android学习----基础UI编程(二)之TextView 和 EditView(转)
2012-03-18 13:15
666 查看
android学习----基础UI编程(二)(转)
7. TextView 和 EditView 共舞预达到效果:在EditText中输入同时TextView进行输出
//前提:在main.xml中添加EditText 和 TextView控件
核心代码示例:
public class EX_Ctrl_1
extends Activity {
private TextView mTextView01;
private EditText mEditText01;
// Called when the activity is first created.
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
// 取得TextView、EditText
mTextView01 = (TextView) findViewById(R.id.myTextView);
mEditText01 = (EditText) findViewById(R.id.myEditText);
// 设置EditText 用OnKeyListener 事件来启动
mEditText01.setOnKeyListener(new EditText.OnKeyListener(){
@Override
public boolean onKey(View v,
int keyCode, KeyEvent event) {
// TODO Auto-generated method stub
//当有key事件时,就获取当前edit的text 显示到 textview 上
mTextView01.setText(mEditText01.getText().toString());
return false;
}
});
}
}
关键点
1. View.setOnKeyListener
接口原型:
public void
setOnKeyListener(View.OnKeyListener
l)
Register a callback to be invoked when a key is pressed in this view.
参数:
l : | the key listener to attach to this view |
---|
ImageButton 的背景图片设置方法
1) ImageButton.setImageResource(资源ID)
或
ImageButton.setImageDrawable
(Drawable drawable)
或

2) XML文件中 <ImageButton> 的
android:src 或 android:background 属性
1)在 ImageButton 的焦点和事件处理中,通过 setImageResource 进行图片设置
示例代码
① 新建工程
② 准备png 图片资源clickimage.png、lostfocusimage.png、onfocusimage.png

③ 在string.xml 中添加字符串
<?xml
version="1.0" encoding="utf-8"?>
<resources>
<string name="app_name">EX_Ctrl_2</string>
<string name="unknown">图片按钮状态:未知</string>
<string
name="onfocus">图片按钮状态:Got Focus</string>
<string name="lostfocus">图片按钮状态:Lost Focus</string>
<string name="onclick">图片按钮状态:Got Click</string>
<string name="normalbutton">一般按钮</string>
</resources>
④ 修改main.xml 布局,添加布局元素
<?xml version="1.0"
encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#EE559611">
<TextView
android:id="@+id/show_TextView"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/unknown"
/>
<ImageButton
android:id="@+id/image_Button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
/>
<Button
android:id="@+id/normal_Button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/normalbutton"
/>
</LinearLayout>
⑤ 修改mainActivity.java
package zyf.EX_Ctrl_2;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.view.View.OnFocusChangeListener;
import android.widget.Button;
import android.widget.ImageButton;
import android.widget.TextView;
public class EX_Ctrl_2
extends Activity {
// Called when the activity is first created.
// 声明三个对象变量(图片按钮,按钮,与TextView)
private ImageButton mImageButton1;
private Button mButton1;
private TextView mTextView1;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
// 通过findViewById 构造三个对象
mImageButton1 = (ImageButton) findViewById(R.id.image_Button );
mButton1 = (Button) findViewById(R.id.normal_Button );
mTextView1 = (TextView) findViewById(R.id.show_TextView);
// 通过OnFocusChangeListener 来响应ImageButton 的onFous 事件
mImageButton1.setOnFocusChangeListener(new
OnFocusChangeListener() {
public void
onFocusChange(View arg0, boolean isFocused) {
// TODO Auto-generated method stub
//若ImageButton 状态为onFocus 改变ImageButton 的图片
//并改变textView 的文字
if (isFocused == true) {
mTextView1.setText(R.string.onfocus);
mImageButton1.setImageResource(R.drawable.onfocusimage);
} //若ImageButton 状态为offFocus 改变ImageButton 的图片并改变textView 的文字
else {
mTextView1.setText(R.string.lostfocus );
mImageButton1.setImageResource(R.drawable.lostfocusimage);
}
}
});
//通过onClickListener 来响应ImageButton 的onClick 事件
mImageButton1.setOnClickListener(new
OnClickListener() {
public void
onClick(View v){
// TODO Auto-generated method stub
//若ImageButton 状态为onClick 改变ImageButton 的图片并改变textView 的文字
mTextView1.setText(R.string.onclick);
mImageButton1.setImageResource(R.drawable.clickimage);
}
});
//通过onClickListener 来响应Button 的onClick 事件
mButton1.setOnClickListener(new OnClickListener(){
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
//若Button 状态为onClick 改变ImageButton 的图片 并改变textView 的文字
mTextView1.setText(R.string.lostfocus);
mImageButton1.setImageResource(R.drawable.lostfocusimage);
}
});
}
}
⑥ 结果

关键点
1. ImageButton
Displays a button with an image (instead of text) that can be pressed or clicked by the user.
By default, an ImageButton looks like a regular(普通的) Button, with the standard(标准) button background that changes color during different button states.
2. ImageButton 的焦点切换监听
实现继承于 android.view.view 的接口:
public void
setOnFocusChangeListener
(View.OnFocusChangeListener l)
Register a callback to be invoked when focus of this view changed
参数:
l :The callback that will run。 在这个里面还要重写 OnFocusChange 方法。
3. ImageButton 的点击监听
方法一:
实现继承于 android.view.view 的接口:
public void
setOnClickListener
(View.OnClickListener l)
Register a callback to be invoked when this view is clicked. If this view is not clickable, it becomes clickable.
参数:
l :The callback that will run。
在这个里面还要重写 OnClick 方法。
方法二:
在XML文件中,设置<ImageButton>的
android:onClick 属性。
通过button的android:onClick属性指定一个方法,以替代在activity中为button设置OnClickListener。例如:
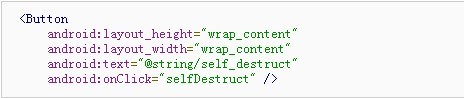
现在,当用户点击按钮时,Android 系统调用 activity 的 selfDestruct(View) 方法。为了正确执行,这个方法必须是public并且仅接受一个View类型的参数。例如:

PS.
继承于 android.view.view 的接口另有
setOnKeyListener setOnTouchListener ...等
2)使用
res/drawable/文件夹下的
XML文件实现按钮状态改变时的背景图设置,赋该XML的ID给<ImageButton>的
android:src
或
android:background
属性
前提须知,在XML文件中设置按钮背景图片:onFocus() 与onClick() 事件处理 分别对应 <Item> 标签的android:state_focused 和 android:state_pressed 属性。
示例代码
① 新建工程
② 准备png 背景图片defaultimage.png 、onfocusimage.png、clickimage.png

③在 res/drawable 文件夹中添加一个 advancedbutton.xml 设置<selector> 和 <item>标签:

④ 设置<ImageButton> 的android:background 属性值

这就OK了~
关键点
1.
<selector> 和
<item>标签
<selector>元素:背景选择器。配置于
res/drawable/****.xml
。在系统使用该 xml
文件时,根据 selector
中的列表项的状态来使用相应的背景图片。
例如如下
res/drawable/list_item_bg.xml
<?xml
version="1.0"
encoding="utf-8"
?>
<selector
xmlns:android="http://schemas.android.com/apk/res/android">
//默认时的背景图片
<item
android:drawable="@drawable/pic1"
/>
//没有焦点时的背景图片
<item
android:state_window_focused="false"
android:drawable="@drawable/pic1"
/>
//非触摸模式下获得焦点并单击时的背景图片
<item
android:state_focused="true"
android:state_pressed="true"
android:drawable=
"@drawable/pic2"
/>
//触摸模式下单击时的背景图片
<item
android:state_focused="false"
android:state_pressed="true"
android:drawable="@drawable/pic3"
/>
//选中时的图片背景
<item
android:state_selected="true"
android:drawable="@drawable/pic4"
/>
//获得焦点时的图片背景
<item
android:state_focused="true"
android:drawable="@drawable/pic5"
/>
</selector>
如何使用这种xml文件:
第一种:是在布局文件中配置:
android:listSelector="@drawable/list_item_bg
或者在布局文件的item中添加属性:
android:background=“@drawable/list_item_bg"即可实现。
第二种:是在Java代码中使用:
Drawable drawable = getResources().getDrawable(R.drawable.list_item_bg);
ListView.setSelector(drawable);同样的效果。
但是这样会出现列表有时为黑的情况,需要加上:android:cacheColorHint="@android:color/transparent"
使其透明。
其次再来看看Button的一些背景效果
android:state_selected是选中
android:state_focused是获得焦点
android:state_pressed是点击
android:state_enabled是设置是否响应事件,指所有事件
根据这些状态同样可以设置button的selector效果。也可以设置selector改变button中的文字状态。
以下就是配置button中的文字效果:
<?xml
version="1.0"
encoding="utf-8"?>
<selector
xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:state_selected="true"
android:color="#FFF"
/>
<item
android:state_focused="true"
android:color="#FFF"
/>
<item
android:state_pressed="true"
android:color="#FFF"
/>
<item
android:color="#000"
/>
</selector>
相关文章推荐
- Android学习-常见的UI控件 TextView、EditText和ImageView
- Android常用UI编程_TextView实现跑马灯效果
- Android基础学习 - 简单控件,EditText 、 Button :
- android基础知识学习(1) TextView属性大全+单行显示长文本
- 【幻化万千戏红尘】qianfeng-Android-Day04-Spinner、ScrollVie、AutoCompleteTextView,Activity初步认识基础学习:
- android学习中关于Textview,Button,EditText,连接的设置,Intent,Activity不同状态等的一系列基础知识点
- 【Android UI控件】EditText属性大全,TextView属性大全
- Android UI学习之TextView
- Android之UI学习篇五:AutoCompleteTextView自动完成输入内容
- Android常用UI编程_TextView实现Activity转变
- 【Android基础学习】TextView参数设置
- Android学习 11 ->控件之TextView和EditText
- Android 开发基础篇——UI——基础控件(TextView、Button、EditText)
- Android UI学习之EditText
- 【android基础学习之四】——基础控件CheckBox,Spinner,AutoCompleteTextView,DatePicker,TimePicker
- Android基础学习总结(六)——TextInputLayout+EditText 轻松实现登录界面
- [Android--UI]TextView的使用学习
- Android基础UI控件之AutoCompleteTextView
- Android UI 设计之 TextView EditText 组件属性方法最详细解析
- [Android新手学习笔记11]-UI控件之TextView