = obj.graphics.beginFill("#043C6B");
break;
case 3:
fillColor = obj.graphics.beginFill("#3F8FD2");
break;
case 4:
fillColor = obj.graphics.beginFill("#66A1D2");
break;
default:
break;
}
break;
case "black":
switch((Math.random() * 5 | 0 ) % 5){
case 0:
fillColor = obj.graphics.beginFill("#000");
break;
case 1:
fillColor = obj.graphics.beginFill("#111");
break;
case 2:
fillColor = obj.graphics.beginFill("#191919");
break;
case 3:
fillColor = obj.graphics.beginFill("#2a2a2a");
break;
case 4:
fillColor = obj.graphics.beginFill("#3b3b3b");
break;
default:
break;
}
break;
case "red":
switch((Math.random() * 5 | 0 ) % 5){
case 0:
fillColor = obj.graphics.beginFill("#FF0000");
break;
case 1:
fillColor = obj.graphics.beginFill("#BF3030");
break;
case 2:
fillColor = obj.graphics.beginFill("#A60000");
break;
case 3:
fillColor = obj.graphics.beginFill("#FF4040");
break;
case 4:
fillColor = obj.graphics.beginFill("FF7373");
break;
default:
break;
}
break;
case "yellow":
switch((Math.random() * 5 | 0 ) % 5){
case 0:
fillColor = obj.graphics.beginFill("#FFF500");
break;
case 1:
fillColor = obj.graphics.beginFill("#BFBA30");
break;
case 2:
fillColor = obj.graphics.beginFill("#A69F00");
break;
case 3:
fillColor = obj.graphics.beginFill("#FFF840");
break;
case 4:
fillColor = obj.graphics.beginFill("#FFFA73");
break;
default:
break;
}
break;
case "green":
switch((Math.random() * 5 | 0 ) % 5){
case 0:
fillColor = obj.graphics.beginFill("#25D500");
break;
case 1:
fillColor = obj.graphics.beginFill("#3DA028");
break;
case 2:
fillColor = obj.graphics.beginFill("#188A00");
break;
case 3:
fillColor = obj.graphics.beginFill("#59EA3A");
break;
case 4:
fillColor = obj.graphics.beginFill("#80EA69");
break;
default:
break;
}
break;
default:
break;
}
return fillColor;
}
init();
好的,大家可以仔细研读,最好在理清思路之后自行实现一下。
当然,大家也可以看看本博客的另一篇文章《SVG奥林匹克五环动画》,或是看看codepen上的另一个效果。
html文件非常简单,仅仅是弄了个canvas
[html]
view plaincopy

<canvas id="world"></canvas>
没有用到CSS,JS文件如下,在这个效果里面五环我们需要两个类,环类和粒子类。这里用到了CreateJS里面的easeljs。我们需要导入这个文件。 http://code.createjs.com/easeljs-0.7.1.min.js
[javascript]
view plaincopy

/*-----------------------------------
变量
-----------------------------------*/
var cj = createjs,
stage,
particles = [],
centerX,
centerY,
particleNum = window.innerWidth / 6,
color = ["blue","black","red","yellow","green"];
speed = Math.PI / 60,
RADIUS = window.innerWidth / 10, //圆的半径
margin = RADIUS / 10;
SPEED_MIN = RADIUS / 2,
SPEED_MAX = RADIUS;
/*-----------------------------------
初始化
-----------------------------------*/
function init(){
var rotateCenterX,
rotateCenterY,
circle,
radius = RADIUS;
//生成舞台
stage = new cj.Stage("world");
stage.canvas.width = window.innerWidth;
stage.canvas.height = window.innerHeight;
for(var i = 1;i <= 3;i++){
circle = new Circle(i,1,radius,color[i - 1]);
circle.create();
}
circle = new Circle(1,2,radius,color[3]);
circle.create();
circle = new Circle(2,2,radius,color[4]);
circle.create();
//渲染到舞台
stage.update();
}
cj.Ticker.timingMode = cj.Ticker.RAF;
cj.Ticker.addEventListener("tick",tick);
/*-----------------------------------
自動更新
-----------------------------------*/
function tick(){
for(var i = 0;i < particles.length;i++){
var particle = particles[i];
particle.move();
}
//渲染到舞台
stage.update();
}
/*-----------------------------------
粒子构造方法
-----------------------------------*/
function Particle(cx,cy,_angle,_radius,_color){
this.initialize();
//半径
this.radius = getRandomNum(5,10);
getColor(this,_color);
this.graphics.drawCircle(0,0,getRandomNum(1,10))
.endFill();
//中心位置
this.centerX = cx;
this.centerY = cy;
//角度
this.angle = _angle;
//角速度
if(getRandomNum(1,10) % 2 == 0){
this.speed = Math.PI / (getRandomNum(SPEED_MIN,SPEED_MAX));
}else{
this.speed = - Math.PI / (getRandomNum(SPEED_MIN,SPEED_MAX));
}
this.rotateCenterX = cx + _radius;
this.rotateCenterY = cy;
this.compositeOperation = "darker";
}
//継承 <
104f0
/span>
Particle.prototype = new cj.Shape();
/*-----------------------------------
粒子移动位置
-----------------------------------*/
Particle.prototype.move = function(){
this.angle += this.speed;
this.rotateCenterX = this.centerX + (RADIUS - margin) * Math.cos(this.angle / 5);
this.rotateCenterY = this.centerY + (RADIUS - margin) * Math.sin(this.angle / 5);
this.x = this.rotateCenterX + this.radius * Math.cos(this.angle / 360) * Math.cos(this.angle);
this.y = this.rotateCenterY + this.radius * Math.sin(this.angle / 360) * Math.sin(this.angle);
};
/*-----------------------------------
环类构造方法
-----------------------------------*/
function Circle(cx,cy,r,_color){
if(cy == 1){//上段
this.centerX = r + 2 * r * (cx - 1) + ((window.innerWidth / 2) - 3 * r);
this.centerY = r * cy + ((window.innerHeight / 2) - 1.5 * r);
}else{//下段
this.centerX = r + 2 * r * (cx - 1) + r + ((window.innerWidth / 2) - 3 * r);
this.centerY = r * cy + ((window.innerHeight / 2) - 1.5 * r);
}
this.radius = r;
this.color = _color;
}
/*-----------------------------------
环类的生成方法
-----------------------------------*/
Circle.prototype.create = function(){
var rotateCenterX = this.centerX + this.radius,
rotateCenterY = this.centerY;
for(var j = 1;j < particleNum;j++){
var angle = j * 15 * 10;
var particle = new Particle(this.centerX,this.centerY,angle,this.radius,this.color);
particles.push(particle);
stage.addChild(particle);
}
};
/*-----------------------------------
取得随机数
-----------------------------------*/
function getRandomNum( min, max ) {
return ( Math.random() * ( max - min ) + min ) | 0;
}
/*-----------------------------------
取得颜色
-----------------------------------*/
function getColor(obj,_color){
var fillColor;
switch(_color){
case "blue":
switch((Math.random() * 5 | 0 ) % 5){
case 0:
fillColor = obj.graphics.beginFill("#0B5FA5");
break;
case 1:
fillColor = obj.graphics.beginFill("#25547B");
break;
case 2:
fillColor = obj.graphics.beginFill("#043C6B");
break;
case 3:
fillColor = obj.graphics.beginFill("#3F8FD2");
break;
case 4:
fillColor = obj.graphics.beginFill("#66A1D2");
break;
default:
break;
}
break;
case "black":
switch((Math.random() * 5 | 0 ) % 5){
case 0:
fillColor = obj.graphics.beginFill("#000");
break;
case 1:
fillColor = obj.graphics.beginFill("#111");
break;
case 2:
fillColor = obj.graphics.beginFill("#191919");
break;
case 3:
fillColor = obj.graphics.beginFill("#2a2a2a");
break;
case 4:
fillColor = obj.graphics.beginFill("#3b3b3b");
break;
default:
break;
}
break;
case "red":
switch((Math.random() * 5 | 0 ) % 5){
case 0:
fillColor = obj.graphics.beginFill("#FF0000");
break;
case 1:
fillColor = obj.graphics.beginFill("#BF3030");
break;
case 2:
fillColor = obj.graphics.beginFill("#A60000");
break;
case 3:
fillColor = obj.graphics.beginFill("#FF4040");
break;
case 4:
fillColor = obj.graphics.beginFill("FF7373");
break;
default:
break;
}
break;
case "yellow":
switch((Math.random() * 5 | 0 ) % 5){
case 0:
fillColor = obj.graphics.beginFill("#FFF500");
break;
case 1:
fillColor = obj.graphics.beginFill("#BFBA30");
break;
case 2:
fillColor = obj.graphics.beginFill("#A69F00");
break;
case 3:
fillColor = obj.graphics.beginFill("#FFF840");
break;
case 4:
fillColor = obj.graphics.beginFill("#FFFA73");
break;
default:
break;
}
break;
case "green":
switch((Math.random() * 5 | 0 ) % 5){
case 0:
fillColor = obj.graphics.beginFill("#25D500");
break;
case 1:
fillColor = obj.graphics.beginFill("#3DA028");
break;
case 2:
fillColor = obj.graphics.beginFill("#188A00");
break;
case 3:
fillColor = obj.graphics.beginFill("#59EA3A");
break;
case 4:
fillColor = obj.graphics.beginFill("#80EA69");
break;
default:
break;
}
break;
default:
break;
}
return fillColor;
}
init();
好的,大家可以仔细研读,最好在理清思路之后自行实现一下。
当然,大家也可以看看本博客的另一篇文章《SVG奥林匹克五环动画》,或是看看codepen上的另一个效果。
相关文章推荐
- CreateJS奥运五环动画
- Android 如何在XML文件中定义动画
- WPF窗体动画显示和关闭
- ios 动画浅析
- Android仿美团加载数据、小人奔跑进度动画对话框(附顺丰快递员奔跑效果)
- 核心动画简介
- 【原】iOSCoreAnimation动画系列教程(二):CAKeyFrameAnimation【包会】
- 轻松学习之二——iOS利用Runtime自定义控制器POP手势动画
- Android吸入动画效果
- 显式动画
- ios 动画坑
- 15.5.4_创建太阳系的动画
- Android 动画框架详解
- Android animation 动画背景图自动播放的实现
- swift 实现简单的动画
- CSS3动画笔记
- android gif动画开源框架android-gif-drawable
- 源码推荐(0724):直播类送礼动画,将Storyboard生成约束代码
- 精妙无比 8款HTML5动画实例及源码
- Android——属性动画