Trie原理、扩展及Python实现
2013-08-06 20:01
441 查看
关于Trie树的原理这里不做介绍,网上相关的资料非常多,可以参考July的文章:http://blog.csdn.net/v_july_v/article/details/6897097。不过Trie确实是非常的强大,原理不复杂,使用起来也非常的方便。代码实现其实也不难,如果用C++实现的话需要自己定义数据结构(结构体)来构建树,这里我介绍怎样用Python实现,用Python实现起来尤为的方便,不用自己定义数据结构,用Python的dictionary类型即可。说一句题外话:我发现自从学会用Python以后就爱不释手,确实是使用起来非常方便。言归正传,看看Python实现的简易Trie树,为什么说是简易呢?因为没有实现复杂的功能,只是为了说明Trie的基本原理,而且只支持ascii字符。实现了以下几项基本功能:
1、添加单词:LBTrie的add方法;
2、查找单词:LBTrie的search方法;
3、打印Trie树:LBTrie的output方法。
打印的Trie树如下图所示:
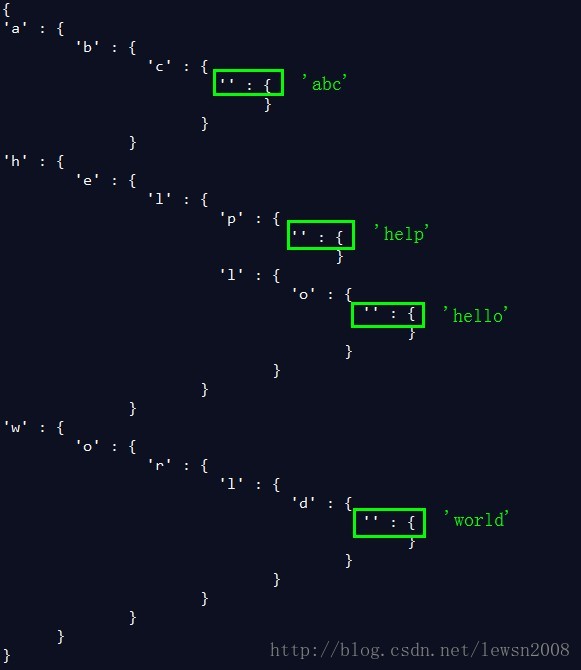
查找输出结果为:
Yes
No
【扩展】
1. Trie树的应用之一:搜索引擎的Suggestion,文章中有介绍:http://blog.csdn.net/stormbjm/article/details/12752317
2. 1中的文章讲了基于Trie树的搜索引擎Suggestion,同时推荐了基于另外一种数据结构的Suggestion:一种基于Trie变种的数据结构——三叉树(Ternary Tree),见文章:http://igoro.com/archive/efficient-auto-complete-with-a-ternary-search-tree/,文章讲到:三叉树比Trie有更高的存储效率。
1、添加单词:LBTrie的add方法;
2、查找单词:LBTrie的search方法;
3、打印Trie树:LBTrie的output方法。
class LBTrie: """ simple implemention of Trie in Python by authon liubing, which is not perfect but just to illustrate the basis and principle of Trie. """ def __init__(self): self.trie = {} self.size = 0 #添加单词 def add(self, word): p = self.trie word = word.strip() for c in word: if not c in p: p[c] = {} p = p[c] if word != '': #在单词末尾处添加键值''作为标记,即只要某个字符的字典中含有''键即为单词结尾 p[''] = '' #查询单词 def search(self, word): p = self.trie word = word.lstrip() for c in word: if not c in p: return False p = p[c] #判断单词结束标记'' if '' in p: return True return False #打印Trie树的接口 def output(self): print '{' self.__print_item(self.trie) print '}' #实现Trie树打印的私有递归函数,indent控制缩进 def __print_item(self, p, indent=0): if p: ind = '' + '\t' * indent for key in p.keys(): label = "'%s' : " % key print ind + label + '{' self.__print_item(p[key], indent+1) print ind + ' '*len(label) + '}' if __name__ == '__main__': trie_obj = LBTrie() #添加单词 trie_obj.add('hello') trie_obj.add('help') trie_obj.add('world') trie_obj.add('abc') #打印构建的Trie树 trie_obj.output() #查找单词 if trie_obj.search('hello'): print 'Yes' else: print 'No' if trie_obj.search('China'): print 'Yes' else: print 'No'
打印的Trie树如下图所示:
查找输出结果为:
Yes
No
【扩展】
1. Trie树的应用之一:搜索引擎的Suggestion,文章中有介绍:http://blog.csdn.net/stormbjm/article/details/12752317
2. 1中的文章讲了基于Trie树的搜索引擎Suggestion,同时推荐了基于另外一种数据结构的Suggestion:一种基于Trie变种的数据结构——三叉树(Ternary Tree),见文章:http://igoro.com/archive/efficient-auto-complete-with-a-ternary-search-tree/,文章讲到:三叉树比Trie有更高的存储效率。
相关文章推荐
- Trie 的原理和实现 (python 实现)
- Good Features to track特征点检测原理与opencv(python)实现
- dubbo扩展的实现原理
- 决策树原理及Python代码实现
- Python 继承实现的原理(继承顺序)
- 快速实现python c扩展模块
- 层次聚类算法的原理及python实现
- LRUCache的实现原理及利用python实现的方法
- 决策树之CART算法原理及python实现
- python扩展实现方法--python与c混和编程
- Python使用扩展库pywin32实现批量文档打印
- 逻辑回归(Logistic Regression)原理及Python实现
- DFA和trie字典树实现敏感词过滤(python和c语言)
- 最小二乘法 多项式曲线拟合 原理公式理解 Python 实现
- 基于python的PCA的实现(1)--原理
- 可绑定可扩展的帐号系统设计原理及其实现
- 主成分分析法原理及其python实现
- 回归分析---线性回归原理和Python实现
- 梯度下降原理及Python实现
- windows 下 使用codeblocks 实现C语言对python的扩展