Jquery读取.Net WebService Json数据 推荐
2010-12-10 21:10
274 查看
鉴于上篇文章中提到的Json优点:易读性、可扩展性和操作方便,接下来我们实现一个简单的例子Demo,场景是:查询商品信息;实现过程:Web程序通过Jquery调用WebService,Web Service返回Json对象,成功调用后,对返回的JSon结果进行处理,下面我们看一下具体实现过程:
1、 首先创建一个Aap.net web 应用程序,如下图:
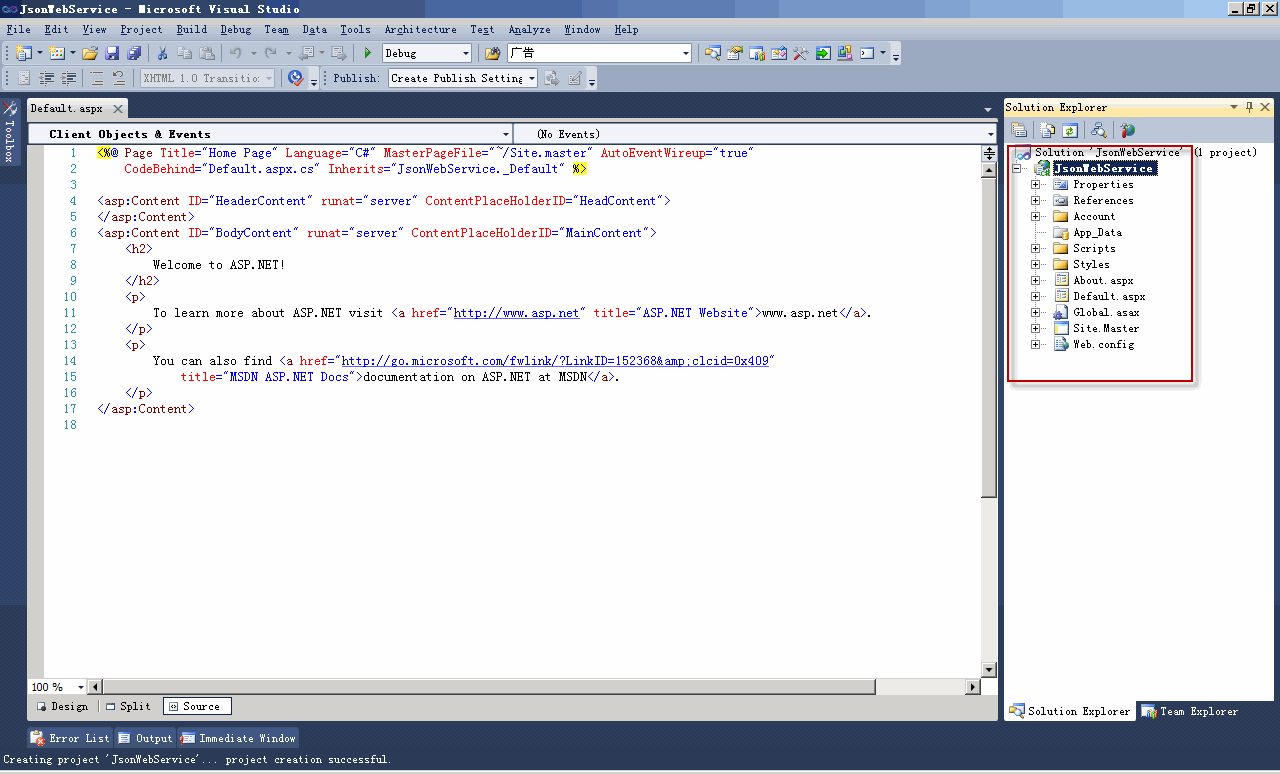
2、 添加实体类Product.cs到工程中,并且序列化,代码如下
3、 添加数据类ProductData.cs类到工程中,此类主要完成数据的查询操作,代码如下所示:
4、 添加ASP.NET Web Service 到工程中,命名为ProductServic.asmx,如下图:
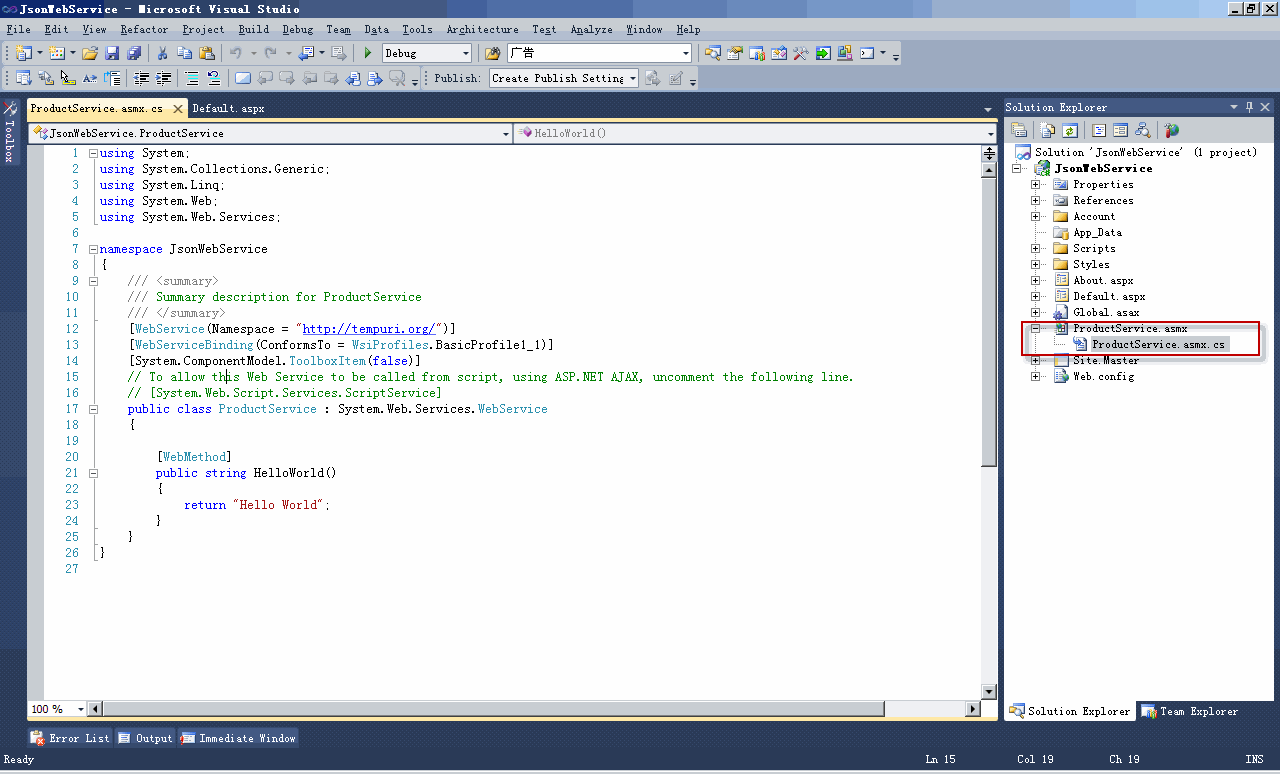
此Web Service调用ProductData类,完成数据查询操作,具体代码如下:
注:
Ø 引用命名空间:System.Web.Script.Services.ScriptService
Ø 给方法添加注解:[ScriptMethod(ResponseFormat = TheResponseFormat, UseHttpGet = true/false)]
ResponseFormat:方法要返回的类型,一般为Json或者XML
UseHttpGet:true表示“Get”可以访问此方法
5、 在Defualt.aspx页面引用Jquery类库
引用Google网络jquery 类库http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.js
或者本地类库<script src="Scripts/jquery-1.4.1.js" type="text/javascript"></script>
6、 在Defualt.aspx页添加如下HTML代码:
Jquery会动态向id=ProductList的Table中添加查询到的数据
7、 在Defualt.aspx页添加如下样式代码:
8、 使用Jquery的Ajax方法调用Web Service中方法,代码如下:
注:
$.ajax方法有以下属性:
Type: HTTP请求方法,在做查询操作时,经常用Get方法
contentType:在请求头部的类型,由于Web Service返回Json对象,此处值为:application/json; charset=utf-8
url:请求的URL地址
dataTyep:定义返回的类型
Success:调用成功时,要执行的方法
error:调用失败是,要执行的方法
9、 执行程序,效果如下:
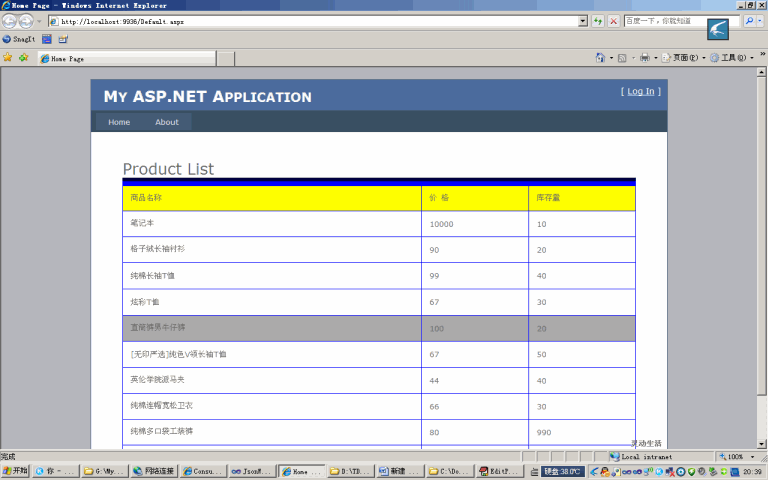
至此,使用Jquery查询Web Service返回的JSon对象已经完成
源代码下载地址:http://files.cnblogs.com/ywqu/JsonWebService.rar
1、 首先创建一个Aap.net web 应用程序,如下图:
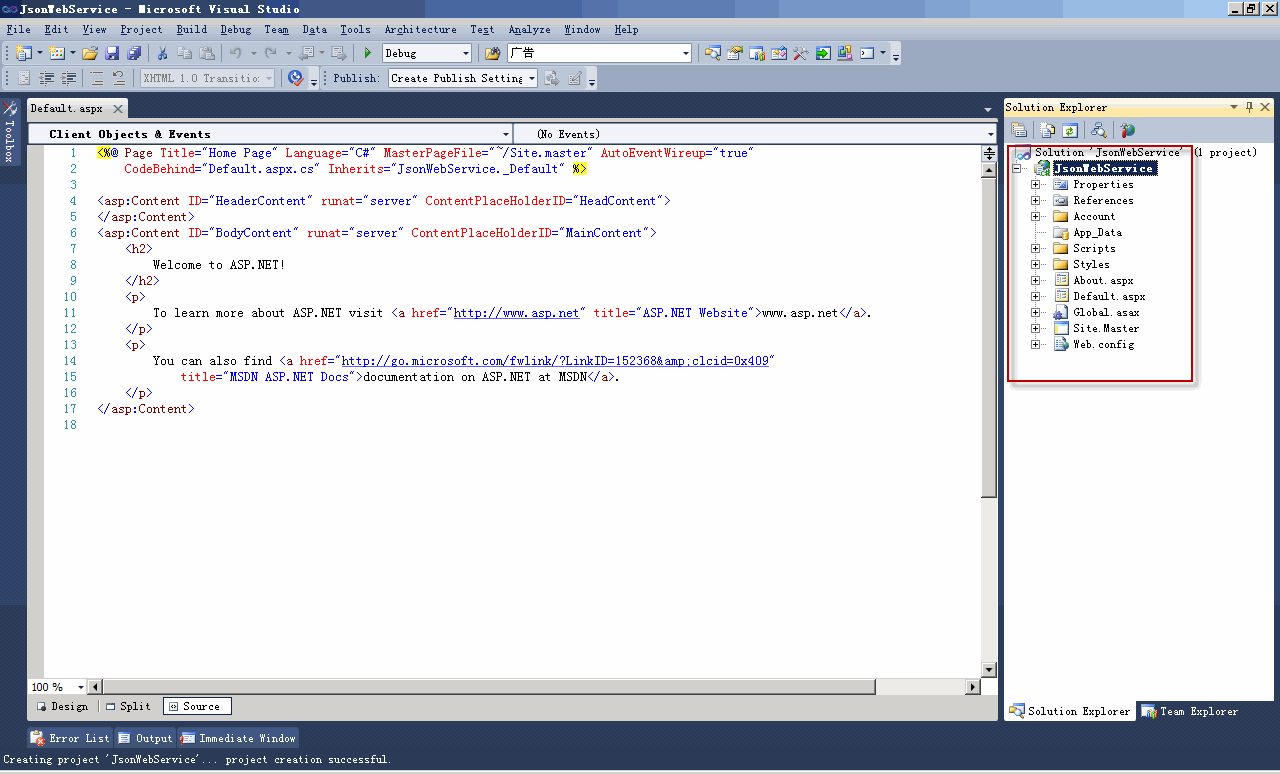
2、 添加实体类Product.cs到工程中,并且序列化,代码如下
[Serializable] public class Product { public long ProductId { get; set; } public string ProductName { get; set; } public decimal Price { get; set; } public int Stock { get; set; } } |
public class ProductData { private List<Product> productList = new List<Product>() { new Product(){ProductId=1,ProductName="笔记本", Price=10000M, Stock=10}, new Product(){ProductId=2,ProductName="格子绒长袖衬衫", Price=90M, Stock=20}, new Product(){ProductId=3,ProductName="纯棉长袖T恤", Price=99M, Stock=40}, new Product(){ProductId=4,ProductName="炫彩T恤", Price=67M, Stock=30}, new Product(){ProductId=5,ProductName="直筒裤男牛仔裤", Price=100M, Stock=20}, new Product(){ProductId=6,ProductName="[无印严选]纯色V领长袖T恤", Price=67M, Stock=50}, new Product(){ProductId=7,ProductName="英伦学院派马夹", Price=44M, Stock=40}, new Product(){ProductId=8,ProductName="纯棉连帽宽松卫衣", Price=66M, Stock=30}, new Product(){ProductId=9,ProductName="纯棉多口袋工装裤", Price=80M, Stock=990}, new Product(){ProductId=10,ProductName="假两件长袖T恤", Price=89M, Stock=30}, }; /// <summary> /// 查询所有商品 /// </summary> /// <returns>所有商品</returns> public List<Product> GetProducts() { return productList; } /// <summary> /// 根据商品Id查询商品 /// </summary> /// <param name="id">商品编号</param> /// <returns>商品</returns> public Product GetProductById(long id) { return productList.FirstOrDefault(p => p.ProductId == id); } /// <summary> /// 根据商品名称查询商品 /// </summary> /// <param name="id">商品名称</param> /// <returns>商品</returns> public List<Product> GetProductByName(string name) { return productList.Where(p => p.ProductName == name).ToList(); } |
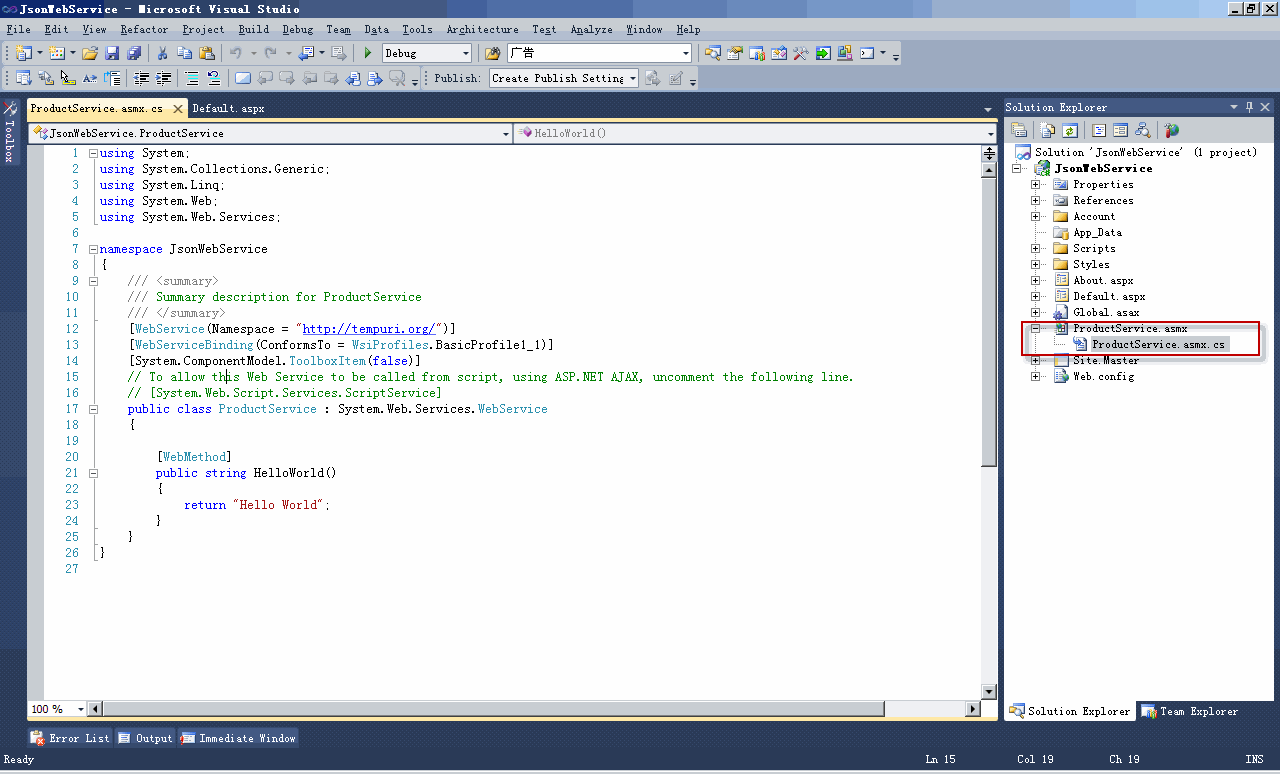
此Web Service调用ProductData类,完成数据查询操作,具体代码如下:
/// <summary> /// Summary description for ProductService /// </summary> [WebService(Namespace = "http://tempuri.org/")] [WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)] [System.ComponentModel.ToolboxItem(false)] // To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line. [System.Web.Script.Services.ScriptService] public class ProductService : System.Web.Services.WebService { private ProductData dataSource { get { return new ProductData(); } } /// <summary> /// Get the Products /// </summary> /// <returns></returns> [WebMethod] [ScriptMethod(ResponseFormat=ResponseFormat.Json,UseHttpGet=true)] public List<Product> GetProducts() { return dataSource.GetProducts(); } /// <summary> /// 根据商品Id查询商品 /// </summary> /// <param name="object">商品编号</param> /// <returns>商品</returns> [WebMethod] [ScriptMethod(ResponseFormat=ResponseFormat.Json,UseHttpGet=true)] public Product GetProductById(object id) { Product p = null; if (id!=null) { int productId = 0; int.TryParse(id.ToString(),out productId); p = dataSource.GetProductById(productId); } return p; } /// <summary> /// 根据商品名称查询商品 /// </summary> /// <param name="id">商品名称</param> /// <returns>商品</returns> [WebMethod] [ScriptMethod(ResponseFormat = ResponseFormat.Json, UseHttpGet = true)] public List<Product> GetProductById(string name) { return dataSource.GetProductByName(name); } } } |
Ø 引用命名空间:System.Web.Script.Services.ScriptService
Ø 给方法添加注解:[ScriptMethod(ResponseFormat = TheResponseFormat, UseHttpGet = true/false)]
ResponseFormat:方法要返回的类型,一般为Json或者XML
UseHttpGet:true表示“Get”可以访问此方法
5、 在Defualt.aspx页面引用Jquery类库
引用Google网络jquery 类库http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.js
或者本地类库<script src="Scripts/jquery-1.4.1.js" type="text/javascript"></script>
6、 在Defualt.aspx页添加如下HTML代码:
<div id="listContainer" class="container"> <div id="title"> Product List</div> <table id="productList"> <thead> </thead> <tbody> </tbody> </table> </div> |
7、 在Defualt.aspx页添加如下样式代码:
<style type="text/css"> body { font-family: Verdana,Arial; } .container { width: auto; padding: 1.5em; margin: 1em; } .container #title { font-size: 2em; width: 100%; border-bottom: groove 0.5em blue; } #productList { width: 100%; border-collapse: collapse; } #productList td { padding: 1em; border-style: solid; border-width: 1px; border-color: Blue; margin: 0; } thead td { background-color: Yellow; } tbody tr:hover { background-color: #aaa; } </style> |
$(document).ready(function () { // Get Product list $.ajax({ type: "GET", contentType: "application/json; charset=utf-8", url: "ProductService.asmx/GetProducts", dataType: "json", success: insertCallback, error: errorCallback }); function insertCallback(result) { $("#productList").find("tr:gt(0)").remove(); if (result["d"].length > 0) { $('#productList > thead:last').append('<tr><td>商品名称</td><td>价格</td><td>库存量</td></tr>'); } for (var i = 0; i < result["d"].length; i++) { var product = eval(result["d"][i]); $('#productList > tbody:last').append('<tr onclick="ShowDetail(' + product.ProductId + ')"><td>' + product.ProductName + '</td><td>' + product.Price + '</td><td>' + product.Stock + '</td></tr>'); } } function errorCallback(XMLHttpRequest, textStatus, errorThrown) { alert(errorThrown + ':' + textStatus); } |
$.ajax方法有以下属性:
Type: HTTP请求方法,在做查询操作时,经常用Get方法
contentType:在请求头部的类型,由于Web Service返回Json对象,此处值为:application/json; charset=utf-8
url:请求的URL地址
dataTyep:定义返回的类型
Success:调用成功时,要执行的方法
error:调用失败是,要执行的方法
9、 执行程序,效果如下:
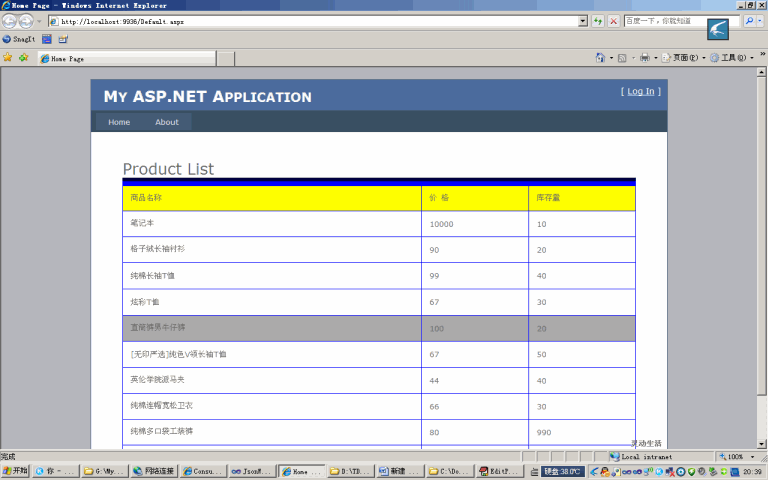
至此,使用Jquery查询Web Service返回的JSon对象已经完成
源代码下载地址:http://files.cnblogs.com/ywqu/JsonWebService.rar
相关文章推荐
- [入门篇]Jquery读取.Net WebService Json数据
- [入门篇]Jquery读取.Net WebService Json数据
- [转载]Jquery读取.Net WebService Json数据
- [入门篇]Jquery读取.Net WebService Json数据
- 利用JQuery jsonp实现Ajax跨域请求 .Net 的*.handler 和 WebService,返回json数据
- 利用JQuery jsonp实现Ajax跨域请求 .Net 的*.handler 和 WebService,返回json数据
- 利用JQuery jsonp实现Ajax跨域请求 .Net 的*.handler 和 WebService,返回json数据
- 利用JQuery jsonp实现Ajax跨域请求 .Net 的*.handler 和 WebService,返回json数据
- jquery简单ajax示例_读取json文件数据
- jQuery调用WebService返回JSON数据
- jQuery调用WebService返回JSON数据
- jquery $.getJSON 与.NET 结合用法推荐
- IT咨询顾问:一次吐血的项目救火 java或判断优化小技巧 asp.net core Session的测试使用心得 【.NET架构】BIM软件架构02:Web管控平台后台架构 NetCore入门篇:(十一)NetCore项目读取配置文件appsettings.json 使用LINQ生成Where的SQL语句 js_jquery_创建cookie有效期问题_时区问题
- JQuery读取Json数据之解析多维Json数据格式
- asp.net 使用Jquery 调用WebService返回JSON 类型数据
- jquery调用基于.NET Framework 3.5的WebService返回JSON数据 (转)
- jQuery读取JSON数据
- Jquery 调用.net WebService 返回Json、XML方法
- .NET读取json数据并绑定到对象