python(pil)图像处理(等比例压缩、裁剪压缩) 缩略(水印)图
2013-06-08 07:45
671 查看
在开始前,你可以先了解一下PIL基础知识:
http://tech.seety.org/python/python_imaging.html
我在这里就不多说了,直接上代码:
效果图:
原图:
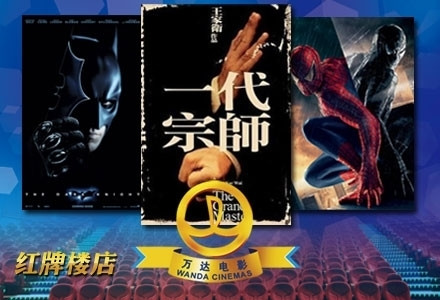
裁剪压缩(保证了大小是94*94,但是边缘部分被裁掉了):

等比例压缩(保证了图片完整性,但是图片高与宽在很不成比例情况下会被压扁,且大小为:94*64):

PS:PHP版
http://fc-lamp.blog.163.com/blog/static/174566687201247570315/
另PIL图像的绘制
PIL中的ImageDraw模组提供给我们绘制影像内容的能力。在使用ImageDraw之前,要先建立好空白的新影像:
最后建出来的draw是一个ImageDraw物件会提供各种绘制影像的方法。针对几何图形,draw物件提供arc() (弧线)、chord() (弦)、line() (线段)、ellipse() (椭圆)、point() (点)、rectangle() (矩形)与polygon () (多边形)。
这里要介绍的不是几何图形,而是文字的绘制。我们要再介绍一个模组:ImageFont,并且以实例来说明如何用PIL 「写字」:
这样就在一个黑色底图上用白笔写了"TEXT" 四个大字
接着一一说明刚刚作的动作。首先我们用ImageFont的truetype()函式建立了一个TrueType字型,大小设定为16点。truetype()函式的第一个参数必须是字型档的搜寻路径,第二个参数是字型的点数。然后依序建立影像物件与draw物件。写字的动作用draw物件的text()方法来完成,它接受两个参数,分别是文字的左上角点、字串,另外可以用font选项来指定所使用的字型(若不指定,便使用预设字型)。
在1.1.4 版之前,PIL 是只能使用点阵字型的。现在PIL 加入了TrueType 向量字型的支援,对于要「写字」的人来说实在是一大福音。对点阵字来说,想改变字型的大小得要更换字型才作得到,但TrueType 就没有这个限制。如果我们想要写出两串不同大小的文字,这样作就可以了:
以上就是在PIL 里建立文字图形的方法。
最后,我们要说明如何改变绘制图形(文字)时的颜色;绘图时画笔的颜色是透过draw物件的ink属性来改变的:
以上会把画笔设成绿色。ink值必须要是一个整数,其值由色彩的RGB值算出。举几个ink值的例子:
红色的ink值应设为255(R) + 0(G)*256 + 0(B)*256*256,
蓝色的ink值应设为0(R) + 0(G)*256 + 255(B)*256*256,
靛色的ink值应设为0(R) + 255(G)*256 + 255(B)*256*256
所设定的ink会影响所有后续的绘图动作。
http://tech.seety.org/python/python_imaging.html
我在这里就不多说了,直接上代码:
#coding:utf-8 ''' python图片处理 @author:fc_lamp @blog:http://fc-lamp.blog.163.com/ ''' import Image as image #等比例压缩图片 def resizeImg(**args): args_key = {'ori_img':'','dst_img':'','dst_w':'','dst_h':'','save_q':75} arg = {} for key in args_key: if key in args: arg[key] = args[key] im = image.open(arg['ori_img']) ori_w,ori_h = im.size widthRatio = heightRatio = None ratio = 1 if (ori_w and ori_w > arg['dst_w']) or (ori_h and ori_h > arg['dst_h']): if arg['dst_w'] and ori_w > arg['dst_w']: widthRatio = float(arg['dst_w']) / ori_w #正确获取小数的方式 if arg['dst_h'] and ori_h > arg['dst_h']: heightRatio = float(arg['dst_h']) / ori_h if widthRatio and heightRatio: if widthRatio < heightRatio: ratio = widthRatio else: ratio = heightRatio if widthRatio and not heightRatio: ratio = widthRatio if heightRatio and not widthRatio: ratio = heightRatio newWidth = int(ori_w * ratio) newHeight = int(ori_h * ratio) else: newWidth = ori_w newHeight = ori_h im.resize((newWidth,newHeight),image.ANTIALIAS).save(arg['dst_img'],quality=arg['save_q']) ''' image.ANTIALIAS还有如下值: NEAREST: use nearest neighbour BILINEAR: linear interpolation in a 2x2 environment BICUBIC:cubic spline interpolation in a 4x4 environment ANTIALIAS:best down-sizing filter ''' #裁剪压缩图片 def clipResizeImg(**args): args_key = {'ori_img':'','dst_img':'','dst_w':'','dst_h':'','save_q':75} arg = {} for key in args_key: if key in args: arg[key] = args[key] im = image.open(arg['ori_img']) ori_w,ori_h = im.size dst_scale = float(arg['dst_h']) / arg['dst_w'] #目标高宽比 ori_scale = float(ori_h) / ori_w #原高宽比 if ori_scale >= dst_scale: #过高 width = ori_w height = int(width*dst_scale) x = 0 y = (ori_h - height) / 3 else: #过宽 height = ori_h width = int(height*dst_scale) x = (ori_w - width) / 2 y = 0 #裁剪 box = (x,y,width+x,height+y) #这里的参数可以这么认为:从某图的(x,y)坐标开始截,截到(width+x,height+y)坐标 #所包围的图像,crop方法与php中的imagecopy方法大为不一样 newIm = im.crop(box) im = None #压缩 ratio = float(arg['dst_w']) / width newWidth = int(width * ratio) newHeight = int(height * ratio) newIm.resize((newWidth,newHeight),image.ANTIALIAS).save(arg['dst_img'],quality=arg['save_q']) #水印(这里仅为图片水印) def waterMark(**args): args_key = {'ori_img':'','dst_img':'','mark_img':'','water_opt':''} arg = {} for key in args_key: if key in args: arg[key] = args[key] im = image.open(arg['ori_img']) ori_w,ori_h = im.size mark_im = image.open(arg['mark_img']) mark_w,mark_h = mark_im.size option ={'leftup':(0,0),'rightup':(ori_w-mark_w,0),'leftlow':(0,ori_h-mark_h), 'rightlow':(ori_w-mark_w,ori_h-mark_h) } im.paste(mark_im,option[arg['water_opt']],mark_im.convert('RGBA')) im.save(arg['dst_img']) #Demon #源图片 ori_img = 'D:/tt.jpg' #水印标 mark_img = 'D:/mark.png' #水印位置(右下) water_opt = 'rightlow' #目标图片 dst_img = 'D:/python_2.jpg' #目标图片大小 dst_w = 94 dst_h = 94 #保存的图片质量 save_q = 35 #裁剪压缩 clipResizeImg(ori_img=ori_img,dst_img=dst_img,dst_w=dst_w,dst_h=dst_h,save_q = save_q) #等比例压缩 #resizeImg(ori_img=ori_img,dst_img=dst_img,dst_w=dst_w,dst_h=dst_h,save_q=save_q) #水印 #waterMark(ori_img=ori_img,dst_img=dst_img,mark_img=mark_img,water_opt=water_opt)
效果图:
原图:
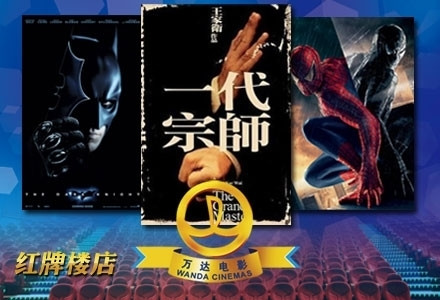
裁剪压缩(保证了大小是94*94,但是边缘部分被裁掉了):

等比例压缩(保证了图片完整性,但是图片高与宽在很不成比例情况下会被压扁,且大小为:94*64):

PS:PHP版
http://fc-lamp.blog.163.com/blog/static/174566687201247570315/
另PIL图像的绘制
PIL中的ImageDraw模组提供给我们绘制影像内容的能力。在使用ImageDraw之前,要先建立好空白的新影像:
>>> import ImageDraw >>> im = Image.new( "RGB", (400,300) ) >>> draw = ImageDraw.Draw( im )
最后建出来的draw是一个ImageDraw物件会提供各种绘制影像的方法。针对几何图形,draw物件提供arc() (弧线)、chord() (弦)、line() (线段)、ellipse() (椭圆)、point() (点)、rectangle() (矩形)与polygon () (多边形)。
这里要介绍的不是几何图形,而是文字的绘制。我们要再介绍一个模组:ImageFont,并且以实例来说明如何用PIL 「写字」:
>>> import Image, ImageDraw, ImageFont >>> font = ImageFont.truetype( \ ... "/usr/share/fonts/truetype/freefont/FreeMono.ttf", 24 ) >>> im = Image.new( "RGB", (400,300) ) >>> draw = ImageDraw.Draw( im ) >>> draw.text( (20,20), "TEXT", font=font ) >>> im.save( "text.jpg" )
这样就在一个黑色底图上用白笔写了"TEXT" 四个大字
接着一一说明刚刚作的动作。首先我们用ImageFont的truetype()函式建立了一个TrueType字型,大小设定为16点。truetype()函式的第一个参数必须是字型档的搜寻路径,第二个参数是字型的点数。然后依序建立影像物件与draw物件。写字的动作用draw物件的text()方法来完成,它接受两个参数,分别是文字的左上角点、字串,另外可以用font选项来指定所使用的字型(若不指定,便使用预设字型)。
在1.1.4 版之前,PIL 是只能使用点阵字型的。现在PIL 加入了TrueType 向量字型的支援,对于要「写字」的人来说实在是一大福音。对点阵字来说,想改变字型的大小得要更换字型才作得到,但TrueType 就没有这个限制。如果我们想要写出两串不同大小的文字,这样作就可以了:
>>> largefont = ImageFont.truetype( \ ... "/usr/share/fonts/truetype/freefont/FreeMono.ttf", 48 ) >>> smallfont = ImageFont.truetype( \ ... "/usr/share/fonts/truetype/freefont/FreeMono.ttf", 24 ) >>> im = Image.new( "RBG", (400,300) ) >>> draw = ImageDraw.Draw( im ) >>> draw.text( (20,20), "SmallTEXT", font=smallfont ) >>> draw.text( (20,120), "LargeTEXT", font=largefont ) >>> im.save( "multitext.jpg" )
以上就是在PIL 里建立文字图形的方法。
最后,我们要说明如何改变绘制图形(文字)时的颜色;绘图时画笔的颜色是透过draw物件的ink属性来改变的:
>>> draw.ink = 0 + 255*256 + 0*256*256
以上会把画笔设成绿色。ink值必须要是一个整数,其值由色彩的RGB值算出。举几个ink值的例子:
红色的ink值应设为255(R) + 0(G)*256 + 0(B)*256*256,
蓝色的ink值应设为0(R) + 0(G)*256 + 255(B)*256*256,
靛色的ink值应设为0(R) + 255(G)*256 + 255(B)*256*256
所设定的ink会影响所有后续的绘图动作。
相关文章推荐
- python(pil)图像处理(等比例压缩、裁剪压缩) 缩略(水印)图
- python使用pil进行图像处理(等比例压缩、裁剪)实例代码
- Java图片缩略图裁剪水印缩放旋转压缩转格式-Thumbnailator图像处理
- Java图片缩略图裁剪水印缩放旋转压缩转格式-Thumbnailator图像处理
- Java图片缩略图裁剪水印缩放旋转压缩转格式-Thumbnailator图像处理
- Java图片缩略图裁剪水印缩放旋转压缩转格式-Thumbnailator图像处理
- Java图片缩略图裁剪水印缩放旋转压缩转格式-Thumbnailator图像处理
- PHP图片处理函数 类 (水印图,缩略图)[关于等比例压缩与裁剪压缩]
- Java图片缩略图裁剪水印缩放旋转压缩转格式-Thumbnailator图像处理
- python深度学习库pytorch::transforms练习:opencv,scikit-image,PIL图像处理库比较
- 1-python图像处理之PIL,pylab
- 在python3下用PIL做图像处理
- Python 之 使用 PIL 库做图像处理
- python PIL/Pillow图像扩展、复制、粘贴处理
- 安装Python图像处理库PIL、pytesseract进行验证码识别
- 【脚本语言系列】关于Python图像处理PIL,你需要知道的事
- Win7 64位下Python安装PIL图像处理库
- Python PIL图像处理-----图像的手绘效果
- Python图像处理库PIL的ImageGrab模块介绍
- Python图像处理之PIL模块