播放SDcard上面的音频文件
2013-07-29 19:02
471 查看
这个程序是将sdcard上的音频文件读取出来,将其路径放到ArrayList中,在添加到ListView中,然后通过MediaPlayer播放音频文件~~~
一如既往,有码有真相~~

布局文件源码
运行截图:
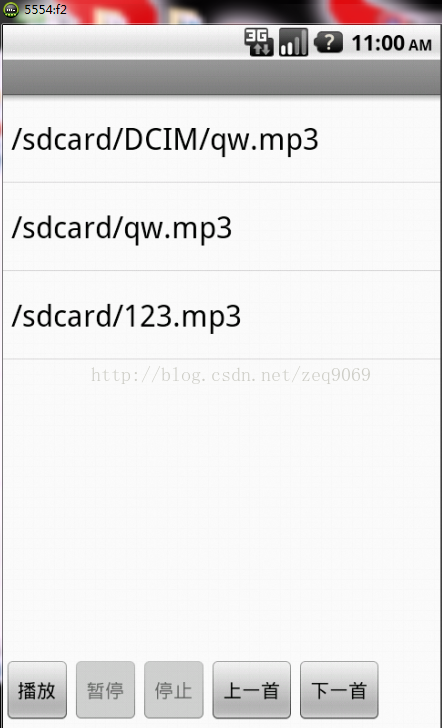
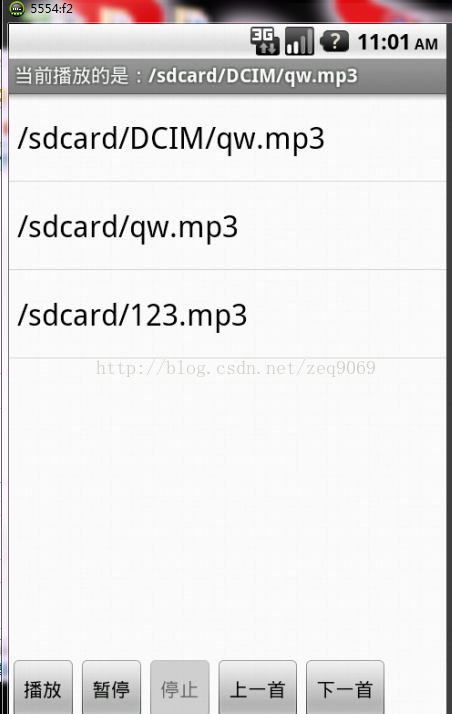
一如既往,有码有真相~~

package com.example.sdcardmusicplay; import java.io.File; import java.io.IOException; import java.util.ArrayList; import android.media.MediaPlayer; import android.media.MediaPlayer.OnCompletionListener; import android.os.Bundle; import android.app.Activity; import android.view.Menu; import android.view.View; import android.view.View.OnClickListener; import android.widget.AdapterView; import android.widget.AdapterView.OnItemClickListener; import android.widget.ArrayAdapter; import android.widget.Button; import android.widget.ListView; import android.widget.Toast; public class MainActivity extends Activity { private MediaPlayer mp; private int currentItem=0;//记录当前播放的item private ArrayList<String> list = new ArrayList<String>(); private Button b1, b2, b3, b4, b5; private boolean isplay=false;//////记录是否在播放 public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); mp = new MediaPlayer(); b1 = (Button) findViewById(R.id.play); b2 = (Button) findViewById(R.id.pause); b3 = (Button) findViewById(R.id.stop); b4 = (Button) findViewById(R.id.up); b5 = (Button) findViewById(R.id.down); b2.setEnabled(false); b3.setEnabled(false); audiolist(); b1.setOnClickListener(new OnClickListener() { public void onClick(View v) { playMusic(list.get(currentItem)); b1.setEnabled(false); b2.setText("暂停"); b3.setEnabled(true); isplay = true; MainActivity.this.setTitle("当前播放的是:" + list.get(currentItem)); } }); b2.setOnClickListener(new OnClickListener() { public void onClick(View v) { if (isplay) { MainActivity.this.setTitle("当前播放的是:" + list.get(currentItem)); mp.pause(); b1.setEnabled(false); b2.setText("继续"); b3.setEnabled(true); isplay = false; } else { mp.start(); b1.setEnabled(false); b2.setText("暂停"); b3.setEnabled(true); isplay = true; MainActivity.this.setTitle("当前播放的是:" + list.get(currentItem)); } } }); b3.setOnClickListener(new OnClickListener() { public void onClick(View v) { mp.stop(); b1.setEnabled(true); b2.setEnabled(false); b3.setEnabled(false); MainActivity.this.setTitle(""); } }); b4.setOnClickListener(new OnClickListener() { public void onClick(View v) { upOne(); MainActivity.this.setTitle("当前播放的是:" + list.get(currentItem)); isplay = true; b1.setEnabled(false); b2.setEnabled(true); b2.setText("暂停"); b3.setEnabled(true); } }); b5.setOnClickListener(new OnClickListener() { public void onClick(View v) { nextOne(); isplay = true; b1.setEnabled(false); b2.setEnabled(true); b2.setText("暂停"); b3.setEnabled(true); MainActivity.this.setTitle("当前播放的是:" + list.get(currentItem)); } }); mp.setOnCompletionListener(new OnCompletionListener() { @Override public void onCompletion(MediaPlayer mp) { // TODO Auto-generated method stub Toast.makeText(MainActivity.this, "播放完毕~~~", Toast.LENGTH_SHORT); MainActivity.this.setTitle(""); } }); } public void audiolist() {/////////制作ListView getFiles("/sdcard/");///////读取音频文件,将其添加到list中 ListView lv = (ListView) findViewById(R.id.list); ArrayAdapter<String> aa = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, list); lv.setAdapter(aa); lv.setOnItemClickListener(new OnItemClickListener() { public void onItemClick(AdapterView<?> arg0, View arg1, int position, long arg3) { currentItem = position; playMusic(list.get(position)); MainActivity.this.setTitle("当前播放的是:"+list.get(currentItem)); } }); } public void playMusic(String path) {//根据path播放音频 mp.reset(); try { mp.setDataSource(path); } catch (IllegalArgumentException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (SecurityException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (IllegalStateException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } try { mp.prepare(); } catch (IllegalStateException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } mp.start(); b2.setEnabled(true); b2.setText("暂停"); } public void getFiles(String url){//查找获取音频文件,添加到list中 File files=new File(url); File [] file=files.listFiles(); if(file!=null){//////////////////有可能是空目录,所以用来排除空目录 for(File f:file){/////////遍历file数组 if(f.isDirectory()){ getFiles(f.getAbsolutePath());///////递归调用 }else{ if(isAudioFile(f.getPath())){/////调用方法判断是不是音频文件 list.add(f.getPath()); } } } } } public boolean isAudioFile(String path){ String [] formatt={"mp3","wav","3gp"}; for(String s:formatt){ if(path.contains(s)){ return true; } } return false; } public void nextOne(){////下一首 if(++currentItem>=list.size()){ currentItem=0; } playMusic(list.get(currentItem)); } public void upOne(){////上一首 if(--currentItem>=0){ if(currentItem>=list.size()){ currentItem=0; } }else{ currentItem=list.size()-1; } playMusic(list.get(currentItem)); } protected void onDestroy() {// //////当程序退出时,释放资源 // TODO Auto-generated method stub mp.release(); super.onDestroy(); } }
布局文件源码
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity" android:orientation="vertical" > <ListView android:id="@+id/list" android:layout_width="fill_parent" android:layout_height="fill_parent" android:isScrollContainer="true" android:layout_weight="1" android:drawSelectorOnTop="false" /> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="horizontal" android:layout_weight="6" > <Button android:id="@+id/play" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="播放" /> <Button android:id="@+id/pause" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="暂停/继续" /> <Button android:id="@+id/stop" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="停止" /> <Button android:id="@+id/up" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="上一首" /> <Button android:id="@+id/down" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="下一首" /> </LinearLayout> </LinearLayout>
运行截图:
相关文章推荐
- Android实现sdcard音频文件浏览及MediaPlayer播放
- Android MediaPlayer 简单综合应用------列出sdcard里所有.mp3文件,并且可以点击播放!
- Android MediaPlayer 简单综合应用------列出sdcard里所有.mp3文件,并且可以点击播放!
- Android MediaPlayer 简单综合应用------列出sdcard里所有.mp3文件,并且可以点击播放!
- android扫描sdcard中的音频、视频、图片等文件的方法
- FileNotFoundException: /mnt/sdcard/test: open failed: 。。文件找不到异常
- android WebView 控件加载本地sdcard中html文件图片的问题
- android 选择手机sdcard中的文件,获得其路径
- 在mt7628实现alsa架构解码并播放音频文件
- unity播放加密的音频文件
- 深度分析:Android4.3下MMS发送到附件为音频文件(音频为系统内置音频)的彩信给自己,添加音频-发送彩信-接收彩信-下载音频附件-预览-播放(一,添加附件)
- Android文件存储之SDcard
- 文件从android的sdcard读出时显示乱码问题
- Android 拷贝raw文件夹下面的sqlite数据库文件到SDCard中 然后读取数据库并绑定
- C++ 播放音频文件
- 文件操作与SDCard读写访问
- android sdcard存储方案三(基于fuse文件系统):
- iphone利用AudioQueue播放音频文件(mp3,aac,caf,wav等)
- VLC 播放PCM音频文件
- android操作sdcard中的多媒体文件(一)——音乐列表的制作