添加、删除、数据绑定、登录、修改的实现
2013-11-30 20:25
525 查看
添加、删除、数据绑定、登录、修改是一个系统中最常见,最基本的操作。
这里用产品信息这个栗子(简单三层结构没有使用dbhlper)来分析添加、删除、数据绑定、修改操作。
用学生考试系统的学生登录栗子(简单三层机构使用了dbhlper)分析登录操作
一、添加
前台:
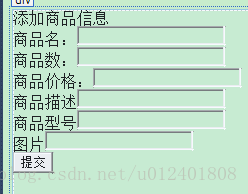
后台:
数据层代码
二、数据绑定、删除、修改
商品操作页面

商品修改

后台代码(商品操作页面和商品修改的后台代码):
数据层代码:
三、登录操作

后台代码:
数据层代码:
这里用产品信息这个栗子(简单三层结构没有使用dbhlper)来分析添加、删除、数据绑定、修改操作。
用学生考试系统的学生登录栗子(简单三层机构使用了dbhlper)分析登录操作
一、添加
前台:
后台:
protected void adButton_Click(object sender, EventArgs e) { Model.ProductInfo model = new Model.ProductInfo(); model.Product_Item = TextBox5.Text; model.Product_name = TextBox1.Text; model.Product_num = Int32.Parse(TextBox2.Text); model.Product_price = Decimal.Parse(TextBox3.Text); model.Product_Desc = TextBox4.Text; model.Product_Item = TextBox5.Text; model.Product_Pic = TextBox6.Text; bool result = new Bll.ProductBll().ProductAdd(model); if (result) Response.Write("<script language=\"javascript\">alert('添加成功')</script>"); else Response.Write("<script language=\"javascript\">alert('添加失败')</script>"); }
数据层代码
public bool ProductAdd(Model.ProductInfo model) { #region 产品添加的数据库操作 //存储过程,这里没有用到dbhlper int num = -1; SqlConnection myCon = new SqlConnection("server=BOYI-PC;database=ProductInforDB; User Id=sa; pwd=818181"); try { myCon.Open(); SqlCommand myCmd; string cmdText = "Insert into Product_Table(Product_name,Product_num,Product_price,Product_Desc,product_item,product_pic) values('" + model.Product_name + "'," + model.Product_num + "," + model.Product_price + ",'"+model.Product_Desc+"','"+model.Product_Item+"','"+model.Product_Pic+"')"; myCmd = new SqlCommand(cmdText, myCon); num = myCmd.ExecuteNonQuery(); //ExecuteNonQuery执行一个SQLCommand返回操作影响的行数,这个多半是判断操作是否成功的,例如插入删除和更新操作,如果影响的行数为0的话,则表示操作是不成功的,大于0证明操作成功。 } finally { myCon.Close(); } return num > 0; #endregion }
二、数据绑定、删除、修改
商品操作页面

商品修改

后台代码(商品操作页面和商品修改的后台代码):
namespace ProductInfor { public partial class MainPage : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) { BindListData(); //调用数据绑定函数BindListData } } /// /// 数据删除按钮事件 /// /// /// protected void proRepeater_ItemCommand(object source, RepeaterCommandEventArgs e) //CommandArgument获取命令的参数即在页面中绑定的PaperInfoId { int Del_Id = int.Parse(e.CommandArgument.ToString()); if (new Bll.ProductBll().ProductDel(Del_Id)) { Response.Write(""); BindListData(); } else { Response.Write(""); } } private void BindListData() { this.proRepeater.DataSource = new Bll.ProductBll().ProductList(); //调用函数,以指点repeater控件的数据。 this.proRepeater.DataBind(); //绑定数据 } protected void addButton_Click1(object sender, EventArgs e) { Response.Redirect("ProductAdd.aspx"); } } } namespace ProductInfor { public partial class ProductEdit : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) { if (Request.QueryString["Id"] == null) { Response.Write("没有参数"); Response.End(); } Model.ProductInfo model = new Bll.ProductBll().GetInfo(int.Parse(Request.QueryString["Id"])); //调用GetInfo方法 if (model == null) { Response.Write("没有参数"); } else { TextBox1.Text = model.Product_name; //将model的值赋给文本框的值 TextBox2.Text = model.Product_num.ToString(); TextBox3.Text = model.Product_price.ToString(); } } } protected void cfButton_Click(object sender, EventArgs e) { #region 修改产品信息按钮事件 Model.ProductInfo model = new Model.ProductInfo(); model.Product_Id = int.Parse(Request.QueryString["Id"]); model.Product_name = TextBox1.Text; model.Product_num = Int32.Parse(TextBox2.Text); model.Product_price = Decimal.Parse(TextBox3.Text); bool result = new Bll.ProductBll().ProductModify(model); //调用ProductModify方法 if (result) Page.Response.Write(""); else Response.Write(""); } #endregion } }
数据层代码:
public Model.ProductInfo GetInfo(int Id) { #region 在修改前获取产品信息 Model.ProductInfo model = null; SqlConnection myCon = new SqlConnection("server=BOYI-PC;database=ProductInforDB; User Id=sa; pwd=818181"); try { myCon.Open(); SqlCommand myCmd; string cmdText = "select * from Product_Table where Product_Id=" + Id; // Response.Write(cmdText); // Response.End(); myCmd = new SqlCommand(cmdText, myCon); SqlDataReader reader = myCmd.ExecuteReader(); while (reader.Read()) { model = new Model.ProductInfo(); model.Product_name = reader["Product_name"].ToString(); model.Product_num = int.Parse(reader["product_num"].ToString()); model.Product_price = decimal.Parse(reader["Product_price"].ToString()); model.Product_Desc = reader["Product_Desc"].ToString(); } read 4000 er.Close(); return model; } finally { myCon.Close(); } #endregion } public bool ProductModify(Model.ProductInfo model) { #region 产品信息修改的数据库操作 SqlConnection myCon = new SqlConnection("server=BOYI-PC;database=ProductInforDB; User Id=sa; pwd=818181"); try { myCon.Open(); SqlCommand myCmd; string cmdText = "Update Product_Table set Product_name ='" + model.Product_name + "',Product_num='" + model.Product_num + "',Product_price=" + model.Product_price + " where Product_Id=" + model.Product_Id; myCmd = new SqlCommand(cmdText, myCon); int num = myCmd.ExecuteNonQuery(); //ExecuteNonQuery执行一个SQLCommand返回操作影响的行数,这个多半是判断操作是否成功的,例如插入删除和更新操作,如果影响的行数为0的话,则表示操作是不成功的,大于0证明操作成功。 //ExecuteNonQuery执行一个SQLCommand返回操作影响的行数,这个多半是判断操作是否成功的,例如插入删除和更新操作,如果影响的行数为0的话,则表示操作是不成功的,大于0证明操作成功。 return num > 0; } finally { myCon.Close(); } #endregion } public DataTable ProductList() { #region 指定repeater的数据 SqlConnection myCon = new SqlConnection("server=BOYI-PC;database=ProductInforDB; User Id=sa; pwd=818181"); try { myCon.Open(); string cmdText = "select * from Product_Table"; SqlDataAdapter adapter = new SqlDataAdapter(cmdText,myCon); DataSet dataSet = new DataSet(); adapter.Fill(dataSet, "Result"); if (dataSet.Tables.Count > 0) { return dataSet.Tables["Result"]; } return null; } finally { myCon.Close(); } #endregion } public bool ProductDel(int Id) { #region 产品信息删除的数据库操作 SqlConnection myCon = new SqlConnection("server=BOYI-PC;database=ProductInforDB; User Id=sa; pwd=818181"); try { myCon.Open(); SqlCommand myCmd; string cmdText = "delete from Product_Table where Product_Id=" + Id; myCmd = new SqlCommand(cmdText, myCon); int num = myCmd.ExecuteNonQuery(); return num > 0; } finally { myCon.Close(); } #endregion }
三、登录操作

后台代码:
public void btnLogin_Click(object sender, EventArgs e) { string username = txtName.Text.Trim(); string password = txtPwd.Text.Trim(); #region 登录 STSModel.studentModel model = new STSModel.studentModel(); model = sdb.GetInfo(username); //调用GetInfo函数,判断如果model为空,则学生账号不存在;否则,再判断密码是否错误 if (model == null) { Response.Write(""); } else { if (model.stuPwd.ToString() != password) { Response.Write(""); } else { HttpCookie cookie = new HttpCookie("Stucookie"); //实列化cookie cookie.Values["StuName"] = model.stuName; //给cookie赋变量值 cookie.Values["StuId"] = model.stuId.ToString(); cookie.Expires = DateTime.Now.AddHours(1); //设置cookie的过期时间或时间 HttpContext.Current.Response.Cookies.Add(cookie); Response.Redirect("stuIndex.aspx"); //登录成功跳转到stuIndex页面 } } #endregion }
数据层代码:
public STSModel.studentModel GetInfo(string stuName) { #region GetInfo函数,在后台调用时用来提取学生id、学生姓名、学生密码的值 string cmdText = "SELECT * FROM TE_student WHERE stuName='" + stuName + "'"; DataTable dt = DbHelper.ExecuteTable(DbHelper.ConnectionString, CommandType.Text, cmdText, null); STSModel.studentModel model = null; if (dt.Rows.Count > 0) { model = new STSModel.studentModel(); model.stuId = int.Parse(dt.Rows[0]["stuId"].ToString()); model.stuName = dt.Rows[0]["stuName"].ToString(); model.stuPwd = dt.Rows[0]["stuPwd"].ToString(); return model; } // dr.Close(); return model; #endregion }
相关文章推荐
- C#实现SQL数据库中的表的查询、添加、修改、删除数据
- 稳扎稳打Silverlight(58) - 4.0通信之WCF RIA Services: 通过 Domain Service, 以 MVVM 模式实现数据的添加、删除、修改和查询
- 稳扎稳打Silverlight(58) - 4.0通信之WCF RIA Services: 通过 Domain Service, 以 MVVM 模式实现数据的添加、删除、修改和查询
- php处理登录、添加数据、删除数据和修改数据
- 用Nhibernate怎么实现数据的添加、删除、修改简单程序
- C#实现对数据库中的表的查询、添加、修改、删除数据
- 稳扎稳打Silverlight(58) - 4.0通信之WCF RIA Services: 通过 Domain Service, 以 MVVM 模式实现数据的添加、删除、修改和查询
- ORM框架实现数据的自动绑定添加修改
- 稳扎稳打Silverlight(58) - 4.0通信之WCF RIA Services: 通过 Domain Service, 以 MVVM 模式实现数据的添加、删除、修改和查询
- 七、SQL数据库,代码实现创建表,添加列,修改数据,删除数据
- php+mysqli预处理技术实现添加、修改及删除多条数据的方法
- 稳扎稳打Silverlight(58) - 4.0通信之WCF RIA Services: 通过 Domain Service, 以 MVVM 模式实现数据的添加、删除、修改和查询
- 实现页面无刷新,在gridview中添加,删除,修改数据
- [导入]ORM框架实现数据的自动绑定添加修改
- ORM框架实现数据的自动绑定添加修改
- ADO.NET使用带参数方法实现添加、删除和修改数据
- 用Nhibernate怎么实现数据的添加、删除、修改简单程序
- Hibernate jap 配置 Persistence 注解实现创建表添加修改删除 时态数据
- 用Nhibernate实现数据的添加、删除、修改
- 稳扎稳打Silverlight(58) - 4.0通信之WCF RIA Services: 通过 Domain Service, 以 MVVM 模式实现数据的添加、删除、修改和查询