使用Commons-fileupload组件和jstl实现文件上传
2013-12-30 17:45
786 查看
一、文件上传的需要:
1、<form action="${pageContext.request.contextPath}/uploadFile.do" enctype="multipart/form-data" method="post">
选择文件:<input type="file" name="upload"/><br/>
</form>
2、必须要设置input输入项的name属性,否则浏览器将不会发送上传文件的数据。
3、必须把form的enctype属值设为multipart/form-data.设置该值后,浏览器在上传文件时, method="post",将把文件数据附带在http请求消息体中,并使用MIME协议对上传的文件进行描述,以方便接收方对上传数据进行解析和处理。
4、使用Commons-fileupload组件实现文件上传,需要导入该组件相应的支撑jar包:Commons-fileupload和commons-io。commons-io 不属于文件上传组件的开发jar文件,但Commons-fileupload 组件从1.1 版本开始,它工作时需要commons-io包的支持。
二、文件的上传案例:
1、需要导入的文件:在WebRoot下新建文件夹common
(1)需要导入的jstl标签:tag.jsp
(2)需要引入jQuery easyui的js文件:jquery.jsp
(3) 需要创建的国际化资源的文件
csdn.properties

csdn_zh.properties
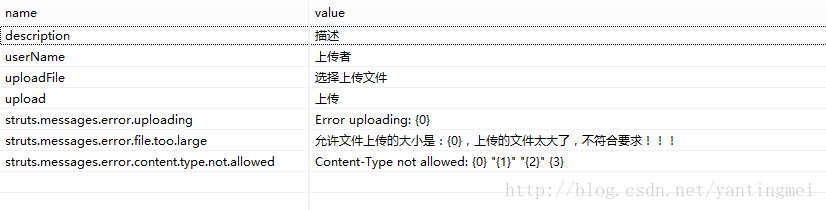
csdn_en.properties
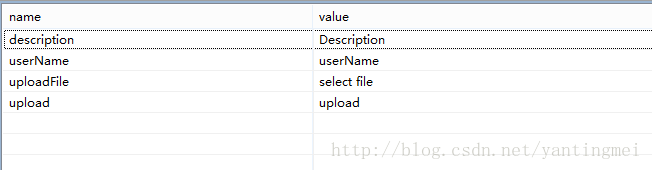
2、主页面:index.jsp
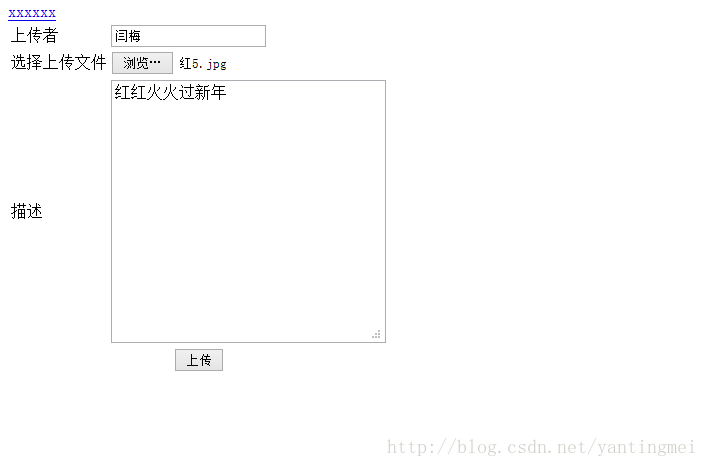
3.连接数据库
(1)db.properties
(2)Upload.java(封装)
(3)BaseDao.java接口
(4)继承BaseDao接口UploadDao.java
(5)工具包
DBConn.java
Pagination.java
(6)实现接口的类 UploadDaoImpl.java
四、文件上传在servlet的处理
UploadServlet.java
UploaadListServlet.java
web.xml
五、数据库查询页面
listfile.jsp
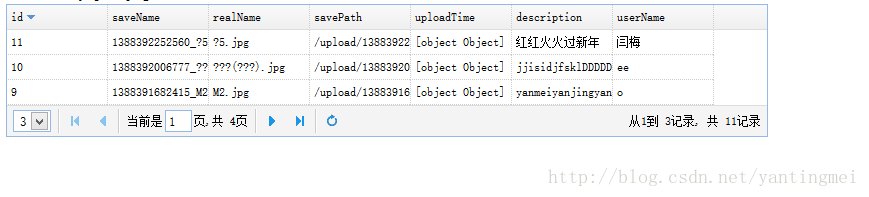
1、<form action="${pageContext.request.contextPath}/uploadFile.do" enctype="multipart/form-data" method="post">
选择文件:<input type="file" name="upload"/><br/>
</form>
2、必须要设置input输入项的name属性,否则浏览器将不会发送上传文件的数据。
3、必须把form的enctype属值设为multipart/form-data.设置该值后,浏览器在上传文件时, method="post",将把文件数据附带在http请求消息体中,并使用MIME协议对上传的文件进行描述,以方便接收方对上传数据进行解析和处理。
4、使用Commons-fileupload组件实现文件上传,需要导入该组件相应的支撑jar包:Commons-fileupload和commons-io。commons-io 不属于文件上传组件的开发jar文件,但Commons-fileupload 组件从1.1 版本开始,它工作时需要commons-io包的支持。
二、文件的上传案例:
1、需要导入的文件:在WebRoot下新建文件夹common
(1)需要导入的jstl标签:tag.jsp
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/functions" prefix="fn"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/fmt" prefix="fmt"%>
(2)需要引入jQuery easyui的js文件:jquery.jsp
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%> <% String path = request.getContextPath(); String basePath = request.getScheme() + "://" + request.getServerName() + ":" + request.getServerPort() + path + "/"; %> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <base href="<%=basePath%>"> <title>My JSP 'jquery.jsp' starting page</title> <meta http-equiv="pragma" content="no-cache"> <meta http-equiv="cache-control" content="no-cache"> <meta http-equiv="expires" content="0"> <meta http-equiv="keywords" content="keyword1,keyword2,keyword3"> <meta http-equiv="description" content="This is my page"> <!-- 引入jquery easyui的css样式 --> <link rel="stylesheet" type="text/css" href="${pageContext.request.contextPath}/themes/default/easyui.css"> <link rel="stylesheet" type="text/css" href="${pageContext.request.contextPath}/themes/icon.css"> <!-- 引入jQuery easyui的js文件 并且引入了jquery.js文件 --> <script type="text/javascript" src="${pageContext.request.contextPath}/js/jquery.min.js"></script> <script type="text/javascript" src="${pageContext.request.contextPath}/js/jquery.easyui.min.js"></script> </head> <body> </body> </html>
(3) 需要创建的国际化资源的文件
csdn.properties
csdn_zh.properties
csdn_en.properties
2、主页面:index.jsp
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%> <%@include file="/common/tag.jsp" %> <%@include file="/common/jquery.jsp" %> <fmt:setBundle basename="csdn"/> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <title>My JSP 'index.jsp' starting page</title> <meta http-equiv="pragma" content="no-cache"> <meta http-equiv="cache-control" content="no-cache"> <meta http-equiv="expires" content="0"> <meta http-equiv="keywords" content="keyword1,keyword2,keyword3"> <meta http-equiv="description" content="This is my page"> <!-- <link rel="stylesheet" type="text/css" href="styles.css"> --> </head> <body> <a href="${pageContext.request.contextPath}/uploadOper.do">xxxxxx</a> <c:if test="${!empty(requestScope.msg)}"> <fmt:message key="struts.messages.error.file.too.large"> <fmt:param value="${requestScope.msg }"/> </fmt:message> </c:if> <form action="${pageContext.request.contextPath}/uploadOper.do" enctype="multipart/form-data" method="post"> <table> <tr> <td><fmt:message key="userName"/></td> <td><input type="text" name="userName"/></td> </tr> <tr> <td><fmt:message key="uploadFile"/></td> <td><input type="file" name="uploadFile"/></td> </tr> <tr> <td><fmt:message key="description"/></td> <td><textarea rows="12" cols="30" name="description"></textarea></td> </tr> <tr align="center"> <td colspan="2"> <input type="hidden" name="oper" value="insert"/> <input type="submit" value='<fmt:message key="upload"/>'> </td> </tr> </table> </form> </body> </html>
3.连接数据库
(1)db.properties
url=jdbc\:mysql\://localhost\:3306/csdn?useUnicode\=true&characterEncoding\=UTF-8 user=root pass=root driverClassName=com.mysql.jdbc.Driver
(2)Upload.java(封装)
package www.csdn.net.upload.bean; import java.io.Serializable; import java.sql.Date; import java.sql.Timestamp; public class Upload implements Serializable { /** * */ private static final long serialVersionUID = 1L; private Integer id; private String saveName; private String realName; private String savePath; private Timestamp uploadTime; private String description; private String userName; public Upload() { super(); // TODO Auto-generated constructor stub } public Upload(Integer id, String saveName, String realName, String savePath, Timestamp uploadTime, String description, String userName) { super(); this.id = id; this.saveName = saveName; this.realName = realName; this.savePath = savePath; this.uploadTime = uploadTime; this.description = description; this.userName = userName; } public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getSaveName() { return saveName; } public void setSaveName(String saveName) { this.saveName = saveName; } public String getRealName() { return realName; } public void setRealName(String realName) { this.realName = realName; } public String getSavePath() { return savePath; } public void setSavePath(String savePath) { this.savePath = savePath; } public Timestamp getUploadTime() { return uploadTime; } public void setUploadTime(Timestamp uploadTime) { this.uploadTime = uploadTime; } public String getDescription() { return description; } public void setDescription(String description) { this.description = description; } public String getUserName() { return userName; } public void setUserName(String userName) { this.userName = userName; } @Override public String toString() { return "Upload [id=" + id + ", saveName=" + saveName + ", realName=" + realName + ", savePath=" + savePath + ", uploadTime=" + uploadTime + ", description=" + description + ", userName=" + userName + "]"; } }
(3)BaseDao.java接口
package www.csdn.net.upload.dao; import java.util.List; public interface BaseDao<T, PK> { T findById(PK id); void deleteById(PK id) throws Exception; void delete(T entity) throws Exception; void deletes(String ids[]) throws Exception; void update(T entity) throws Exception; void insert(T entity) throws Exception; List<T> findAll(); List<T> findNowPageInfo(int starIndex,int endIndex,String sortName,String sortOrder); }
(4)继承BaseDao接口UploadDao.java
package www.csdn.net.upload.dao; import www.csdn.net.upload.bean.Upload; public interface UploadDao extends BaseDao<Upload, Integer> { }
(5)工具包
DBConn.java
package www.csdn.net.uploadutil; import java.io.InputStream; import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.util.Properties; public class DBConn { private static Connection conn; private DBConn() { } public static Connection getConn() { try { if (conn == null) { // 创建集合对象 Properties properties = new Properties(); // 装载 properties.load(DBConn.class.getClassLoader() .getResourceAsStream("db.properties")); // 加载驱动程序 Class.forName(properties.getProperty("driverClassName")); // 获取连接对象 conn = DriverManager.getConnection( properties.getProperty("url"), properties.getProperty("user"), properties.getProperty("pass")); // 修改事务 为手动提交方式 conn.setAutoCommit(false); } } catch (Exception e) { e.printStackTrace(); } return conn; } public static void update(String sql, Object params[], PreparedStatement pstmt) throws Exception { try { pstmt = getConn().prepareStatement(sql); for (int i = 0; i < params.length; i++) { pstmt.setObject(i + 1, params[i]); } pstmt.executeUpdate(); conn.commit(); } catch (Exception e) { conn.rollback(); e.printStackTrace(); } finally { release(null, pstmt); } } public static void release(ResultSet rs, PreparedStatement pstmt) { if (rs != null) { try { rs.close(); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } if (pstmt != null) { try { pstmt.close(); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } }
Pagination.java
package www.csdn.net.uploadutil; import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.util.List; public class Pagination<T> { public static final Integer PAGESIZE=3; private Integer pageSize;// 每页显示的记录数 private Integer nowPage; // 当前页 private Integer total;// 总记录数 private Integer countPage;// 总页数 // 数据库中开始记录数和结束记录数 private Integer startIndex;// 开始记录数 private Integer endIndex;// 结束记录数 // 封装的每页显示的记录 private List<T> rows; public Pagination(Integer nowPage, Integer pageSize, String table) { // 当前页 this.nowPage = nowPage; this.pageSize = pageSize; // 判断当前页是否小于1 if (this.nowPage < 1) { this.nowPage = 1; } // 获取总记录数 this.total = this.selectCountSize(table); // 计算总页数 this.countPage = this.total % this.pageSize == 0 ? this.total / this.pageSize : this.total / this.pageSize + 1; // 判断当前页是否大于等于总页数 if (this.nowPage >= this.countPage) { this.nowPage = this.countPage; } // 计算开始的记录数和结束的记录数 // select * from table limit ?,? this.startIndex = (this.nowPage - 1) * this.pageSize; this.endIndex = this.pageSize; } public Integer getStartIndex() { return startIndex; } public Integer getEndIndex() { return endIndex; } public List<T> getRows() { return rows; } public void setRows(List<T> rows) { this.rows = rows; } /** * 获取总记录数 * * @param table * @return */ public Integer selectCountSize(String table) { int countSize = 0; String sql = "select count(*) as c from " + table; Connection conn = DBConn.getConn(); PreparedStatement pstmt = null; ResultSet rs = null; try { pstmt = conn.prepareStatement(sql); rs = pstmt.executeQuery(); if (rs.next()) { countSize = rs.getInt("c"); } } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } finally { DBConn.release(rs, pstmt); } return countSize; } public Integer getTotal() { return total; } }
(6)实现接口的类 UploadDaoImpl.java
package www.csdn.net.upload.dao.impl; import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.util.ArrayList; import java.util.List; import www.csdn.net.upload.bean.NewsType; import www.csdn.net.upload.bean.Upload; import www.csdn.net.upload.dao.UploadDao; import www.csdn.net.uploadutil.DBConn; public class UploadDaoImpl implements UploadDao{ private Connection conn; private PreparedStatement pstmt; private ResultSet rs; @Override public Upload findById(Integer id) { return null; } @Override public void deleteById(Integer id) throws Exception { } @Override public void delete(Upload entity) throws Exception { } @Override public void deletes(String[] ids) throws Exception { } @Override public void update(Upload entity) throws Exception { } @Override public void insert(Upload entity) throws Exception { String sql="insert into upload(saveName,realName,savePath,uploadTime,description,userName) values(?,?,?,?,?,?)"; DBConn.update(sql,new Object[]{entity.getSaveName(),entity.getRealName(),entity.getSavePath(),entity.getUploadTime(),entity.getDescription(),entity.getUserName()}, pstmt); } @Override public List<Upload> findAll() { List<Upload> entitys=new ArrayList<Upload>(); String sql="select id,saveName,realName,savePath,uploadTime,description,userName from upload"; conn=DBConn.getConn(); try { pstmt=conn.prepareStatement(sql); rs=pstmt.executeQuery(); while(rs.next()){ Upload upload=new Upload(); upload.setId(rs.getInt("id")); upload.setSaveName(rs.getString("saveName")); upload.setRealName(rs.getString("realName")); upload.setSavePath(rs.getString("savePath")); upload.setUploadTime(null); upload.setDescription(rs.getString("description")); upload.setUserName(rs.getString("userName")); entitys.add(upload); } } catch (SQLException e) { e.printStackTrace(); }finally{ DBConn.release(rs, pstmt); } return entitys; } @Override public List<Upload> findNowPageInfo(int starIndex, int endIndex, String sortName, String sortOrder) { List<Upload> entities=new ArrayList<Upload>(); String sql=""; if(sortName==null||sortOrder==null){ sql="select id,saveName,realName,savePath,uploadTime,description,userName from upload limit ?,?"; }else{ sql="select id,saveName,realName,savePath,uploadTime,description,userName from upload order by "+sortName+" "+sortOrder+" limit ?,? "; } conn=DBConn.getConn(); try { pstmt=conn.prepareStatement(sql); int index=1; pstmt.setInt(index++,starIndex); pstmt.setInt(index++, endIndex); rs=pstmt.executeQuery(); while(rs.next()){ Upload upload=new Upload(); upload.setId(rs.getInt("id")); upload.setSaveName(rs.getString("saveName")); upload.setRealName(rs.getString("realName")); upload.setSavePath(rs.getString("savePath")); upload.setUploadTime(rs.getTimestamp("uploadTime")); upload.setDescription(rs.getString("description")); upload.setUserName(rs.getString("userName")); entities.add(upload); } } catch (SQLException e) { e.printStackTrace(); }finally{ DBConn.release(rs, pstmt); } return entities; } }
四、文件上传在servlet的处理
UploadServlet.java
package www.csdn.net.upload.servlet; import java.io.File; import java.io.FileOutputStream; import java.io.IOException; import java.io.InputStream; import java.io.PrintWriter; import java.lang.reflect.InvocationTargetException; import java.sql.Timestamp; import java.util.List; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.apache.commons.beanutils.BeanUtils; import org.apache.commons.fileupload.FileItem; import org.apache.commons.fileupload.FileUploadException; import org.apache.commons.fileupload.ProgressListener; import org.apache.commons.fileupload.disk.DiskFileItemFactory; import org.apache.commons.fileupload.servlet.ServletFileUpload; import www.csdn.net.upload.bean.Upload; import www.csdn.net.upload.dao.UploadDao; import www.csdn.net.upload.dao.impl.UploadDaoImpl; public class UploadServlet extends HttpServlet { private UploadDao uploadDao=new UploadDaoImpl(); private String savePath="/upload"; public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // 判断请求的表单提交方式 if (ServletFileUpload.isMultipartContent(request)) { // 创建工厂 对象 DiskFileItemFactory diskFileItemFactory = new DiskFileItemFactory(); // 判断存储路径是否存在 File tempDir = new File(request.getServletContext() .getRealPath("/"), "temp"); if (!tempDir.exists()) { tempDir.mkdir(); } // 设置上传 临时文件的保存路径 diskFileItemFactory.setRepository(tempDir); // 创建解析器 ServletFileUpload fileUpload = new ServletFileUpload( diskFileItemFactory); // 设置上传的编码 fileUpload.setHeaderEncoding("UTF-8"); // 设置文件上传的大小的限制为 1M fileUpload.setFileSizeMax(1024 * 1024); // 注册监听 已经上传的进度 fileUpload.setProgressListener(new ProgressListener() { @Override public void update(long pBytesRead, long pContentLength, int pItems) { System.out.println("到现在为止," + pBytesRead + "字节已上传,总大小为" + pContentLength + "---" + pItems); } }); // 解析request请求 try { List<FileItem> fileItems = fileUpload.parseRequest(request); //创建实体对象 Upload entity=new Upload(); // 遍历操作 for (FileItem fileItem : fileItems) { // 判断是否是普通文本 if (fileItem.isFormField()) { // 获取字段name值 String name = fileItem.getFieldName(); String value = fileItem.getString(); //通过BeanUtils框架 设置jsp页面中传递的 userName \description BeanUtils.setProperty(entity, name, value); } else { // 获取上传文件的名称 String fileName = fileItem.getName(); // 浏览器 int index = fileName.lastIndexOf("\\"); if (index != -1) { fileName = fileName.substring(index + 1); } //设置真实的名称 entity.setRealName(fileName); // 添加时间戳 fileName = System.currentTimeMillis() + "_" + fileName; //设置保存的名称 entity.setSaveName(fileName); // 设置保存文件路径 String path = request.getServletContext().getRealPath( savePath); // 创建保存文件 File file = new File(path, fileName); //设置保存的路径 entity.setSavePath(savePath+"/"+fileName); // 获取请求的输入流 InputStream is = fileItem.getInputStream(); // 输出流对象 FileOutputStream fos = new FileOutputStream(file); byte[] buffer = new byte[1024]; int len = 0; while ((len = is.read(buffer)) != -1) { // 写入文件 fos.write(buffer, 0, len); } fos.close(); is.close(); // 删除临时文件 fileItem.delete(); } } //设置保存的时间 entity.setUploadTime(new Timestamp(System.currentTimeMillis())); uploadDao.insert(entity); // 转发 request.getRequestDispatcher("/listfile.jsp") .forward(request, response); } catch (FileUploadException e) { //获取错误的异常信息 String message=e.getMessage(); if(message.contains("permitted size of")){ request.setAttribute("msg","1M"); } //转发页面 request.getRequestDispatcher("./index.jsp").forward(request, response); e.printStackTrace(); } catch (IllegalAccessException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (Exception e) { // TODO Auto-generated catch block e.printStackTrace(); } } else { } } public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { this.doGet(request, response); } }
UploaadListServlet.java
package www.csdn.net.upload.servlet; import java.io.IOException; import java.io.PrintWriter; import java.util.List; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import net.sf.json.JSONObject; import www.csdn.net.upload.bean.Upload; import www.csdn.net.upload.dao.UploadDao; import www.csdn.net.upload.dao.impl.UploadDaoImpl; import www.csdn.net.uploadutil.Pagination; public class UploaadListServlet extends HttpServlet { private UploadDao uploadDao=new UploadDaoImpl(); public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { /* List<Upload> entities=uploadDao.findAll(); request.setAttribute("entities",entities); request.getRequestDispatcher("./listfile.jsp").forward(request, response); */ request.setCharacterEncoding("UTF-8"); String page=request.getParameter("page"); String rows=request.getParameter("rows"); String sortName=request.getParameter("sort"); String sortOrder=request.getParameter("order"); Integer nowPage=null; Integer pageSize=null; if(page!=null){ nowPage=Integer.valueOf(page); }else{ nowPage=1; } if(rows!=null){ pageSize=Integer.valueOf(rows); }else{ pageSize=Pagination.PAGESIZE; } //创建分页封装的对象 Pagination<Upload> pagination=new Pagination<Upload>(nowPage, pageSize, "upload"); List<Upload> entities=uploadDao.findNowPageInfo(pagination.getStartIndex(), pagination.getEndIndex(), sortName, sortOrder); pagination.setRows(entities); JSONObject jsonObject=JSONObject.fromObject(pagination); response.setContentType("application/json"); PrintWriter out=response.getWriter(); out.write(jsonObject.toString()); out.close(); } public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { this.doGet(request, response); } }
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>jqueryeasyui</display-name> <servlet> <servlet-name>UploadServlet</servlet-name> <servlet-class>www.csdn.net.upload.servlet.UploadServlet</servlet-class> </servlet> <servlet> <servlet-name>UploaadListServlet</servlet-name> <servlet-class>www.csdn.net.upload.servlet.UploaadListServlet</servlet-class> </servlet> <servlet> <servlet-name>DownFileServlet</servlet-name> <servlet-class>www.csdn.net.upload.servlet.DownFileServlet</servlet-class> </servlet> <servlet> <servlet-name>NewsTypeServlet</servlet-name> <servlet-class>www.csdn.net.upload.servlet.NewsTypeServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>UploadServlet</servlet-name> <url-pattern>/uploadOper.do</url-pattern> </servlet-mapping> <servlet-mapping> <servlet-name>UploaadListServlet</servlet-name> <url-pattern>/uploadList.do</url-pattern> </servlet-mapping> <servlet-mapping> <servlet-name>DownFileServlet</servlet-name> <url-pattern>/downFile.do</url-pattern> </servlet-mapping> <servlet-mapping> <servlet-name>NewsTypeServlet</servlet-name> <url-pattern>/newstype.do</url-pattern> </servlet-mapping> <welcome-file-list> <welcome-file>index.html</welcome-file> <welcome-file>index.htm</welcome-file> <welcome-file>index.jsp</welcome-file> <welcome-file>default.html</welcome-file> <welcome-file>default.htm</welcome-file> <welcome-file>default.jsp</welcome-file> </welcome-file-list> </web-app>
五、数据库查询页面
listfile.jsp
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%> <%@include file="/common/tag.jsp"%> <%@include file="/common/jquery.jsp"%> <fmt:setBundle basename="csdn" /> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <title>My JSP 'index.jsp' starting page</title> <meta http-equiv="pragma" content="no-cache"> <meta http-equiv="cache-control" content="no-cache"> <meta http-equiv="expires" content="0"> <meta http-equiv="keywords" content="keyword1,keyword2,keyword3"> <meta http-equiv="description" content="This is my page"> <!-- <link rel="stylesheet" type="text/css" href="styles.css"> --> <script type="text/javascript"> $(document).ready(function() { $("#dg").datagrid({ url : "./uploadList.do", sortName : "id", sortOrder : "desc", columns : [ [ //id,saveName,realName,savePath,uploadTime,description,userName { field : "id", title : "id", width : 100, sortable : true }, { field : "saveName", title : "saveName", width : 100 }, { field : "realName", title : "realName", width : 100 }, { field : "savePath", title : "savePath", width : 100 }, { field : "uploadTime", title : "uploadTime", width : 100 }, { field : "description", title : "description", width : 100 }, { field : "userName", title : "userName", width : 100 } ] ], pageSize : 3, pageList : [ 3, 5, 8 ], pagination : true }); $("#dg").datagrid("getPager").pagination({ beforePageText : "当前是", afterPageText : "页,共 {pages}页", displayMsg : "从{from}到 {to}记录, 共 {total}记录" }); }); </script> </head> <body> <%-- <table align="center" border="1px" cellpadding="0" cellspacing="0"> <tr> <td>序号</td> <td>文件名</td> <td>上传者</td> <td>上传描述</td> <td>上传时间</td> </tr> <c:forEach var="entity" items="${requestScope.entities}"> <tr> <td>${entity.id}</td> <td><c:url var="downUrl" value="/downFile.do"> <c:param name="filePath" value="${entity.savePath}"></c:param> <c:param name="fileName" value="${entity.realName}"></c:param> </c:url> <a href="${downUrl}">${entity.realName}</a></td> <td>${entity.userName}</td> <td><c:choose> <c:when test="${fn:length(entity.description) gt 4}"> ${fn:replace(entity.description,fn:substring(entity.description,4,fn:length(entity.description)),".....")} </c:when> <c:otherwise> ${entity.description} </c:otherwise> </c:choose></td> <td><fmt:formatDate value="${entity.uploadTime}" type="both" /> </td> </tr> </c:forEach> </table> --%> <div align="center"> <table id="dg"></table> </div> </body> </html>
相关文章推荐
- Java使用Commons-FileUpload组件实现文件上传最佳方案
- 使用commons-fileupload组件实现文件上传
- JSP使用commons-fileupload组件实现文件上传代码示例
- 使用commons-fileupload-1.2.1.jar等组件实现文件上传
- 使用commons-fileupload-1.2.1.jar等组件实现文件上传
- 使用Apache的commons-fileupload和commmons-io组件实现文件上传
- 使用Commons-fileupload组件实现文件上传的注意细节
- 使用Commons-fileupload组件实现文件上传
- 使用Commons-fileupload组件实现文件上传
- java组件commons-fileupload实现文件上传
- 《Java》----详解用apache的commons-fileupload组件实现文件上传
- 使用FileUpload组件实现文件上传
- 使用fileupload组件实现文件上传
- 用Commons-FileUpload组件实现文件上传
- 使用commons-fileupload组件上传下载文件
- 使用commons-fileUpload组件上传文件
- JSP组件commons-fileupload实现文件上传
- Jsp 使用commons-fileupload实现文件上传
- 使用fileupload组件实现文件上传
- 使用Commons-fileupload组件实现文件下载