Android应用程序组件之Service
2013-12-11 16:04
477 查看
本节讲述Android应用程序组件中的Service。所谓Service就是在后台执行且没有提供用户界面的组件,即使用户切换到其他应用程序,service仍然在后台运行不受影响。通常service被用来在后台执行耗时的操作,比如网络通信、播放音乐、执行文件I/O、与content provider交互等。
Service有两种类型,分为启动(started)和绑定(bound)两种。启动service由startService()完成,一旦service启动,它能够一直在后台运行即使启动它的组件已经被销毁,通常被用来执行单独的操作且并不返回结果给调用者。而绑定service是由bindService()完成,它的作用在于能够让组件之间进行交互,发送请求、获取结果以及完成进程间通信的任务,这种service在其他组件绑定它时会一直运行,直到所有组件取消绑定才销毁。当然,你也可以在实现service时支持这两种模式,仅仅需要你实现对应的回调函数即可:onStartCommand()和onBind()。
注意,service运行在进程的主线程中,并不会创建新的线程也不会运行在单独的进程中(除非你指定)。意味着如果你的service要完成CPU密集型的工作或阻塞的操作,那么你应该在service中创建新的线程来完成,这样可以降低ANR(应用程序无法响应)的风险,同时主线程仍然用来用户界面交互。
onStartCommand():调用startService()时系统会调用onStartCommand()方法,一旦该方法执行,service便已经启动且能在后台运行。如果实现了该方法,需要程序员自行调用stopSelf()或stopService()来停止服务。
onBind():调用bindService()时系统会调用onBind()方法,在该方法的实现中需要返回一个IBinder,为客户端与service通信提供接口。该方法必须要实现,如果不需要进行绑定则返回null。
onCreate():在service第一次创建时会调用onCreate()方法,执行一次性的设置操作,当服务已经在运行时,该方法不再被调用。
onDestroy():当service被销毁时,需要实现onDestroy()方法来清除所有资源,如线程、注册的监听器等。
与activity类似,service组件也需要在AndroidManifest.xml中进行声明,在<application>元素中添加<service>子标签,如下:
创建started service可以继承Service或IntentService。其中,Service类是所有service的基类,继承Service类需要在service中创建新的线程来完成任务,因为它使用应用程序的主线程。IntentService类是Service类的子类,它使用工作线程依次处理所有启动请求,如果不需要同时处理多个请求,这种方式会是最好的选择,你需要做的只是实现onHandleIntent()方法,用来接收所有请求的intent从而完成相应工作。
IntentService完成了以下工作:
(1)默认创建一个工作线程来执行所有传入onStartCommand()的intent请求;
(2)创建一个工作队列,它会每次向onHandleIntent()传入一个intent,这样就不用担心多线程的问题;
(3)在所有请求被处理完成后,停止service,因此你不需要自行调用stopSelf();
(4)默认提供的onBind()实现会返回null;
(5)默认提供的onStartCommand()会将intent传向工作队列,然后传向你所实现的onHandleIntent()。
总之,你只需要实现onHandleIntent(),可能还需要实现构造函数,参考下面的例子:
注意:如果要重写IntentService类的其他方法(onBind()除外),如onCreate()、onStartCommand()或onDestroy(),那么一定要调用父类的实现,这样IntentService才能正常执行工作线程的生命周期。如重写onStartCommand()方法:
START_NOT_STICKY:在onStartCommand()返回后系统杀死service时不会重建service,除非有pending intent要发送。避免在不必要时运行service,应用程序可以随时重启任何未完成的任务。
START_STICKY:在onStartCommand()返回后系统杀死service时会重建service并调用onStartCommand(),但不会重新传送上次的intent。在这种情况下,onStartCommand()接受的intent为null,除非有pending intent要启动service。它适用于媒体播放器或类似服务,不执行命令,但会等待任务。
START_REDELIVER_INTENT:在onStartCommand()返回后系统杀死service时会重建service并调用onStartCommand(),传入上次的intent,任何pending intent会轮流发送。它适用于需要立即重启的任务,如下载文件。
如果希望service返回结果,创建PendingIntent传入startService(),service会使用广播来发送结果。
当service同时处理多个启动请求时,你不能在处理完一个启动请求后停止service,因为有可能已经接收到了一个新的启动请求。为了避免这个问题,可以调用stopSelf(int)方法,它会匹配最新请求的ID,保证所有请求处理完毕后停止service。
请求service运行在前台,要调用startForeground(),该方法有两个参数:一个int值唯一标识notification(不能为0),还有一个状态栏的Notification,例如:
鉴于service有started和bound两种,简单回顾一下:
started service:其他组件调用startService()时创建service,此后service会一直运行,直到自己调用stopSelf()或者其他组件调用stopService()停止service,系统最终会销毁service。
bound service:其他组件调用bindService()时创建service,客户端通过IBinder来与service进行通信,调用unbindService()可以关闭与service之间的连接,当所有组件解除与service之间的绑定时,系统会销毁service。
这两者也不是完全分离的,你可以绑定已经由startService()启动的service。例如,后台音乐服务可以由startService()启动,intent指定要播放的音乐,接下来如果用户要对播放器进行控制或者获取当前歌曲信息,该activity可以调用bindService()绑定到service上。在这种情况下,stopService()和stopSelf()并不能停止服务,只有等到所有客户端解除绑定才会停止服务。
与activity类似,service也有一系列生命周期回调函数,实现这些函数能够监听service的状态并在适当的时候执行任务,下面的例子给出了这些回调函数:
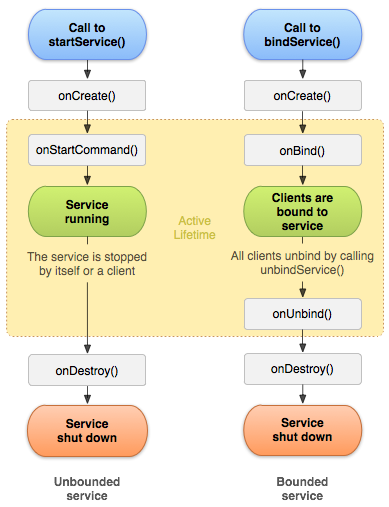
如上图为service的生命周期图。左边表示调用startService()的生命周期,右边表示调用bindService()的生命周期。
参考资料:Google ADT doc
Except as noted, this content is licensed
under Creative
Commons Attribution 2.5.
Service有两种类型,分为启动(started)和绑定(bound)两种。启动service由startService()完成,一旦service启动,它能够一直在后台运行即使启动它的组件已经被销毁,通常被用来执行单独的操作且并不返回结果给调用者。而绑定service是由bindService()完成,它的作用在于能够让组件之间进行交互,发送请求、获取结果以及完成进程间通信的任务,这种service在其他组件绑定它时会一直运行,直到所有组件取消绑定才销毁。当然,你也可以在实现service时支持这两种模式,仅仅需要你实现对应的回调函数即可:onStartCommand()和onBind()。
注意,service运行在进程的主线程中,并不会创建新的线程也不会运行在单独的进程中(除非你指定)。意味着如果你的service要完成CPU密集型的工作或阻塞的操作,那么你应该在service中创建新的线程来完成,这样可以降低ANR(应用程序无法响应)的风险,同时主线程仍然用来用户界面交互。
创建service
创建service必须创建Service子类,在实现中要重写一些关键的回调函数,如onStartCommand()、onBind()、onCreate()和onDestroy(),附上简要介绍:onStartCommand():调用startService()时系统会调用onStartCommand()方法,一旦该方法执行,service便已经启动且能在后台运行。如果实现了该方法,需要程序员自行调用stopSelf()或stopService()来停止服务。
onBind():调用bindService()时系统会调用onBind()方法,在该方法的实现中需要返回一个IBinder,为客户端与service通信提供接口。该方法必须要实现,如果不需要进行绑定则返回null。
onCreate():在service第一次创建时会调用onCreate()方法,执行一次性的设置操作,当服务已经在运行时,该方法不再被调用。
onDestroy():当service被销毁时,需要实现onDestroy()方法来清除所有资源,如线程、注册的监听器等。
与activity类似,service组件也需要在AndroidManifest.xml中进行声明,在<application>元素中添加<service>子标签,如下:
<manifest ... > ... <application ... > <service android:name=".ExampleService" /> ... </application> </manifest>同样,启动service需要使用Intent,也支持显式(需要指定service类名)和隐式(需要指定<intent-filter>)两种方式。
创建started service可以继承Service或IntentService。其中,Service类是所有service的基类,继承Service类需要在service中创建新的线程来完成任务,因为它使用应用程序的主线程。IntentService类是Service类的子类,它使用工作线程依次处理所有启动请求,如果不需要同时处理多个请求,这种方式会是最好的选择,你需要做的只是实现onHandleIntent()方法,用来接收所有请求的intent从而完成相应工作。
IntentService完成了以下工作:
(1)默认创建一个工作线程来执行所有传入onStartCommand()的intent请求;
(2)创建一个工作队列,它会每次向onHandleIntent()传入一个intent,这样就不用担心多线程的问题;
(3)在所有请求被处理完成后,停止service,因此你不需要自行调用stopSelf();
(4)默认提供的onBind()实现会返回null;
(5)默认提供的onStartCommand()会将intent传向工作队列,然后传向你所实现的onHandleIntent()。
总之,你只需要实现onHandleIntent(),可能还需要实现构造函数,参考下面的例子:
public class HelloIntentService extends IntentService { /** * A constructor is required, and must call the super IntentService(String) * constructor with a name for the worker thread. */ public HelloIntentService() { super("HelloIntentService"); } /** * The IntentService calls this method from the default worker thread with * the intent that started the service. When this method returns, IntentService * stops the service, as appropriate. */ @Override protected void onHandleIntent(Intent intent) { // Normally we would do some work here, like download a file. // For our sample, we just sleep for 5 seconds. long endTime = System.currentTimeMillis() + 5*1000; while (System.currentTimeMillis() < endTime) { synchronized (this) { try { wait(endTime - System.currentTimeMillis()); } catch (Exception e) { } } } } }
注意:如果要重写IntentService类的其他方法(onBind()除外),如onCreate()、onStartCommand()或onDestroy(),那么一定要调用父类的实现,这样IntentService才能正常执行工作线程的生命周期。如重写onStartCommand()方法:
@Override public int onStartCommand(Intent intent, int flags, int startId) { Toast.makeText(this, "service starting", Toast.LENGTH_SHORT).show(); return super.onStartCommand(intent,flags,startId); }相对继承IntentService而言,继承Service会稍微复杂的多,但它所处理的情况通常是执行多线程任务,参考下面的例子:
public class HelloService extends Service { private Looper mServiceLooper; private ServiceHandler mServiceHandler; // Handler that receives messages from the thread private final class ServiceHandler extends Handler { public ServiceHandler(Looper looper) { super(looper); } @Override public void handleMessage(Message msg) { // Normally we would do some work here, like download a file. // For our sample, we just sleep for 5 seconds. long endTime = System.currentTimeMillis() + 5*1000; while (System.currentTimeMillis() < endTime) { synchronized (this) { try { wait(endTime - System.currentTimeMillis()); } catch (Exception e) { } } } // Stop the service using the startId, so that we don't stop // the service in the middle of handling another job stopSelf(msg.arg1); } } @Override public void onCreate() { // Start up the thread running the service. Note that we create a // separate thread because the service normally runs in the process's // main thread, which we don't want to block. We also make it // background priority so CPU-intensive work will not disrupt our UI. HandlerThread thread = new HandlerThread("ServiceStartArguments", Process.THREAD_PRIORITY_BACKGROUND); thread.start(); // Get the HandlerThread's Looper and use it for our Handler mServiceLooper = thread.getLooper(); mServiceHandler = new ServiceHandler(mServiceLooper); } @Override public int onStartCommand(Intent intent, int flags, int startId) { Toast.makeText(this, "service starting", Toast.LENGTH_SHORT).show(); // For each start request, send a message to start a job and deliver the // start ID so we know which request we're stopping when we finish the job Message msg = mServiceHandler.obtainMessage(); msg.arg1 = startId; mServiceHandler.sendMessage(msg); // If we get killed, after returning from here, restart return START_STICKY; } @Override public IBinder onBind(Intent intent) { // We don't provide binding, so return null return null; } @Override public void onDestroy() { Toast.makeText(this, "service done", Toast.LENGTH_SHORT).show(); } }注意到onStartCommand()方法的返回值是int类型,文档上规定onStartCommand()方法只能返回如下常量中一个:
START_NOT_STICKY:在onStartCommand()返回后系统杀死service时不会重建service,除非有pending intent要发送。避免在不必要时运行service,应用程序可以随时重启任何未完成的任务。
START_STICKY:在onStartCommand()返回后系统杀死service时会重建service并调用onStartCommand(),但不会重新传送上次的intent。在这种情况下,onStartCommand()接受的intent为null,除非有pending intent要启动service。它适用于媒体播放器或类似服务,不执行命令,但会等待任务。
START_REDELIVER_INTENT:在onStartCommand()返回后系统杀死service时会重建service并调用onStartCommand(),传入上次的intent,任何pending intent会轮流发送。它适用于需要立即重启的任务,如下载文件。
启动Service
调用startService()并传入intent,如显式启动HelloService:Intent intent = new Intent(this, HelloService.class); startService(intent);这种情况下,startService()会立即返回,系统会调用onStartCommand(),如果service未启动会先调用onCreate(),再调用onStartCommand()。
如果希望service返回结果,创建PendingIntent传入startService(),service会使用广播来发送结果。
停止service
一个started service要维护它的生命周期。只有当系统需要回收内存时才会停止或销毁service,service在onStartCommand()返回后仍然保持运行状态。因此,service自身要调用stopSelf()停止,或其他组件调用stopService(),这样系统会尽快销毁service。当service同时处理多个启动请求时,你不能在处理完一个启动请求后停止service,因为有可能已经接收到了一个新的启动请求。为了避免这个问题,可以调用stopSelf(int)方法,它会匹配最新请求的ID,保证所有请求处理完毕后停止service。
创建Bound Service
bound service允许应用程序组件调用bindService()进行绑定,允许应用程序其他组件与service进行交互,或者通过IPC使应用程序功能暴露给其他应用程序。创建bound service要实现onBind()回调函数,它会返回IBinder对象,该对象定义了与service通信的接口。当客户端完成与service交互后,调用unbindService()解除绑定。由于创建Bound Service比创建Started Service更加复杂,后面会有单独的一篇博文予以介绍。在前台运行Service
前台service可以理解为用户所关心的,在内存紧缺时系统不会杀死的service。前台service必须在状态栏(status bar)提供一个notification,该notification不能够被移除,除非service停止或者从前台删除。请求service运行在前台,要调用startForeground(),该方法有两个参数:一个int值唯一标识notification(不能为0),还有一个状态栏的Notification,例如:
Notification notification = new Notification(R.drawable.icon, getText(R.string.ticker_text), System.currentTimeMillis()); Intent notificationIntent = new Intent(this, ExampleActivity.class); PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, notificationIntent, 0); notification.setLatestEventInfo(this, getText(R.string.notification_title), getText(R.string.notification_message), pendingIntent); startForeground(ONGOING_NOTIFICATION_ID, notification);从前台删除service,调用stopForeground(),该方法需要指定一个boolean值,表示是否要删除状态栏notification,该方法不会停止service。然而,如果service还在前台运行时停止了service,此时notification也会被删除。
Service生命周期
虽然Service生命周期比Activity生命周期要简单得多,但是还是值得我们关注,因为用户无法感知到service的存在,它会在后台运行。鉴于service有started和bound两种,简单回顾一下:
started service:其他组件调用startService()时创建service,此后service会一直运行,直到自己调用stopSelf()或者其他组件调用stopService()停止service,系统最终会销毁service。
bound service:其他组件调用bindService()时创建service,客户端通过IBinder来与service进行通信,调用unbindService()可以关闭与service之间的连接,当所有组件解除与service之间的绑定时,系统会销毁service。
这两者也不是完全分离的,你可以绑定已经由startService()启动的service。例如,后台音乐服务可以由startService()启动,intent指定要播放的音乐,接下来如果用户要对播放器进行控制或者获取当前歌曲信息,该activity可以调用bindService()绑定到service上。在这种情况下,stopService()和stopSelf()并不能停止服务,只有等到所有客户端解除绑定才会停止服务。
与activity类似,service也有一系列生命周期回调函数,实现这些函数能够监听service的状态并在适当的时候执行任务,下面的例子给出了这些回调函数:
public class ExampleService extends Service { int mStartMode; // indicates how to behave if the service is killed IBinder mBinder; // interface for clients that bind boolean mAllowRebind; // indicates whether onRebind should be used @Override public void onCreate() { // The service is being created } @Override public int onStartCommand(Intent intent, int flags, int startId) { // The service is starting, due to a call to startService() return mStartMode; } @Override public IBinder onBind(Intent intent) { // A client is binding to the service with bindService() return mBinder; } @Override public boolean onUnbind(Intent intent) { // All clients have unbound with unbindService() return mAllowRebind; } @Override public void onRebind(Intent intent) { // A client is binding to the service with bindService(), // after onUnbind() has already been called } @Override public void onDestroy() { // The service is no longer used and is being destroyed } }需要注意的是,与activity不同之处在于,在自定义的service中不允许调用父类的这些回调函数。
如上图为service的生命周期图。左边表示调用startService()的生命周期,右边表示调用bindService()的生命周期。
参考资料:Google ADT doc
Except as noted, this content is licensed
under Creative
Commons Attribution 2.5.
相关文章推荐
- Android应用程序四大组件之Service(一)
- Android应用程序四大组件之使用AIDL如何实现跨进程调用Service
- 第三部分:Android 应用程序接口指南---第一节:应用程序组件---第二章2-1.Bound Service
- Android 4大应用程序组件 之服务 (Service)
- Android应用程序四大组件之使用AIDL如何实现跨进程调用Service
- Android-Service组件
- Android 中Service组件
- Android中ActivityManagerService与应用程序(客户端)通信模型分析
- Android中ActivityManagerService与应用程序(客户端)通信模型分析
- Android四大组件----Service
- Android四大组件之Service(二)
- Android - day four 四大组件之 Service
- Android组件通讯之Service的基础绑定
- Android开发者确保应用程序运行的四大组件
- Android 组件Service(二)之Handler、AsyncTask异步消息
- Android四大组件之Activity、Service
- Android四大组件之Service的使用
- Service与Android系统实现(1)-- 应用程序里的Service
- Android开发总结笔记 四大组件之Service(中) 1-2-5