大话设计模式——装饰模式
2013-04-28 12:44
295 查看
装饰模式:
动态地给一个对象添加一些额外的职责。优点:把类中的装饰功能从类中搬移出去,这样可以简化原有的类。有效地把类的核心功能和装饰功能区分开了。
解决的问题:
已经开发完毕的对象,后期由于业务需要,对旧的对象需要扩展特别多的功能,这时候使用给对象动态地添加新的状态或者行为(即装饰模式)方法,而不是使用子类静态继承。
装饰模式是为已有功能动态地添加更多功能的一种模式。当系统需要新功能时,一般做法是向旧的类中添加新的代码,这些新
加的代码通常影响了原有类的核心职责或行为,在主类中加入新的字段、方法或是逻辑,从而增加了主类的复杂性,而这些新加入
的代码仅仅是为了满足一些只在某种特定情况下才会发生的特殊行为的需要。装饰模式提供了一个非常好的解决方案,它把每个要
装饰的功能放在单独的类中,并让这个类包装它所有要装饰的对象,这样当需要执行特殊行为时,客户代码就可以在运行时根据需
要有选择性地,按顺序的使用装饰功能包装的对象了。
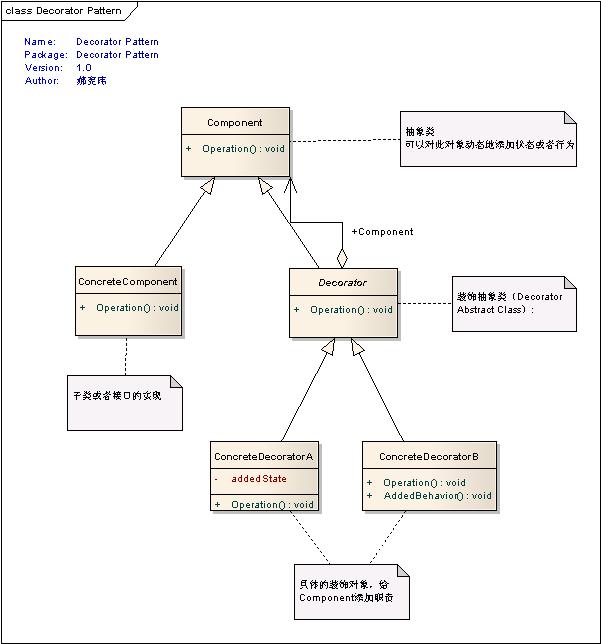
代码如下:
Component.h
#ifndef COMPONENT_H #define COMPONENT_H class Component { public: virtual void Operation() = 0; }; #endif
Concretecomponent.h
#include"Component.h" #ifndef CONCRETECOMPONENT_H #define CONCRETECOMPONENT_H class Concretecomponent : public Component { public: void Operation(); }; #endif
Concretecomponent.cpp
#include<iostream> #include"ConcreteComponent.h" using namespace std; void Concretecomponent::Operation() { cout<<"具体对象的操作"<<endl; }
Decorator.h
#include"Component.h" #ifndef DECORATOR_H #define DECORATOR_H class Decorator : public Component { protected: Component* component; public: void Operation(); void SetComponent(Component* _component); }; #endif
Decorator.cpp
#include<iostream> #include"Decorator.h" using namespace std; void Decorator::Operation() { if(component != NULL) component->Operation(); } void Decorator::SetComponent(Component* _component) { this->component = _component; }
ConcreteDecoratorA.h
#include"Decorator.h" #ifndef CONCRETEDECORATORA_H #define CONCRETEDECORATORA_H class ConcreteDecoratorA : public Decorator { public: void Operation(); private: void AddedBehavior(); }; #endif
ConcreteDecoratorA.cpp
#include<iostream> #include"ConcreteDecoratorA.h" using namespace std; void ConcreteDecoratorA::Operation() { Decorator::Operation(); AddedBehavior(); cout<<"The ConcreteDecoratorA Called"<<endl; } void ConcreteDecoratorA::AddedBehavior() { cout<<"ConcreteDecoratorA AddedBehavior"<<endl; }
ConcreteDecoratorB.h
#include"Decorator.h" #ifndef CONCRETEDECORATORB_H #define CONCRETEDECORATORB_H class ConcreteDecoratorB : public Decorator { public: void Operation(); private: void AddedBehavior(); }; #endif
ConcreteDecoratorB.cpp
#include<iostream> #include"ConcreteDecoratorB.h" using namespace std; void ConcreteDecoratorB::Operation() { Decorator::Operation(); AddedBehavior(); cout<<"ConcreteDecoratorB Operation "<<endl; } void ConcreteDecoratorB::AddedBehavior() { cout<<"ConcreteDecoratorB AddedBehavior Called"<<endl; }
main.cpp
#include<iostream> #include"ConcreteComponent.h" #include"ConcreteDecoratorA.h" #include"ConcreteDecoratorB.h" using namespace std; int main() { Concretecomponent c; ConcreteDecoratorA a; ConcreteDecoratorB b; a.SetComponent(&c); b.SetComponent(&a); b.Operation(); return 0; }
相关文章推荐
- 《大话设计模式》读书笔记4 装饰模式
- 大话设计模式---装饰模式
- 大话设计模式之装饰模式
- 《大话设计模式》读书笔记:装饰模式与均值计算实例
- 《大话设计模式》——读后感 (3)穿什么有这么重要?——装饰模式之理解实例(2)
- 大话设计模式之装饰模式
- 大话设计模式-装饰模式(C++)
- 《大话设计模式》之--第6章 穿什么有这么重要?----装饰模式
- 装饰模式【大话设计模式Demo】
- 大话设计模式之装饰模式
- 大话设计模式_装饰模式
- 大话设计模式之装饰设计模式
- 大话设计模式--装饰模式
- 大话设计模式☞——装饰着模式
- 大话设计模式(四)装饰模式
- 大话设计模式(Python版)--装饰器模式
- 大话设计模式之装饰模式(小菜扮靓)
- 《大话设计模式》读书笔记(C++代码实现) 第六章:装饰模式
- 《大话设计模式》读书笔记(C++代码实现) 第六章:装饰模式
- 第6章 穿什么有这么重要?-装饰模式 大话设计模式