使用Swing制作类似QQ界面|圆角界面
2012-07-24 10:15
344 查看
制作这个界面需要jdk1.6update10以上的版本,因为使用了透明窗体。
完成的界面如下:
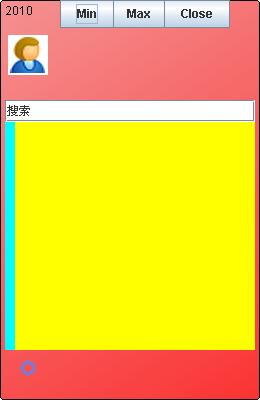
这里只列出部分关键代码:
首先是制作圆角界面:
制作渐变:
界面描边:
最大化按钮:
最小化按钮可以将任务栏隐藏:
下面是最关键的界面控制代码,可以改变界面的位置大小,以及对四个角的拖放操作:
完成的界面如下:
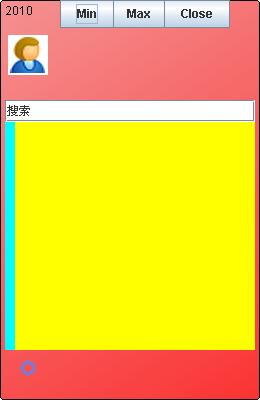
这里只列出部分关键代码:
首先是制作圆角界面:
Shape shape = null; shape = new RoundRectangle2D.Double(0, 0, width, height, 5.5D, 5.5D); AWTUtilities.setWindowShape(frame, shape);
制作渐变:
final Paint p = new GradientPaint(0.0f, 0.0f, new Color(R, G, B, 100),getWidth(), getHeight(), new Color(R, G, B, 200), true);
界面描边:
Shape shape = null; shape = new RoundRectangle2D.Double(0, 0, frame.getWidth()-1, frame.getHeight()-1, 5.0D, 5.0D);//绘制窗体边框 g2d.draw(shape);
最大化按钮:
public void actionPerformed(ActionEvent e) { final Rectangle rec = translucencyCapableGC.getBounds();//获取图形环境桌面大小 Insets d = Toolkit.getDefaultToolkit().getScreenInsets(translucencyCapableGC);//获取桌面工作区大小 frame.setSize(rec.width,rec.height-d.bottom);//设置窗体大小 //重新设定可见区域 setVisibleRegion(frame.getWidth(),frame.getHeight()); frame.repaint(); location=frame.getLocation(); frame.setLocation(0,0); setMax(true); }
最小化按钮可以将任务栏隐藏:
public void actionPerformed(ActionEvent e) { if (isMax()) {//判断是否是最大化状态 //如果是最大化状态则还原最小化时的坐标和值 frame.setSize(size); //重新设定可见区域 setVisibleRegion(frame.getWidth(),frame.getHeight()); frame.repaint(); if (location != null) { frame.setLocation(location); } setMax(false); }else{ //正常状态最小化 setExtendedState(JFrame.ICONIFIED); frame.hide();//隐藏任务栏 } }
下面是最关键的界面控制代码,可以改变界面的位置大小,以及对四个角的拖放操作:
//界面鼠标监听事件 private class MouseInputHandler implements MouseInputListener { private Rectangle r; int chx=0; int chy=0; public void mouseClicked(MouseEvent e) { } public void mouseEntered(MouseEvent e) { } public void mouseExited(MouseEvent e) { } //鼠标按下 public void mousePressed(MouseEvent e) { //记录按下时鼠标的坐标位置 origin.x = e.getX(); origin.y = e.getY(); } public void mouseReleased(MouseEvent e) { } //鼠标拖拽 public void mouseDragged(MouseEvent e) { final Cursor cursor=frame.getCursor();//获取光标显示方式 final Point point=e.getPoint();//获取鼠标当前坐标 //获取界面显示区域 r = frame.getBounds(); //初始化偏移量 chx=0; chy=0; //使用单独的线程控制界面变化 SwingUtilities.invokeLater(new Runnable() { public void run() { //判断光标样式 switch(cursor.getType()){ case Cursor.SE_RESIZE_CURSOR://左上角 //System.out.println("SE_RESIZE_CURSOR"); chx=point.x-origin.x; chy=point.y-origin.y; r.x+=chx; r.y+=chy; r.width-=chx; r.height-=chy; break; case Cursor.SW_RESIZE_CURSOR://右上角 //System.out.println("SW_RESIZE_CURSOR"); chx=point.x-origin.x; chy=point.y-origin.y; origin.x=point.x; origin.y=point.y; r.y+=chy; r.width+=chx; r.height-=chy; break; case Cursor.NE_RESIZE_CURSOR://左下角 //System.out.println("NE_RESIZE_CURSOR"); chx=point.x-origin.x; chy=point.y-origin.y; origin.x=point.x; origin.y=point.y; r.x+=chx; r.width-=chx; r.height+=chy; break; case Cursor.NW_RESIZE_CURSOR://右下角 //System.out.println("NW_RESIZE_CURSOR"); chx=point.x-origin.x; chy=point.y-origin.y; origin.x=point.x; origin.y=point.y; if ((r.width + chx) > frame.getMinimumSize().getWidth()//下边界拉伸高度只能大于最小高度 && (r.height + chy) > frame.getMinimumSize()//下边界拉伸高度只能大于最小高度 .getHeight()) { r.width += chx; r.height += chy; } break; case Cursor.E_RESIZE_CURSOR://左边界 //System.out.println("E_RESIZE_CURSOR"); chx=point.x-origin.x; r.x+=chx; r.width-=chx; break; case Cursor.W_RESIZE_CURSOR://右边界 //System.out.println("W_RESIZE_CURSOR"); chx=point.x-origin.x; origin.x=point.x; if((r.width+chx)>frame.getMinimumSize().getWidth())//下边界拉伸高度只能大于最小宽度 r.width+=chx; break; case Cursor.N_RESIZE_CURSOR://上边界 //System.out.println("N_RESIZE_CURSOR"); chy=origin.y-point.y; r.y-=chy; r.width+=chx; r.height+=chy; break; case Cursor.S_RESIZE_CURSOR://下边界 //System.out.println("S_RESIZE_CURSOR"); chy=point.y-origin.y; origin.y=point.y; if((r.height+chy)>frame.getMinimumSize().getHeight())//下边界拉伸高度只能大于最小高度 r.height+=chy; break; case Cursor.DEFAULT_CURSOR://默认光标样式 //System.out.println("DEFAULT_CURSOR"); r.x+=point.x - origin.x; r.y+=point.y - origin.y; break; } //重新设定可见区域 setVisibleRegion(r.width,r.height); //重新设定界面显示区域 reshape(r.x,r.y,r.width,r.height); repaint(); } }); } public void mouseMoved(MouseEvent e) { Point p=e.getPoint();//获取当前坐标 Point framePoint=new Point(0,0);//设定界面起点坐标 Dimension dim=frame.getSize();//获取界面大小 Rectangle serect=new Rectangle(0,0,3,3);//设定左上角拖动区域 Rectangle swrect=new Rectangle(dim.width-3, 0, dim.width, 3);//设定右上角拖动区域 Rectangle nerect=new Rectangle(0, dim.height-3, 3, dim.height);//设定左下角拖动区域 Rectangle nwrect=new Rectangle(dim.width-3, dim.height-3, dim.width, dim.height);//设定右下角拖动区域 if(serect.contains(p)){//判断光标位置是否在左上角拖动区域 setCursor(Cursor.SE_RESIZE_CURSOR); }else if(swrect.contains(p)){//判断光标位置是否在右上角拖动区域 setCursor(Cursor.SW_RESIZE_CURSOR); }else if(nerect.contains(p)){//判断光标位置是否在左下角拖动区域 setCursor(Cursor.NE_RESIZE_CURSOR); }else if(nwrect.contains(p)){//判断光标位置是否在右下角拖动区域 setCursor(Cursor.NW_RESIZE_CURSOR); }else if(p.x==framePoint.x){//判断光标位置是否在左边界 setCursor(Cursor.E_RESIZE_CURSOR); }else if(p.x==(dim.width-1)){//判断光标位置是否在右边界 setCursor(Cursor.W_RESIZE_CURSOR); }else if(p.y==framePoint.y){//判断光标位置是否在上边界 setCursor(Cursor.N_RESIZE_CURSOR); }else if(p.y==(dim.height-1)){//判断光标位置是否在下边界 setCursor(Cursor.S_RESIZE_CURSOR); }else{//判断光标位置是否在窗口中 setCursor(Cursor.DEFAULT_CURSOR); } } }
相关文章推荐
- 使用Swing制作类似QQ界面|圆角界面
- Swing制作类似QQ的自动隐藏界面
- 使用WPF制作一个类似QQ的界面
- 使用WPF制作一个类似QQ的界面
- Swing制作高仿QQ界面包含主界面、聊天窗口、系统设置窗口|圆角界面|透明|颜色|渲染|换肤
- 使用 electron 实现类似新版 QQ 的登录界面效果(阴影、背景动画、窗体3D翻转)
- Swing学习----------QQ登录界面制作(一)
- Swing学习----------QQ登录界面制作(二)
- Swing学习----------QQ登录界面制作(一)
- 使用 electron 实现类似新版 QQ 的登录界面效果(阴影、背景动画、窗体3D翻转)
- Swing学习----------QQ登录界面制作(二)
- java在线聊天项目 使用SWT快速制作登录窗口,可视化窗口Design 更换窗口默认皮肤(切换Swing自带的几种皮肤如矩形带圆角)
- Android应用中使用ViewPager实现类似QQ的界面切换效果
- 特殊类型窗体制作: 实现类似 QQ 的程序界面
- 使用ViewPager实现几个界面的切换,类似QQ
- 制作一个类似QQ第三方登陆的sdk平台(安卓版)
- ViewPager+Fragment的结合使用,实现QQ界面的理解
- C#+ html 实现类似QQ聊天界面的气泡效果
- MFC 列表控件CListCtrl加载类似QQ界面的头像与文字
- 类似QQ聊天界面