Android UI系列:TextView显示文本
2013-10-15 22:09
309 查看
Android下有众多的Widget,而TextView可以说是最基础的,或者说是最基本的的控件。和其它编程语言相同的label标签一样,一般用来显示固定长度的文本字符串。
一、实例
在介绍前先演示一下实例
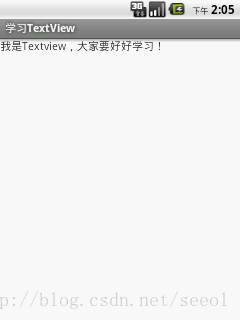
效果图一如上
布局文件text_view.xml
java代码TextViewActivity.java
再在AndroidManifest.xml配置一下
其中用的字符串资源是
<string name="textView_content">我是Textview,大家要好好学习!</string>
↳ android.view.View
↳ android.widget.TextView
直接子类
Button, CheckedTextView, Chronometer, DigitalClock, EditText, TextClock
间接子类
AutoCompleteTextView, CheckBox, CompoundButton, ExtractEditText, MultiAutoCompleteTextView, RadioButton, Switch, ToggleButton
Button,EditText,CheckBox,RadioButton,ToggleButton 等都是它的直接子类或间接子类,所以我们要一定学好TextView,才能更好地学习其它控件。
三、TextView属性
四、能过代码方式建立TextView
TextView可以通过在布局文件进行设置,也可以通过代码生成
运行的效果和图一完全一样
五、走马灯效果
加上以上属性就可以实现走马灯的效果了
注意:
1.要单行,如果没有设置单行,多行可以全部显示出来,是没有走马灯的效果的
2.设置为焦点
3没有滚动球的设备 android:focusableInTouchMode="true"属性是必须的
4.如果内容一行就可以显示出来的,请重复一次内容,如果重复后还是可以一行显示的,请再重复,如
闪烁效果
别人的走马灯效果要闪烁一下,那我们也来实现
以上的效果是半秒就改变一下字体的颜色,红黑不停地切换
答案是确定的,我们可以对android:autoLink属性进行设置,或者调用 setAutoLinkMask(int)方法
Must be one or more (separated by '|') of the following constant values.
例如xml设置
android:autoLink="web"
android:autoLink="web|email"
android:autoLink="all"
java代码设置
textView.setAutoLinkMask(Linkify.EMAIL_ADDRESSES);
textView.setAutoLinkMask(Linkify.EMAIL_ADDRESSES|Linkify.WEB_URLS);
textView.setAutoLinkMask(Linkify.ALL);
七、获取本地资源及格式化
方法1
public final CharSequence getText (int resId)
Return a localized, styled CharSequence from the application's package's default string table.
方法2
public final String getString (int resId)
Return a localized string from the application's package's default string table.
方法3
getResources().getString(int resId)
Return the string value associated with a particular resource ID. It will be stripped of any styled text information.
演示:
<string name="text_format">Plain, <b>bold</b>, <i>italic</i>, <b><i>bold-italic</i></b></string>
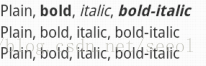
TextView 也可以支持html格式显示
主要是使用Html.fromHtml(String str)方法
string.xml特殊字符
string.xml中有特殊的字符,会报错的,那应该怎样解决呢
一个方法是用转义符,还有一个更简单的方法就是用<![CDATA[]]>形式
string.xml字符串中带参数
结果显示:我是科学家,我的年龄是
%1$s 1表示第一个参数,s表示为字符串
%2$d 2表示第二个参数,d表示为数字,整数、小数都可以
八、显示图片
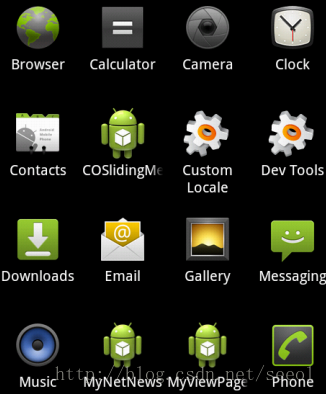
上图是android桌面上的应用图标,应用名称上面是应用logo,这个怎样的实现的
是不是用了两个控件,一个是ImageView,另一个是TextView
<ImageView />
<TextView />
其实不是,真正实现只是用了一个TextView
<TextView android:drawableTop="@drawable/ic_launcher"/>
android:drawableTop表示是textView上面显示图片
android:drawableBottom表示底部显示图片
android:drawableLeft表示左边显示图片
android:drawableRight表示右边显示图片
内容过长时,可以加省略号
android:ellipsize = "none" 默认值,没有省略号
android:ellipsize = "end" 省略号在结尾
android:ellipsize = "start" 省略号在开头
android:ellipsize = "middle" 省略号在中间
android:ellipsize = "marquee" 跑马灯
注意要加行数限制,要不省略号不起作用
如 android:maxLines="2" 超过两行将出现省略号
或者android:singleLine="true"限制为1行
一、实例
在介绍前先演示一下实例效果图一如上
布局文件text_view.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent" > <TextView android:id="@+id/text_view" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/textView_content" /> </LinearLayout>
java代码TextViewActivity.java
package com.example.test; import android.app.Activity; import android.os.Bundle; public class TextViewActivity extends Activity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.text_view); } }
再在AndroidManifest.xml配置一下
<activity android:name="TextViewActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity>
其中用的字符串资源是
<string name="textView_content">我是Textview,大家要好好学习!</string>
二、TextView类结构
java.lang.Object↳ android.view.View
↳ android.widget.TextView
直接子类
Button, CheckedTextView, Chronometer, DigitalClock, EditText, TextClock
间接子类
AutoCompleteTextView, CheckBox, CompoundButton, ExtractEditText, MultiAutoCompleteTextView, RadioButton, Switch, ToggleButton
Button,EditText,CheckBox,RadioButton,ToggleButton 等都是它的直接子类或间接子类,所以我们要一定学好TextView,才能更好地学习其它控件。
三、TextView属性
XML Attributes | ||
---|---|---|
Attribute Name | Related Method | Description |
android:autoLink | setAutoLinkMask(int) | Controls whether links such as urls and email addresses are automatically found and converted to clickable links. |
android:autoText | setKeyListener(KeyListener) | If set, specifies that this TextView has a textual input method and automatically corrects some common spelling errors. |
android:bufferType | setText(CharSequence,TextView.BufferType) | Determines the minimum type that getText() will return. |
android:capitalize | setKeyListener(KeyListener) | If set, specifies that this TextView has a textual input method and should automatically capitalize what the user types. |
android:cursorVisible | setCursorVisible(boolean) | Makes the cursor visible (the default) or invisible. |
android:digits | setKeyListener(KeyListener) | If set, specifies that this TextView has a numeric input method and that these specific characters are the ones that it will accept. |
android:drawableBottom | setCompoundDrawablesWithIntrinsicBounds(int,int,int,int) | The drawable to be drawn below the text. |
android:drawableEnd | setCompoundDrawablesRelativeWithIntrinsicBounds(int,int,int,int) | The drawable to be drawn to the end of the text. |
android:drawableLeft | setCompoundDrawablesWithIntrinsicBounds(int,int,int,int) | The drawable to be drawn to the left of the text. |
android:drawablePadding | setCompoundDrawablePadding(int) | The padding between the drawables and the text. |
android:drawableRight | setCompoundDrawablesWithIntrinsicBounds(int,int,int,int) | The drawable to be drawn to the right of the text. |
android:drawableStart | setCompoundDrawablesRelativeWithIntrinsicBounds(int,int,int,int) | The drawable to be drawn to the start of the text. |
android:drawableTop | setCompoundDrawablesWithIntrinsicBounds(int,int,int,int) | The drawable to be drawn above the text. |
android:editable | If set, specifies that this TextView has an input method. | |
android:editorExtras | setInputExtras(int) | Reference to an <input-extras>XML resource containing additional data to supply to an input method, which is private to the implementation of the input method. |
android:ellipsize | setEllipsize(TextUtils.TruncateAt) | If set, causes words that are longer than the view is wide to be ellipsized instead of broken in the middle. |
android:ems | setEms(int) | Makes the TextView be exactly this many ems wide. |
android:fontFamily | setTypeface(Typeface) | Font family (named by string) for the text. |
android:freezesText | setFreezesText(boolean) | If set, the text view will include its current complete text inside of its frozen icicle in addition to meta-data such as the current cursor position. |
android:gravity | setGravity(int) | Specifies how to align the text by the view's x- and/or y-axis when the text is smaller than the view. |
android:height | setHeight(int) | Makes the TextView be exactly this many pixels tall. |
android:hint | setHint(int) | Hint text to display when the text is empty. |
android:imeActionId | setImeActionLabel(CharSequence,int) | Supply a value for EditorInfo.actionIdused when an input method is connected to the text view. |
android:imeActionLabel | setImeActionLabel(CharSequence,int) | Supply a value for EditorInfo.actionLabelused when an input method is connected to the text view. |
android:imeOptions | setImeOptions(int) | Additional features you can enable in an IME associated with an editor to improve the integration with your application. |
android:includeFontPadding | setIncludeFontPadding(boolean) | Leave enough room for ascenders and descenders instead of using the font ascent and descent strictly. |
android:inputMethod | setKeyListener(KeyListener) | If set, specifies that this TextView should use the specified input method (specified by fully-qualified class name). |
android:inputType | setRawInputType(int) | The type of data being placed in a text field, used to help an input method decide how to let the user enter text. |
android:lineSpacingExtra | setLineSpacing(float,float) | Extra spacing between lines of text. |
android:lineSpacingMultiplier | setLineSpacing(float,float) | Extra spacing between lines of text, as a multiplier. |
android:lines | setLines(int) | Makes the TextView be exactly this many lines tall. |
android:linksClickable | setLinksClickable(boolean) | If set to false, keeps the movement method from being set to the link movement method even if autoLink causes links to be found. |
android:marqueeRepeatLimit | setMarqueeRepeatLimit(int) | The number of times to repeat the marquee animation. |
android:maxEms | setMaxEms(int) | Makes the TextView be at most this many ems wide. |
android:maxHeight | setMaxHeight(int) | Makes the TextView be at most this many pixels tall. |
android:maxLength | setFilters(InputFilter) | Set an input filter to constrain the text length to the specified number. |
android:maxLines | setMaxLines(int) | Makes the TextView be at most this many lines tall. |
android:maxWidth | setMaxWidth(int) | Makes the TextView be at most this many pixels wide. |
android:minEms | setMinEms(int) | Makes the TextView be at least this many ems wide. |
android:minHeight | setMinHeight(int) | Makes the TextView be at least this many pixels tall. |
android:minLines | setMinLines(int) | Makes the TextView be at least this many lines tall. |
android:minWidth | setMinWidth(int) | Makes the TextView be at least this many pixels wide. |
android:numeric | setKeyListener(KeyListener) | If set, specifies that this TextView has a numeric input method. |
android:password | setTransformationMethod(TransformationMethod) | Whether the characters of the field are displayed as password dots instead of themselves. |
android:phoneNumber | setKeyListener(KeyListener) | If set, specifies that this TextView has a phone number input method. |
android:privateImeOptions | setPrivateImeOptions(String) | An addition content type description to supply to the input method attached to the text view, which is private to the implementation of the input method. |
android:scrollHorizontally | setHorizontallyScrolling(boolean) | Whether the text is allowed to be wider than the view (and therefore can be scrolled horizontally). |
android:selectAllOnFocus | setSelectAllOnFocus(boolean) | If the text is selectable, select it all when the view takes focus. |
android:shadowColor | setShadowLayer(float,float,float,int) | Place a shadow of the specified color behind the text. |
android:shadowDx | setShadowLayer(float,float,float,int) | Horizontal offset of the shadow. |
android:shadowDy | setShadowLayer(float,float,float,int) | Vertical offset of the shadow. |
android:shadowRadius | setShadowLayer(float,float,float,int) | Radius of the shadow. |
android:singleLine | setTransformationMethod(TransformationMethod) | Constrains the text to a single horizontally scrolling line instead of letting it wrap onto multiple lines, and advances focus instead of inserting a newline when you press the enter key. |
android:text | setText(CharSequence,TextView.BufferType) | Text to display. |
android:textAllCaps | setAllCaps(boolean) | Present the text in ALL CAPS. |
android:textAppearance | Base text color, typeface, size, and style. | |
android:textColor | setTextColor(int) | Text color. |
android:textColorHighlight | setHighlightColor(int) | Color of the text selection highlight. |
android:textColorHint | setHintTextColor(int) | Color of the hint text. |
android:textColorLink | setLinkTextColor(int) | Text color for links. |
android:textIsSelectable | isTextSelectable() | Indicates that the content of a non-editable text can be selected. |
android:textScaleX | setTextScaleX(float) | Sets the horizontal scaling factor for the text. |
android:textSize | setTextSize(int,float) | Size of the text. |
android:textStyle | setTypeface(Typeface) | Style (bold, italic, bolditalic) for the text. |
android:typeface | setTypeface(Typeface) | Typeface (normal, sans, serif, monospace) for the text. |
android:width | setWidth(int) | Makes the TextView be exactly this many pixels wide. |
四、能过代码方式建立TextView
TextView可以通过在布局文件进行设置,也可以通过代码生成@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); TextView textView= new TextView(this); textView.setText(getText(R.string.textView_content)); setContentView(textView); }
运行的效果和图一完全一样
五、走马灯效果
android:ellipsize="marquee" android:focusable="true" android:focusableInTouchMode="true" android:marqueeRepeatLimit="marquee_forever" android:singleLine="true"
加上以上属性就可以实现走马灯的效果了
注意:
1.要单行,如果没有设置单行,多行可以全部显示出来,是没有走马灯的效果的
2.设置为焦点
3没有滚动球的设备 android:focusableInTouchMode="true"属性是必须的
4.如果内容一行就可以显示出来的,请重复一次内容,如果重复后还是可以一行显示的,请再重复,如
<string name="textView_content">我是Textview,大家要好好学习!我是Textview,大家要好好学习!</string>
闪烁效果
别人的走马灯效果要闪烁一下,那我们也来实现
public class TextViewActivity extends Activity { private TextView textView = null; private boolean changeColor = false; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.text_view); textView = (TextView) findViewById(R.id.text_view); new Thread(new MyThread()).start(); } //实现半秒就改变一次颜色 class MyThread implements Runnable { @Override public void run() { //传说中死循环,半秒就改变一次 while (true) { try { Thread.sleep(500); } catch (InterruptedException e) { e.printStackTrace(); } Message message = new Message(); if (changeColor = !changeColor) { message.what = COLOR_BLACK; } else { message.what = COLOR_RED; } handler.sendMessage(message); } }// end run } private final int COLOR_RED = 1;//红色 private final int COLOR_BLACK = 2;//黑色 private MyHandler handler = new MyHandler(); class MyHandler extends Handler { @Override public void handleMessage(Message msg) { switch (msg.what) { case COLOR_RED: textView.setTextColor(Color.RED); break; case COLOR_BLACK: textView.setTextColor(Color.BLACK); break; default: break; } } } }
以上的效果是半秒就改变一下字体的颜色,红黑不停地切换
六、在文本中创建上下文链接
如果TextView中的文本字符串包含有网址、邮箱、电话号码或地址,是否可以点击进行相应的跳转到我们要去的页面,如字符串的网址链接,跳到相应的网页,那样我们就可以直接查看内容了,这不是一件很美妙的事吗?答案是确定的,我们可以对android:autoLink属性进行设置,或者调用 setAutoLinkMask(int)方法
Must be one or more (separated by '|') of the following constant values.
Constant | Value | Description |
---|---|---|
none | 0x00 | 都不匹配 (default). |
web | 0x01 | 匹配网址链接 |
0x02 | 匹配email地址 | |
phone | 0x04 | 匹配电话号码 |
map | 0x08 | 匹配地图地址. |
all | 0x0f | 匹配所有(equivalent to web|email|phone|map). |
android:autoLink="web"
android:autoLink="web|email"
android:autoLink="all"
java代码设置
textView.setAutoLinkMask(Linkify.EMAIL_ADDRESSES);
textView.setAutoLinkMask(Linkify.EMAIL_ADDRESSES|Linkify.WEB_URLS);
textView.setAutoLinkMask(Linkify.ALL);
七、获取本地资源及格式化
方法1public final CharSequence getText (int resId)
Return a localized, styled CharSequence from the application's package's default string table.
方法2
public final String getString (int resId)
Return a localized string from the application's package's default string table.
方法3
getResources().getString(int resId)
Return the string value associated with a particular resource ID. It will be stripped of any styled text information.
演示:
<string name="text_format">Plain, <b>bold</b>, <i>italic</i>, <b><i>bold-italic</i></b></string>
text_view_1.setText(getText(R.string.text_format)); text_view_2.setText(getString(R.string.text_format)); text_view_3.setText(getResources().getString(R.string.text_format));
TextView 也可以支持html格式显示
主要是使用Html.fromHtml(String str)方法
String htmlStr="我是<font color=red>中国人</font>。<br/>我你他也是<font color=green>中国人</font>呀。"; text_view_4.setText(Html.fromHtml(htmlStr));
string.xml特殊字符
string.xml中有特殊的字符,会报错的,那应该怎样解决呢
一个方法是用转义符,还有一个更简单的方法就是用<![CDATA[]]>形式
<string name="other _format"><![CDATA[在这里输入特殊的字符,不会报错的]]></string>
string.xml字符串中带参数
<string name="textview_param">我是%1$s,我的年龄是%2$d</string> text_view_5.setText(getString(R.string.textview_param, "科学家",20));
结果显示:我是科学家,我的年龄是
%1$s 1表示第一个参数,s表示为字符串
%2$d 2表示第二个参数,d表示为数字,整数、小数都可以
八、显示图片
上图是android桌面上的应用图标,应用名称上面是应用logo,这个怎样的实现的
是不是用了两个控件,一个是ImageView,另一个是TextView
<ImageView />
<TextView />
其实不是,真正实现只是用了一个TextView
<TextView android:drawableTop="@drawable/ic_launcher"/>
android:drawableTop表示是textView上面显示图片
android:drawableBottom表示底部显示图片
android:drawableLeft表示左边显示图片
android:drawableRight表示右边显示图片
九、属性介绍
android:ellipsize内容过长时,可以加省略号
android:ellipsize = "none" 默认值,没有省略号
android:ellipsize = "end" 省略号在结尾
android:ellipsize = "start" 省略号在开头
android:ellipsize = "middle" 省略号在中间
android:ellipsize = "marquee" 跑马灯
注意要加行数限制,要不省略号不起作用
如 android:maxLines="2" 超过两行将出现省略号
或者android:singleLine="true"限制为1行
相关文章推荐
- Android实现表情 抓取新浪表情
- 详解Android解析Xml的三种方式——DOM、SAX以及XMLpull
- flex 控件的重要属性
- web下载的ActiveX控件自动更新
- Android的TextView与Html相结合的具体方法
- ASP.NET的HtmlForm控件学习及Post与Get的区别概述
- ASP.net 动态加载控件时一些问题的总结
- android textview 显示html方法解析
- android开发环境遇到adt无法启动的问题分析及解决方法
- Android开发 旋转屏幕导致Activity重建解决方法
- Android开发技巧之在a标签或TextView控件中单击链接弹出Activity(自定义动作)
- Android实现TextView中文字链接的4种方式介绍及代码
- android TextView属性的详细介绍 分享
- ASP.NET 4中的可扩展输出缓存(可以缓存页面/控件等)
- .NET中TextBox控件设置ReadOnly=true后台取不到值三种解决方法
- asp.net DataGrid控件中弹出详细信息窗口
- asp.net Repeater控件的说明及详细介绍及使用方法
- 分析10个ASP.NET控件最有用的属性详解
- javascript利用控件对windows的操作实现原理与应用