队列的入队,出队,测长,打印操作
2011-09-23 17:40
363 查看
#include "stdafx.h" #include <iostream> //队列 using namespace std; typedef struct node{ node *next;//指向链表下一个节点 int data; }node; //node表示队列中的每个节点元素,queue表示队列 typedef struct queue { node *front;//队首 node *rear;//队尾 }queue; //创建空队列 queue *CreateQueue(){ queue *q=new queue; q->front=NULL;//把队首指针置空 q->rear=NULL;//把队尾指针置空 return q; } //入队,从队尾一端插入节点 queue *EnQueue(queue *q,int data){ if (q==NULL){//如果指针为NULL,返回NULL return NULL; } node *pnode=new node; pnode->data=data; pnode->next=NULL; if (q->rear==NULL){//如果队列为空,则新节点即是队首又是队尾 q->rear=q->front=pnode; }else{ //如果队列不为空,新节点放在队尾,队尾指针指向新节点 q->rear->next=pnode; //末尾节点的指针指向新节点 q->rear=pnode; //末尾指针指向新节点 } return q; } //出队,从队头一端删除节点 queue *QuQueue(queue *q){ node *pnode=NULL; pnode=q->front; //指向队头 if (pnode==NULL){ //如果队列为空,则返回NULL cout<<"Empty queue!\n"; return NULL; } q->front=q->front->next; //把头节点的下一个节点作为头节点 if (q->front==NULL){ //若删除后队列为空,需对rear置空 q->rear=NULL; } delete pnode; //释放内存 return q; } //队列的测长 int GetLength(queue *q){ if (q==NULL || q->rear==NULL){ return 0; } int i=1; node *pnode=q->front; while (pnode->next!=NULL){ pnode=pnode->next; i++; } return i; } //队列的打印 void Print(queue *q){ node *pnode=q->front; if (pnode==NULL){//如果队列为空,直接返回 cout<<"Empty queue!\n"; return; } while (pnode!=NULL){ cout<<pnode->data<<" "; pnode=pnode->next; } cout<<endl; } int _tmain(int argc, _TCHAR* argv[]) { queue *pA=NULL; pA=CreateQueue(); pA=EnQueue(pA,2); pA=EnQueue(pA,3); pA=EnQueue(pA,4); pA=EnQueue(pA,5); Print(pA); cout<<"The Length:"<<GetLength(pA)<<endl; pA=QuQueue(pA); pA=QuQueue(pA); Print(pA); cout<<"The Length:"<<GetLength(pA)<<endl; system("pause"); delete [] pA; return 0; }
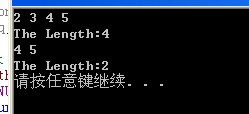
相关文章推荐
- 队列的入队,出队,测长,打印操作 .
- 队列的入队,出队,测长度,打印c++代码
- 实验C—3 顺序队列基本操作入队与出队
- 数据结构之队列的基本操作入队出队初始化删除-c++代码实现及运行实例结果
- 用栈实现队列入队出队操作
- 队列的入队,出队,测长度,打印c++代码
- 实验C—4 链队列基本操作出队与入队
- 编程实现队列的入队/出队操作
- 数据结构之队列的基本操作入队出队初始化删除-c++代码实现及运行实例结果
- 队列的入队,出队,测长度,打印c++代码
- 两个栈实现一个队列,并实现队列入队、出队、取队头、取队尾相关操作
- 数据结构之队列的基本操作入队出队初始化删除-c++代码实现及运行实例结果
- c之队列相关操作------初始化,入队,出队,队列清空,销毁,遍历
- c语言:用双向链表实现双端队列(队列两端都可以进行入队出队操作)
- 循环队列的定义、入队、出队等操作 C++代码实现
- 队列的入队,出队,测长度,打印c++代码
- 数据结构之队列的基本操作入队出队初始化删除-c++代码实现及运行实例结果
- 利用顺序存储结构实现双端队列的入队和出队操作
- C++实现普通队列,循环队列的基本操作(初始化,入队,出队,获取队列首元素等)
- 队列的入队,出队,测长度,打印c++代码