jsp+servlet 实现登录功能
2013-12-06 16:32
399 查看
现在的框架比较流行,为了更好的理解框架,javaweb的基本开发形式还需要了解。
下面是运用jsp+servlet技术是实现登录。(没有运用数据库,数据操作比较简单,这个例子主要描述开发流程)
project:jspServletLoginTest
项目的目录结构如下:
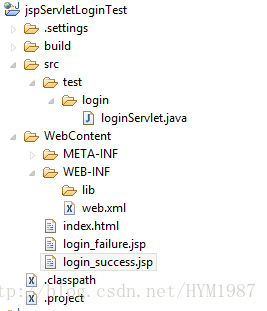
下面贴上代码:
index.html
login_success.jsp
login_failure.jsp
servlet类,表单的数据需要提交到这个类进行处理(页面跳转的控制类),若要深入理解Form的提交和处理原理,则需要深入研究web的HTTP协议
loginServlet.java
到目前为止,项目的主要的功能文件已经完成。但是还要在web.xml中注册项目运行的欢迎文件以及servlet的注册。(关于web.xml文件的工作原理有机会在补上了)
web.xml
如此就实现了jsp+servlet实现的用户登录功能。
下面是运用jsp+servlet技术是实现登录。(没有运用数据库,数据操作比较简单,这个例子主要描述开发流程)
project:jspServletLoginTest
项目的目录结构如下:
下面贴上代码:
index.html
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <!-- 该Login页面是一个简单的登录界面 --> <html > <head> <title>Login</title> <meta http-equiv= "content-type" content = "text/html; charset=UTF-8"> <meta http-equiv= "Content-Language" content = "ch-cn"> </head> <body > <!-- Form 用来提取用户填入并提交的信息--> <form method = "post" name = "frmLogin" action="loginServlet"> <h1 align = "center"> USER LOGIN</h1> <br > <div align = "center" > User name: <input type = "text" name = "txtUserName" value = "hym" size = "20" maxlength = "20" > <br> Password: <input type = "password" name = "txtPassword" value = "Your password" size = "20" maxlength = "20" > <br> <input type = "submit" name = "Submit" value= "submit" onClick = "validateLogin();" > <input type = "reset" name = "Reset" value = "reset" > <br> </div> </form> <!-- javaScript 函数 validateLogin(),null validate--> <script language = "javaScript"> function validateLogin( ) { var sUserName = document. frmLogin. txtUserName. value ; var sPassword = document. frmLogin. txtPassword. value ; if( sUserName= = "" ) { alert( "input user name!" ) ; return false; } if( sPassword= = "" ) { alert( "input password!" ) ; return false; } } </script> </body> </html>
login_success.jsp
<%@ page language= "java" contentType= "text/html; charset=UTF-8" pageEncoding= "UTF-8" %> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" > <html> <head> <title> My JSP 'login_failure.jsp' starting page </title> <meta http-equiv= "content-type" content = "text/html; charset=UTF-8"> <meta http-equiv= "pragma" content = "no-cache"> <meta http-equiv= "cache-control" content = "no-cache"> <meta http-equiv= "expires" content = "0"> <meta http-equiv= "keywords" content = "keyword1,keyword2,keyword3"> <meta http-equiv= "description" content = "This is my page"> </head> <body> <% String userName = ( String ) session. getAttribute ( "UserName" ) ; %> <div align = center> <%=userName%> welcome,logined! </div> </body> </html>
login_failure.jsp
<%@page language= "java" contentType= "text/html; charset=UTF-8" pageEncoding= "UTF-8" %> <! DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <title> My JSP 'login_failure.jsp' starting page </title> <meta http-equiv= "content-type" content = "text/html; charset=UTF-8"> <meta http-equiv= "pragma" content = "no-cache"> <meta http-equiv= "cache-control" content = "no-cache"> <meta http-equiv= "expires" content = "0"> <meta http-equiv= "keywords" content = "keyword1,keyword2,keyword3"> <meta http-equiv= "description" content = "This is my page"> </head> <body> <% String userName = ( String ) session. getAttribute ( "UserName" ) ; %> <div align = center> <%=userName%> failure access! </div> </body> </html>
servlet类,表单的数据需要提交到这个类进行处理(页面跳转的控制类),若要深入理解Form的提交和处理原理,则需要深入研究web的HTTP协议
loginServlet.java
package test.login; import java.io.IOException; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; public class loginServlet extends HttpServlet { private static final long serialVersionUID = 1L; public loginServlet() { super(); } protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { } protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response. setContentType ( "text/html" ) ; // get username String sUserName = request.getParameter("txtUserName") ; // get password String sPasswd = request.getParameter("txtPassword") ; //test System.out.println("username:"+sUserName+"\r\n"+"password:"+sPasswd); request . getSession( ).setAttribute ( "UserName" , sUserName ) ; request.getSession().setAttribute("password", sPasswd); //just compare with hym&123,not do database operation if(sUserName.equals("hym")&&sPasswd.equals("123")) { response. sendRedirect ("login_success.jsp") ; } else { response. sendRedirect ("login_failure.jsp") ; } } }
到目前为止,项目的主要的功能文件已经完成。但是还要在web.xml中注册项目运行的欢迎文件以及servlet的注册。(关于web.xml文件的工作原理有机会在补上了)
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app id="WebApp_ID" version="2.4" xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd"> <display-name>jspServletLoginTest</display-name> <servlet> <description> </description> <display-name>loginServlet</display-name> <servlet-name>loginServlet</servlet-name> <servlet-class> test.login.loginServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>loginServlet</servlet-name> <url-pattern>/loginServlet</url-pattern> </servlet-mapping> <!-- 通过如下的设置web project在tomcat服务器上运行的时候,可以首先启动和运行webcontent目录下面 相应的页面,会根据welcome-file的list去检索 --> <welcome-file-list> <welcome-file>index.html</welcome-file> <welcome-file>index.jsp</welcome-file> </welcome-file-list> </web-app>
如此就实现了jsp+servlet实现的用户登录功能。
相关文章推荐
- JSP+Servlet实现注册登录功能
- jsp+javaBean+servlet+mysql完整的实现一个登录功能
- JSP+JavaBean+Servlet技术实现某网站用户注册和登录功能
- JSP+Servlet培训班作业管理系统[1]-登录功能的简单实现
- JSP+Servlet培训班作业管理系统[9] -登录功能的实现
- jsp+servlet+mysql 实现简单的银行登录转账功能
- Jsp&Servlet 用户登录功能实现
- 利用JSP+JS+CSS+Servlet实现用户登录,保存用户名密码功能
- jsp+javabean+servlet+Mysql实现MVC模式下的注册登录留言功能
- JSP+Servlet+javabean实现登录功能模块
- JSP+Servlet实现登录功能.
- servlet+jsp+jdbc实现简单的登录功能(所用平台:win7+Eclipse+tomcat+mysql)
- 用jsp+servlet+jdbc实现登录功能(体现mvc设计思想)
- JavaWeb实现用户登录注册功能实例代码(基于Servlet+JSP+JavaBean模式)
- JSP+Servlet实现注册登录功能
- 一个简单的jsp+servlet实例,实现简单的登录
- JSP+SERVLET实现后登陆用户挤掉之前登录用户
- 一个简单的jsp+servlet实例,实现简单的登录
- jsp+servlet实现验证码功能
- Servlet简单实现的注册登录功能