公有继承、保护继承、私有继承总结
2012-11-22 00:00
134 查看
1、公有继承

2、保护继承

3、私有继承

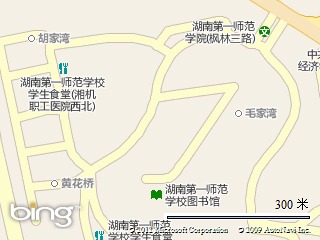

#include <iostream> using namespace std; class base0 { public: void fun0() { _base0_i = 0; } void display() { cout<<"from base0 : _base0_i = "<<_base0_i<<endl; } private: int _base0_i; protected: int _base0_j; }; /*公有继承,父类的公有成员和保护成员在派生类中保 *持原有访问属性,父类私有成员,仍为基类所有*/ class base1: public base0 { public: void fun1() { /* * 公有继承中 * 在派生类中可不可以对父类的私有数据成员、保护数据成员赋值呢? * 由以下的测试可知:父类的私有数据成员不可以,保护数据成员可以。 * 若要对从父类继承而来的父类的私有数据成员赋值,需要通过父类的公有成员函数 */ //_base0_i = 0; //错误,int base0::i0是私有的;PublicInheritance.cpp:15:13: error: 'int base0::i0' is private //base0::_base0_i = 0; //错误,int base0::i0是私有的;PublicInheritance.cpp:15:13: error: 'int base0::i0' is private _base0_j = 0; //公有继承中,可以在派生类函数中对父类的保护数据成员赋值 fun0(); //通过从父类继承而来的公有成员函数可以给从父类继承而来的父类的私有数据成员赋值 _base1_i = 2; _base0_j = 1; } void display() { cout<<"base1 heritance from base0"<<endl; base0::display(); //若不限定display(),将会不停调用display()将不停调用自身(属于base1类),直至栈溢出; cout<<"from base1 : _base1_i = "<<_base1_i<<endl; } private: int _base1_i; protected: int _base1_j; }; void halving_line(int num) { for(int i=0;i<num;i++) { cout<<"-"; } cout<<endl; } void come_from(const char * base) { cout<<"object defined by "<<base<<endl; } int main() { base0 b0; base1 b1; //b0._base0_j = 0; //PrivateInheritance.cpp:17:13: error: 'int base0::_base0_j' is protected //b1._base0_j = 0; //是保护的。PrivateInheritance.cpp:17:13: error: 'int base0::_base0_j' is protected come_from("class base1 "); b1.fun1(); b1.base0::display(); halving_line(60); come_from("class base0 "); b0.fun0(); b0.display(); halving_line(60); come_from("class base1 "); b1.fun1(); b1.display(); halving_line(60); return 0; }
2、保护继承

#include <iostream> using namespace std; class base0 { public: void fun0() { _base0_i = 0; } void display() { cout<<"from base0 : _base0_i = "<<_base0_i<<endl; } private: int _base0_i; protected: int _base0_j; }; /*保护继承,父类的公有成员和保护成员在派生类中成了保护成员, *父类私有成员,仍为基类所有*/ class base1: protected base0 { public: void fun1() { /* * 保护继承中 * 在派生类中可不可以对父类的私有数据成员、保护数据成员赋值呢? * 由以下的测试可知:父类的私有数据成员不可以,保护数据成员可以。 * 若要对从父类继承而来的父类的私有数据成员赋值,需要通过父类的公有成员函数 */ //_base0_i = 0; //错误,int base0::i0是私有的;ProtectedInheritance.cpp:15:13: error: 'int base0::i0' is private //base0::_base0_i = 0; //错误,int base0::i0是私有的;ProtectedInheritance.cpp:15:13: error: 'int base0::i0' is private _base0_j = 0; //保护继承中,可以在派生类函数中对父类的保护数据成员赋值 fun0(); _base1_i = 1; } void display() { cout<<"base1 heritance from base0"<<endl; base0::display(); /**/ cout<<"from base1 : _base1_i = "<<_base1_i<<endl; } private: int _base1_i; private: int _base1_j; }; void halving_line(int num) { for(int i=0;i<num;i++) { cout<<"-"; } cout<<endl; } void come_from(const char * base) { cout<<"object defined by "<<base<<endl; } int main() { base0 b0; base1 b1; //b0._base0_j = 0; //PrivateInheritance.cpp:17:13: error: 'int base0::_base0_j' is protected //b1._base0_j = 0; //是保护的,也是私有的。PrivateInheritance.cpp:17:13: error: 'int base0::_base0_j' is protected come_from("class base1 "); b1.fun1(); //b1.fun0(); //ProtectedInheritance.cpp:70:13: error: 'base0' is not an accessible base of 'base1' //b1.base0::display(); //ProtectedInheritance.cpp:62:15: error: 'base0' is an inaccessible base of 'base1' halving_line(60); come_from("class base0 "); b0.fun0(); b0.display(); halving_line(60); come_from("class base1 "); b1.fun1(); b1.display(); halving_line(60); return 0; }
3、私有继承

#include <iostream> using namespace std; class base0 { public: void fun0() { _base0_i = 0; } void display() { cout<<"from base0 : _base0_i = "<<_base0_i<<endl; } private: int _base0_i; protected: int _base0_j; }; /*私有继承,父类的公有成员和保护成员在派生类中成了私有成员, *父类私有成员,仍为基类所有*/ class base1: private base0 { public: void fun1() { /* * 私有继承中 * 在派生类中可不可以对父类的私有数据成员、保护数据成员赋值呢? * 由以下的测试可知:父类的私有数据成员不可以,保护数据成员可以。 * 若要对从父类继承而来的父类的私有数据成员赋值,需要通过父类的公有成员函数 */ //_base0_i = 0; //错误,int base0::i0是私有的;ProtectedInheritance.cpp:15:13: error: 'int base0::i0' is private //base0::_base0_i = 0; //错误,int base0::i0是私有的;ProtectedInheritance.cpp:15:13: error: 'int base0::i0' is private _base0_j = 0; //私有继承中,父类的保护成员被继承为派生类的私有成员 fun0(); _base1_i = 1; } void display() { cout<<"base1 heritance from base0"<<endl; base0::display(); /**/ cout<<"from base1 : _base1_i = "<<_base1_i<<endl; } private: int _base1_i; private: int _base1_j; }; void halving_line(int num) { for(int i=0;i<num;i++) { cout<<"-"; } cout<<endl; } void come_from(const char * base) { cout<<"object defined by "<<base<<endl; } int main() { base0 b0; base1 b1; //b0._base0_j = 0; //PrivateInheritance.cpp:17:13: error: 'int base0::_base0_j' is protected //b1._base0_j = 0; //是保护的,也是私有的。PrivateInheritance.cpp:17:13: error: 'int base0::_base0_j' is protected come_from("class base1 "); b1.fun1(); //b1.fun0(); //ProtectedInheritance.cpp:70:13: error: 'base0' is not an accessible base of 'base1' //b1.base0::display(); //ProtectedInheritance.cpp:62:15: error: 'base0' is an inaccessible base of 'base1' halving_line(60); come_from("class base0 "); b0.fun0(); b0.display(); halving_line(60); come_from("class base1 "); b1.fun1(); b1.display(); halving_line(60); return 0; }
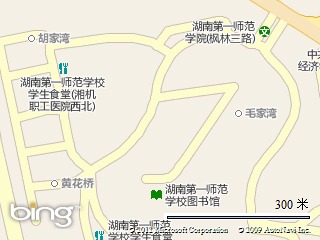
相关文章推荐
- 例4.4 保护继承的访问规则举例
- 共有继承,保护继承,私有继承的区别c++代码实例
- C++继承:共有 私有 保护
- 继承与派生:派生类对基类成员的访问控制之保护继承与私有继承
- C++继承类型:公有、保护、私有、虚拟
- C++继承详解:共有(public)继承,私有(private)继承,保护(protected)继承
- 公有继承、私有继承和保护继承之间的对比
- 输入输出保护继承
- 类的保护继承
- C++继承:公有,私有,保护
- C++继承:公有,私有,保护 http://www.cnblogs.com/qlwy/archive/2011/08/25/2153584.html
- C++继承:公有,私有,保护
- c++ 公有继承、保护继承和私有继承的区别
- C++中公有继承、保护继承、私有继承的区别
- C++中公有继承、保护继承、私有继承的区别
- C#不存在私有继承和保护继承,只有公有继承,这点和C++相比还是简单了很多
- C++笔记——公有继承、私有继承、保护继承、多重继承
- 第十七周oj刷题——Problem F: C++习题 输入输出--保护继承
- 概念:类继承—公有继承、私有继承、保护继承
- 059day(继承和复合关系,覆盖和保护成员)