简单的银行存款取款业务模拟程序
2011-11-26 23:30
260 查看
实验三 静态成员和友元函数
一、实验目的和任务1) 熟练掌握友元函数设计的方法
2)掌握友元函数的含义,友元函数和成员函数的区别。
二、实验原理介绍
根据要求正确定义静态成员和友元函数。将别的模块声明为友元,使类中本隐藏的信息如私有和保护成员就可以被友元访问。

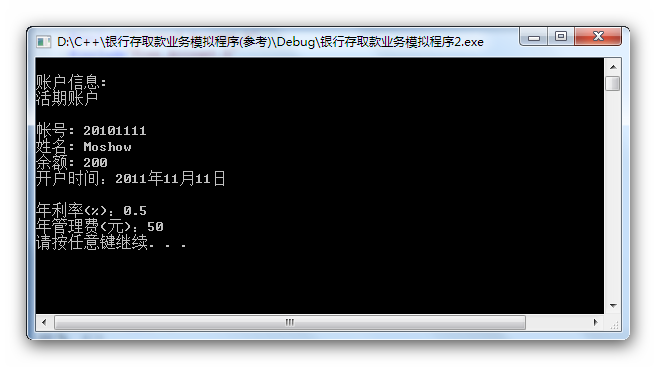
三、实验内容和步骤
【实验3-1】 静态成员
为账户类ACCOUNT增加静态成员count和 InterestRate表示账户数量和利率,
增加静态成员函数GetCount返回账户数目 GetInterestRate返回银行利率
class ACCOUNT {
public:
ACCOUNT(string accno,string name,float balance);
~ACCOUNT();
void Deposit(float amount);
void Withdraw(float amount);
float GetBalance();
void Show(void);
static int GetCount();
static float GetInterestRate();
private:
static int count;
static float InterestRate;
string _accno,_accname;
float _balance;
};
【实验3-2】 友元函数
为账户类ACCOUNT增加一个友元函数,实现账户结息,要求输出结息后的余额(不计算利息税),并在main函数中调用进行测试。
friend void Update(ACCOUNT& a);
【实验3-3】(加分题)
在main函数中,设计一个ACCOUNT类型的数组,为数组中的10个对象初始化,并调用成员函数测试存款、取款、显示等函数,再调用友元函数测试进行结息。
n ACCOUNT accArray[10]; //静态数组的方法
n ACCOUNT * accArray= new ACCOUNT[10]; //动态数组的方法
cout<<"帐户数目:"<<ACCOUNT::GetCount()<<endl;
注:
1. 用以上方法时,会调用缺省构造函数,此时应将构造函数修改成缺省构造函数,例如:
ACCOUNT::ACCOUNT(string accno="0000",string accname="bank",float balance=0.0f)
2. 对各对象再进行设置时,可增加一个setData函数,用于设置账号、姓名和余额。
【Account.h】
#include<iostream>
#include<cmath>
#include<string>
using namespace std;
class Limited_Account;
class Free_Account;
struct Date
{
double year;
double month;
double day;
};
class Account
{
public:
Account(){}
~Account(){}
void inputData();
void input_Endingtime();
double GetBalance();
double Gettime();
void Show();
void Deposit();
void Withdraw(double,double);
private:
string _accno;
string _accname;
double _balance;
Date Beginning;
Date Ending;
friend Limited_Account;
friend Free_Account;
};
void Account::Deposit()
{
double Deposit;
cout<<"请输入存款金额:";
for(;;)
{
cin>>Deposit;
if(Deposit>=0) break;
else cout<<"输入错误。\n请重新输入:\n";
}
_balance+=Deposit;
cout<<"\n当前账户余额为:"<<_balance<<"元。\n";
system("pause");
}
void Account::Withdraw(double x,double y)
{
double withdraw;
cout<<"\n请输入取款金额:";
for(;;)
{
cin>>withdraw;
if(withdraw<=(x-y)) break;
else cout<<"\n无法透支。\n请重新输入:";
}
_balance=x-withdraw;
cout<<"\n当前金额为:"<<_balance<<"元。\n";
system("pause");
}
void Account::inputData()
{
cout<<"\n开户时间\n年:";
cin>>Beginning.year;
cout<<"月:";
cin>>Beginning.month;
cout<<"日:";
cin>>Beginning.day;
cout<<"\n账号:";
cin>>_accno;
cout<<"姓名:";
cin>>_accname;
cout<<"账户余额(元):";
cin>>_balance;
}
void Account::input_Endingtime()
{
cout<<"\n取款时间\n年:";
cin>>Ending.year;
cout<<"月:";
cin>>Ending.month;
cout<<"日:";
cin>>Ending.day;
}
double Account::GetBalance()
{
return _balance;
}
double Account::Gettime()
{
double Time=0,a=0,b=0;
if(Ending.month<Beginning.month && (Ending.year-Beginning.year)>=1)
{
a=Ending.year-Beginning.year-1;
b=(Ending.month+12-Beginning.month)/12;
Time=a+b;
}
else if(Ending.month>=Beginning.month)
{
a=Ending.year-Beginning.year;
b=(Ending.month-Beginning.month)/12;
Time=a+b;
}
return Time;
}
void Account::Show()
{
cout<<"\n帐号: "<<_accno<<endl
<<"姓名: "<<_accname<<endl
<<"余额: "<<_balance<<endl
<<"开户时间:"<<Beginning.year
<<"年"<<Beginning.month
<<"月"<<Beginning.day<<"日"<<endl;
}
【Free_Account.h】
#ifndef ACCOUNT
#define ACCOUNT
#include"Account.h"
#endif
class FreeAccount:public Account
{
private:
double FInterestRate;
double ManagingFee;
double Interest;
public:
FreeAccount(){}
~FreeAccount(){}
void FinputData();
void Fshow();
void Deposit();
void Withdraw();
double GetInterest();
friend int main();
};
void FreeAccount::FinputData()
{
cout<<"\n请输入账户信息:\n";
Account::inputData();
cout<<"年管理费(元):";
cin>>ManagingFee;
}
void FreeAccount::Fshow()
{
cout<<"\n账户信息:\n"
<<"活期账户\n";
Account::Show();
FInterestRate=0.50;
cout<<"\n年利率(%):"<<FInterestRate
<<"\n年管理费(元):"<<ManagingFee<<endl;
system("pause");
}
void FreeAccount::Deposit()
{
Account::Deposit();
}
void FreeAccount::Withdraw()
{
//Account::input_Endingtime();
double t=Account::Gettime();
double x=Account::GetBalance();
double I=1+FInterestRate/100;
x=x*pow(I,t);
Account::Withdraw(x,ManagingFee);
}
double FreeAccount::GetInterest()
{
double a=Account::GetBalance();
Interest=a*FInterestRate;
return Interest;
}
【Limited_Account.h】
#ifndef ACCOUNT
#define ACCOUNT
#include"Account.h"
#endif
class LimitedAccount:public Account
{
private:
double LInterestRate;
double ManagingFee;
double Interest;
double Ltime;
public:
LimitedAccount(){}
~LimitedAccount(){}
void LinputData();
void Lshow();
void Deposit();
void Withdraw();
double GetInterest();
friend int main();
};
void LimitedAccount::LinputData()
{
cout<<"\n请输入账户信息\n";
Account::inputData();
cout<<"存储年限:";
cin>>Ltime;
cout<<"年管理费:";
cin>>ManagingFee;
}
void LimitedAccount::Lshow()
{
cout<<"账户信息:\n"
<<"\n定期账户\n";
Account::Show();
if (Ltime<=0.25) LInterestRate=0;
else if (Ltime<=0.5) LInterestRate=2.85;
else if (Ltime<=1) LInterestRate=3.05;
e
4000
lse if (Ltime<=2) LInterestRate=3.25;
else if (Ltime<=3) LInterestRate=4.15;
else if (Ltime<=5) LInterestRate=4.75;
else LInterestRate=5.25;
cout<<"存储年限:"<<Ltime
<<"\n年利率(%):"<<LInterestRate
<<"\n年管理费(元):"<<ManagingFee<<endl;
system("pause");
}
void LimitedAccount::Deposit()
{
Account::Deposit();
}
void LimitedAccount::Withdraw()
{
Account::input_Endingtime();
double t=Account::Gettime();
cout<<"已存时间为:"<<t<<"年"<<endl;
double x=Account::GetBalance();
if(t<Ltime)
{
char a;
cout<<"存储时间不足"<<Ltime<<"年。\n若仍要取款将按活期利率进行结息!\n是否继续取款(y/n):";
cin>>a;
if(a=='y')
{
LInterestRate=0.5;
double I=1+LInterestRate/100;
x=x*pow(I,t);
Account::Withdraw(x,ManagingFee);
}
}
else if(t>=Ltime)
{
double I=1+LInterestRate/100;
x=x*pow(I,Ltime);
LInterestRate=0.5;
I=1+LInterestRate/100;
x=x*pow(I,t-Ltime);
Account::Withdraw(x,ManagingFee);
}
}
double LimitedAccount::GetInterest()
{
double a=Account::GetBalance();
Interest=a*LInterestRate;
return Interest;
}
【main.cpp】
#include"Limited_Account.h" #include"Free_Account.h" int main() { FreeAccount F; LimitedAccount L; int a=3; while(1) { cout<<"主菜单\n" <<"(1)活期账户\n" <<"(2)定期账户\n" <<"(3)查询账户信息\n" <<"(4)存款\n" <<"(5)取款\n" <<"(6)退出系统\n"; int userChoice; for(;;) { cin>>userChoice; if (a!=1 && a!=0 && userChoice!=1 && userChoice!=2 && userChoice!=6) { system("cls"); cout<<"未创建帐户,请选择:\n(1)创建活期帐户\n(2)创建定期帐户。\n(6)退出程序。"<<endl; } else break; } system("cls"); switch(userChoice) { case 1:F.FinputData();a=1;break; case 2:L.LinputData();a=0;break; case 3: { if(a==1) F.Fshow(); else L.Lshow(); } break; case 4: { if(a==1) F.Deposit(); else L.Deposit(); } break; case 5: { if(a==1) F.Withdraw(); else L.Withdraw(); } break; case 6:return 0; default:return 0; } system("cls"); } }
相关文章推荐
- 一个简单的计算器模拟程序-2011腾讯笔试填空题
- c语言:模拟实现一个输入密码自动取款的程序
- 【练习题】构造方法 编写Java程序,模拟简单的计算器。
- [c语言]模拟银行atm机,实现存款、取款等业务
- 简单的扫描枪模拟程序
- Android模拟内存紧张,应用被杀死如何模拟应用程序被杀掉? 更新:Daniel Lew指出,最简单的方法是在DDMS中点击”Stop Porcess”杀掉你的程序,在你调试程序的时候可以这样做。
- 十字路口交通简单模拟程序
- 十字路口交通简单模拟程序
- 模拟一个简单的基于tcp的远程关机程序
- 一个模拟简单的物品掉落的程序
- 模拟一个简单的基于tcp的远程关机程序
- python简单模拟数据库程序
- MFC实现简单的键盘模拟程序
- 简单实现stm32f103芯片usb模拟U盘进行IAP更新用户程序
- WinForm学习 --简单的模拟时钟程序
- 简单模拟qq聊天程序(TCP版)
- 实现一个简单的银行储蓄系统,承担活期用户的存款和取款业务 (只是初步的写出)
- 【C语言】没事可以试试这个小程序,使用文件操作,模拟实现一个简单的文件拷贝工具!
- c语言:模拟实现一个输入密码自动取款的程序
- strophe与openfire模拟的XMPP简单hello程序