Android入门:使用Android GPS实现简单的定位
2013-02-04 08:53
549 查看
Activity:
GPSLocationListener:
效果如下:
package com.van.gps; import android.app.Activity; import android.app.AlertDialog; import android.app.AlertDialog.Builder; import android.content.Context; import android.content.DialogInterface; import android.content.Intent; import android.location.Criteria; import android.location.Location; import android.location.LocationManager; import android.os.Bundle; import android.provider.Settings; import android.widget.TextView; public class GPSTestActivity extends Activity { private TextView textView;//显示文本框 private LocationManager locationManager;//位置管理 private GPSLocationListener locationListener;//位置监听器 @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); textView = (TextView) this.findViewById(R.id.textView_location); locationListener=new GPSLocationListener(textView); //首先打开GPS,查找位置。 openGPSSettings(); } /** * 设置GPS。 */ private void openGPSSettings() { locationManager = (LocationManager) this.getSystemService(Context.LOCATION_SERVICE); if (locationManager.isProviderEnabled(android.location.LocationManager.GPS_PROVIDER)) { getLocation(); return; } //提示用户打开GPS AlertDialog.Builder builder = new Builder(GPSTestActivity.this); builder.setMessage("必须要开启GPS才能使用此程序,开启?"); builder.setTitle("提示"); builder.setPositiveButton("确认", new android.content.DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int which) { Intent intent = new Intent(Settings.ACTION_LOCATION_SOURCE_SETTINGS); startActivityForResult(intent,0); //此为设置完成后返回到获取界面 } }); builder.setNegativeButton("退出", new android.content.DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int which) { dialog.dismiss(); GPSTestActivity.this.finish(); } }); builder.create().show(); } /** * 获取地理位置。 */ private void getLocation(){ // 查找到服务信息 Criteria criteria = new Criteria(); criteria.setAccuracy(Criteria.ACCURACY_FINE); // 高精度 criteria.setAltitudeRequired(false); criteria.setBearingRequired(false); criteria.setCostAllowed(true); criteria.setPowerRequirement(Criteria.POWER_LOW); // 低功耗 /** * ANDROID中有两种获取位置的方式,LocationManager.NETWORK_PROVIDER和LocationManager.GPS_PROVIDER; * 前者用于移动网络中获取位置,精度较低但速度很快, 后者使用GPS进行定位,精度很高但一般需要10-60秒时 * 间才能开始第1次定位,如果是在 室内则基本上无法定位。 * 此方法使用Criteria得到最佳的方式 */ String provider = locationManager.getBestProvider(criteria, true); // 获取GPS信息 Location location = locationManager.getLastKnownLocation(provider); // 通过GPS获取位置 locationListener.updateLocation(location);//调用方法,更新位置信息 // 设置监听器,1秒监听一次 locationManager.requestLocationUpdates(provider, 1000, 0 ,locationListener); }
GPSLocationListener:
package com.van.gps; import android.location.Location; import android.location.LocationListener; import android.os.Bundle; import android.widget.TextView; public class GPSLocationListener implements LocationListener{ //显示文本 private TextView textView; /** * 构造. * @param textView */ public GPSLocationListener(TextView textView){ this.textView=textView; } @Override public void onLocationChanged(Location location) { updateLocation(location); } @Override public void onProviderDisabled(String provider) { // TODO Auto-generated method stub } @Override public void onProviderEnabled(String provider) { // TODO Auto-generated method stub } @Override public void onStatusChanged(String provider, int status, Bundle extras) { // TODO Auto-generated method stub } /** * 更新位置显示. * @param location */ public void updateLocation(Location location) { if (location != null) { double latitude = location.getLatitude(); double longitude= location.getLongitude(); textView.setText("维度:" + latitude+ "\n经度:" + longitude); } else { textView.setText("无法获取地理信息"); } } }
效果如下:
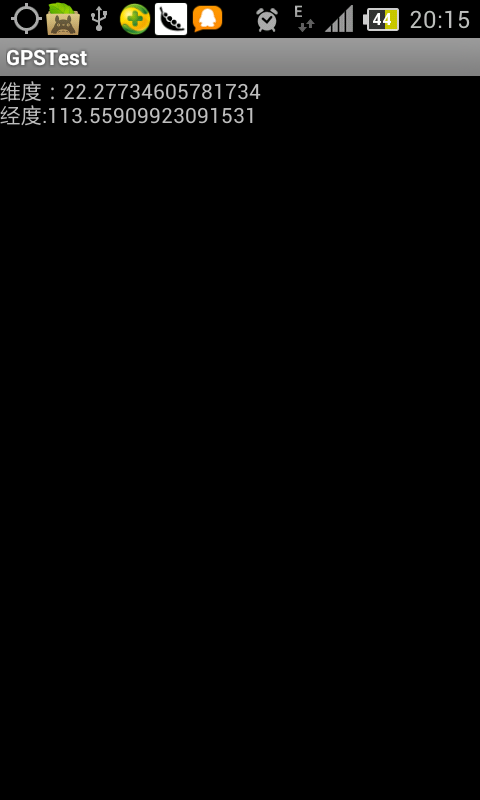
相关文章推荐
- Android无网络状态下使用GPS定位到省市县的一种简单实现方式
- Android上实现一个简单的天气预报APP(十四) 使用百度API定位城市
- Android GPS 定位的实现(2-1) 使用Google地图
- Android入门之简单GPS定位实例
- Android中实现GPS定位的简单例子
- Android 使用高德地图简单实现地图定位
- Android之使用GPS和NetWork定位
- android开源库---Dagger2入门学习(简单使用)
- android使用GPS定位及在googlemap添加标记
- Android应用程序实现定位功能(使用百度定位SDK)
- Android入门(44)——第六章 使用OptionsMenu实现选项菜单
- android使用百度地图、定位SDK实现地图和定位功能!(最新、可用+吐槽)
- Android中使用GPS和NetWork获取定位信息
- Android 实现同个Activity中存在多个Fragment多次切换之后依次返回(一)(Fragment回退栈简单使用)
- Android入门(34)——第十一章 使用ViewFlipper实现屏幕切换动画效果
- android定位之GPS,WIFI和GPRS(NETWORK)方式的定位(含实现源码)
- 使用Android简单实现有道电子词典
- 使用Android简单实现有道电子词典
- android使用socket实现简单的点对点通信
- android中通过实现Parcelable来在两个页面之间传递对象的简单使用